In "llama.cpp" grammar, commands are structured to execute specific functions with clear syntax and parameters, allowing for efficient manipulation of data and logic in C++.
Here’s an example of a simple C++ command that defines a function to output a greeting:
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet();
return 0;
}
Understanding Llama.cpp
What is Llama.cpp?
Llama.cpp is an innovative tool designed to integrate seamlessly with C++ programming, providing a streamlined way to utilize both standard and enhanced features. Its primary purpose is to simplify complex coding tasks and improve workflow efficiency. By understanding its capabilities, developers can harness its potential in various applications, from simple scripts to larger, more complex software solutions.
How Llama.cpp Works
The architecture of llama.cpp leverages C++'s inherent strengths while adding additional layers of functionality. Central to its design are several key components, including enhanced error handling, optimized performance processing, and a user-friendly interface that supports both beginners and experienced programmers. Unlike traditional cpp commands, llama.cpp allows for a more intuitive coding experience, often requiring fewer lines of code to achieve the same outcomes.
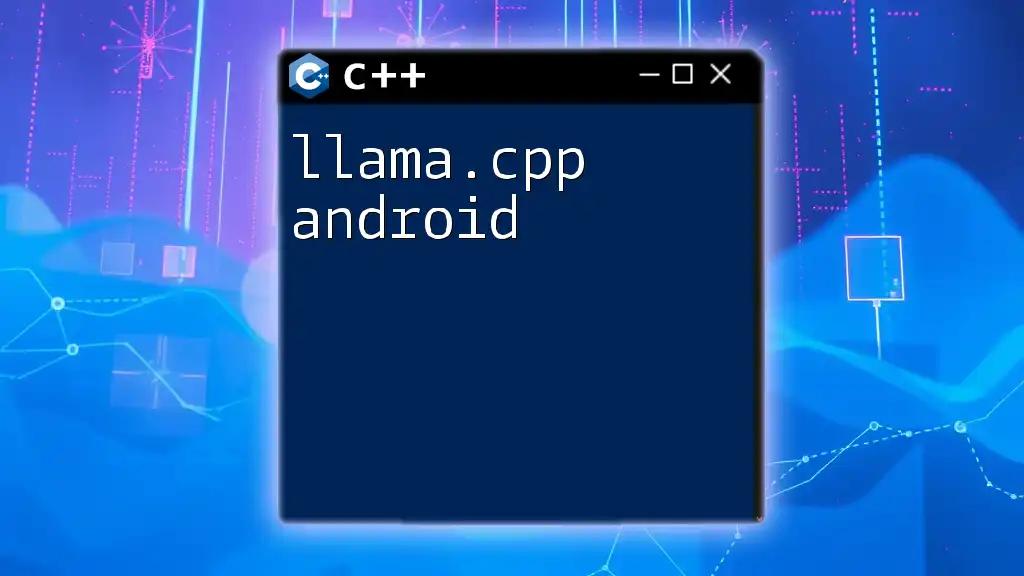
Grammar Fundamentals
What is Grammar in the Context of Llama.cpp?
In programming, grammar refers to the set of rules that dictate the structure and form of valid code. Understanding grammar is essential, as it ensures that code can be parsed and executed correctly by the compiler. In llama.cpp, grasping the nuances of grammar is critical for writing effective and error-free code, influencing everything from syntax to performance.
Basic Syntax Rules
Llama.cpp introduces specific syntax elements that, while often reminiscent of standard C++, can include unique constructs. Observing these rules is vital; even a minor omission can lead to syntax errors.
For instance, ensure that function calls correctly utilize commas to separate arguments. This small detail could mean the difference between functional code and frustrating debugging.
Example of correct vs. incorrect syntax:
// Example of correct syntax
llama_function(arg1, arg2);
// Example of incorrect syntax
llama_function(arg1 arg2); // Missing comma
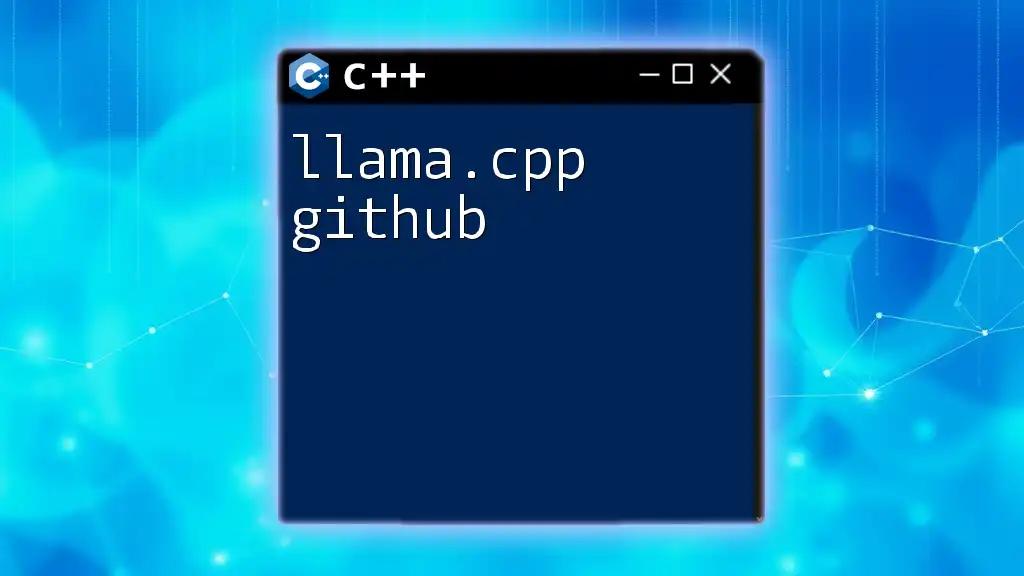
Key Components of Llama.cpp Grammar
Functions and Parameters
Defining Functions
Defining a function in llama.cpp requires understanding its structure. A standard function declaration includes the return type, function name, and parameters. Here's how to create a basic function:
Example of a basic function in llama.cpp:
llama_function(int x, int y) {
// function body
}
This structure is essential for building reusable code blocks that can be invoked throughout your application.
Using Parameters Effectively
Parameters are crucial for function versatility. Specifying the correct data types for parameters ensures that your functions can accept different kinds of input.
Example of a function with parameters:
llama_function(int length, int width) {
return length * width; // returns area
}
In this example, the function calculates the area of a rectangle using the parameters defined.
Data Types
Built-in Data Types
Understanding built-in data types is fundamental in any programming language, including llama.cpp. These types, such as `int`, `float`, and `char`, dictate how data is stored and manipulated. Each type serves specific purposes in calculations and operations.
Example of declaring data types:
int count = 10;
float price = 19.99;
Custom Data Types
In addition to built-in types, you can create custom types, such as structs and classes, allowing you to define complex data structures suited to your project’s needs.
Example of a custom data type:
struct Rectangle {
int length;
int width;
};
This helps encapsulate data and encourages a modular approach to coding.
Control Structures
Conditional Statements
Conditional statements allow you to dictate the flow of execution based on certain conditions. Llama.cpp supports standard conditionals such as `if`, `else if`, and `switch-case`, enabling developers to create responsive applications.
Example of a conditional statement:
if (count > 10) {
// code here
} else {
// alternative code here
}
This structure helps control the program's logic based on user input or other variables.
Loops
Loops are essential in programming for executing repetitive tasks. Llama.cpp provides several looping constructs, including `for`, `while`, and `do-while`, which can significantly reduce code length and improve efficiency.
Example of a for loop:
for (int i = 0; i < 5; i++) {
// loop body
}
This syntax iterates over a block of code for a specified number of times.
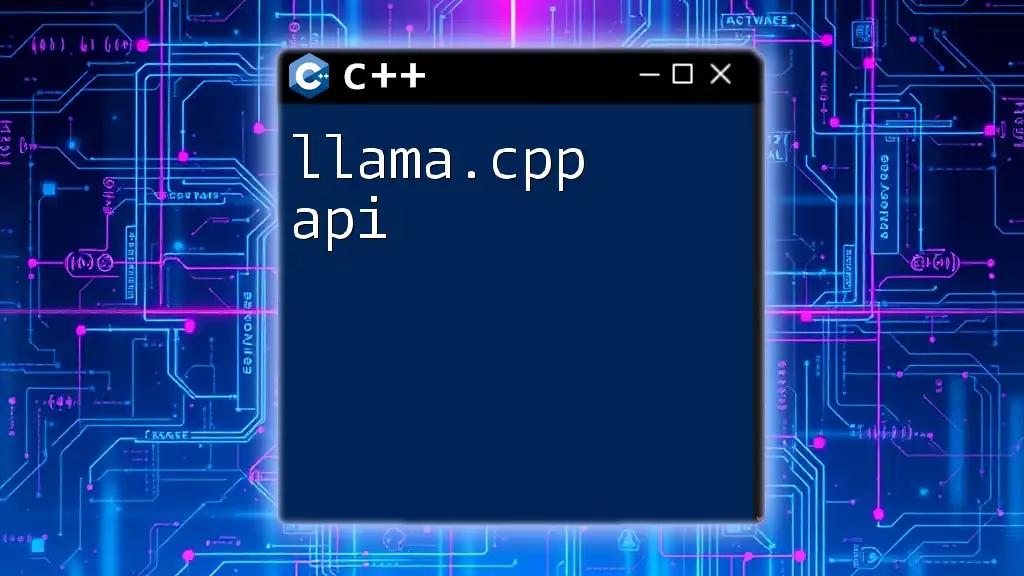
Best Practices for Writing Llama.cpp Code
Writing Clear and Concise Code
The clarity of your code directly affects maintainability and understandability. Strive to write code that is straightforward and avoids unnecessary complexities. Good practices include using meaningful variable names and keeping functions focused on single tasks.
Example of clear vs. poorly written code:
// Clear code
int sum(int a, int b) {
return a + b;
}
// Poorly written code
int s(int a, int b) { return a+b; }
Adhering to these principles will make your code more accessible to others who may work on it in the future.
Commenting Your Code
Adding comments is a fundamental practice that aids in understanding code at a glance. Clear, informative comments explain the purpose of functions, parameters, and complex logic.
Example of effective commenting:
// This function calculates the area of a rectangle
int area(Rectangle rect) {
return rect.length * rect.width;
}
Effective comments provide context and rationale, enhancing overall code readability.
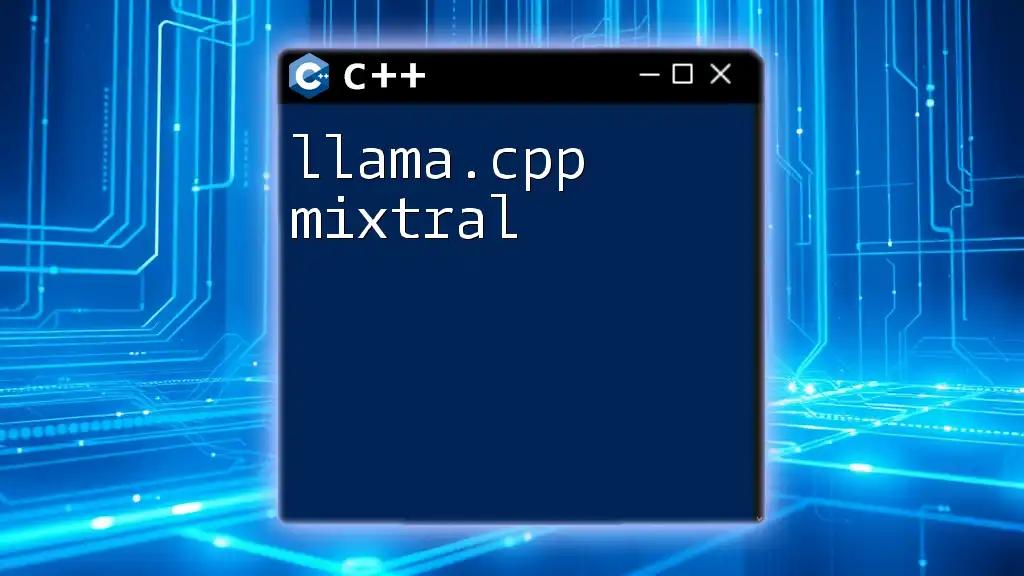
Troubleshooting Common Issues in Llama.cpp Grammar
Identifying Syntax Errors
Syntax errors can be frustrating and can arise from minor oversights like missing semicolons or misused syntax. Understanding common pitfalls can help prevent these mistakes.
Example of a missing semicolon error:
// Example of a missing semicolon error
int num = 5 // Missing semicolon
Recognizing patterns in your coding habits can help you avoid these frequent errors.
Debugging Techniques
For complex issues, employing debugging techniques is essential. Utilize debugging tools available within development environments or print statements to track variable states and flow.
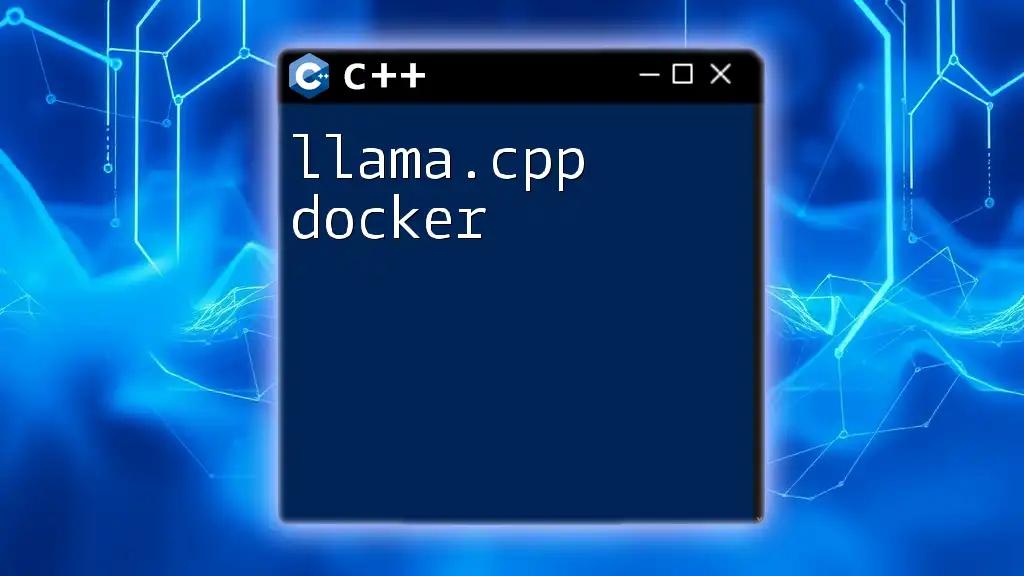
Conclusion
Understanding and mastering llama.cpp grammar unlocks significant potential in C++ programming. By following these guidelines and applying your knowledge consistently, you can enhance your coding skills, solve problems more efficiently, and create robust applications. Practice is key—engage with the community, explore advanced topics, and continually refine your approach to programming.
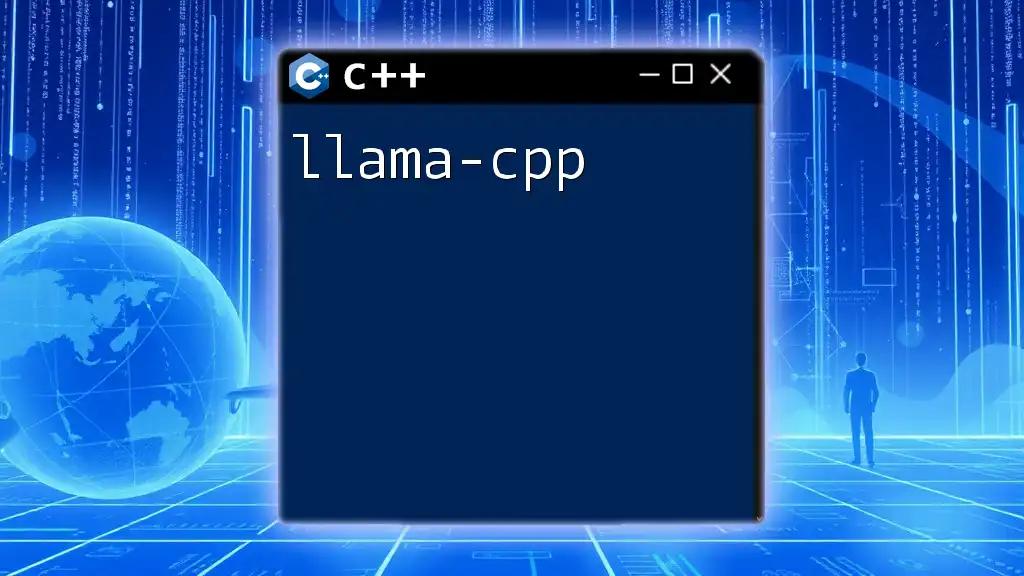
Additional Resources
Consider leveraging books, online courses, and community forums dedicated to C++ and llama.cpp. These resources can provide support and guidance as you advance your learning and development journey. Engage with tools and libraries designed to complement your coding efforts and enhance productivity in your programming endeavors.