The "llama.cpp android" refers to a C++ implementation of the LLaMA language model that can be compiled and run on Android devices, allowing developers to leverage advanced AI capabilities on mobile platforms.
Here’s a simple code snippet to get you started with a basic application:
#include <jni.h>
#include <string>
extern "C"
JNIEXPORT jstring JNICALL
Java_com_example_llama_MainActivity_stringFromJNI(JNIEnv *env, jobject /* this */) {
std::string hello = "Hello from LLaMA!";
return env->NewStringUTF(hello.c_str());
}
What is Llama.cpp?
Llama.cpp is an innovative C++ framework designed to streamline the development process for Android applications. It provides developers with a structured approach to utilize C++ commands effectively within the Android ecosystem, making it an essential tool for those looking to enhance their mobile development skills. One of the key advantages of Llama.cpp is its ability to leverage C++'s high performance while offering a straightforward interface for Android developers.
Why Choose Llama.cpp for Android Development?
The primary reason to choose Llama.cpp is its unique blend of performance and usability.
- Efficient Performance: C++ is known for its speed and resource management, making Llama.cpp an optimal choice for applications that require intensive calculations or graphics rendering.
- Cross-Platform Potential: Llama.cpp opens the possibility for developers to create applications that run seamlessly across multiple platforms, not just Android.
- Accessibility: For developers already skilled in C++, using Llama.cpp minimizes the learning curve compared to other frameworks, enabling faster development cycles.
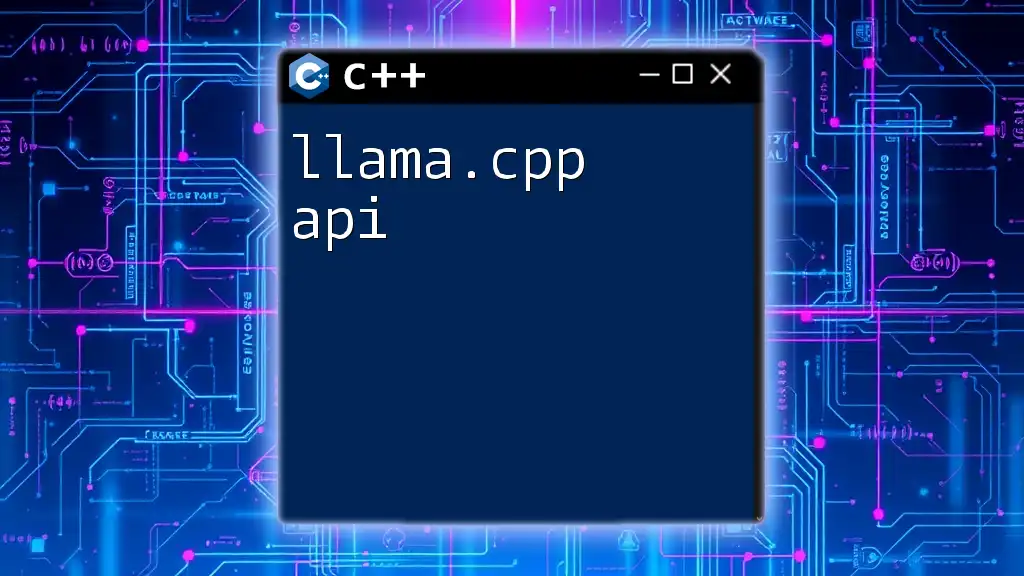
Setting Up Your Development Environment
To get started with Llama.cpp, you need to set up your development environment correctly.
Necessary Tools and Software
- Android Studio: The primary IDE you will use for building Android applications. You can download it from the official [Android Developer website](https://developer.android.com/studio).
- CMake: A tool that helps manage the build process in a simple and efficient way. It usually comes bundled with Android Studio.
Integrating Llama.cpp with Android Studio
Setting up Llama.cpp within Android Studio involves a few key steps:
-
Creating a New Project:
- Open Android Studio and click on "New Project". Choose a Basic Activity template for a simple starting point.
-
Adding Llama.cpp to Your Project Dependencies:
- Navigate to your project’s `build.gradle` file and add Llama.cpp as a dependency. Your `build.gradle` might include a section like this:
dependencies { implementation 'com.example:llama.cpp:1.0' }
- Navigate to your project’s `build.gradle` file and add Llama.cpp as a dependency. Your `build.gradle` might include a section like this:
-
Configuring CMake:
- Create a `CMakeLists.txt` file in the `app/src/main/cpp` directory and configure it to include both your source files and Llama.cpp library:
cmake_minimum_required(VERSION 3.4.1) add_library(llama SHARED src/main/cpp/your_cpp_code.cpp) find_library(log-lib log) target_link_libraries(llama ${log-lib})
- Create a `CMakeLists.txt` file in the `app/src/main/cpp` directory and configure it to include both your source files and Llama.cpp library:
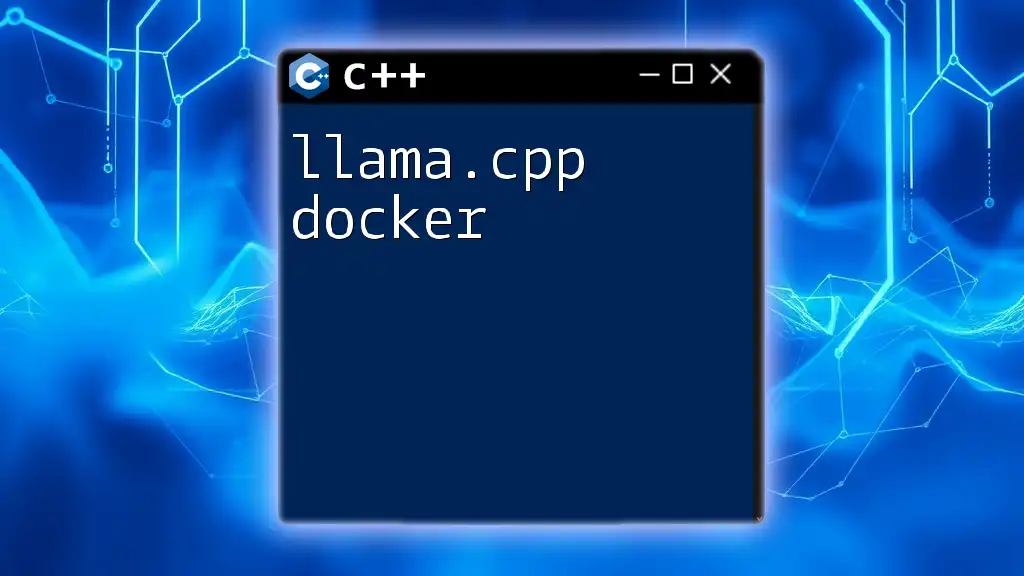
Key Concepts of Llama.cpp for Android Development
To effectively use Llama.cpp, it’s essential to understand its foundational concepts.
Understanding the Basics
Core Concepts: Llama.cpp uses a straightforward command structure that allows developers to issue commands easily. Here’s a basic syntax example:
LlamaCommand("command_name", {"arg1", "arg2"});
Core Components of Llama.cpp
Data Types and Structures
Llama.cpp supports a variety of data types that enhance its capability to interact with Android’s Java components. Here’s an example of a custom data structure:
struct MyData {
int id;
std::string name;
};
Standard Commands
The framework provides a set of commands that developers frequently use. For instance, you might want to define a command that retrieves user input:
std::string getUserInput() {
std::string input;
std::cin >> input; // Basic input from console for demonstration
return input;
}
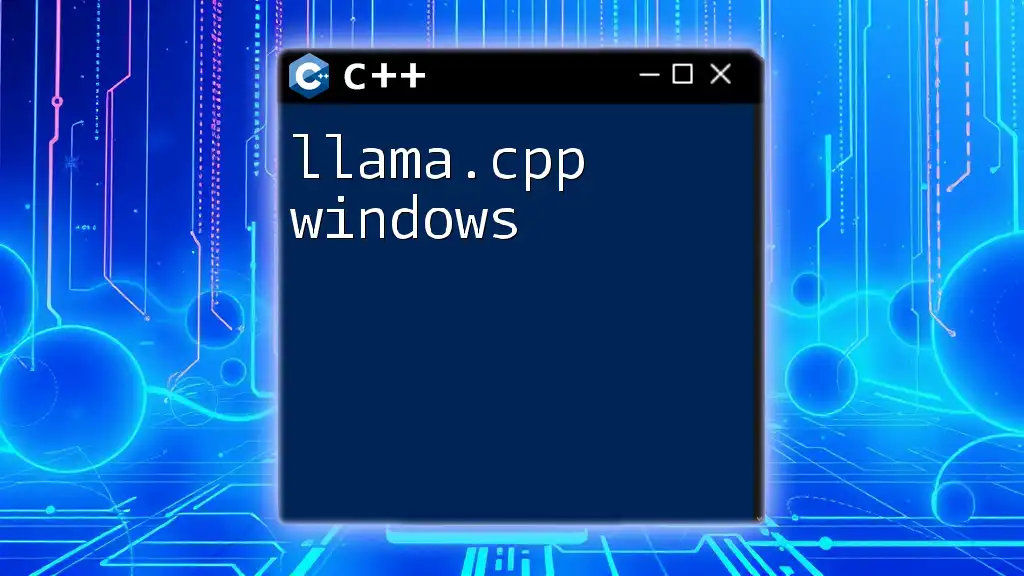
Building Your First Android Application with Llama.cpp
Project Structure Overview
When you create an Android project using Llama.cpp, your project structure will resemble this:
- `app/`
- `src/`
- `main/`
- `java/`
- `cpp/`
- `CMakeLists.txt`
- `your_cpp_code.cpp`
- `res/`
- `AndroidManifest.xml`
- `main/`
- `src/`
Building a Simple App
Creating a User Interface
Using XML layout files, you can easily design the UI. Here’s a simple activity layout:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, Llama!" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me!" />
</LinearLayout>
Implementing Business Logic with C++
You can connect the UI with C++ functions. For instance, when the button is clicked, you may want to process input:
// In your_cpp_code.cpp
extern "C"
JNIEXPORT void JNICALL
Java_com_example_yourapp_MainActivity_onButtonClicked(JNIEnv *env, jobject thiz) {
char input[100];
std::cout << "Enter something: ";
std::cin >> input;
// Logic for what to do with input
}
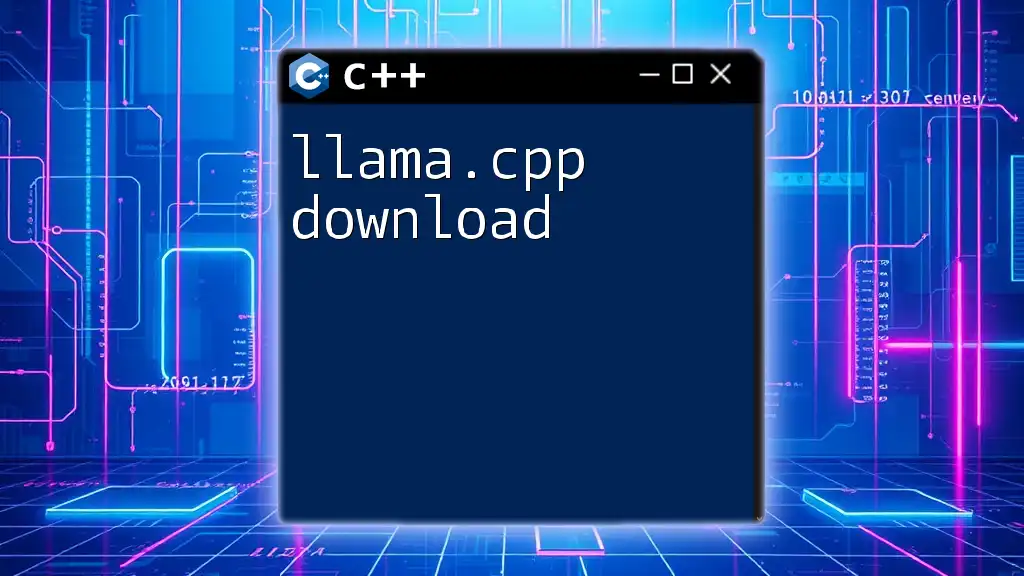
Debugging and Testing
Common Issues and How to Solve Them
When developing with Llama.cpp on Android, you may encounter issues such as linking errors or compatibility problems. To troubleshoot these:
- Use logs effectively. Android’s `Logcat` can display messages to help identify where your code may have issues.
- Implement breakpoints in Android Studio to step through your code and understand the flow better.
Testing Your Llama.cpp Application
Testing your application ensures it behaves as expected. You can use Google’s Google Test Framework for unit testing in C++. Here is a simple example of a test case:
#include <gtest/gtest.h>
TEST(MyDataTest, BasicAssertions) {
MyData data;
data.id = 1;
data.name = "Llama";
EXPECT_EQ(data.id, 1);
EXPECT_EQ(data.name, "Llama");
}
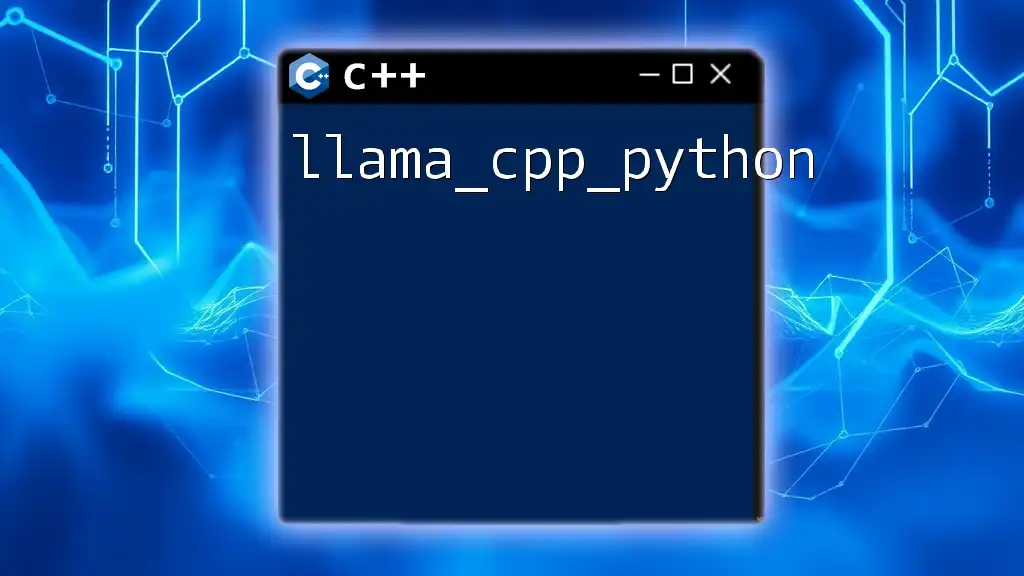
Advanced Features of Llama.cpp
Optimizing Performance
Optimization is key to ensuring your application runs smoothly. Techniques include:
- Memory Management: Understanding pointers and dynamic allocation can help reduce memory overhead.
- Code Profiling: Use tools like Android Profiler to identify bottlenecks in your application's performance.
Integrating with Other Android Libraries
Llama.cpp can be integrated with popular Android libraries, such as OpenGL for graphics rendering. An example of integrating OpenGL might look like this:
extern "C" JNIEXPORT void JNICALL
Java_com_example_yourapp_MainActivity_onSurfaceCreated(JNIEnv *env, jobject thiz) {
// Initialize OpenGL here
}
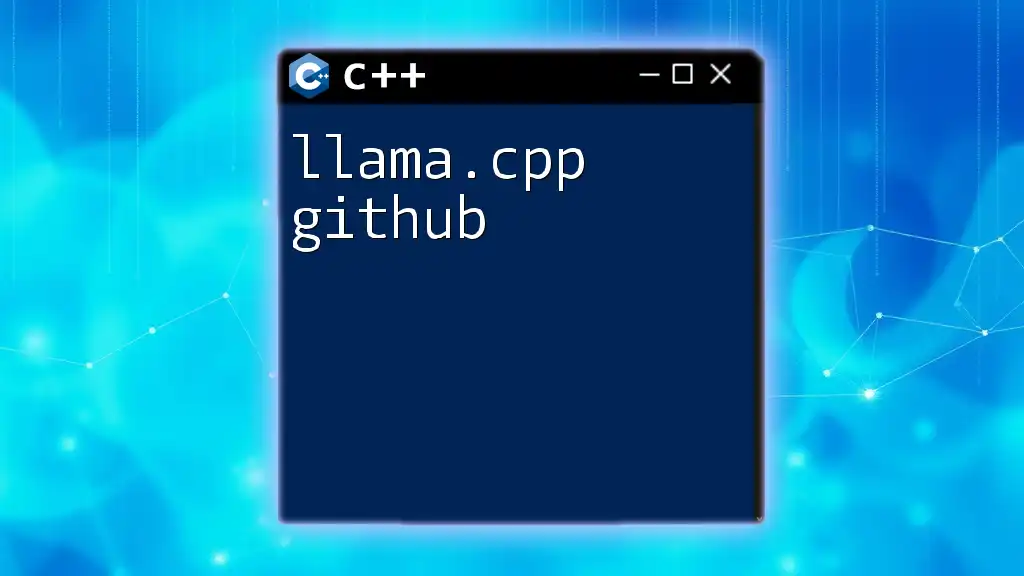
Community and Resources
Where to Get Help
The developer community is a valuable resource. Platforms such as Stack Overflow, GitHub, and dedicated forums for Llama.cpp can provide assistance. It’s also beneficial to follow the official documentation for updates and deeper insights.
Learning Resources
To further enhance your understanding of Llama.cpp and associated technologies, consider:
- Books on C++ and Android Development.
- Online Courses, particularly those focusing on mobile app development.
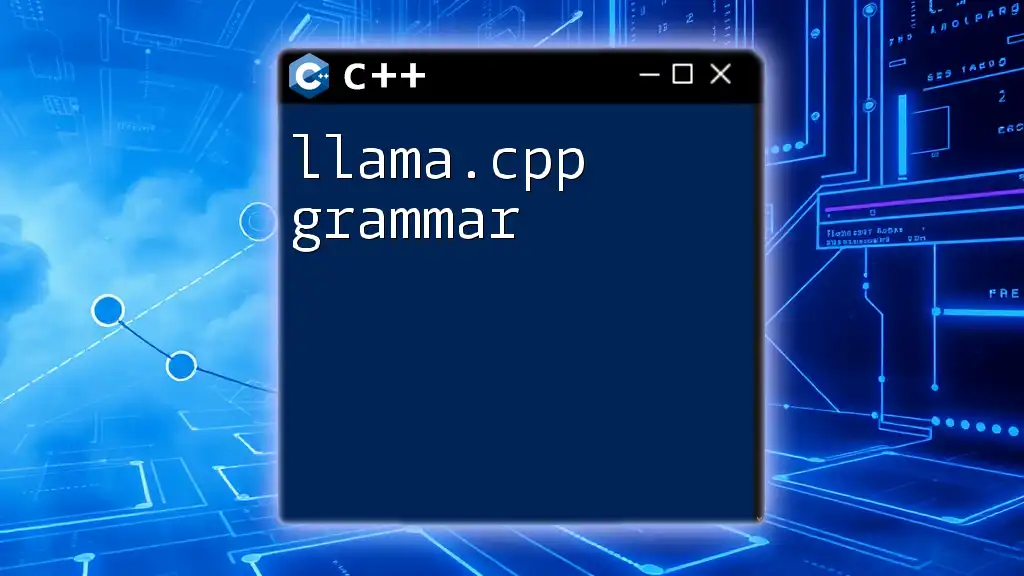
Conclusion
By leveraging llama.cpp android, you position yourself to build robust and efficient applications that harness the power of C++. The framework’s ability to blend C++ performance with Android accessibility makes it an excellent choice for developers keen on mobile app development. Embrace Llama.cpp, experiment, and share your experiences — the developer community thrives on collaboration and innovation.