Langchain is a powerful framework that integrates with large language models like LLaMA (LLaMA.cpp) to streamline tasks such as prompt engineering and text-based applications in a seamless manner.
Here’s a simple example of how to load LLaMA model using langchain with C++:
#include <langchain.h>
int main() {
auto model = LangChain::Llama::load("path/to/llama/model");
std::string prompt = "What is the capital of France?";
std::string response = model.generate(prompt);
std::cout << response << std::endl;
return 0;
}
Understanding LangChain
What is LangChain?
LangChain is a powerful framework designed to create applications powered by language models. It provides developers with the tools needed to effectively manage tasks such as prompt management, model orchestration, and data persistence. With LangChain, you can construct chains of calls to multiple language models, allowing for complex interactions and workflows that mimic human-like reasoning.
How LangChain Works
LangChain operates on a modular architecture that allows different components to interact seamlessly. It abstracts the complexity of language processing by providing a set of APIs that interact with language models—from simple query execution to more advanced features like memory and reasoning.
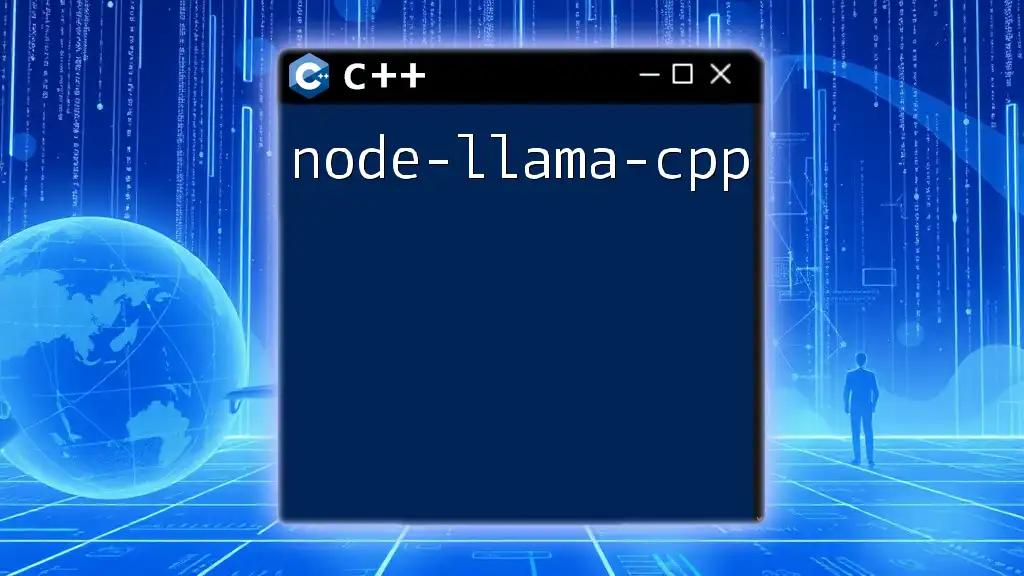
Introduction to Llama.cpp
What is Llama.cpp?
Llama.cpp is a specific backend designed to optimize the inference of language models. It stands out due to its performance efficiencies, especially when dealing with larger models. Its primary role is to facilitate smooth and efficient interactions with language models, enabling developers to harness the full potential of natural language processing.
Key Features of Llama.cpp
Llama.cpp offers several compelling features that make it a go-to choice for language model interactions:
- Performance Optimization: It significantly enhances the speed at which language models can generate outputs, ensuring a responsive user experience.
- Efficiency in Resource Management: Llama.cpp efficiently utilizes system resources, making it suitable for running larger models on less powerful hardware.
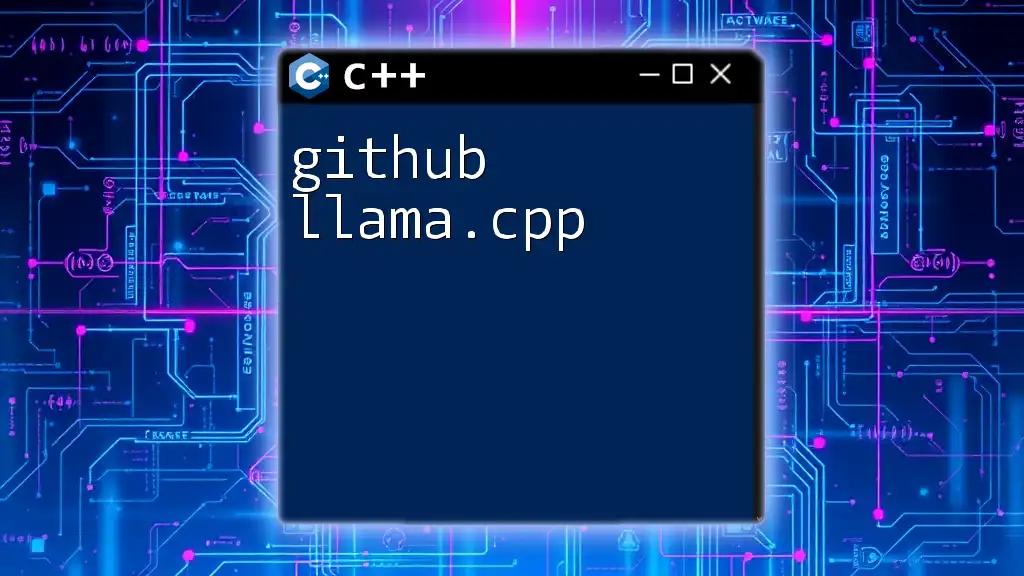
Integration of LangChain and Llama.cpp
Why Use LangChain with Llama.cpp?
Integrating LangChain with Llama.cpp presents numerous benefits:
- Enhanced Performance: The synergy between LangChain's orchestration capabilities and Llama.cpp's efficiency can lead to faster processing times and lower latency.
- Better Resource Utilization: By leveraging Llama.cpp’s resource management, applications built on LangChain can run more smoothly, even in resource-constrained environments.
Setting Up LangChain with Llama.cpp
Prerequisites
Before you get started, ensure that you have installed the necessary tools and libraries. This usually includes a C++ compiler and the corresponding development environment for your operating system.
Installation Guide
Steps to Install LangChain:
- Clone the LangChain repository from GitHub.
- Install any dependencies as specified in the documentation.
Steps to Integrate with Llama.cpp:
- Clone the Llama.cpp repository.
- Link Llama.cpp within your LangChain setup to enable optimizations.
Configuration
Setting Up Environment Variables
To maximize performance, you may need to set certain environment variables. This includes variables that specify paths for caching or model configurations.
Configuring LangChain to Use Llama.cpp
An example configuration might involve specifying the use of Llama.cpp as the backend in your LangChain settings file:
{
"backend": "Llama.cpp",
"model_path": "/path/to/your/model"
}
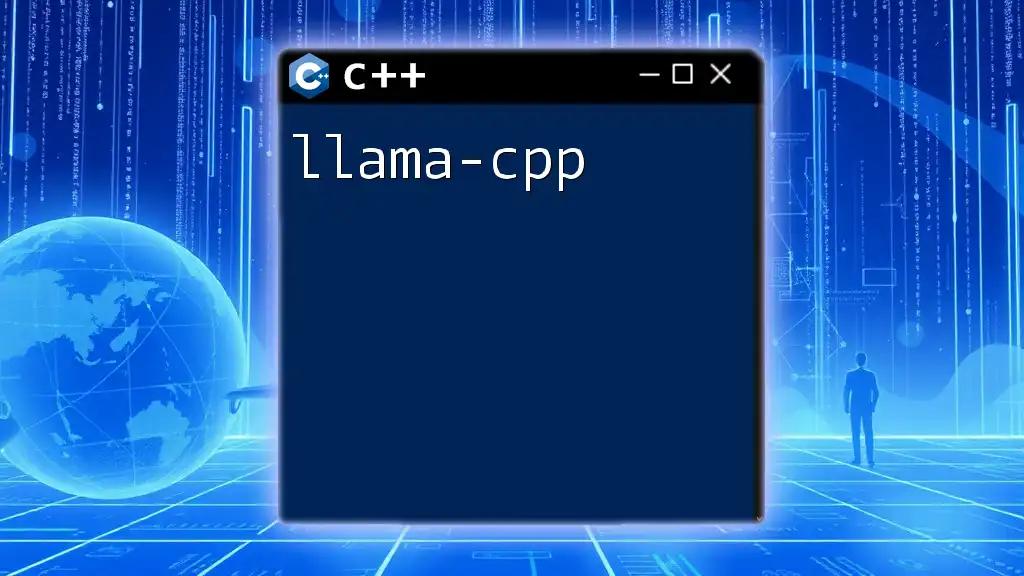
Practical Implementation
Writing Your First Command with LangChain and Llama.cpp
Now that you have everything set up, let's write your first command using LangChain with Llama.cpp. Here is a simple step-by-step example that will guide you:
- Set up a new project directory for your application.
- Write your first cpp command.
#include <iostream>
#include "langchain.h"
int main() {
// Initialize LangChain
LangChain lc;
lc.initialize();
// Use Llama.cpp for language processing
lc.process("Hello, world!", "Llama.cpp");
return 0;
}
In this snippet, we initialize the LangChain framework and call the `process` method, passing a string and specifying Llama.cpp as the method of processing.
Advanced Commands and Techniques
Using Custom Commands
LangChain allows you to create custom commands that can streamline specific tasks. This can be particularly useful for repetitive operations. For example, you might create a command that formats text in a particular way before processing:
void formatAndProcess(const std::string& input) {
std::string formatted = formatText(input);
lc.process(formatted, "Llama.cpp");
}
Debugging and Error Handling
Debugging is an essential part of the development process. Here are some common troubleshooting steps:
- Common Bugs: Watch out for errors related to invalid configurations or missing dependencies.
- Tips for Effective Debugging: Utilize logging to track the flow of data through your commands.
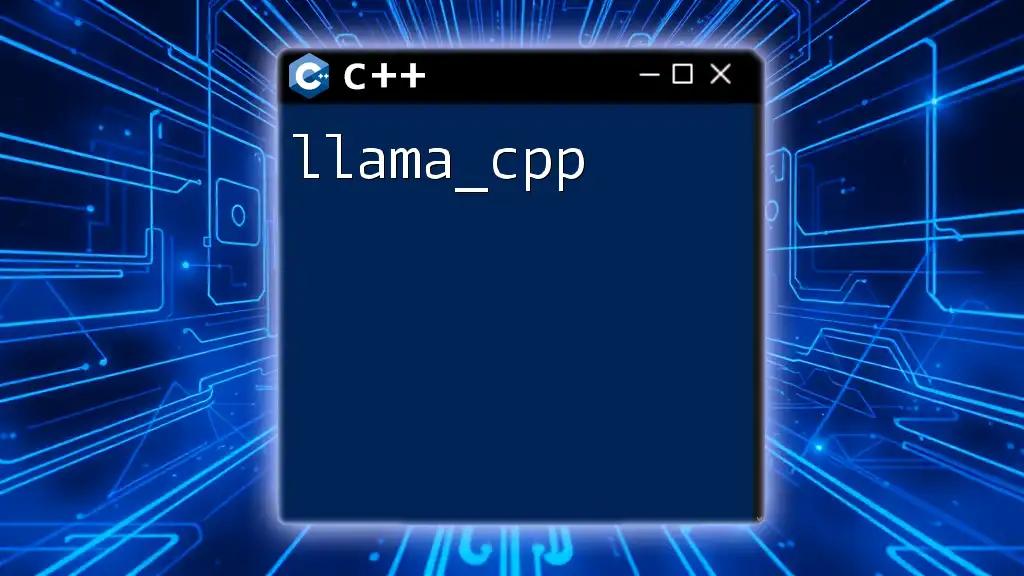
Best Practices
Optimizing Performance
To ensure that your applications run smoothly, here are a few optimization tips:
- Use efficient data structures that minimize resource usage.
- Profile your code to identify bottlenecks and optimize those sections.
Ensuring Scalability
For applications built with LangChain and Llama.cpp, scalability can be achieved by:
- Designing systems that can handle increased loads without performance degradation.
- Implementing caching strategies to reduce repeated processing of the same inputs.
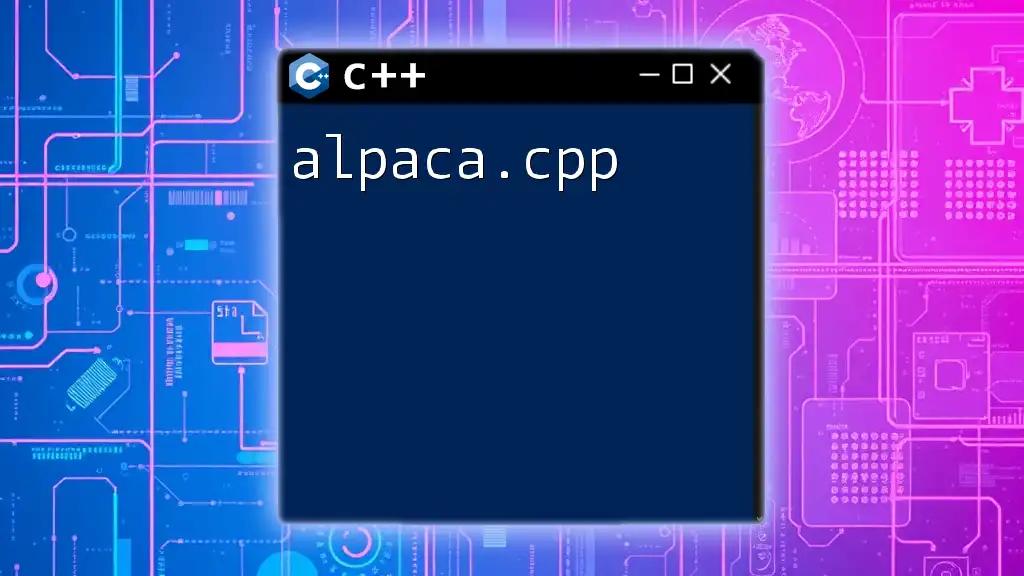
Conclusion
Summary of Key Points
In this article, we explored the integration of LangChain and Llama.cpp, emphasizing their capabilities and advantages. We covered the setup process, practical implementations, and best practices for maximizing performance.
Future of LangChain and Llama.cpp
The landscape of language processing technologies is ever-evolving. As more developers adopt frameworks like LangChain and Llama.cpp, future updates promise to bring even more features, improving performance and expanding capabilities.

Additional Resources
Documentation Links
- [Official LangChain documentation](https://example.com/langchain-docs)
- [Llama.cpp official GitHub repository](https://github.com/example/llama.cpp)
Recommended Communities and Forums
Joining forums or communities focused on C++ and language models can greatly enhance your learning experience. Engage with fellow developers, share knowledge, and seek advice as you embark on your journey with LangChain and Llama.cpp.
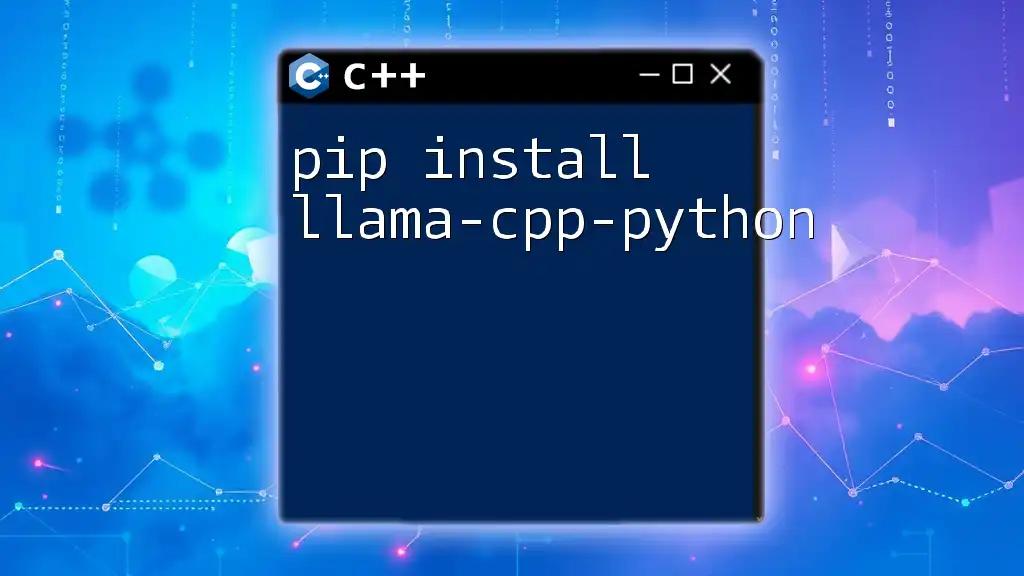
Call to Action
Start experimenting with LangChain and Llama.cpp today! Your journey into the powerful world of language model applications begins now. Consider joining our community or subscribing for additional tutorials and tips to enhance your understanding and skills further.