A C++ `reference_wrapper` is a utility that allows you to store and manipulate references as if they were objects, enabling you to use references in standard containers like `std::vector`.
#include <iostream>
#include <functional>
#include <vector>
int main() {
int x = 10;
std::reference_wrapper<int> ref_x = std::ref(x);
std::vector<std::reference_wrapper<int>> vec;
vec.push_back(ref_x);
// Modifying through the reference wrapper
vec[0].get() = 20;
std::cout << "x after modification: " << x << std::endl; // Outputs: 20
return 0;
}
Understanding C++ References
What are References?
In C++, a reference acts as an alias for another variable, allowing you to refer to the same memory location without needing to use pointers. Unlike pointers, which can be null and require explicit dereferencing, references must be initialized when declared and cannot become null after that. This guarantees that a reference will always refer to an existing object.
Differences Between Pointers and References
- Syntax: References are simpler as they do not require dereferencing (e.g., you access them using just the variable name, not `*`).
- Nullability: A pointer can point to `nullptr`, while a reference must always reference a valid object.
- Rebinding: Once a reference is initialized, it cannot be changed to refer to another variable, while pointers can be reassigned to point to different memory locations.
Advantages of Using References
- Clarity: Code using references can be easier to read and understand because the syntax is cleaner.
- Safety: References provide a layer of safety from null access errors that can occur with pointers.
How References Work
C++ references operate on reference semantics, meaning that when you pass a reference to a function, you are passing the address of the variable rather than its value. This allows for efficient manipulation of large objects without the overhead of copying them. The lifetime of the reference is tied to the lifetime of the object it references.
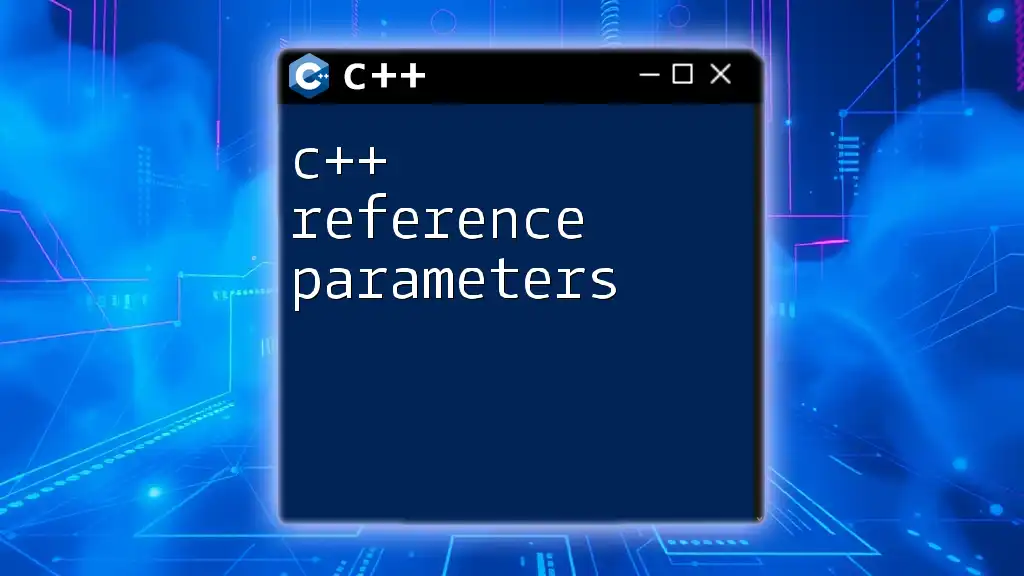
The std::reference_wrapper
Introduction to std::reference_wrapper
The `std::reference_wrapper` class is part of the C++ Standard Library, included in the `<functional>` header. It provides a way to store references in a manner that allows them to be copied, thus enhancing their utility, especially in standard library containers and algorithms.
Creating a Reference Wrapper
To create a reference wrapper, you can use the `std::ref` function, which returns a `std::reference_wrapper` object.
Example: Wrapping a reference
#include <iostream>
#include <functional>
int main() {
int a = 10;
std::reference_wrapper<int> refWrapper = std::ref(a);
std::cout << refWrapper.get() << std::endl; // Output: 10
return 0;
}
In this example, `std::ref(a)` creates a reference wrapper around the variable `a`, allowing easy access while maintaining reference semantics.
Accessing the Wrapped Reference
You can access the wrapped reference using the `.get()` method to retrieve the original variable, or you can simply cast it to the original type to use it directly.
Example: Accessing and modifying the original variable
refWrapper.get() = 20;
std::cout << a; // Output: 20
This demonstrates the ability to modify the original variable through the reference wrapper.
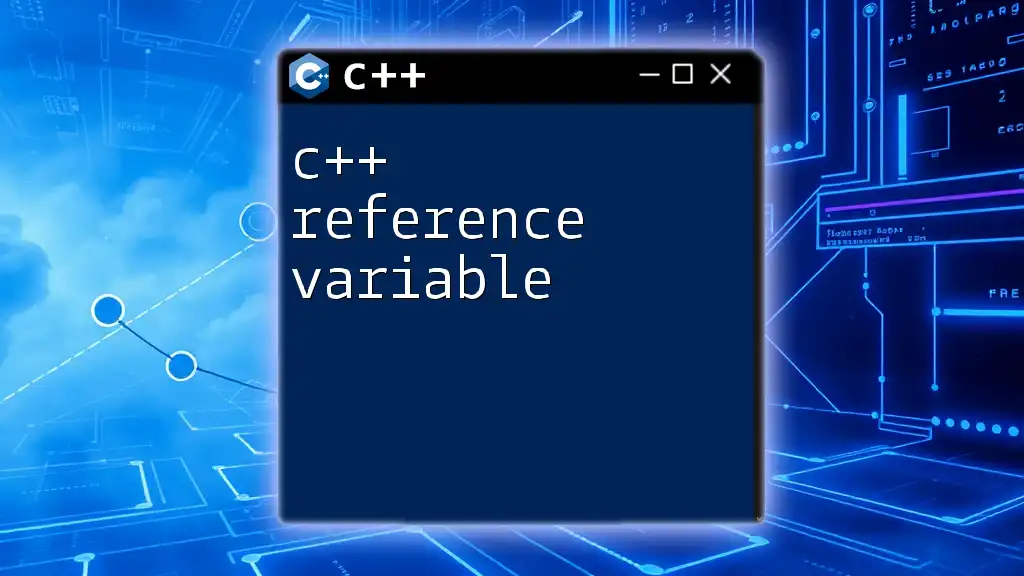
Use Cases of Reference Wrapper in C++
Generic Programming
The `std::reference_wrapper` is particularly useful in generic programming. When you want to write template functions that accept references, you can ensure that those references remain valid by using `std::reference_wrapper`.
Example: Passing by reference in template functions
template <typename T>
void display(std::reference_wrapper<T> ref) {
std::cout << ref.get() << std::endl;
}
This template function will accept a `std::reference_wrapper` and will print the value of the referenced variable.
In STL Containers
C++ STL containers generally store values, not references. By using `std::reference_wrapper`, you can effectively store references in containers like `std::vector` or `std::list`.
Example: Storing references in `std::vector`
std::vector<std::reference_wrapper<int>> vec;
vec.push_back(std::ref(a));
This allows you to manage a collection of references without copying the actual values, providing both efficiency and simplicity.
Storing in Maps or Sets
When using associative containers such as `std::unordered_map` or `std::set`, `std::reference_wrapper` is beneficial to manage references.
Example: Using reference wrappers in `std::unordered_map`
std::unordered_map<int, std::reference_wrapper<int>> myMap;
myMap[1] = std::ref(a);
Here, `myMap` maps an integer key to a reference of an integer, maintaining the integrity of the original data.
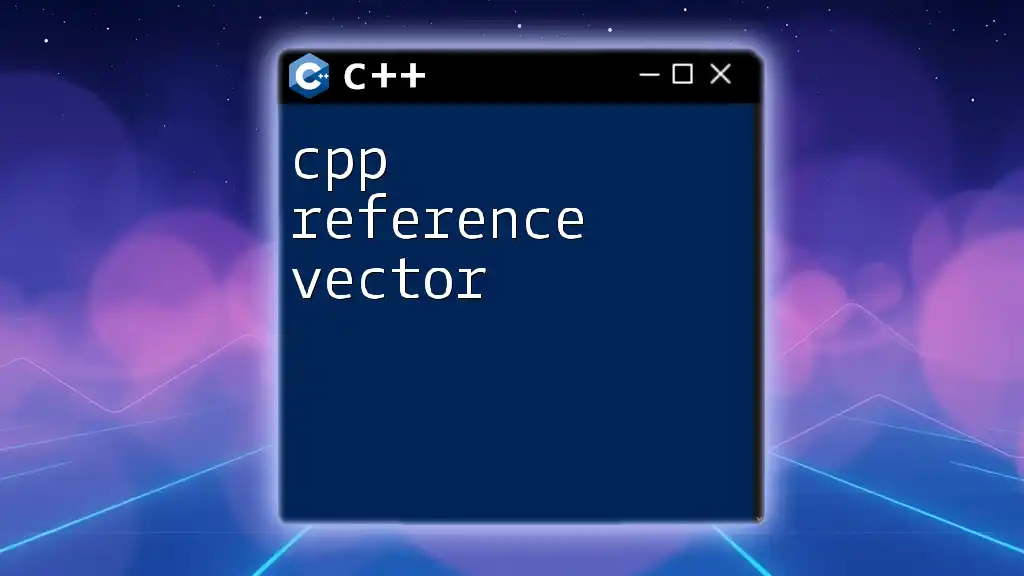
Advantages of Using Reference Wrapper
Simplifying Reference Management
Managing references conveniently with `std::reference_wrapper` offers significant benefits. With it, you can avoid issues related to temporary objects and dangling references that may occur in complex codebases.
Maintaining State Across Contexts
Using reference wrappers allows you to maintain state when employing callback functions. In scenarios where you pass arguments, the original variable remains accessible, leading to elegant and effective solutions.
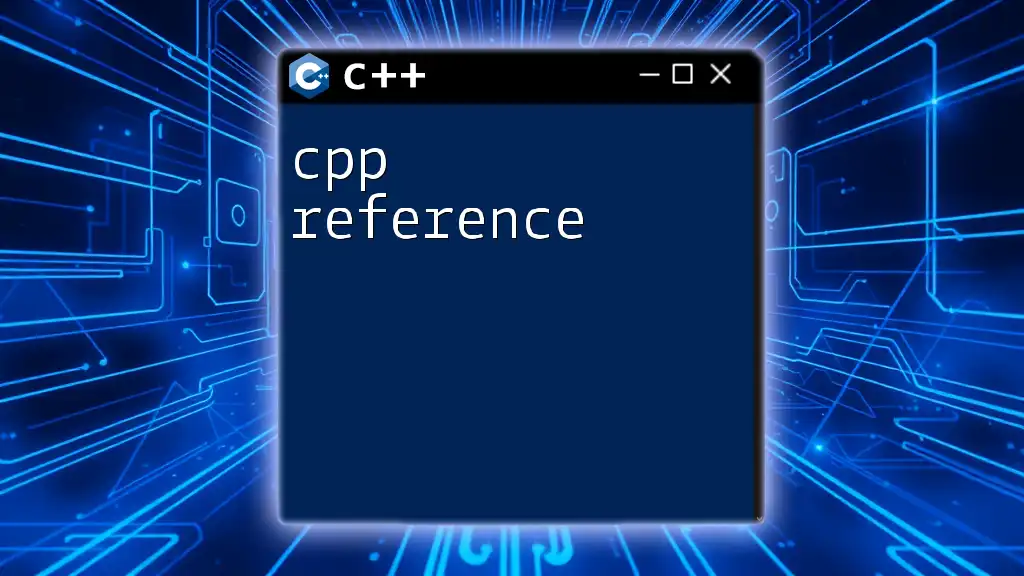
Limitations of Reference Wrapper
Non-Copyable Semantics
While `std::reference_wrapper` is beneficial, it’s crucial to note that references cannot be copied. Cloning a `std::reference_wrapper` will not create a new reference, and any assignment will lead to a potential dangling reference if not managed carefully.
Possible Pitfalls
One major risk of using `std::reference_wrapper` is creating dangling references. This occurs when the original variable goes out of scope while there is still an active reference to it. Always ensure that the lifetime of the referenced object extends beyond the lifetime of the reference wrapper to avoid undefined behavior.
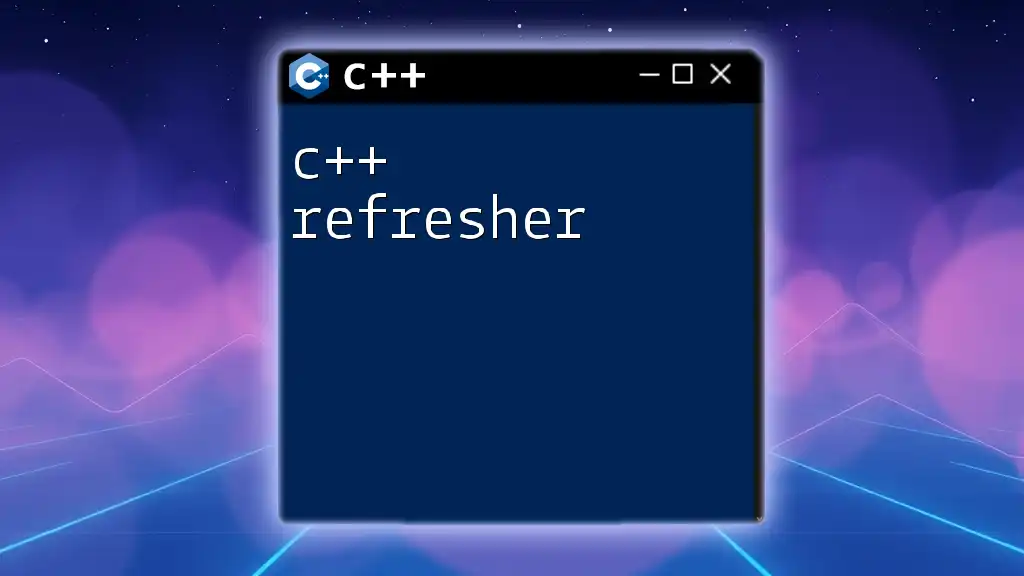
Best Practices for Using Reference Wrapper
When to Use Std::reference_wrapper
It's advisable to use `std::reference_wrapper` when you need the properties of references (like no copies) while handling containers or algorithms that require copyable types. This makes it particularly useful in generic programming and when working with STL.
Best Use Cases:
- When storing references in STL containers
- When passing references to functions in a more flexible manner
Performance Considerations
Generally, using reference wrappers incurs minimal performance overhead compared to directly using references. They allow for seamless handling of references, especially in more complex data structures and algorithms.
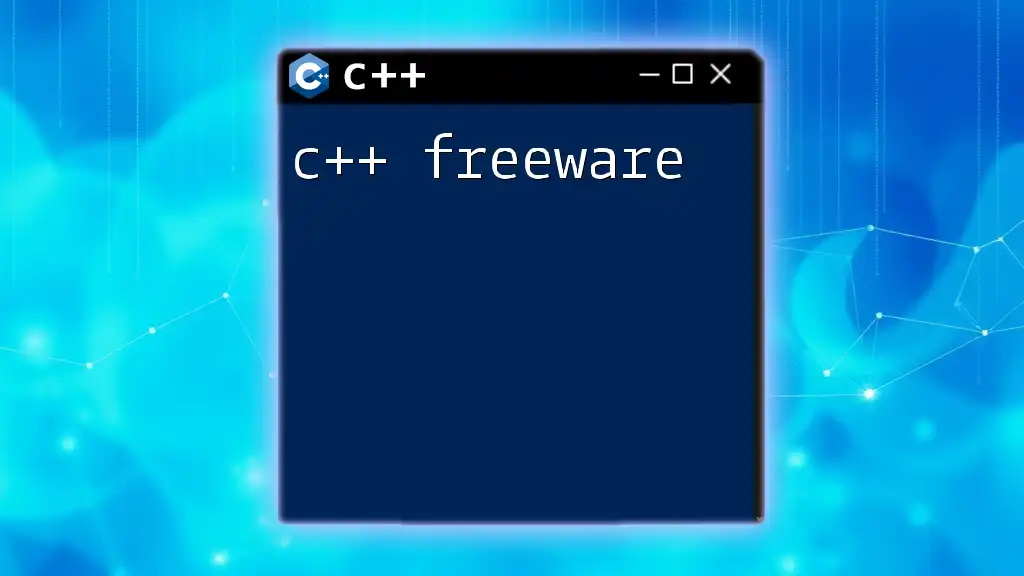
Conclusion
Utilizing `std::reference_wrapper` is a powerful addition to your C++ toolkit. By enabling you to store and work with references effectively, they improve the versatility of your code while maintaining clarity and efficiency. Understanding how to harness its full potential can significantly enhance your programming endeavors.
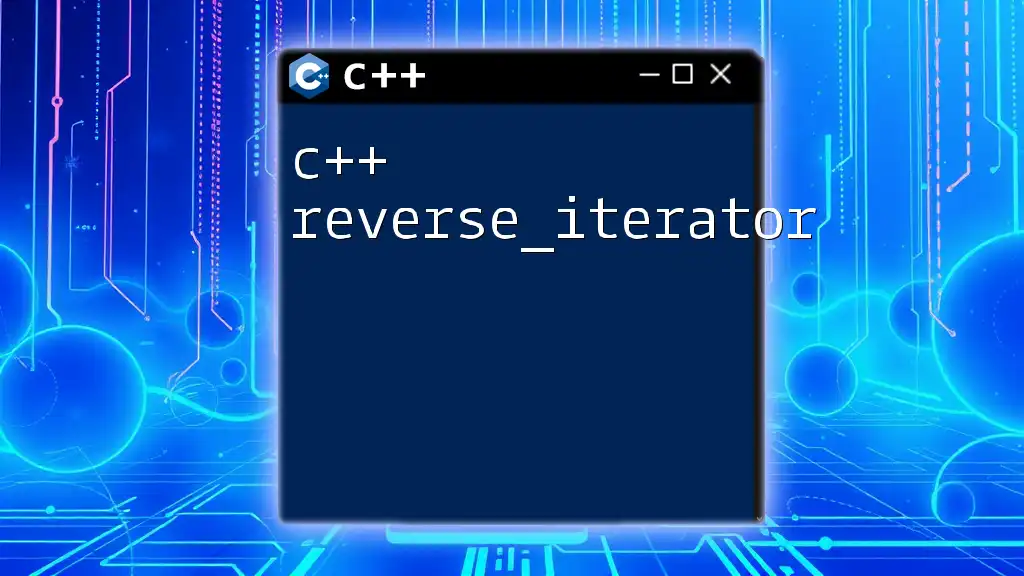
Additional Resources
For further reading and exploration, consider reviewing the official C++ documentation for the `std::reference_wrapper` class. Additionally, various books and tutorials go in-depth into reference semantics and their applications in the language.
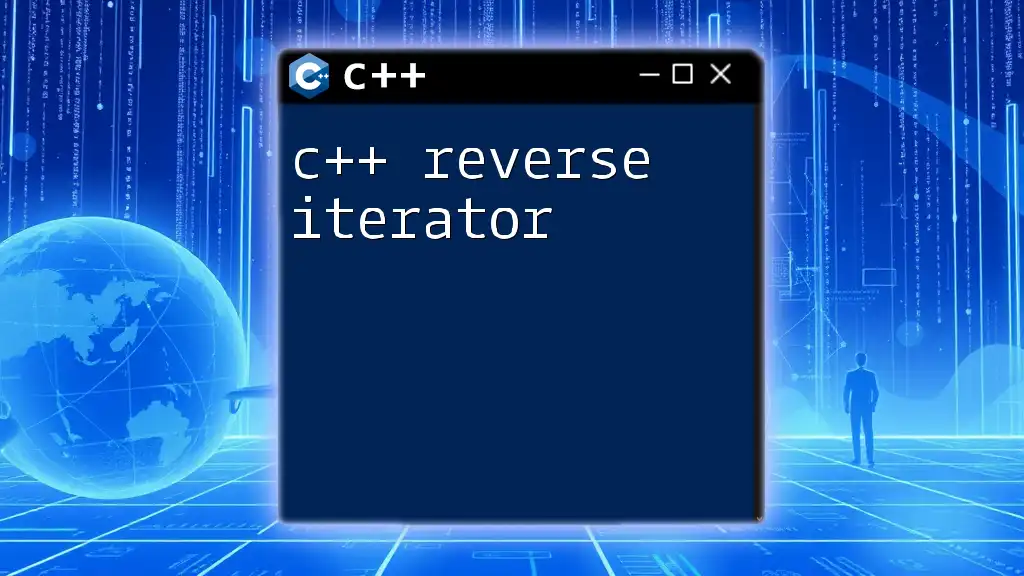
FAQs about C++ Reference Wrapper
Common Questions
-
What happens if I copy a `std::reference_wrapper`? Copying a `std::reference_wrapper` creates a reference to the same object, not a new reference, so care must be taken to avoid dangling references.
-
Can I use `std::reference_wrapper` with any type? Yes, `std::reference_wrapper` can wrap any type, including user-defined types.
-
Are there alternatives to `std::reference_wrapper`? Depending on your needs, you might also consider using smart pointers (`std::shared_ptr`, `std::unique_ptr`) where ownership semantics are more appropriate.