The `llama.cpp` API provides a lightweight interface for interacting with LLaMA models in C++, enabling efficient text generation and processing.
Here's a simple example of how to use `llama.cpp` in your code:
// Example of using llama.cpp to initialize a model and generate text
#include "llama.h"
int main() {
llama::Model model("path/to/model.bin");
std::string prompt = "Once upon a time";
std::string output = model.generate(prompt);
std::cout << output << std::endl;
return 0;
}
What is Llama.cpp?
Llama.cpp is a modern C++ library designed for efficient and intuitive natural language processing tasks. It enables developers to harness the power of advanced language models while simplifying the complexity often associated with traditional programming approaches. Since its inception, Llama.cpp has garnered attention due to its user-friendly API and high performance, paving the way for innovations in chatbot development, content generation, and more.
Key features of Llama.cpp include:
- Ease of Use: The API is structured to minimize the learning curve, making it accessible for both novice and experienced programmers.
- Performance: Engineered for speed, Llama.cpp ensures efficient model loading and text generation, particularly beneficial for real-time applications.
- Flexibility: Its design allows for easy integration with various datasets and projects.
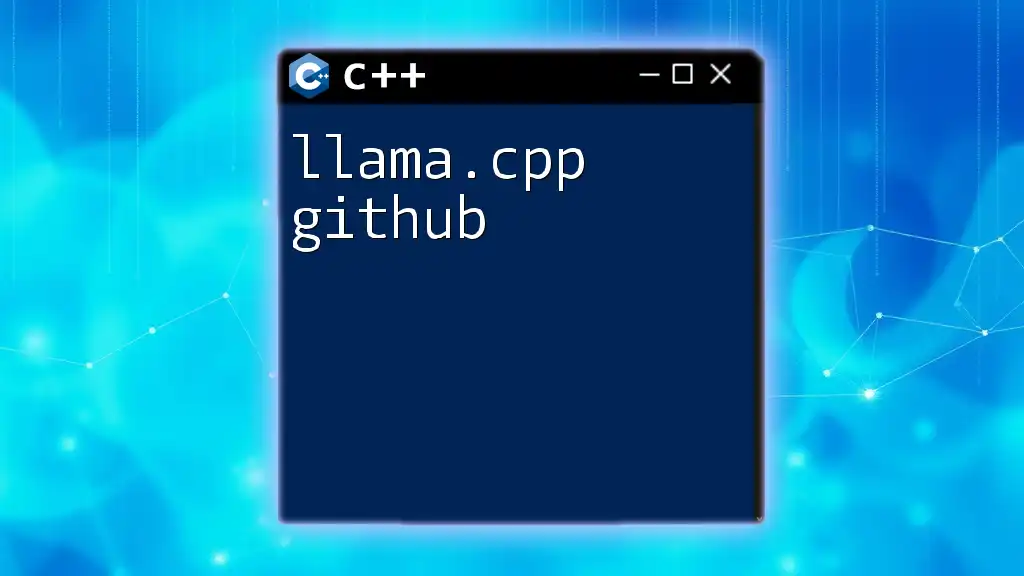
Setting Up Llama.cpp
System Requirements
Before you begin, ensure your system meets the following requirements:
- Operating Systems: Llama.cpp can run on major operating systems including Linux, macOS, and Windows.
- Dependencies: You need to have a C++ compiler that supports C++11 or higher and relevant libraries for Model handling and Tokenization.
Installation Process
Installing Llama.cpp is straightforward. Here’s how you can do it on different platforms:
For Ubuntu, execute the following command in your terminal:
sudo apt-get install llama-cpp
For macOS users, you can install it via Homebrew:
brew install llama-cpp
Windows users can find installation guidelines directly in the Llama.cpp GitHub repository, where they can clone the project and compile it locally.
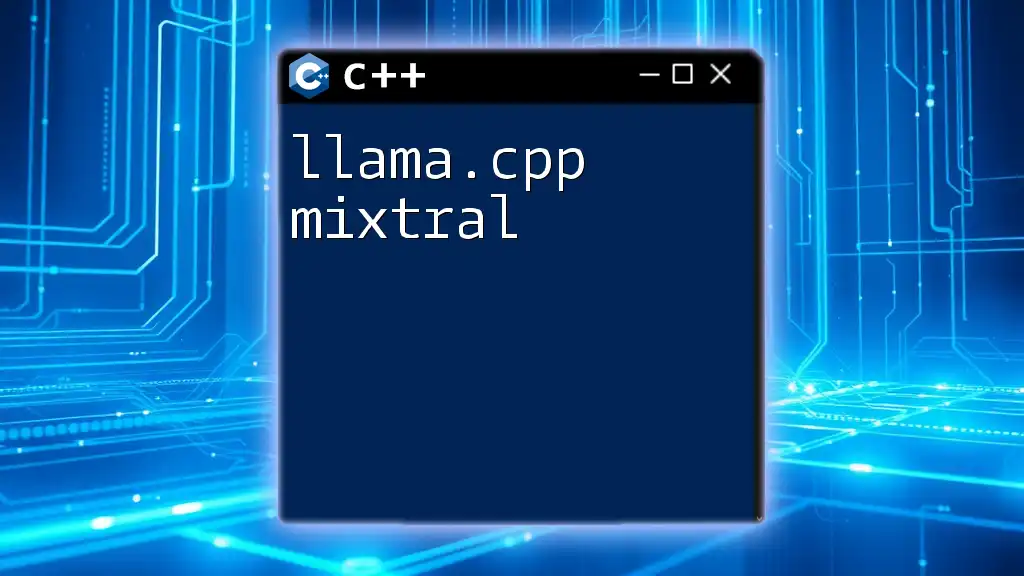
Getting Started with Llama.cpp
Basic Structure of a Llama.cpp Program
Once installed, you can kickstart your journey by creating a simple Llama.cpp program. The following code will initialize the Llama environment:
#include <llama.h>
int main() {
Llama::initialize();
return 0;
}
This tiny snippet demonstrates the basic structure needed to work with the Llama.cpp API, where the `Llama::initialize()` method prepares the API for use.
Understanding the Core Components
At the heart of Llama.cpp are several key components that work together to facilitate various functions:
-
Llama::Model: This is the entity responsible for representing the language model you will use. You can load pre-trained models into this class.
-
Llama::Tokenizer: Tokenization is crucial for breaking down text into manageable pieces. The Tokenizer class allows you to transform strings into tokens that the model can interpret.
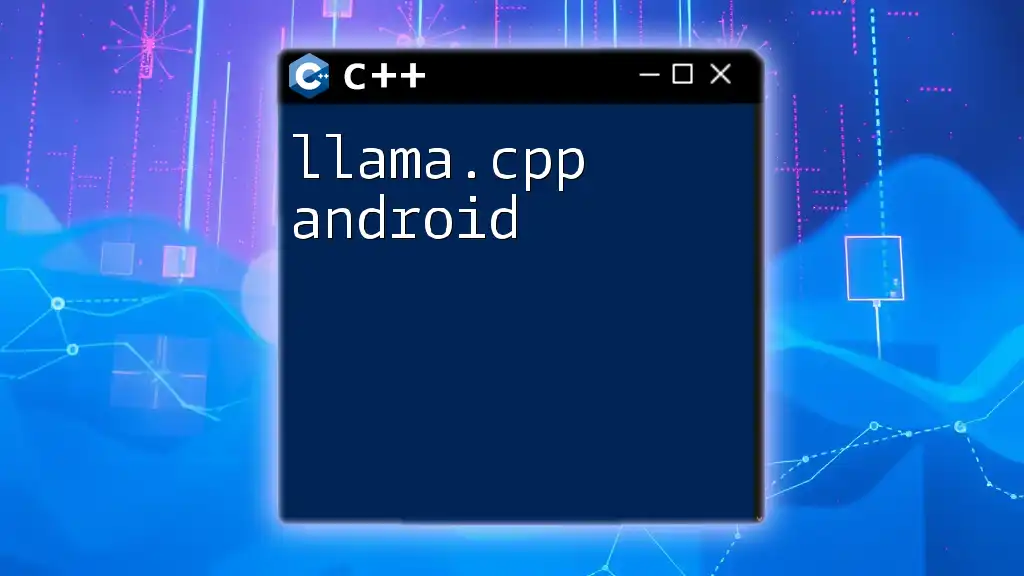
Using the Llama.cpp API
Initializing a Model
To utilize the Llama.cpp API effectively, the first step is to load a model. Start by specifying the path to your pre-trained model:
Llama::Model model("path/to/model");
Ensure that the model file is accessible, or you may encounter errors during loading.
Tokenization
Tokenization is critical in natural language processing. The Llama.cpp Tokenizer allows you to convert plain text into integers representing tokens. Here’s how you can tokenize text using Llama.cpp:
Llama::Tokenizer tokenizer("path/to/tokenizer");
auto tokens = tokenizer.tokenize("Hello, World!");
This example demonstrates how to transform the simple greeting into a format suitable for the model’s input.
Generating Text
Once you have tokens ready, generating text with a loaded model is seamless. Here’s how you can convert tokens back into coherent text:
std::string output = model.generate(tokens);
With this function, the model will produce text based on the input tokens, allowing you to build applications ranging from chatbots to content generators.
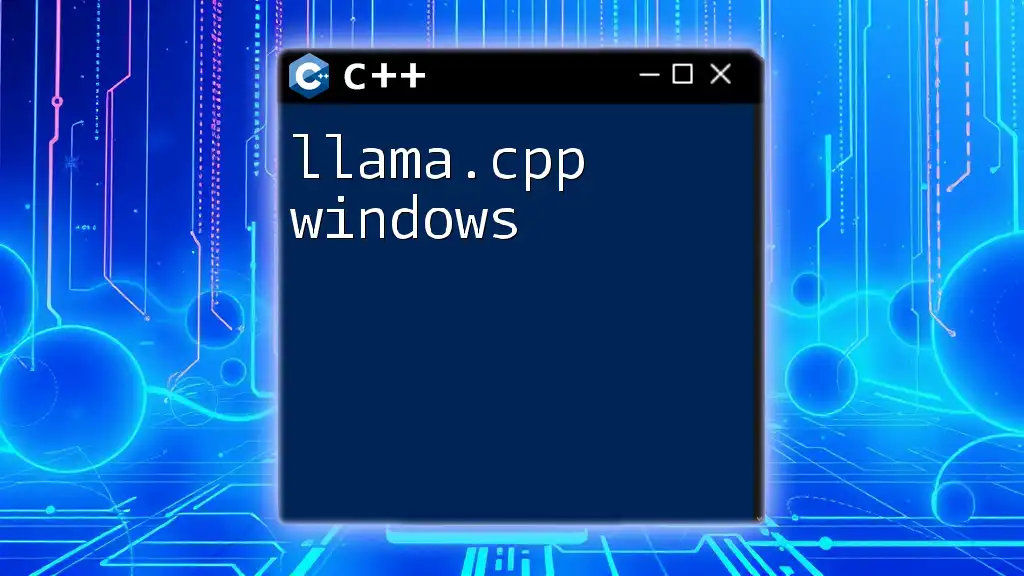
Advanced Usage of Llama.cpp
Customizing Generation Settings
Llama.cpp offers various parameters to tweak the text generation outputs. For instance, adjusting the temperature controls the randomness of the generated text, with lower values resulting in more predictable outputs. Here’s how:
model.set_temperature(0.7);
model.set_max_length(100);
Setting `max_length` ensures your generated text doesn’t exceed a specific number of tokens, allowing for precise control over output length.
Handling Large Datasets
When working with extensive datasets, it’s essential to optimize performance. Here are some best practices:
- Batch processing: Instead of processing text one at a time, batching inputs can lead to performance gains.
- Efficient memory usage: Keep an eye on how memory is allocated and released. Avoid unnecessary copies of data structures and prefer references where possible.
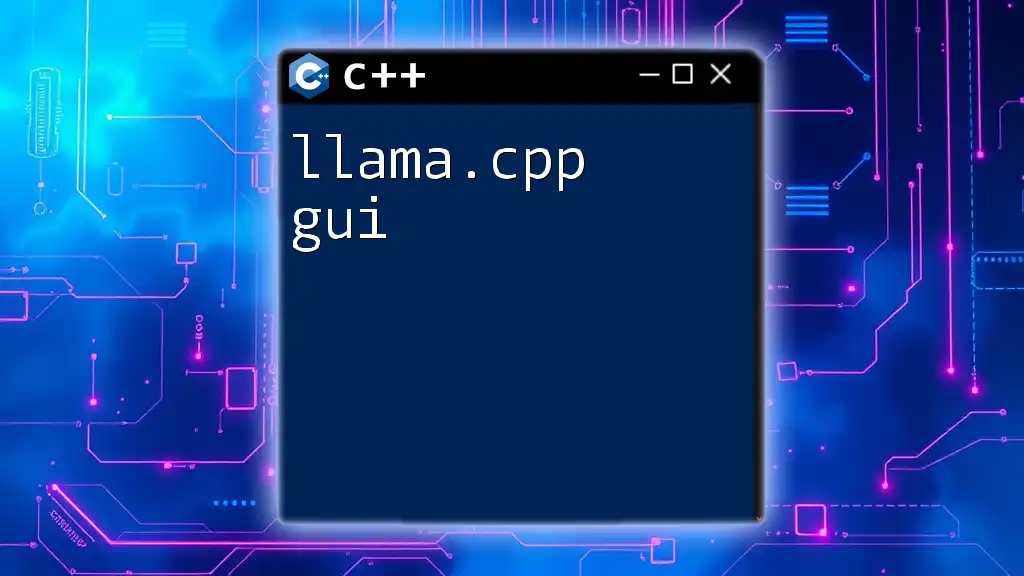
Common Issues and Troubleshooting
Debugging Tips
As with any programming endeavor, encountering errors is inevitable. Here are a few common pitfalls to watch for:
- Model not found: Ensure the model path is correct.
- Memory allocation failures: Check for memory leaks and ensure your system has sufficient RAM for large models.
Performance Optimization
To enhance your experience with Llama.cpp:
- Consider running benchmarks on different models to identify which performs best for your tasks.
- Profiling your application can also provide insights into bottlenecks, allowing targeted optimizations.
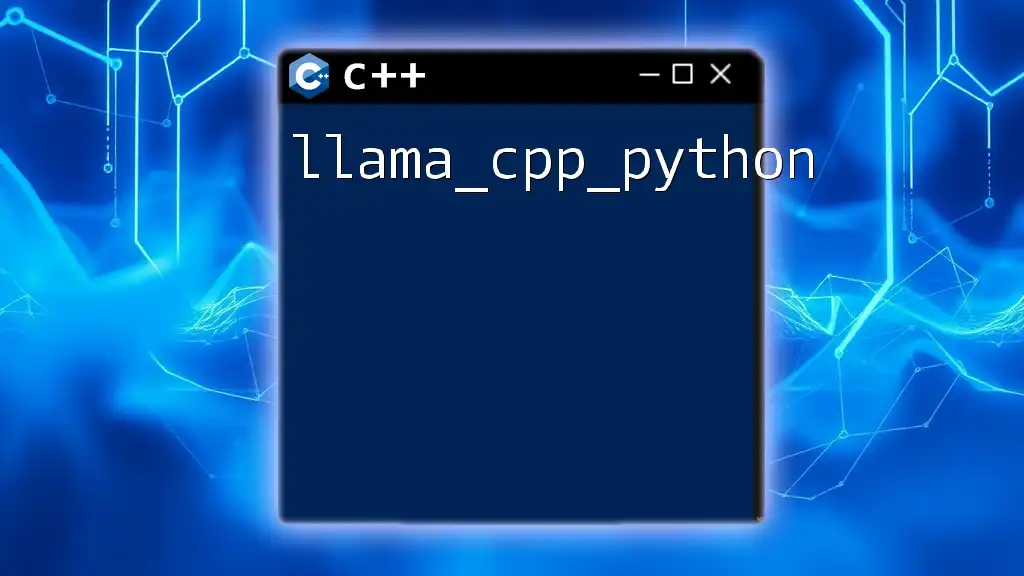
Use Cases and Applications
Example Applications
Llama.cpp excels in various applications, including:
- Chatbots: Automating conversations using contextual understanding.
- Text Summarization: Condensing long articles into digestible summaries.
- Creative Writing Tools: Assisting authors by generating ideas or content outlines.
Case Studies
There are numerous successful projects using Llama.cpp, showcasing its versatility. For instance, a well-known chatbot project integrated Llama.cpp’s functionalities to enhance interaction quality through better understanding and contextual responses.
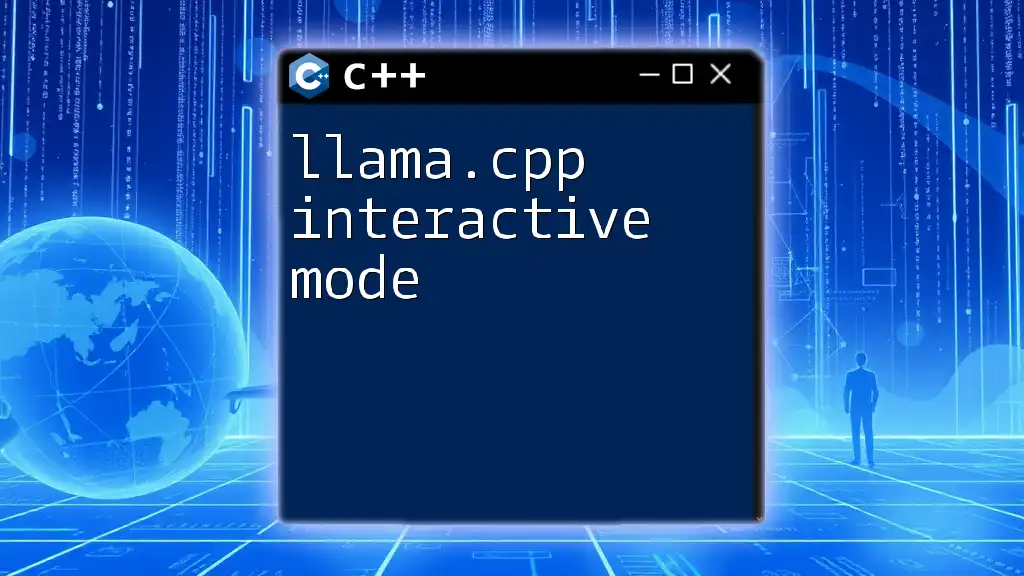
Conclusion
Llama.cpp offers a powerful yet approachable API for anyone looking to venture into language processing with C++. Its ease of use combined with robust performance makes it a valuable tool in the C++ ecosystem. I encourage you to explore and experiment with the Llama.cpp API in your projects, taking full advantage of its capabilities to innovate and enhance your applications.
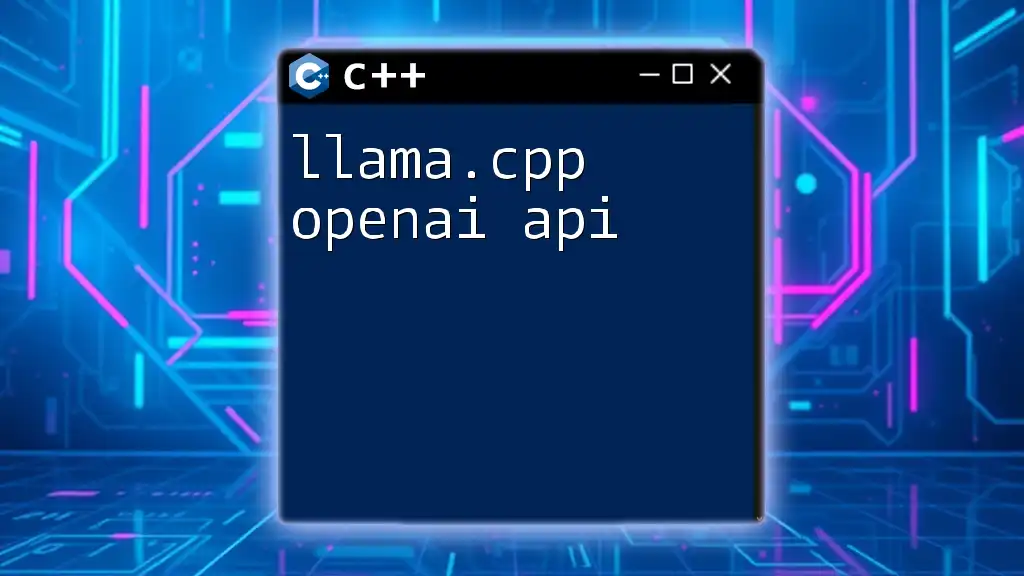
References
For detailed documentation and community support, check out the [Llama.cpp Documentation](insert_link_here). Additionally, explore forums and repositories for further interactions and resources.
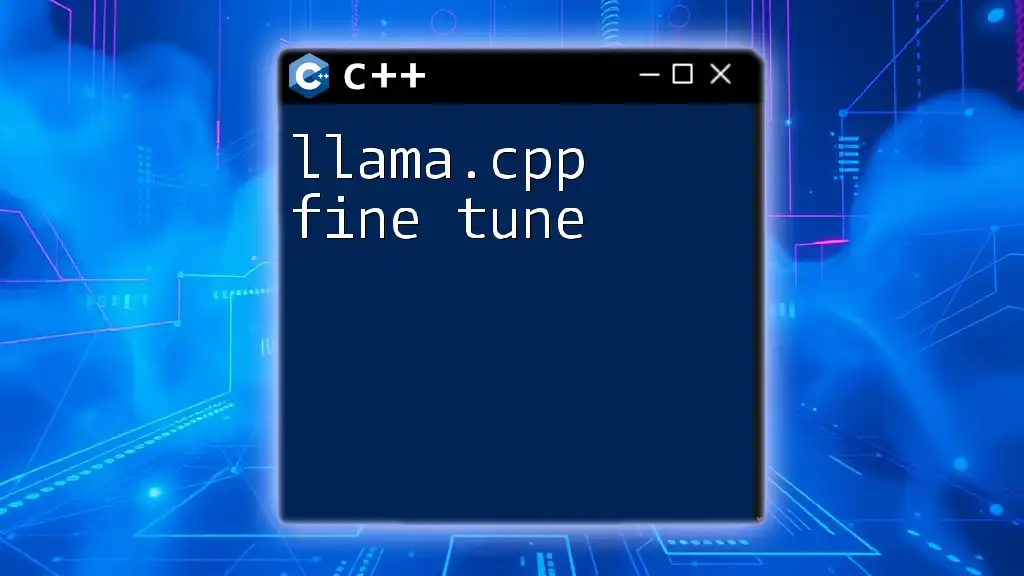
Call to Action
Now it’s your turn to dive into the world of Llama.cpp! Experiment with the API, create fascinating applications, and don't hesitate to share your experiences and feedback. Happy coding!