In C++, you can replace a specific character in a string using the `std::string::replace()` method or by using a simple loop to iterate through the string.
Here’s a code snippet demonstrating how to replace all occurrences of a character in a string:
#include <iostream>
#include <string>
int main() {
std::string str = "hello world";
char oldChar = 'o';
char newChar = 'a';
for (char& c : str) {
if (c == oldChar) {
c = newChar;
}
}
std::cout << str << std::endl; // Output: hella warld
return 0;
}
Understanding C++ Strings
What are C++ Strings?
In C++, strings are represented using the `std::string` class, which is provided by the Standard Library. This class allows you to work with sequences of characters more easily than traditional C-style strings (character arrays).
Advantages of `std::string` include:
- Dynamic sizing: Strings can grow and shrink in size as needed.
- Built-in member functions make string manipulation straightforward.
- It manages memory automatically, which helps prevent common issues like buffer overflows.
Basics of String Operations in C++
Before diving into character replacement, it's essential to know the basic operations available for `std::string`. These include concatenation, determining the string length, and accessing specific characters. You'll typically include the `<string>` and `<iostream>` libraries to use these features effectively.
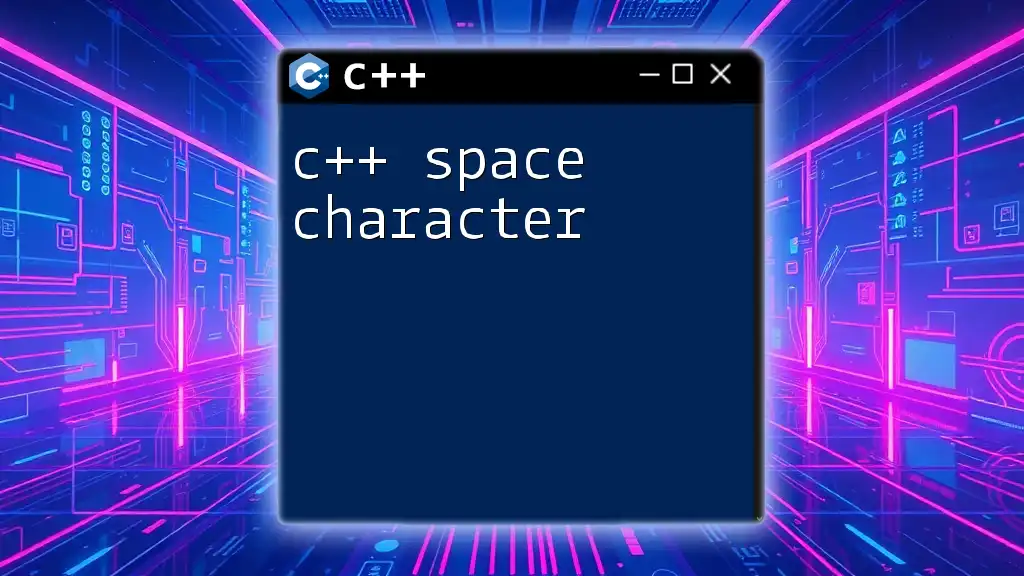
C++ String Replacement Methods
Why Replace Characters in Strings?
Replacing characters in a string is a common task in software development. For instance:
- Correcting user input: Automatically replace certain characters to maintain data integrity.
- Text processing: Modifying strings for display or reporting purposes.
- Data transformation: Changing delimiters in data files from one character to another.
Introduction to C++ String Replacement Functions
C++ provides several functions to replace characters in strings. Understanding these functions is crucial for efficient string manipulation.
Overview of Standard Functions
Key functions to note include:
- `std::string::replace`
- `std::string::find`
These functions form the cornerstone of string replacement in C++.
Using std::string::replace
Syntax of std::string::replace
The `replace` function has the following syntax:
str.replace(pos, len, str);
- pos: The starting position to begin replacing characters.
- len: The number of characters to replace.
- str: The string to insert in place of the removed characters.
Code Snippet Example
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
str.replace(6, 5, "C++");
std::cout << str; // Output: Hello C++!
return 0;
}
In this example, we replace "World" (5 characters starting from position 6) with "C++". The output reflects the change, clearly illustrating how `replace` functions.
Using std::string::find and std::string::replace
Searching for Character(s)
To perform more nuanced replacements, such as replacing only certain occurrences of a character, you can combine `find` and `replace`. This method requires first locating the target character and then replacing it.
Code Snippet Example
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
size_t pos = str.find("o");
while (pos != std::string::npos) {
str.replace(pos, 1, "C++"); // Replace single character
pos = str.find("o", pos + 1);
}
std::cout << str; // Output: HellC++ WCrld!
return 0;
}
In this snippet, we first find the position of "o", replace it with "C++", and then look for the next occurrence until none remain. This showcases how to replace specific characters effectively using a loop.
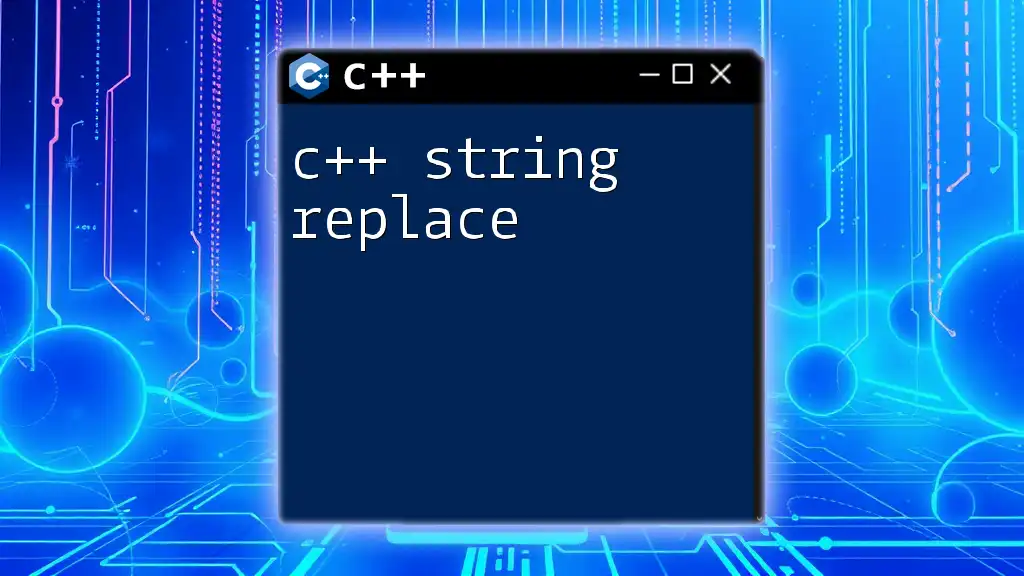
Handling Multiple Replacements
Replacing All Occurrences of a Character
In some situations, you might want to replace all occurrences of a character within a string. A common approach involves looping through the entire string.
Code Snippet Example
#include <iostream>
#include <string>
void replaceAll(std::string &str, char oldChar, char newChar) {
for (size_t i = 0; i < str.size(); ++i) {
if (str[i] == oldChar) {
str[i] = newChar;
}
}
}
int main() {
std::string str = "Hello World!";
replaceAll(str, 'o', 'C++');
std::cout << str; // Output: HellC++ WCrld!
return 0;
}
Here, the `replaceAll` function scans through the string, checking each character. When a match is found, it replaces it with `newChar`. This method is efficient for bulk replacements, as it performs a straightforward scan of the string.
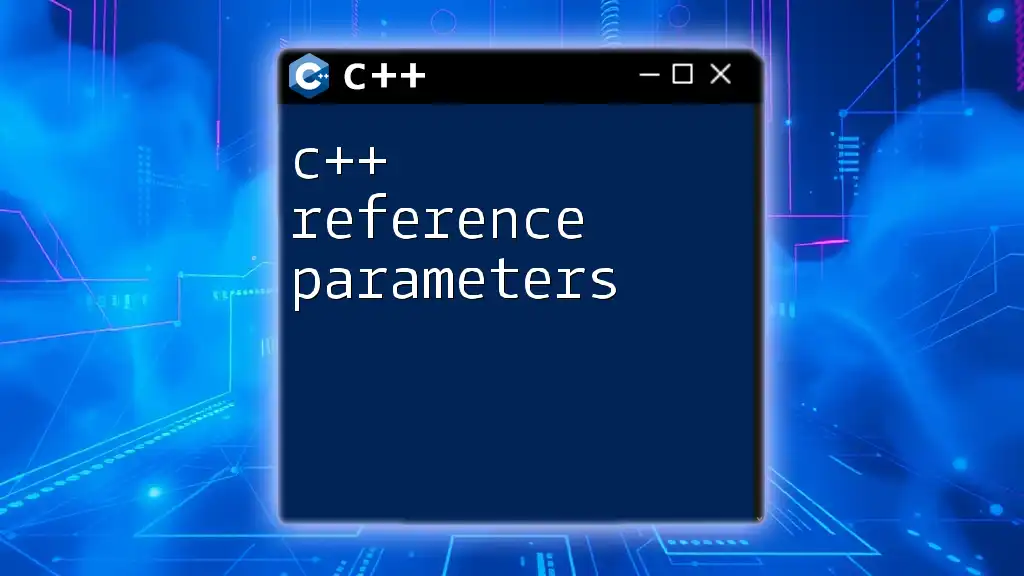
Best Practices for String Replacement in C++
Performance Considerations
When working with strings, particularly in performance-sensitive applications, it’s essential to keep efficiency in mind. Repeatedly modifying strings can lead to unnecessary allocations and deletions.
Best practices include:
- Minimizing copies by working with references when possible.
- Using functions like `reserve()` to allocate enough memory upfront.
Avoiding Common Pitfalls
While replacing characters can seem straightforward, several common mistakes can arise:
- Forgetting to manage string sizes when using `replace`.
- Misusing `find` and `replace`, potentially leading to infinite loops or missed replacements.
Tips for debugging:
- Print intermediate results to verify the correctness of replacements.
- Test with various inputs to cover edge cases.
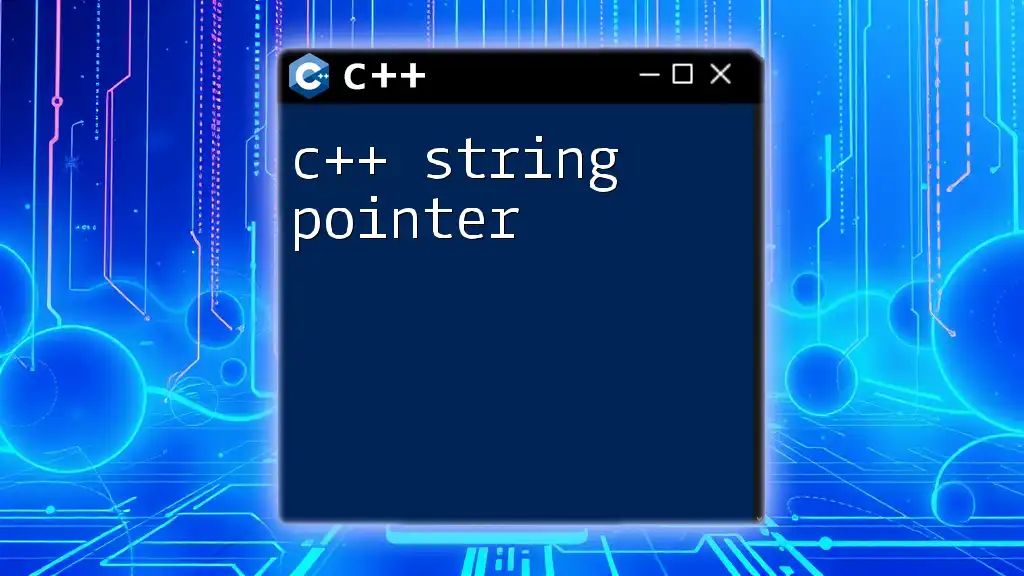
Conclusion
In this guide, we explored the essentials of C++ string replacement, emphasizing the various functions available for effectively replacing characters in strings. Understanding how to manipulate strings is invaluable for any developer working with text data.
As you become more proficient in C++, consider practicing by tackling various string manipulation challenges. You'll find that mastering these techniques not only improves your coding skills but also enhances your overall problem-solving capabilities.
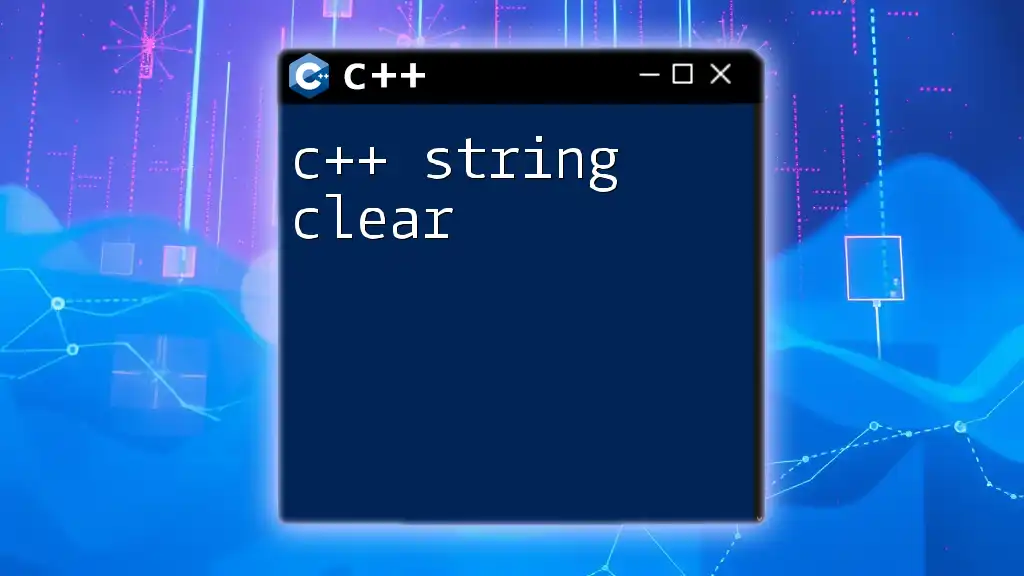
Additional Resources
To continue your journey in mastering C++ strings, consider diving into recommended readings and tutorials. The official C++ documentation is also an invaluable resource for understanding the `std::string` class in-depth.
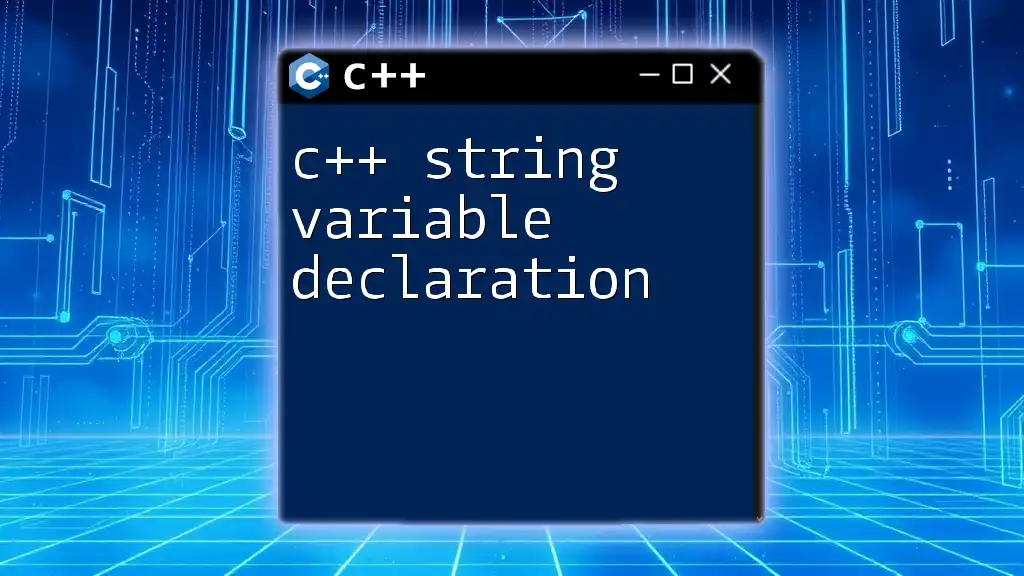
Call to Action
If you're eager to dive deeper into the world of C++, join our courses for hands-on learning about string manipulation and much more. Feel free to share your experiences or any questions you may have in the comments section!