A C++ web server is an application that handles HTTP requests and responses, allowing clients to communicate with it over the web, often implemented using libraries like Boost.Asio or HTTPServer.
Here’s a simple example of a C++ web server using the Boost.Beast library:
#include <beast/core.hpp>
#include <beast/http.hpp>
#include <beast/version.hpp>
#include <boost/asio.hpp>
#include <iostream>
namespace beast = boost::beast;
namespace http = beast::http;
namespace net = boost::asio;
int main() {
try {
net::io_context io_context{1};
net::ip::tcp::acceptor acceptor{io_context, {net::ip::tcp::v4(), 8080}};
for (;;) {
net::ip::tcp::socket socket{io_context};
acceptor.accept(socket);
beast::flat_buffer buffer;
http::request<http::string_body> req;
http::read(socket, buffer, req);
http::response<http::string_body> res{http::status::ok, req.version()};
res.set(http::field::server, "C++ Beast");
res.set(http::field::content_type, "text/plain");
res.body() = "Hello, World!";
res.prepare_payload();
http::write(socket, res);
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << "\n";
}
}
Understanding the Basics of Web Servers
What is a Web Server?
A web server is a software or hardware system responsible for accepting requests from clients (typically browsers) and serving them content over the internet. The primary function of a web server is to handle HTTP requests from clients, process these requests, and return the requested resources, such as HTML pages, images, or other data.
Understanding the difference between static and dynamic content is crucial. Static content is fixed and does not change based on user input, while dynamic content changes based on various factors, such as user preferences, data inputs, or other external conditions. C++ web servers can efficiently serve both types of content, making them versatile in various application scenarios.
Common Protocols
HTTP and HTTPS
The Hypertext Transfer Protocol (HTTP) is the fundamental protocol used for transmitting messages and data across the web. It facilitates communication between clients and servers and is the foundation upon which data exchange on the web is built. HTTPS (HTTP Secure) adds a layer of security by encrypting the communication channel, ensuring that sensitive information remains private.
TCP/IP Fundamentals
At the core of web communication is the TCP/IP protocol suite. TCP (Transmission Control Protocol) is responsible for establishing and maintaining a reliable connection, while IP (Internet Protocol) handles addressing and routing of packets. Understanding these protocols is essential when building a C++ web server, as they are the means by which data travels across the internet.
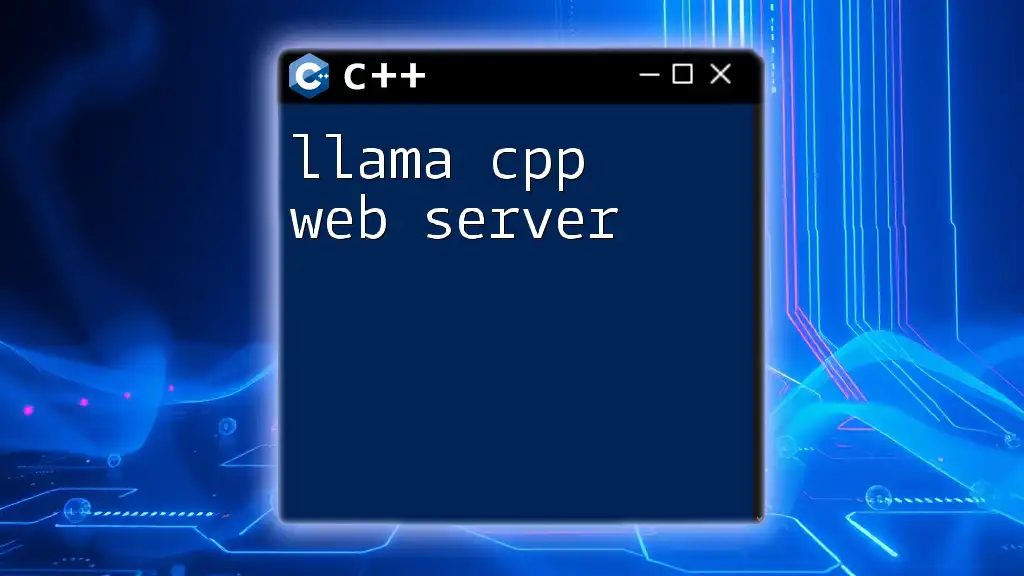
Setting Up Your Development Environment
Choosing the Right Tools
C++ Compiler Options
When developing a C++ web server, choosing the right compiler is critical. Popular compilers include GCC (GNU Compiler Collection), Clang, and MSVC (Microsoft Visual C++). Each has its advantages, depending on your operating system and development requirements.
IDE Recommendations
A robust Integrated Development Environment (IDE) can streamline development. Consider using renowned IDEs such as Visual Studio, Code::Blocks, or CLion for their features, including debugging capabilities, code completion, and project management tools.
Installing Required Libraries
Boost.Asio for Networking
To facilitate network communication in your C++ web server, you'll need a library that simplifies tasks such as socket programming. Boost.Asio is an excellent choice, as it provides a consistent asynchronous IO model. Installation can typically be accomplished through package managers or by downloading the source code directly from the Boost website.
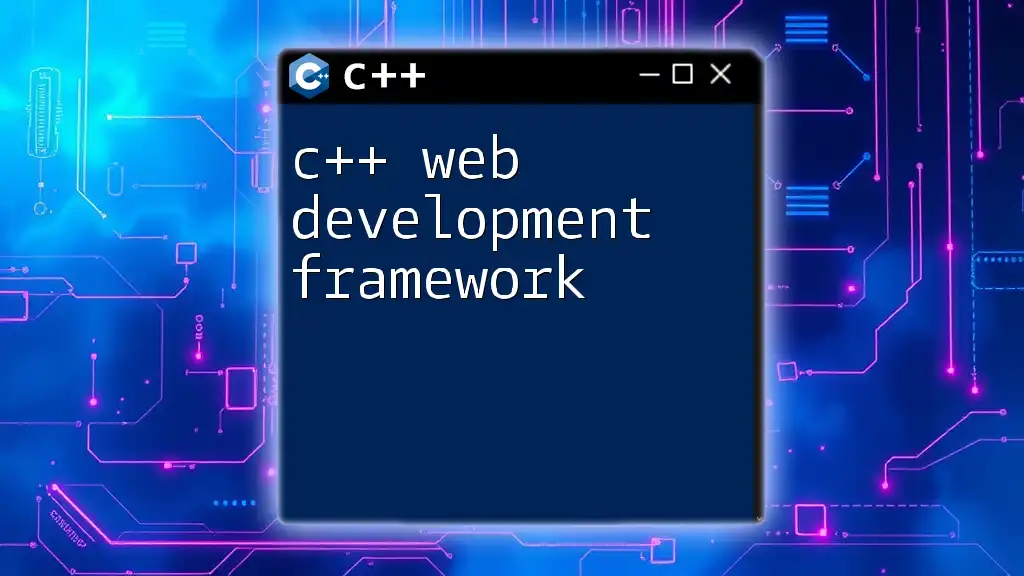
Building Your First C++ Web Server
Setting Up the Project Structure
A well-organized project is the foundation of successful development. It’s beneficial to follow a logical file organization structure. For instance:
- `/src`: Source files
- `/include`: Header files
- `/build`: CMake build files
Additionally, creating a CMakeLists.txt file will help manage the build process efficiently.
Writing the Server Code
Creating a Simple HTTP Server
Start your C++ web server by setting up a basic HTTP server structure. Below is a sample implementation using Boost.Asio:
#include <iostream>
#include <boost/asio.hpp>
using namespace std;
using boost::asio::ip::tcp;
int main() {
try {
boost::asio::io_context io_context;
tcp::acceptor acceptor(io_context, tcp::endpoint(tcp::v4(), 8080));
while (true) {
tcp::socket socket(io_context);
acceptor.accept(socket);
// Handling client connection
}
} catch (std::exception& e) {
std::cerr << "Exception: " << e.what() << "\n";
}
return 0;
}
Explanation of Code Structure: The code starts by initializing an io_context, which is essential for asynchronous operations. The acceptor listens for incoming connections on port 8080, and the server will continue to accept client connections in a loop.
Handling Client Requests
Parsing HTTP Requests
Understanding the request format is vital for processing incoming data. Here is a basic code snippet to read the client's request:
std::string request;
boost::asio::read_until(socket, boost::asio::buffer(request), "\r\n");
std::cout << "Received: " << request << std::endl;
In this example, we read from the socket until a newline character is encountered, indicating the end of the HTTP header. It’s essential to log received requests for debugging and monitoring purposes.
Sending HTTP Responses
Once a request is parsed, the server should respond accordingly. Here’s a code snippet to send a simple HTML response:
std::string response = "HTTP/1.1 200 OK\r\nContent-Type: text/html\r\n\r\n<html><body><h1>Hello, World!</h1></body></html>";
boost::asio::write(socket, boost::asio::buffer(response));
This response code indicates a successful request and includes a minimal HTML payload. It shows how easily C++ can handle responses to clients.
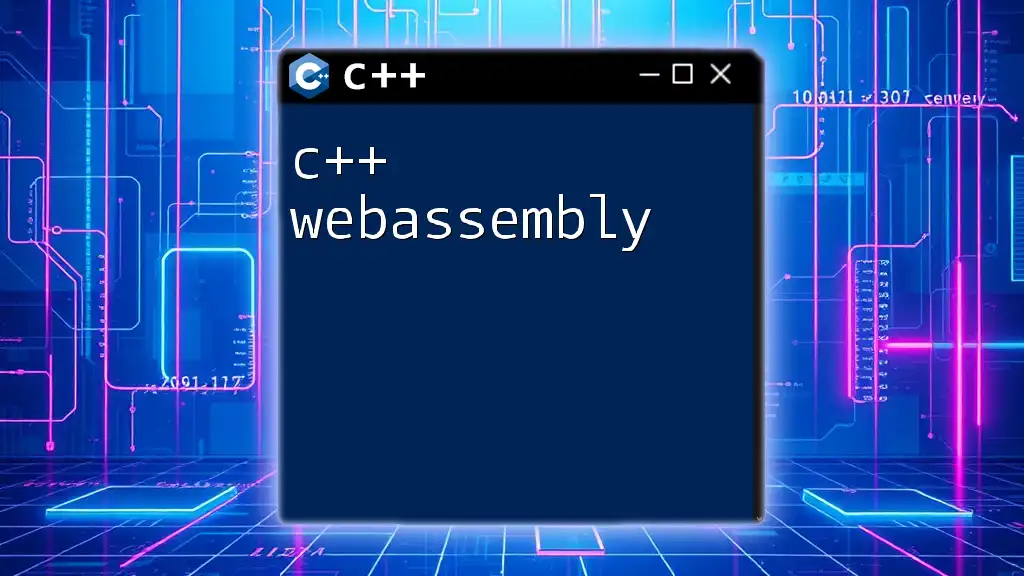
Enhancing Your Web Server
Adding Multi-threading Support
Understanding Concurrency in C++
When building a C++ web server, it’s crucial to handle multiple clients concurrently. This requires knowledge of concurrency in C++. By utilizing threads, you can allow multiple client requests to be processed simultaneously, enhancing your server's performance.
Here’s a simple example using the std::thread class to achieve concurrent request handling:
#include <thread>
void handle_request(tcp::socket socket) {
// Request handling code here
}
// In the main loop
std::thread(handle_request, std::move(socket)).detach();
Implementing Logging and Error Handling
Logging Best Practices
A robust logging mechanism is essential for monitoring the server's behavior and diagnosing issues. Implement a simple logging function like this:
void log(const std::string& message) {
std::cerr << message << std::endl;
}
By logging critical events, errors, and request details, the server will be easier to debug and maintain.
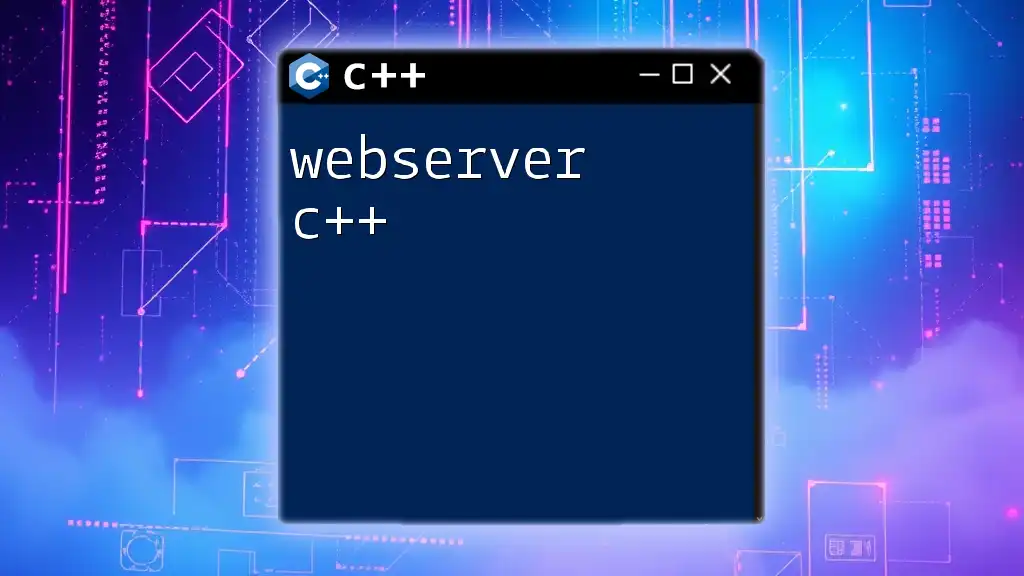
Testing and Debugging Your Web Server
Using Tools for Testing
Postman and curl are excellent tools for testing your web server. Postman offers a graphical interface for sending requests, while curl allows you to make requests from the command line. Testing various endpoints and payloads will help ensure your server operates as intended.
Common Errors and Debugging Tips
While developing your C++ web server, you may encounter various issues. Common errors include socket binding failures or timeout errors. Utilize logging extensively to capture errors and understand behavior under load.
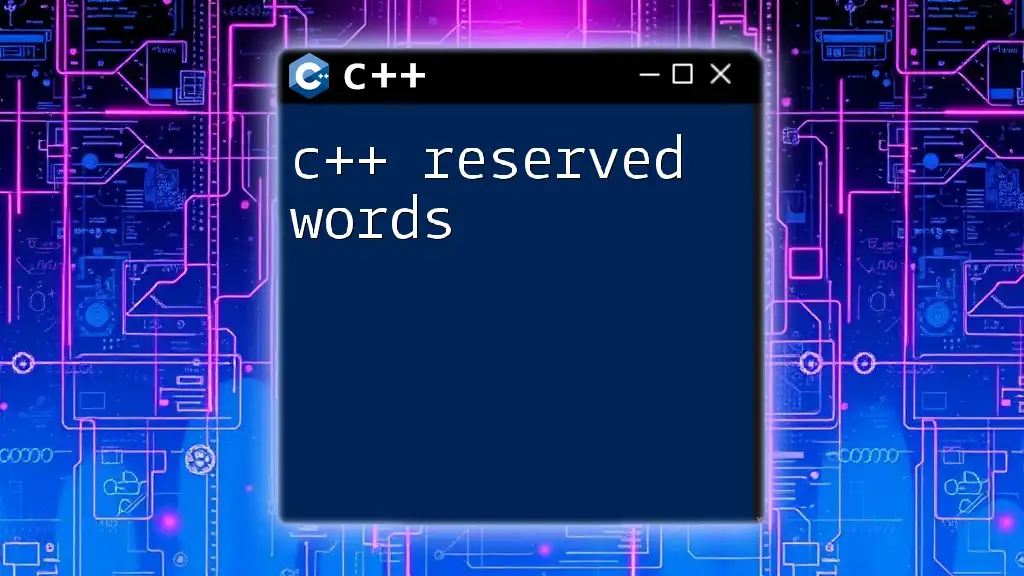
Deploying Your C++ Web Server
Choosing a Hosting Solution
When it comes to deployment, you can choose between self-hosting on your own hardware or leveraging cloud hosting solutions. Cloud platforms like AWS, Google Cloud, or DigitalOcean offer scalable solutions that can be particularly advantageous for handling variable traffic.
Configuring a Production Environment
Setting Up Security Measures
Security is paramount in web applications. Ensure that your C++ web server is equipped with necessary security measures. Implementing firewalls, SSL certificates, and regular software updates can significantly improve security.
Performance Tuning
To ensure optimal performance in production, consider tuning your server configuration. Analyze resource usage and load patterns, and apply optimizations such as connection pooling or caching frequently accessed data.
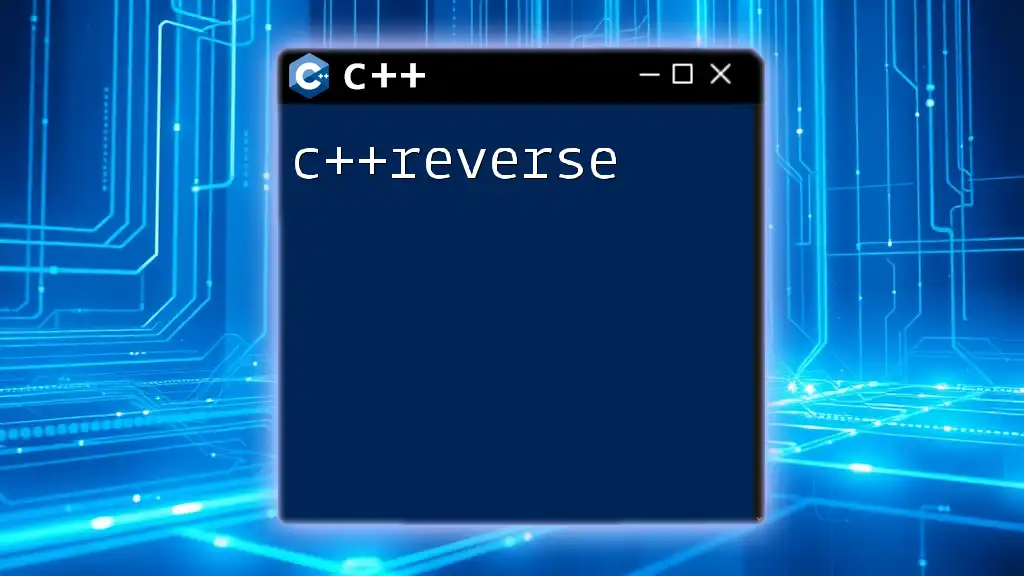
Conclusion
Building a C++ web server offers a rewarding experience and a deep understanding of web technologies. The topics covered in this guide provide a comprehensive overview, from setting up the environment to deploying your server securely.
For those looking to delve even deeper into C++ web development, exploration of advanced libraries, frameworks, and community resources can further enhance your knowledge and skills. Embrace the adventure, and develop a robust, high-performance C++ web server that meets the demands of modern applications.