A C++ refresher provides a quick overview of essential C++ concepts and syntax that are crucial for beginners to understand and effectively utilize the programming language.
Here's a simple code snippet that demonstrates a basic C++ program structure:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
C++ Fundamentals
Basic Syntax
In C++, every program starts with the `main()` function, which serves as the entry point. Additionally, you will need to include headers for necessary libraries, and use namespaces to avoid name conflicts. Here’s how a basic C++ program looks:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This program includes the iostream library, prints "Hello, World!" to the console, and returns `0` to indicate successful execution. Understanding how to structure your program is fundamental to utilizing C++ effectively.
Data Types
C++ supports multiple data types which can be categorized as built-in or user-defined.
Built-in Data Types
- int: for integers.
- float: for single-precision floating-point numbers.
- double: for double-precision floating-point numbers.
- char: for single characters.
- bool: for boolean values (true or false).
Here’s an example of declaring and initializing different data types:
int age = 30;
double salary = 56000.50;
char initial = 'A';
bool isEmployed = true;
Understanding these data types allows you to effectively store and manipulate data in your applications.
User-defined Data Types
C++ allows you to create your own types using `struct`, `enum`, and `union`. For instance, you can create a struct to encapsulate related information:
struct Person {
std::string name;
int age;
};
Person john;
john.name = "John Doe";
john.age = 25;
Using user-defined types enhances the organization and readability of your code, especially when dealing with complex data.
Control Structures
Control structures enable you to dictate the flow of your program.
Conditional Statements
The conditional structure using `if`, `else if`, and `else` allows for decision-making in your code. For example:
if (age < 18) {
std::cout << "Minor";
} else {
std::cout << "Adult";
}
This code checks the age and prints whether the person is a minor or an adult.
Loops
Loops allow you to execute a block of code multiple times. In C++, you can use `for`, `while`, and `do-while` loops. Here’s a simple example using a `for` loop:
int arr[] = {1, 2, 3, 4, 5};
for(int i = 0; i < 5; i++) {
std::cout << arr[i] << std::endl;
}
This loop iterates over an array and prints each element, making it essential for tasks that require repetition.
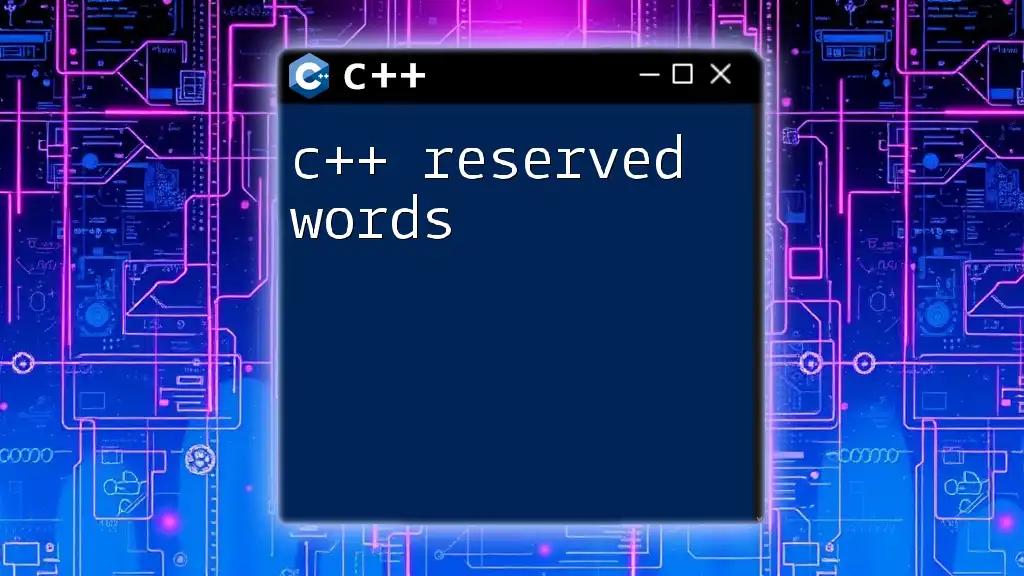
Object-Oriented Programming Concepts
Classes and Objects
C++ is an object-oriented programming language, which means it focuses on using classes and objects. A class serves as a blueprint for creating objects. Here’s how you can define a class:
class Car {
public:
std::string brand;
void honk() {
std::cout << "Beep beep" << std::endl;
}
};
In the example above, the `Car` class has a property called `brand` and a method `honk()`. When you instantiate an object of this class, you can access its features:
Car myCar;
myCar.brand = "Toyota";
myCar.honk();
Understanding classes and objects is crucial for encapsulating functionality and data.
Inheritance
Inheritance allows you to create new classes based on existing ones. This helps in reusing code and establishing a relationship between classes. In C++, you can have single or multiple inheritance. Here’s a simple example:
class Vehicle {
public:
void start() {
std::cout << "Vehicle started";
}
};
class Bike : public Vehicle {
public:
void pedal() {
std::cout << "Biking";
}
};
In this example, `Bike` is a derived class of `Vehicle`. It inherits the `start()` method, enabling you to use it without rewriting the code.
Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon. It comes in two flavors: function overloading and operator overloading. With function overloading, you can write two functions with the same name but different implementations based on their parameters:
int add(int x, int y) {
return x + y;
}
double add(double x, double y) {
return x + y;
}
This allows the function `add()` to behave differently according to the argument types.
Another important aspect is virtual functions, which facilitate runtime polymorphism. Here’s an example:
class Base {
public:
virtual void show() {
std::cout << "Base class" << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived class" << std::endl;
}
};
By marking the function in the base class as `virtual`, you can override it in the derived class, allowing for more flexible implementations.
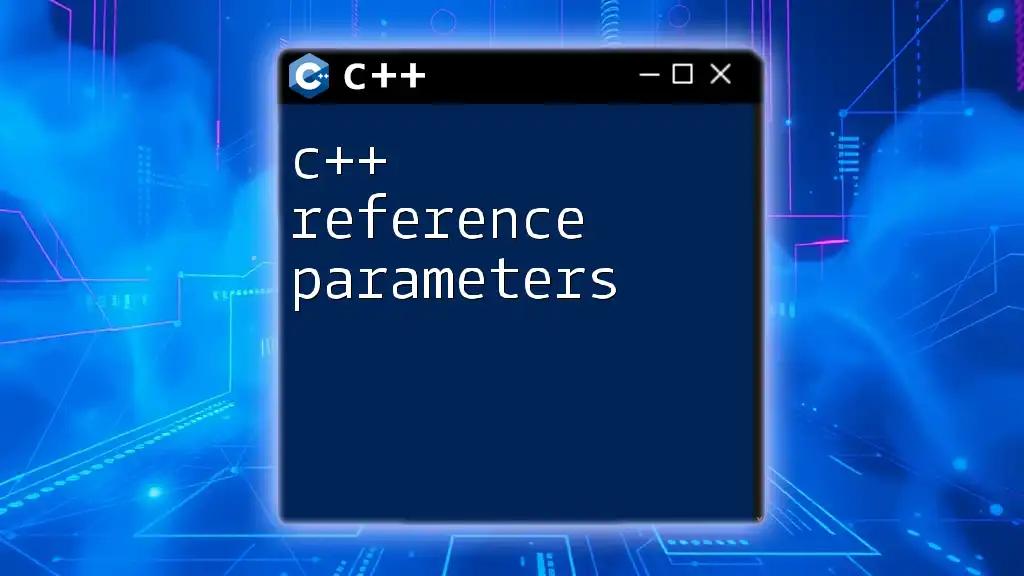
Advanced C++ Concepts
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful feature of C++ that provides templates for data structures and algorithms, enhancing code reusability and efficiency. The key components of STL include containers, iterators, algorithms, and function objects.
Using `vector`
One of the most commonly used containers in STL is the `vector`. It provides dynamic array functionality. Here’s an example of creating and manipulating a vector:
#include <vector>
std::vector<int> nums = {1, 2, 3};
nums.push_back(4);
Vectors automatically manage memory dynamically, making them a preferred choice for many applications.
Exception Handling
C++ offers exception handling mechanisms to manage errors gracefully through the use of `try`, `catch`, and `throw`. By handling exceptions, you can prevent your program from crashing and provide useful feedback to the user.
Here’s a simple example:
try {
throw std::runtime_error("An error occurred");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl;
}
Using exception handling effectively makes your code robust and reliable, important aspects of any successful application.
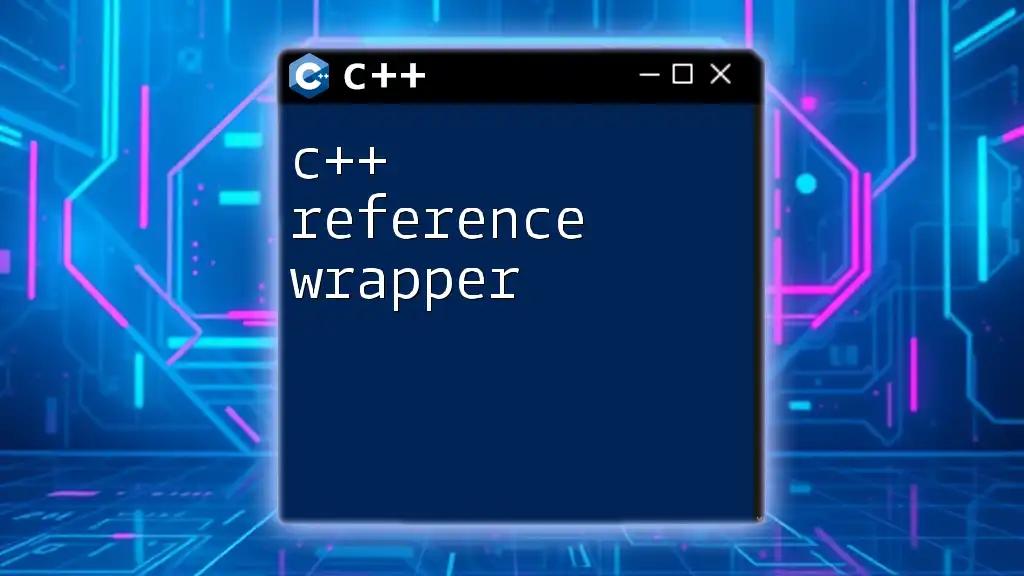
Conclusion
In this C++ refresher, we've covered vital concepts ranging from basic syntax to advanced techniques, including object-oriented programming and the use of the STL. Understanding these principles will not only enhance your C++ skills but also empower you to develop more complex and efficient applications.
Further Learning Resources
To deepen your understanding of C++, consider exploring books, websites, and online courses that provide extensive tutorials and challenges for better learning.
Call to Action
We encourage you to take your C++ learning journey further! Join a course or training session to enhance your skills and network with fellow C++ enthusiasts. Stay updated by signing up for our newsletter or following us on social media!