The "github llama.cpp" project is an implementation for using LLaMA models efficiently in C++, allowing developers to integrate powerful language models into their applications.
Here's an example of a simple C++ snippet that demonstrates how to initialize a LLaMA model:
#include <llama.h>
int main() {
LLaMA model("path/to/llama/model");
model.loadWeights();
std::string output = model.generateText("Input prompt");
std::cout << output << std::endl;
return 0;
}
Understanding Llama.cpp
What is Llama.cpp?
Llama.cpp is a C++ library designed for the implementation and execution of machine learning models. It serves as a bridge for developers who are keen on harnessing the power of C++ for high-performance computing tasks, particularly in the realm of artificial intelligence.
The key features of llama.cpp include a flexible architecture that allows for easy customization of models, efficient data management systems, and built-in support for various machine learning algorithms. Its purpose is to make machine learning more accessible by providing a straightforward yet powerful framework for developers.
Why Use Llama.cpp?
Utilizing C++ for machine learning can significantly enhance performance due to its efficiency and speed. C++ allows for low-level memory manipulation, which can lead to optimized algorithms running faster than those written in higher-level languages.
Some of the benefits of employing llama.cpp include:
- High performance: C++ executes code more quickly than interpreted languages due to its compiled nature.
- Memory control: Developers have greater control over memory usage, crucial for resource-intensive computations.
- Broad applicability: With C++ being widely used in systems programming, gaming, and real-time simulations, llama.cpp can integrate seamlessly into existing C++ projects.
The library's use cases range from academic research in machine learning to commercial applications, such as real-time data analysis and computational modeling.

Getting Started with Llama.cpp
Prerequisites
Before diving into the llama.cpp library, you need to ensure that you have the appropriate software and hardware setup. You will require:
- Operating System: Most versions of Windows, macOS, or Linux will suffice.
- C++ Compiler: Recommended options are GCC or Clang. For Windows users, Microsoft Visual Studio is a robust choice.
- Other Tools: You will need Git for version control and CMake for managing build processes.
Cloning the Repository
To get started with llama.cpp, the first step is to clone its GitHub repository to your local machine. Open your terminal (Command Prompt, PowerShell, or a Unix shell) and enter:
git clone https://github.com/username/repo.git
Once cloned, take a moment to explore the repository's structure. Within the folder, you should find key files such as `README.md` for documentation, `CMakeLists.txt` for building, and source code directories housing the core implementations.
Setting Up the Development Environment
To set up your development environment for llama.cpp:
- Install CMake: This tool simplifies the build process in C++ applications. Follow the installation instructions specific to your operating system.
- Configure Dependencies: Ensure all necessary libraries that llama.cpp depends on are correctly installed. You can typically find this information in the documentation of the repository.
- IDE Setup: Choose an IDE that is well-suited for C++ development. If you're using Visual Studio, create a new project and integrate the llama.cpp repository within it.
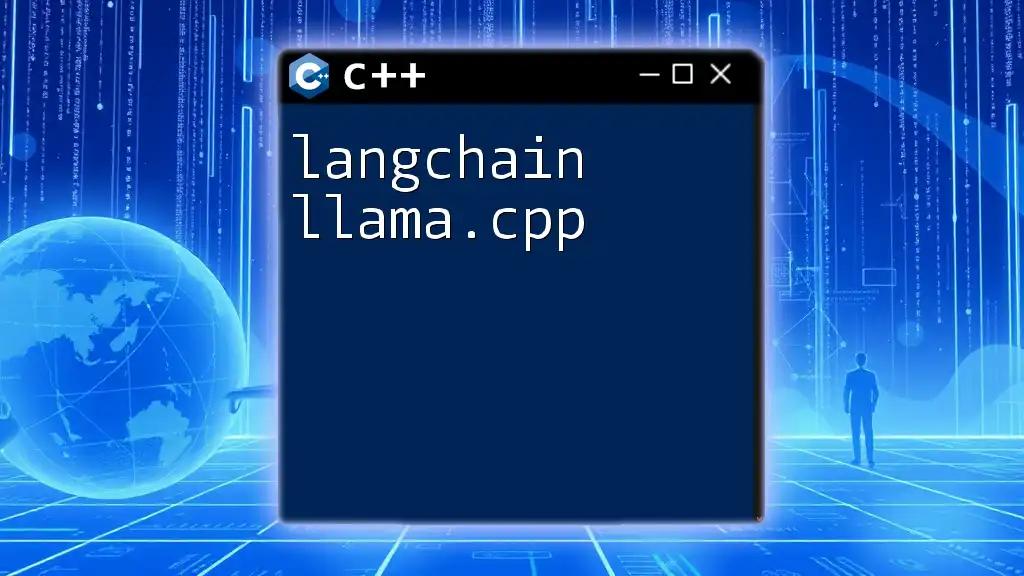
Exploring Core Features of Llama.cpp
Key Components
Llama.cpp comprises several integral components that work together to provide a seamless learning experience. These include:
- Data Handling: Efficient data management routines that facilitate loading, preprocessing, and feeding data into models.
- Model Architecture: Flexible structures allowing for the design of various neural networks and algorithms.
- Training Procedures: Built-in methods for model training, which make it easier to implement various learning strategies.
Basic Commands and Usage
Utilizing basic commands within llama.cpp can be straightforward. Here’s a basic example of how to initiate a simple machine learning task:
#include <iostream>
#include "llama.h"
int main() {
// Initialize model
LlamaModel model;
model.load("model.json"); // Load pre-trained model
// Predict using the model
float prediction = model.predict(inputData);
std::cout << "Predicted value: " << prediction << std::endl;
return 0;
}
This snippet demonstrates the essence of using the llama.cpp library for executing predictions, highlighting its simplicity in interfacing with models.
Advanced Features
As you become more familiar with llama.cpp, you can explore advanced functionalities such as hyperparameter tuning, implementing custom loss functions, or extending model architectures. Each of these capabilities offers further customization for complex machine learning projects.
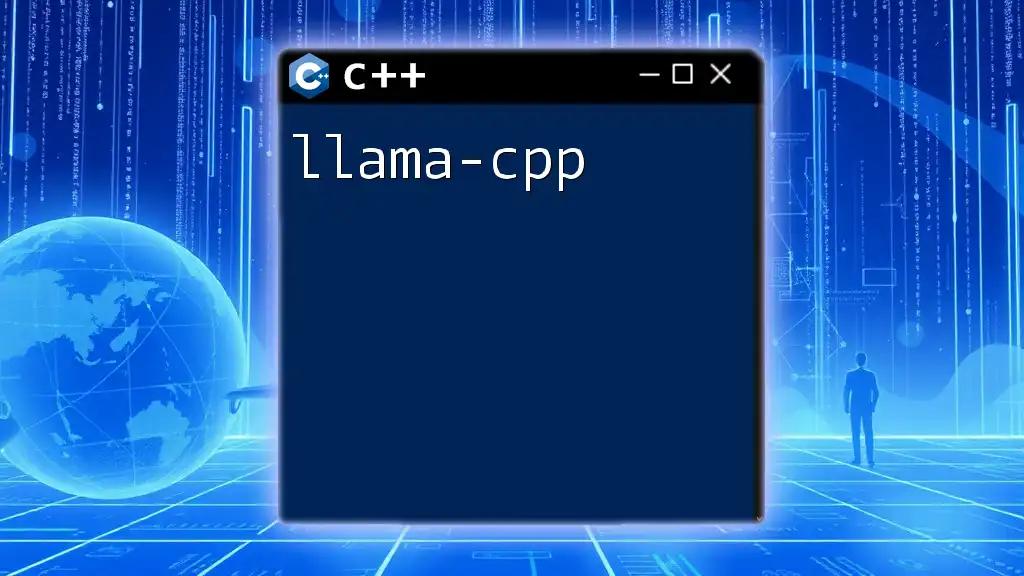
Practical Examples
Implementing a Simple ML Model with Llama.cpp
Let’s build a simple linear regression model using llama.cpp. Follow these steps:
- Load Dataset: You will need to load a dataset and preprocess it.
DataSet dataset = load("data.csv");
- Define Model: Use llama.cpp's framework to define your model.
LlamaModel model;
model.addLayer(new LinearLayer());
model.addLayer(new ActivationLayer("relu"));
- Train Model: Implement a training routine.
model.train(dataset, epochs=100);
- Evaluate Model: Finally, evaluate the model's performance on a test dataset.
float accuracy = model.evaluate(testSet);
std::cout << "Model accuracy: " << accuracy << "%" << std::endl;
Performance Optimization Tips
To maximize the performance of your models using llama.cpp, consider the following techniques:
- Use multi-threading to parallelize computations for data loading and model training.
- Optimize memory usage by using efficient data structures and avoiding unnecessary copies.
- Implement profiling tools to identify bottlenecks in your code and optimize them.
Here's a code snippet showcasing how to implement basic multi-threading:
#include <thread>
void trainModel(LlamaModel& model, DataSet& data) {
model.train(data);
}
int main() {
std::thread t1(trainModel, std::ref(model), std::ref(trainingData));
std::thread t2(trainModel, std::ref(model), std::ref(validationData));
t1.join();
t2.join();
return 0;
}
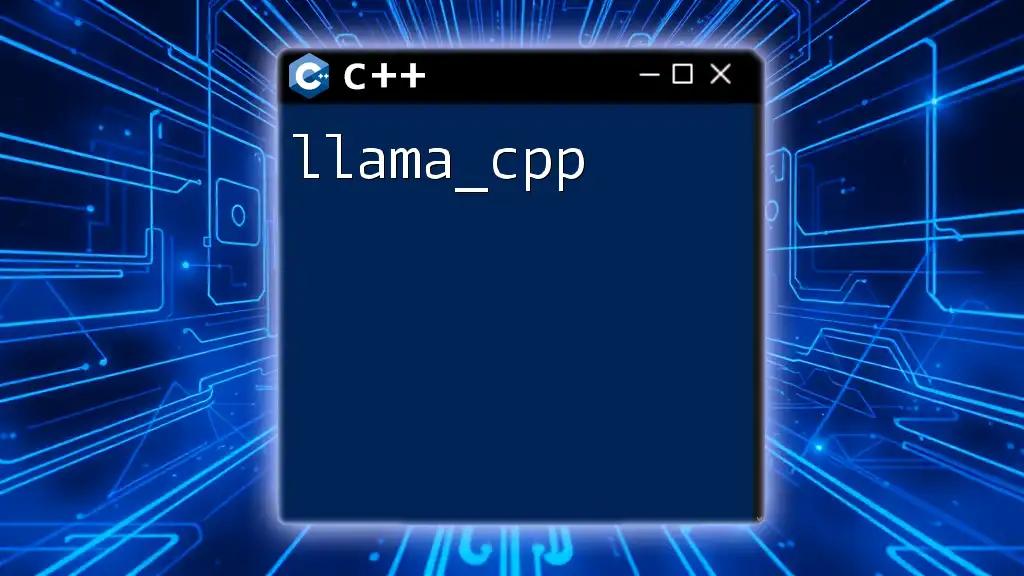
Troubleshooting Common Issues
Common Errors and Their Solutions
When working with llama.cpp, you may encounter several errors. A common one could be related to dependency issues. If you see a missing library error:
- Solution: Ensure all libraries specified in the `README.md` are installed correctly.
Another frequent issue is compilation errors relating to mismatched types. Double-check your data types in function parameters.
Best Practices
To maintain clean and efficient code when utilizing llama.cpp:
- Follow consistent coding standards, including naming conventions and indentation styles.
- Write modular code by encapsulating functionality in functions and classes for better readability.
- Regularly comment your code to clarify complex logic for future reference.
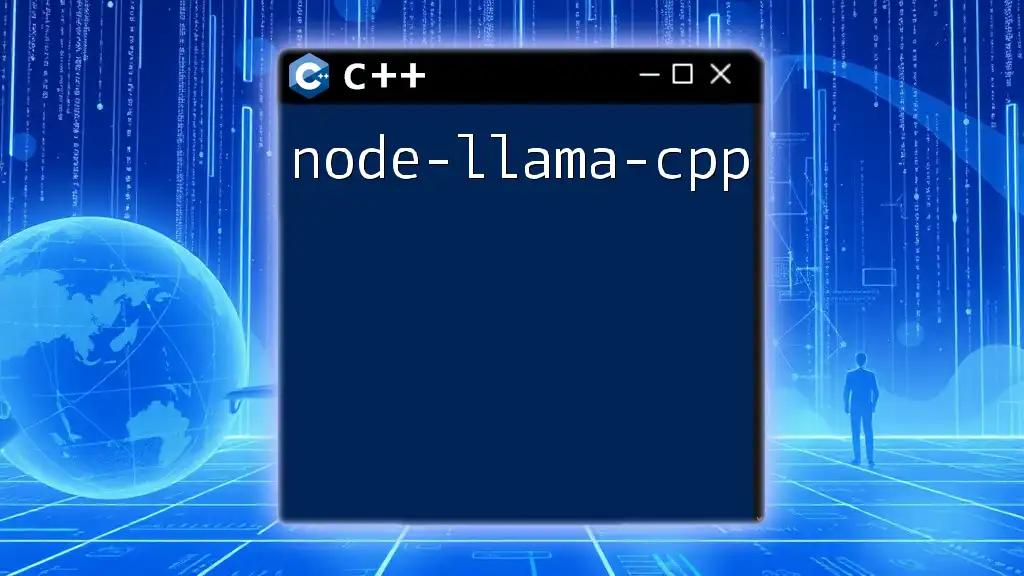
Conclusion
In summary, github llama.cpp presents an optimized pathway for developers eager to explore machine learning through C++. By understanding its core features, setting up your environment correctly, and implementing practical examples, you can significantly enhance your projects.
We encourage you to share your experiences and results using llama.cpp. Your insights contribute to the community and foster collective learning.
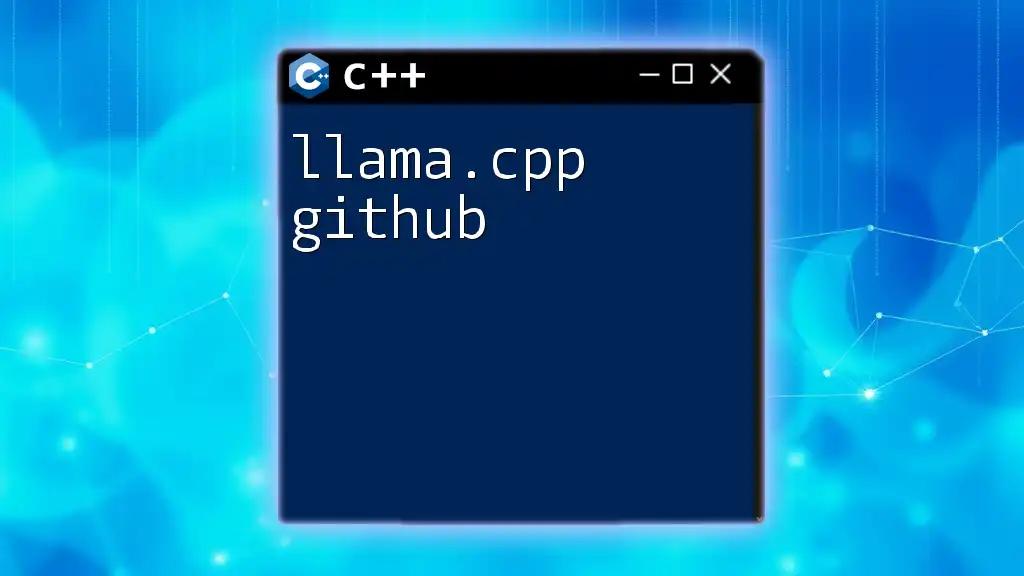
Additional Resources
Links to Documentation
To deepen your understanding, check out the official documentation provided in the llama.cpp repository. This will be your go-to resource for APIs, tutorials, and community insights.
Recommended Tutorials and Courses
For further learning on C++ and machine learning with llama.cpp, explore curated online courses and tutorials that focus on shortcuts to understanding core concepts, ensuring you leverage the full potential of this powerful tool.
Author’s Note
Feel free to share your feedback or personal experiences in the comments section. Your thoughts are invaluable as we create a collaborative learning environment for everyone interested in machine learning and C++.