The Llama CPP Web Server is a lightweight and fast web server that allows developers to quickly set up and serve web applications using C++.
#include <cpprest/http_listener.h>
using namespace web;
using namespace web::http;
using namespace web::http::experimental::listener;
int main() {
uri_builder uri(U("http://localhost:8080"));
auto addr = uri.to_uri().to_string();
http_listener listener(addr);
listener.support(methods::GET, [](http_request request) {
request.reply(status_codes::OK, U("Hello from Llama CPP Web Server!"));
});
listener
.open()
.then([&listener](){ UDEBUG("Starting to listen at: " + listener.uri().to_string()); })
.wait();
std::string line;
std::getline(std::cin, line);
return 0;
}
What is the Llama C++ Web Server?
The Llama C++ Web Server is an innovative web server framework built using C++. Its primary purpose is to facilitate the development and deployment of high-performance web applications. With features designed to take full advantage of C++'s capabilities in handling numerous requests concurrently, Llama allows developers to create efficient servers capable of serving dynamic and static content.
Key features of the Llama C++ Web Server include:
- Lightweight and Fast: Designed to be efficient with resources, Llama enables high-speed processing of requests.
- Asynchronous Processing: Offers robust support for asynchronous programming, allowing multiple operations to run simultaneously without blocking.
- Modular Architecture: The modular design provides flexibility, enabling developers to extend functionalities easily.
- Simple Routing: Intuitive routing mechanisms simplify the process of defining endpoints.
When comparing the Llama C++ Web Server to popular alternatives like Node.js or Apache, it stands out for its ability to leverage C++'s performance benefits, particularly in resource-constrained environments or when needing to handle thousands of requests efficiently.
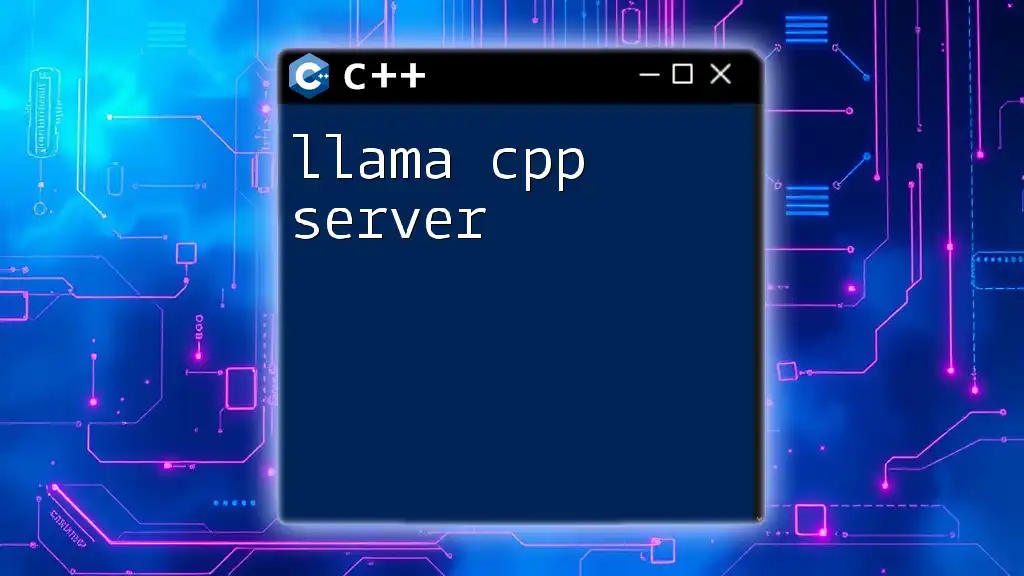
Setting Up Your Development Environment
Required Tools and Libraries
To get started with the Llama CPP Web Server, ensure you have the necessary tools and libraries installed:
C++ Compiler: You will need a competent C++ compiler. Popular options include:
- GCC: A widely-used, open-source compiler that supports C++11 and beyond.
- Clang: Known for its impressive performance and more informative error messages.
CMake: CMake is essential for building the Llama Web Server. You can install it using your package manager (e.g., `apt`, `brew`). Once installed, CMake simplifies the building process of your projects by allowing you to define build processes in a platform-independent manner.
Library Dependencies: Make sure to have necessary library dependencies installed, such as Boost for general C++ library functionality.
Installing the Llama C++ Framework
Begin the installation by cloning the repository and building the server from the source. Follow these steps:
-
Clone the repository using Git:
git clone https://github.com/your-repo/llama-web-server.git
-
Navigate to the cloned directory:
cd llama-web-server
-
Create a build directory and navigate to it:
mkdir build && cd build
-
Run CMake to configure the build:
cmake ..
-
Compile the project:
make
-
Run the initial test:
./llama_server
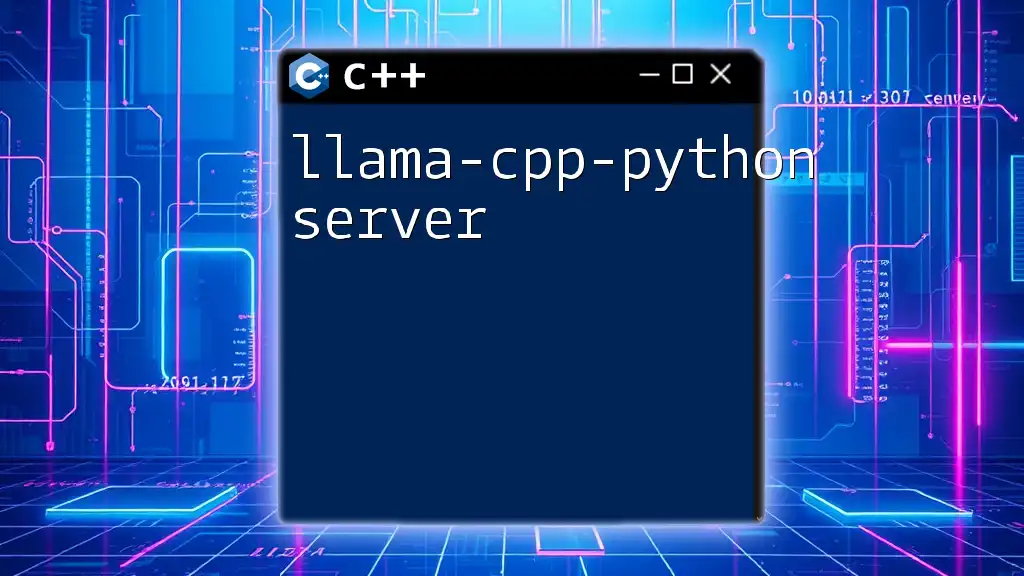
Understanding the Architecture of Llama C++ Web Server
Core Components
The architecture of the Llama C++ Web Server includes several core components that work together seamlessly:
- Request Handling: The server processes incoming HTTP requests and manages the lifecycle of each request.
- Response Generation: After processing a request, the server generates the appropriate HTTP response, which includes headers and body content.
- Routing: Llama provides a simple yet powerful routing mechanism, allowing developers to define how URLs correspond to specific functions or endpoints efficiently.
Request Lifecycle
The request lifecycle is fundamental to understanding how the Llama server operates. It consists of several stages:
- Initialization: The server initializes its resources and listens for incoming network requests.
- Request Parsing: As requests arrive, the server parses the HTTP headers and body to understand the client’s needs.
- Response Generation: After handling the request, the server generates an HTTP response to send back to the client.
An example of a simplified request lifecycle in Llama could involve a function that listens for a specific route and processes incoming data accordingly, reflecting the modular nature of Llama.
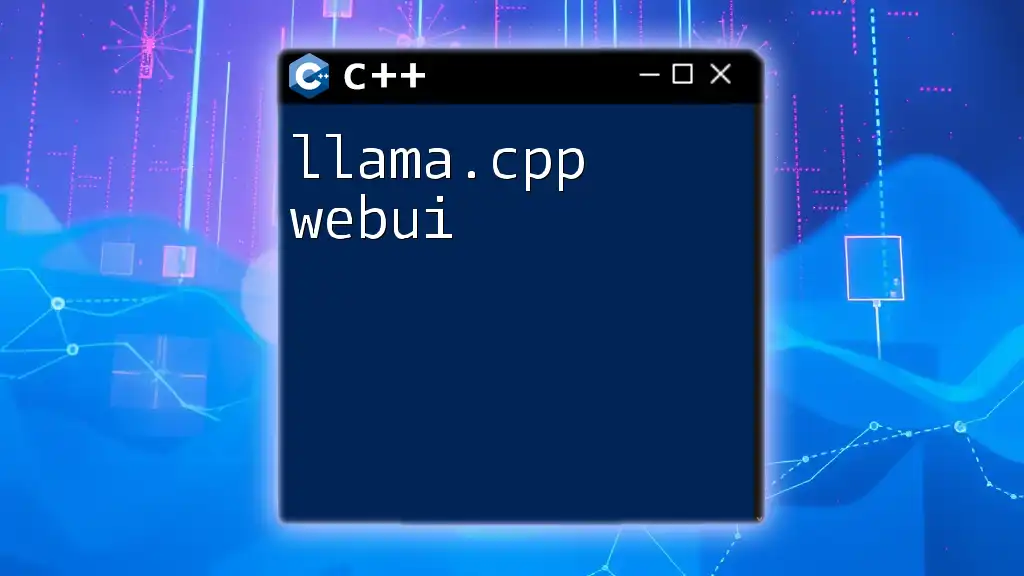
Basic Configuration and Usage
Configuration Files
Configuration plays a crucial role in managing various parameters of the web server. The configuration files determine settings such as server ports and enabled features. The configuration format is typically straightforward, allowing easy modifications as your application grows.
Starting the Server
Starting the server can be accomplished with simple command-line options. A command might look something like this:
./llama_server --port 8080
Handling Basic HTTP Requests
Creating a simple endpoint in Llama is intuitive. For example, you can set up a basic GET request handler as follows:
#include "llama_server.h"
void getHandler(const HttpRequest& req, HttpResponse& res) {
res.setBody("Hello, World!");
}
int main() {
LlamaServer server;
server.onRequest("GET", "/", getHandler);
server.start(8080);
}
In this snippet, the `getHandler` function generates a simple "Hello, World!" response whenever a GET request is made to the root endpoint `/`.
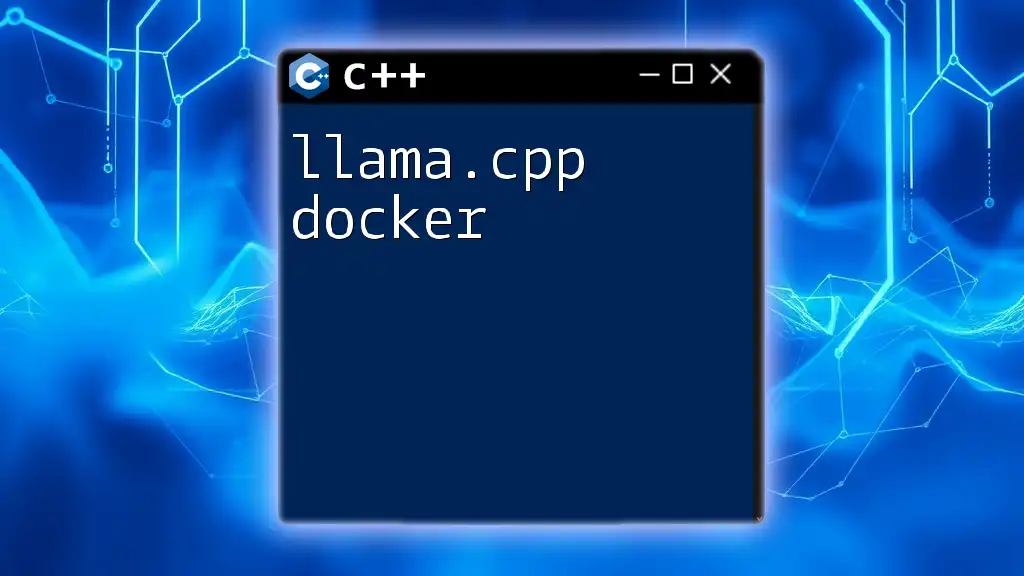
Advanced Features of Llama C++ Web Server
Middleware
Middleware extends the functionality of your server by allowing you to execute code before or after request handling. This can be useful for tasks such as authentication, logging, or modifying requests and responses.
To create a middleware function in Llama, define a function and apply it to the server:
void loggingMiddleware(const HttpRequest& req, HttpResponse& res) {
std::cout << "Request received at " << req.getUrl() << std::endl;
}
int main() {
LlamaServer server;
server.use(loggingMiddleware);
...
}
WebSockets Support
The Llama C++ Web Server has built-in support for WebSockets, make it easy to create real-time applications like chat servers. Defines a WebSocket route and a handler that deals with incoming messages.
Static File Serving
Llama simplifies serving static files like HTML, CSS, and JavaScript through straightforward configuration. By defining a directory, you can serve files using:
server.serveStatic("/public", "/var/www/public");
This command allows files in the `/var/www/public` directory to be accessible via the `/public` URL path.
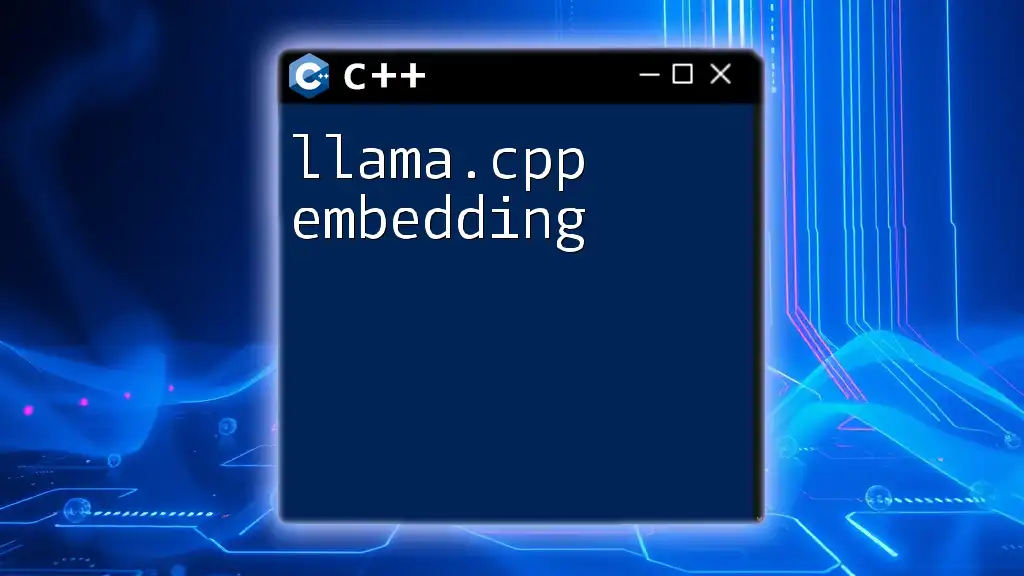
Error Handling and Debugging
Common Errors and Solutions
When developing with the Llama C++ Web Server, you might encounter typical errors, such as port conflicts or misconfigured routes. Understanding error messages and confirming configurations can help in quickly troubleshooting issues.
Logging
Effective logging is vital for debugging. Llama allows you to customize logging levels, helping you control the output based on the severity of messages. Incorporating logging provides insight into the server's operation:
logger.setLogLevel(LogLevel::DEBUG);
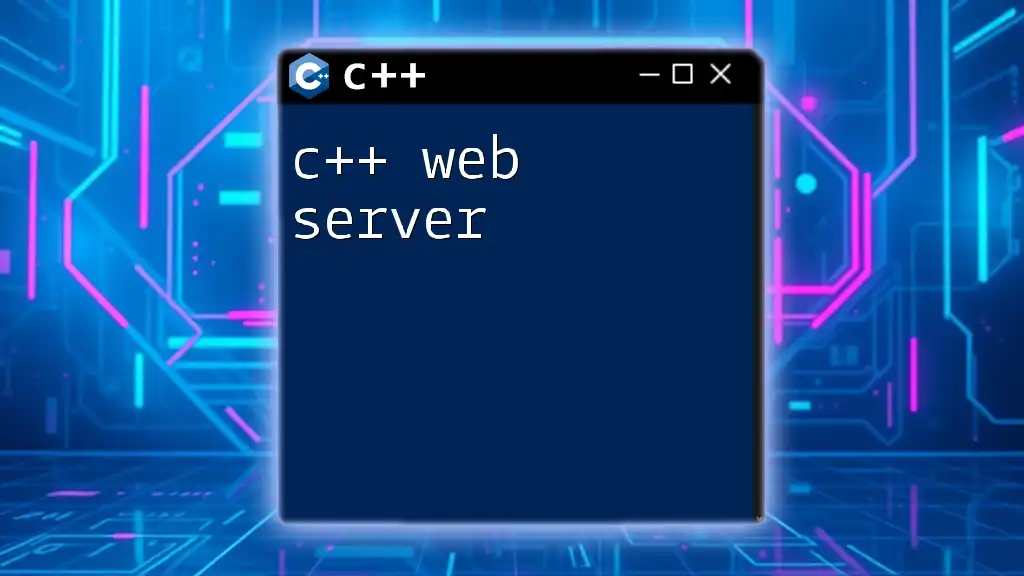
Performance Optimization Techniques
Asynchronous Processing
Embracing asynchronous processing optimizes server performance by allowing tasks like database queries or file reads to occur without blocking other operations. Utilizing asynchronous handlers can significantly boost your server's responsiveness.
Load Balancing
By implementing load balancing strategies with Llama, you can distribute incoming traffic across multiple server instances, thereby enhancing performance and reliability. Achieving this requires configuring your infrastructure correctly to manage incoming requests.
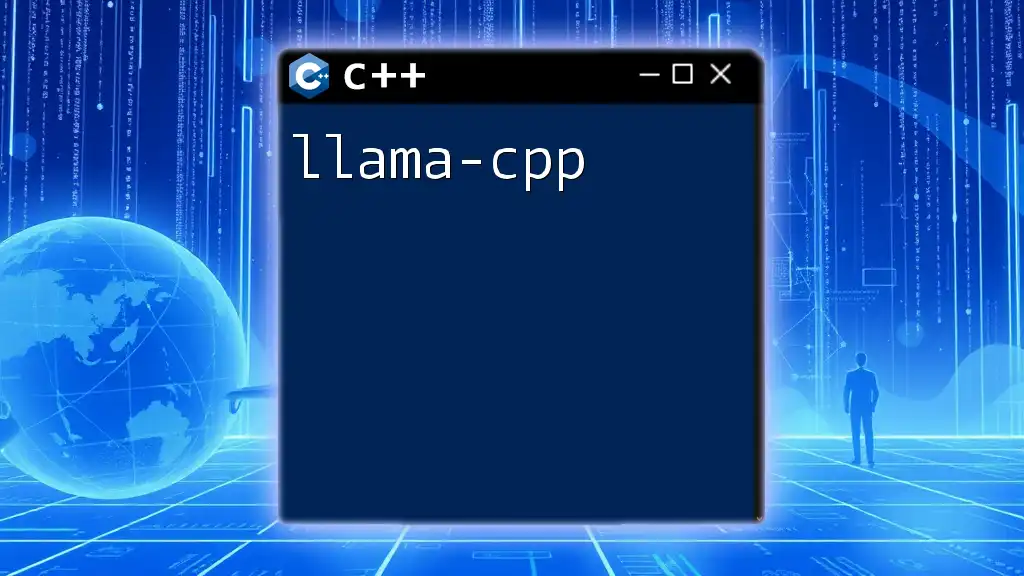
Securing Your Llama C++ Web Server
Understanding Common Security Threats
Being aware of vulnerabilities such as Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF) is essential when developing web applications. Utilize best practices, such as validating and sanitizing inputs, to secure your app against these threats.
Implementing HTTPS
Setting up SSL/TLS is crucial for securing your server’s communications. This can typically be done by obtaining a certificate from a trusted Certificate Authority (CA) like Let's Encrypt and configuring Llama to serve HTTPS requests.
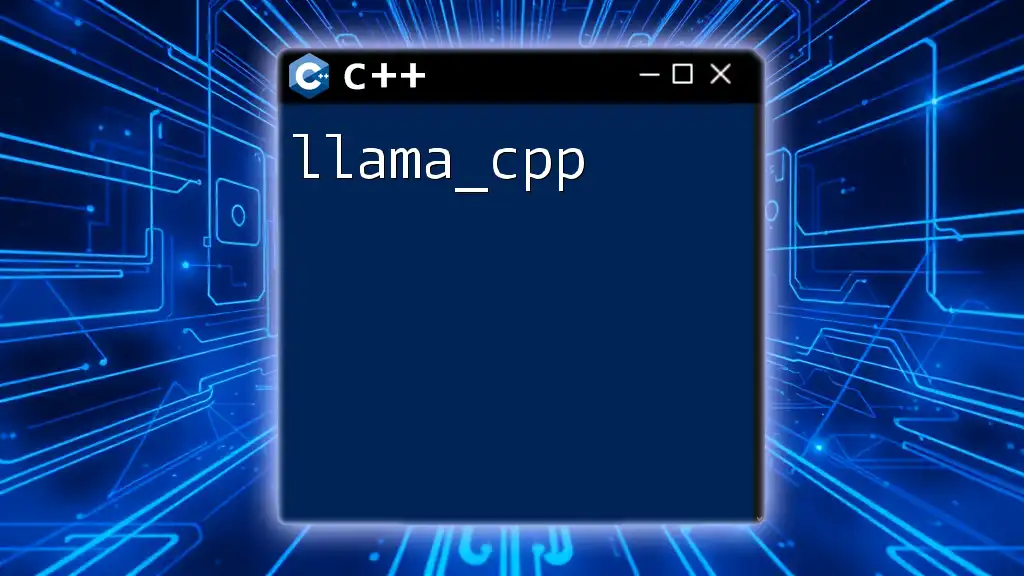
Conclusion
The Llama C++ Web Server is a powerful tool for developers looking to build high-performance, efficient web applications. Its unique features allow for an excellent balance of speed, flexibility, and ease of use. By following this comprehensive guide, you should be well-equipped to dive deeper into using Llama to create sophisticated web applications. Explore further, contribute to the community, and innovate using the capabilities of C++.