Llama-cpp is a C++ library designed to efficiently implement various llama algorithms with simplicity and clarity in mind.
Here's a basic code snippet demonstrating how to use llama-cpp to train a simple model:
#include <llama.h>
int main() {
LlamaModel model("path/to/model");
model.train("path/to/training/data");
model.save("path/to/save/model");
return 0;
}
Getting Started with Llama-CPP
Installation Requirements
Before you can dive into using llama-cpp, you'll need to ensure your environment is ready. Here’s what you need:
- C++ Compiler: A modern C++ compiler, such as GCC or Clang.
- CMake: This is essential for building projects that utilize Llama-CPP.
- Libraries: Check if additional libraries, such as Boost, are required for your specific use cases.
Platforms Supported Llama-CPP is designed to be versatile, supporting various operating systems:
- Windows
- macOS
- Linux
Setting Up Your Environment
To start using llama-cpp, follow these steps:
- Install Dependencies: Ensure you have your C++ compiler and CMake installed. For instance, on Ubuntu, you may run:
sudo apt-get install build-essential cmake
- Clone the Repository: Get the latest version of llama-cpp from the official repository:
git clone https://github.com/example/llama-cpp.git
- Build the Project: Navigate to the directory and build the project:
cd llama-cpp
mkdir build
cd build
cmake ..
make
This will compile llama-cpp and prepare it for use.
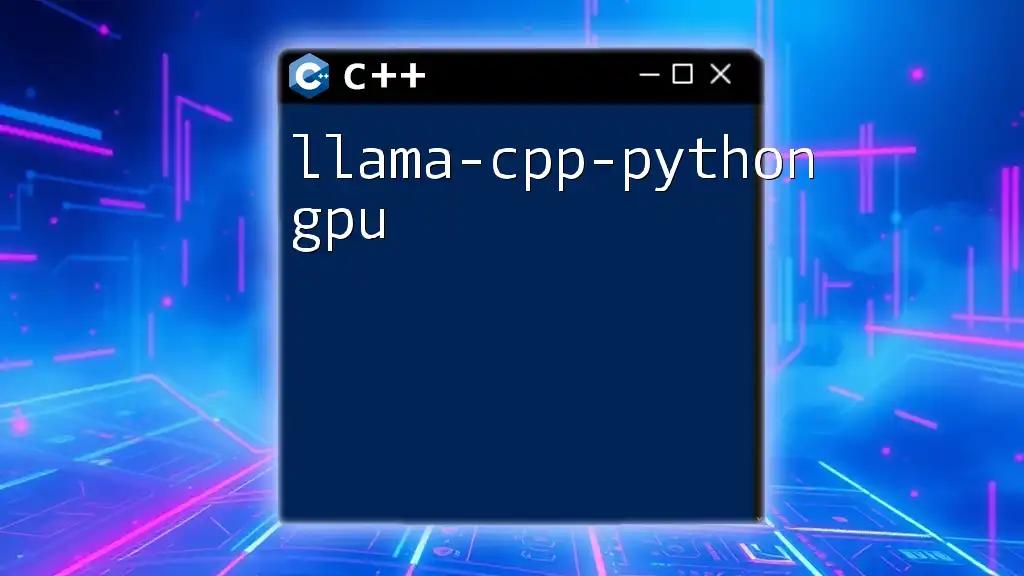
Core Concepts of Llama-CPP
Understanding the Syntax
The syntax of llama-cpp commands is crafted to be intuitive. Generally, you can expect:
-
Clear command structure: Each command follows a straightforward pattern, ensuring ease of understanding.
-
Common pitfalls: New users often mistakenly misplace brackets or forget to include necessary libraries.
To illustrate, here’s a common command structure:
llama_cpp_command(arguments);
Key Features
Efficiency
One of the standout features of llama-cpp is its efficiency in executing commands. For instance, you might notice dramatically shorter execution times when using llama-cpp in comparison to traditional C++ constructs. A simple performance comparison might look like this:
start_time = get_current_time();
// Traditional approach
traditional_function();
// Llama-CPP approach
llama_cpp_function();
end_time = get_current_time();
Modularity
Llama-cpp promotes modular coding practices. You can create components that function independently and are easily reusable. Here’s an example:
// Define a module
module MyModule {
void my_function() {
// Custom functionality
}
}
// Using the module in your code
MyModule::my_function();
Data Types and Structures
In llama-cpp, familiar data types such as integers, floats, and strings are prevalent. Understanding how to effectively utilize these types is crucial. Here’s a snippet showcasing user-defined structures:
struct MyData {
int id;
std::string name;
};
MyData data;
data.id = 1;
data.name = "Example";
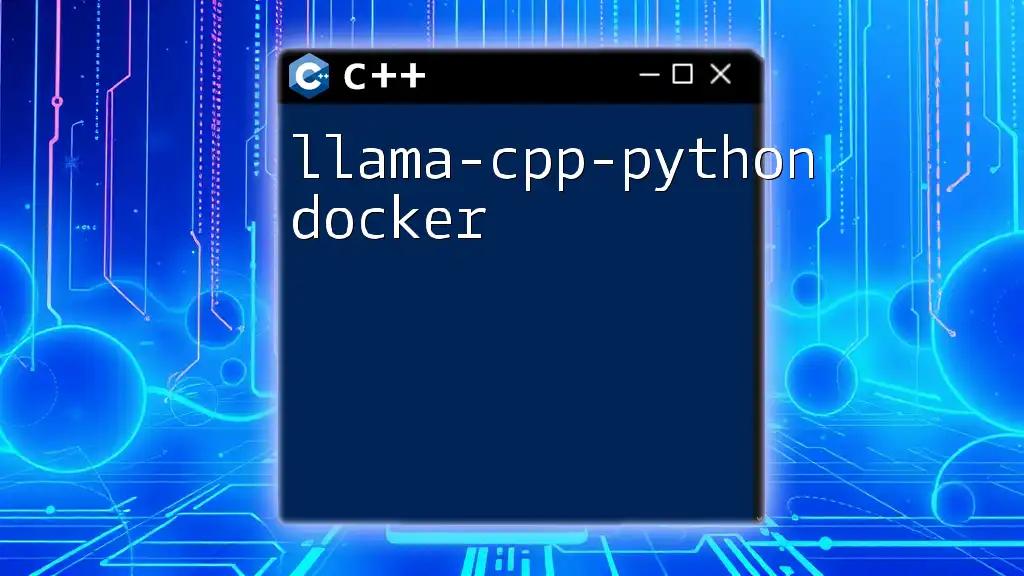
Essential Llama-CPP Commands
Basic Commands
Getting comfortable with llama-cpp begins with mastering some essential commands. For example, to print a message to the console, you might use:
llama_cpp_print("Hello, Llama CPP!");
This command, while simple, showcases how the syntax can lead to quick implementations.
Advanced Commands
Once familiar with the basics, you’ll want to explore more complex commands. For instance, if you’re working with asynchronous operations, you might implement:
llama_cpp_async_function([]() {
// Code to execute asynchronously
});
This facilitates non-blocking operations, improving application responsiveness.
Custom Commands
Creating custom commands is one of the powerful features of llama-cpp. Here’s a quick example:
void MyCustomCommand() {
// Your custom logic here
}
// Execute your custom command
MyCustomCommand();
This promotes enhanced functionality tailored to your specific needs.
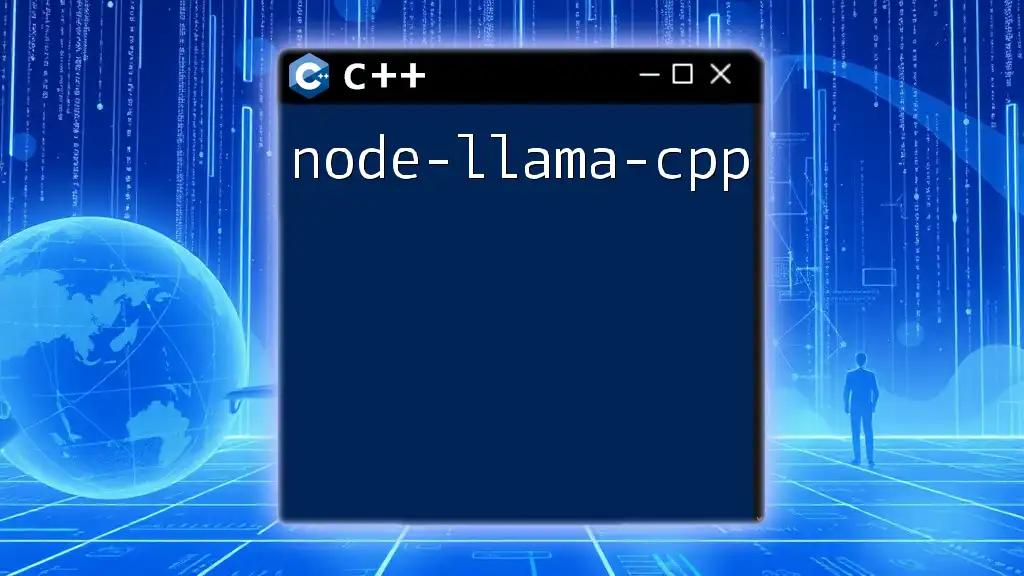
Practical Applications of Llama-CPP
Developing Real-World Applications
Llama-cpp shines in practical viability. For instance, a real-world application such as a file parser can be efficiently implemented as follows:
void parse_file(const std::string &filename) {
// Implementation details
}
Numerous developers have noted that using llama-cpp in complex applications has significantly reduced both development time and error rates, allowing them to focus more on functionality rather than syntactic details.
Building Performance-Optimized Applications
When building performance-optimized applications, utilizing techniques inherent to llama-cpp can lead to substantial performance gains. For instance, consider the difference in code and execution flow:
Before Optimization:
for (int i = 0; i < 1000; i++) {
traditional_operation(i);
}
After Using Llama-CPP:
llama_cpp_parallel_for(0, 1000, [](int i) {
llama_cpp_operation(i);
});
Such optimizations can lead to a reduction in execution time, illustrating the importance of using llama-cpp effectively.
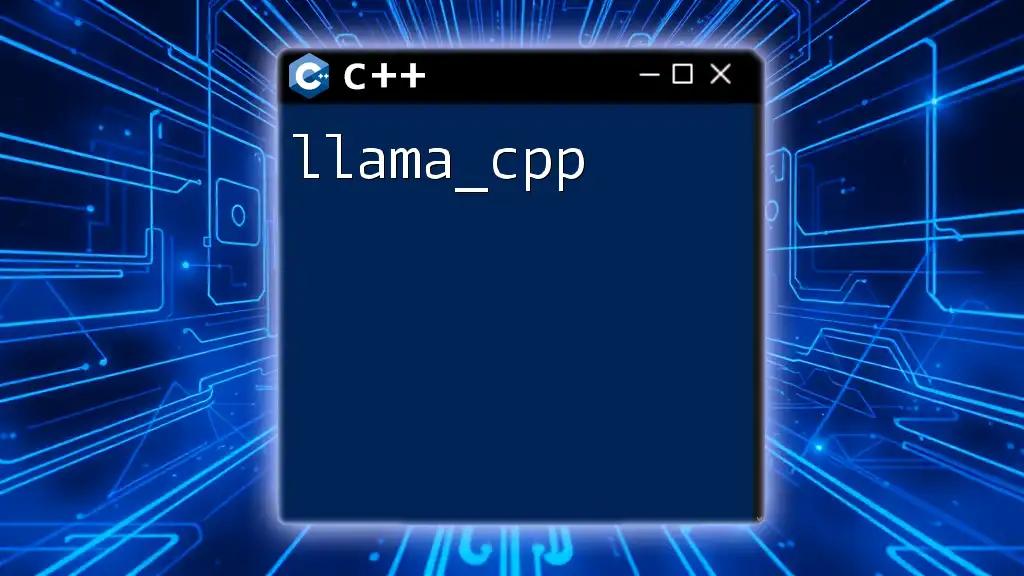
Best Practices for Using Llama-CPP
Effective Coding Techniques
Ensuring that your coding practices remain effective while working with llama-cpp can greatly impact your experience. Emphasize clear naming conventions, consistent syntax, and modularity in all your functions and commands. For example:
void ProcessData(const MyData &data) {
// Logic to process data
}
Such clarity ensures that others can understand your code, promoting better collaboration.
Debugging and Troubleshooting
With every tool, debugging is vital. Common issues, such as missing a required include or syntax errors, can be monitored effectively by using debugging tools. For instance, leveraging the built-in `llama_cpp_debug()` can help trace through your code:
llama_cpp_debug("This is a debug message.");
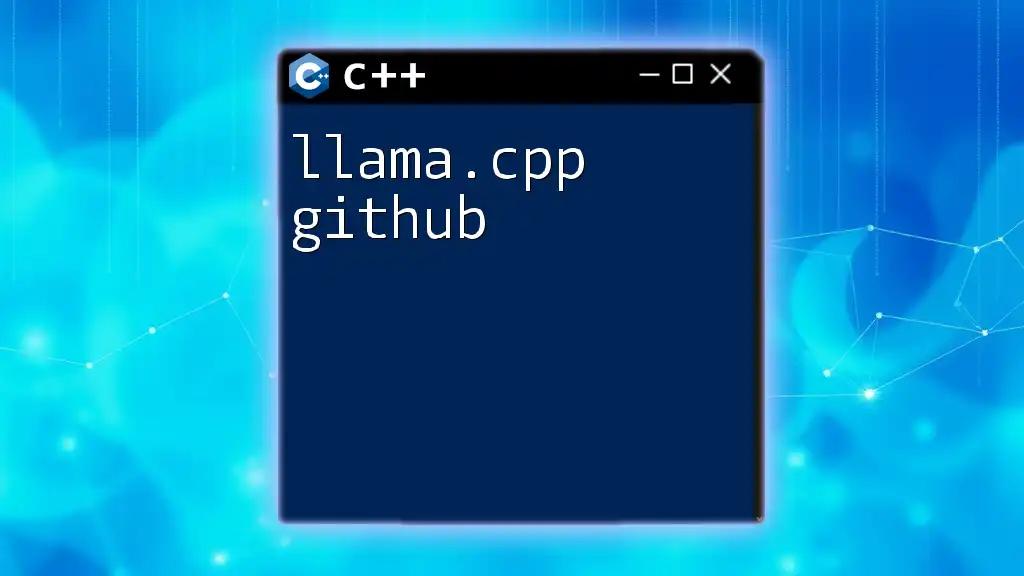
Frequently Asked Questions (FAQ)
Common Queries
A common mistake among new users is the misuse of llama-cpp's data structures. Familiarizing yourself with the types and their proper use is crucial. Additionally, ensure you thoroughly read the documentation to maximize potential.
Answers to often asked questions about Llama-CPP
Q: What platforms can I use Llama-CPP on?
A: You can use it on Windows, macOS, and Linux.
Q: Is it easy to learn for someone new to C++?
A: Yes, Llama-CPP has an intuitive syntax and focuses on reducing complexity.
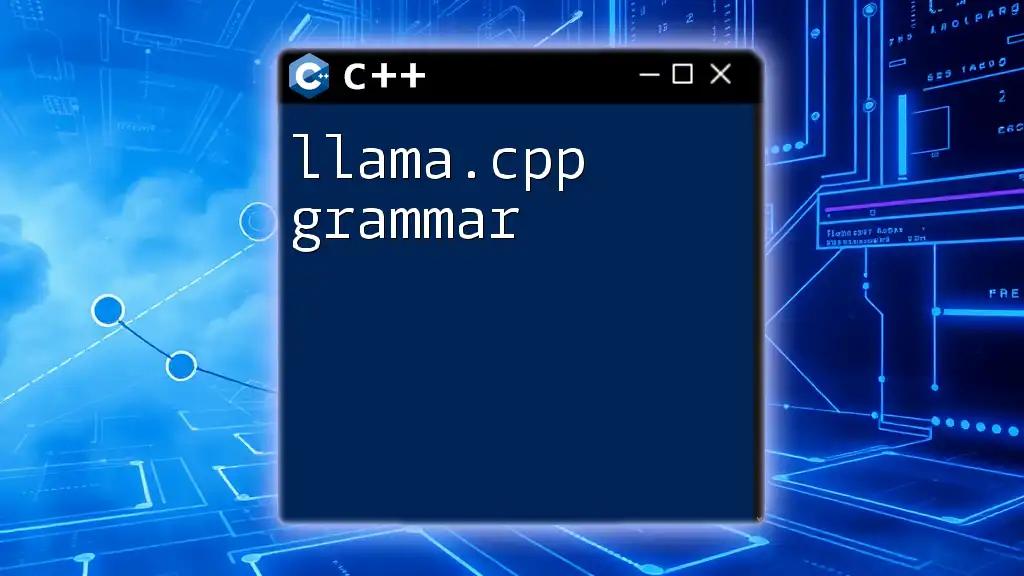
Conclusion
Recap of Learning Points
As outlined in this guide, llama-cpp offers a compelling way to enhance your C++ programming efficiency. By understanding its commands, key features, and applications, you can significantly streamline your coding process.
Additional Resources
To further explore llama-cpp, consider delving into community forums, online courses, or official documentation. Engaging with the community can also provide valuable insights and answers to tricky questions.
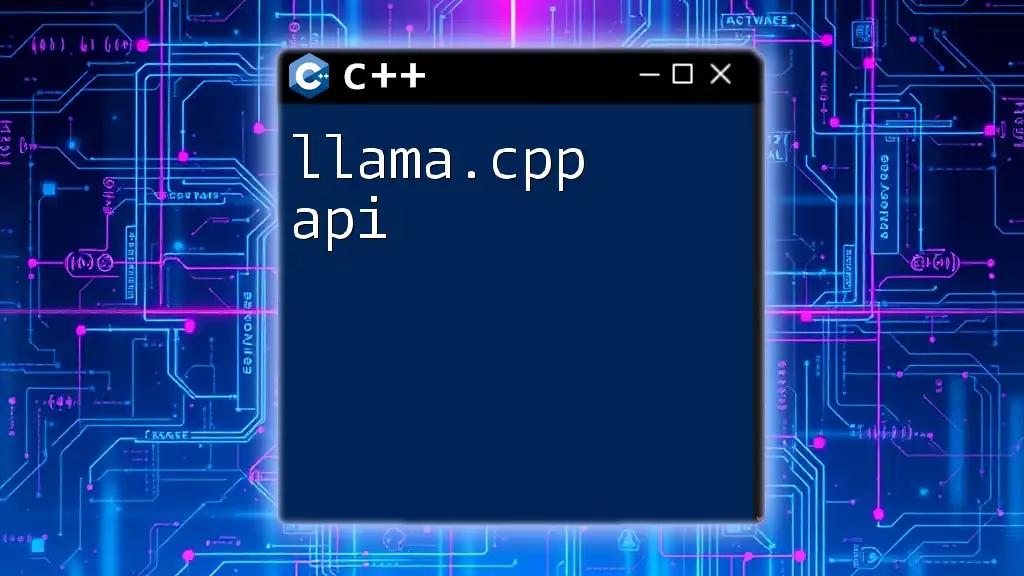
Call to Action
As you embark on your journey with llama-cpp, don’t hesitate to share your experiences. Explore other resources at our company and connect with fellow learners to enrich your understanding of C++. Happy coding!