The term "llama.cpp mixtral" refers to a specific implementation involving LLaMA (Large Language Model) models in a C++ environment, particularly focusing on efficient and optimizable command execution. Here's a simple C++ code snippet demonstrating a basic structure to run a model:
#include <iostream>
#include "llama.h" // Assuming llama.h contains the necessary LLaMA model definitions
int main() {
LlamaModel model("path/to/model");
model.load(); // Load the model
std::string output = model.infer("Hello, how are you?");
std::cout << output << std::endl; // Display the model's output
return 0;
}
Understanding `llama.cpp`
What is `llama.cpp`?
`llama.cpp` is an advanced C++ library that aims to make programming with C++ more efficient and accessible. It provides a set of commands designed to streamline various programming tasks, enhancing productivity for both novice and experienced developers. The core functionalities enable users to execute commands effortlessly, making it a powerful tool in any programmer’s toolkit.
How `llama.cpp` Works
The technical architecture of `llama.cpp` is built around a command-oriented approach. This structure allows users to interact with the language through predefined commands, minimizing the complexity typically associated with C++ programming.
The command structure is intuitive, employing a clean syntax that readily maps to the expected functionalities. This simplicity promotes better understanding, helping users to grasp C++ concepts quickly.
When it comes to performance metrics, `llama.cpp` stands out. Its lightweight design ensures minimal overhead while executing tasks, making it suitable for various applications, from simple scripts to large scale projects.
Benefits of Using `llama.cpp`
Utilizing `llama.cpp` provides numerous benefits. The most significant include:
- Speed and Efficiency: The commands are optimized for performance, allowing for quicker execution compared to traditional coding.
- Ease of Use: The user-friendly command structure makes it especially beneficial for new developers learning C++.
- Extensibility: Its modular nature encourages integration with other tools and libraries, enhancing functionality and adaptability.
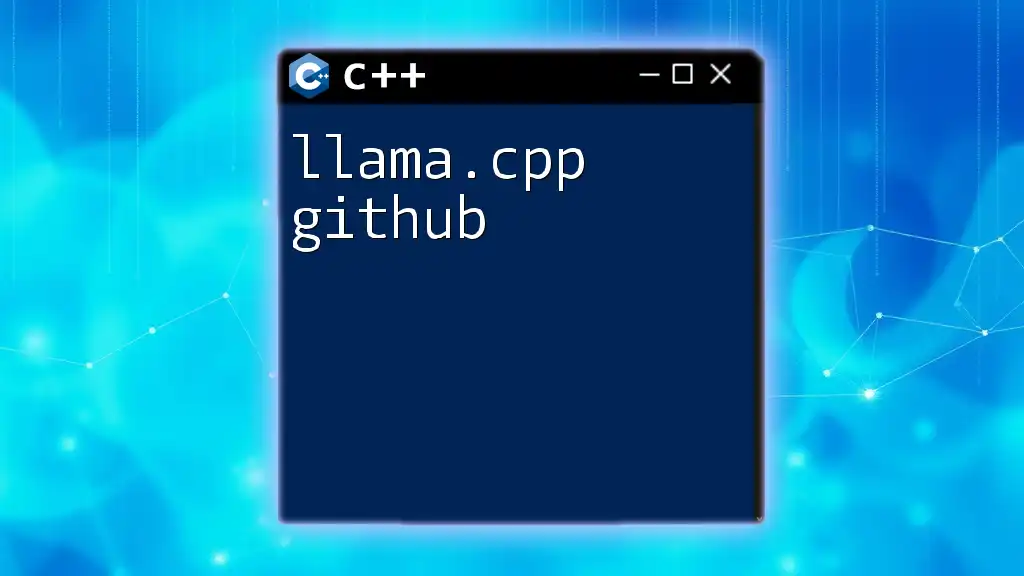
Introduction to Mixtral
Overview of Mixtral
Mixtral is an innovative interface that enhances the command management capabilities of `llama.cpp`. It simplifies the process of learning and executing commands, making it a perfect complement for anyone working with `llama.cpp`. By leveraging Mixtral, users can gain deeper insight into command structures while benefiting from additional functionalities.
Key Features of Mixtral
One of the standout aspects of Mixtral is its comprehensive user interface (UI), designed specifically for ease of navigation and execution. With a clean layout, even those new to programming will find it approachable.
Command management is another crucial feature of Mixtral. It facilitates creating, modifying, and executing commands seamlessly, greatly reducing the time taken to write and test code. Additionally, Mixtral incorporates robust error handling mechanisms, enabling users to debug their commands effectively.
Advantages of Using Mixtral with `llama.cpp`
Integrating Mixtral with `llama.cpp` unleashes enhanced usability. The unified command structure aids developers in seamlessly switching between commands without needing to memorize syntax.
Improved debugging capabilities within Mixtral allow users to identify and rectify issues in their commands more readily. This is particularly beneficial for beginners who may struggle with typical debugging processes.
Streamlined workflows fostered through Mixtral make programming more efficient, freeing developers to focus on creativity rather than syntax issues.
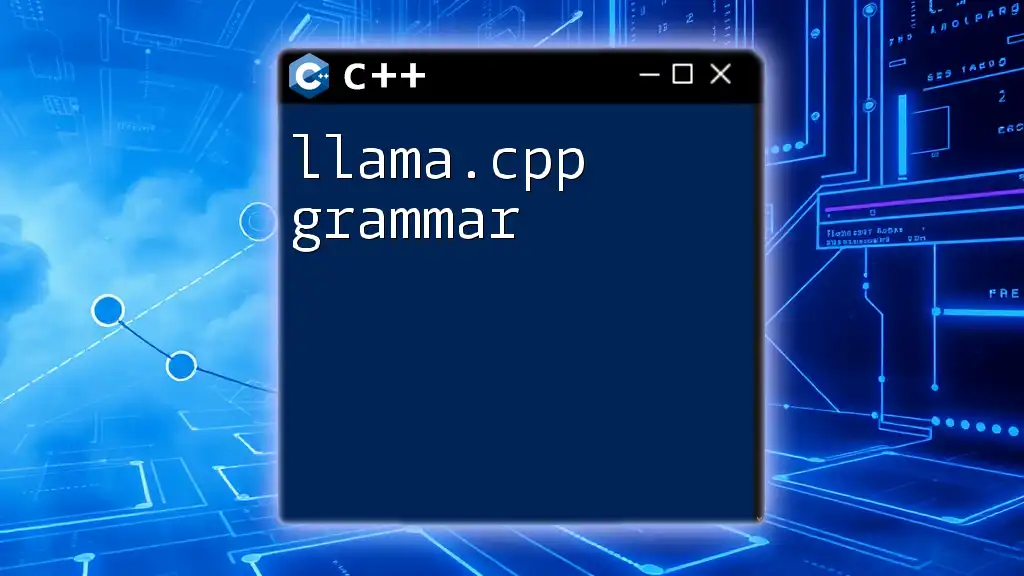
Getting Started with `llama.cpp` and Mixtral
Setting Up Your Environment
To begin using `llama.cpp` with Mixtral, you need to ensure your environment is properly set up.
-
Required Software: Ensure you have a suitable C++ compiler, and install `llama.cpp` and Mixtral as required.
-
Configuration Steps: Follow the setup instructions provided in their respective documentation to ensure everything is installed correctly.
-
Validation of Setup: Once configured, run a simple test command to confirm that both `llama.cpp` and Mixtral are functional.
Basic `llama.cpp` Commands
Understanding basic commands is crucial for effective use of `llama.cpp`. Here are some foundational examples:
Example 1: Hello World Command
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This simple program prints "Hello, World!" to the console, illustrating the basic structure of a C++ program.
Example 2: Simple Arithmetic Operation
#include <iostream>
int main() {
int a = 5, b = 7;
std::cout << "Sum: " << (a + b) << std::endl;
return 0;
}
In this code, we perform a basic arithmetic operation to sum two integers and print the result, showcasing the straightforward syntax of `llama.cpp`.
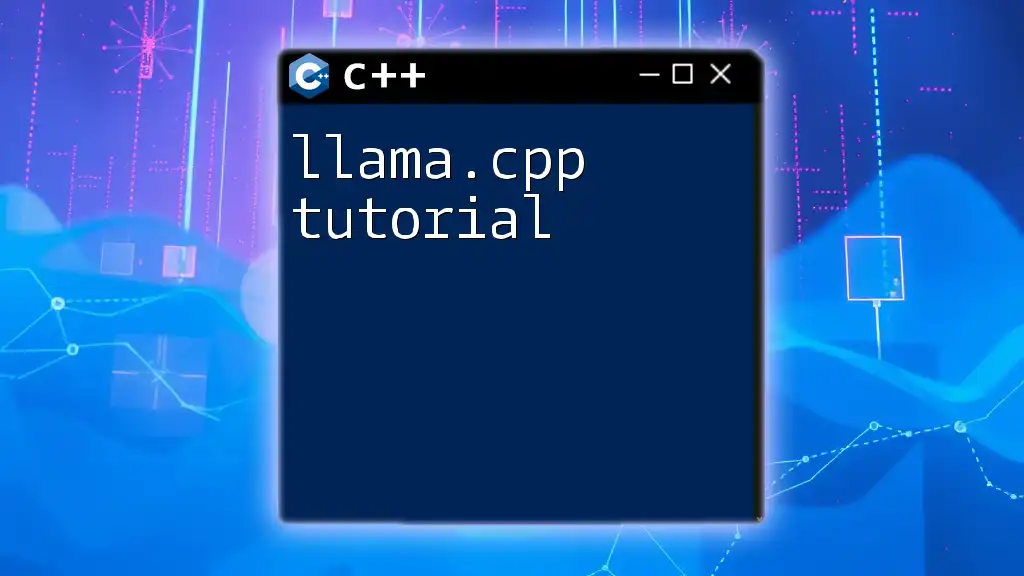
Advanced Commands in `llama.cpp`
Control Flow Statements
Control flow statements enable developers to dictate the logic of their programs. One of the most commonly used statements is the if-else statement.
If-Else Statement Syntax
int number = 10;
if (number > 0) {
std::cout << "Positive" << std::endl;
} else {
std::cout << "Negative or Zero" << std::endl;
}
This snippet checks if a number is positive. If so, it prints "Positive"; otherwise, it states "Negative or Zero."
Loops: For Loop and While Loop
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
The code above demonstrates a simple `for` loop that iterates five times. Each iteration prints its current value, illustrating how loops can control program flow efficiently.
Functions in `llama.cpp`
Functions are essential for creating modular and reusable code.
Defining and Calling Functions
void greet() {
std::cout << "Welcome to Mixtral!" << std::endl;
}
int main() {
greet();
return 0;
}
In this example, we define a function named `greet()` that prints a welcome message. When called in the `main()` function, it showcases the power of functions in maintaining cleaner code structures.
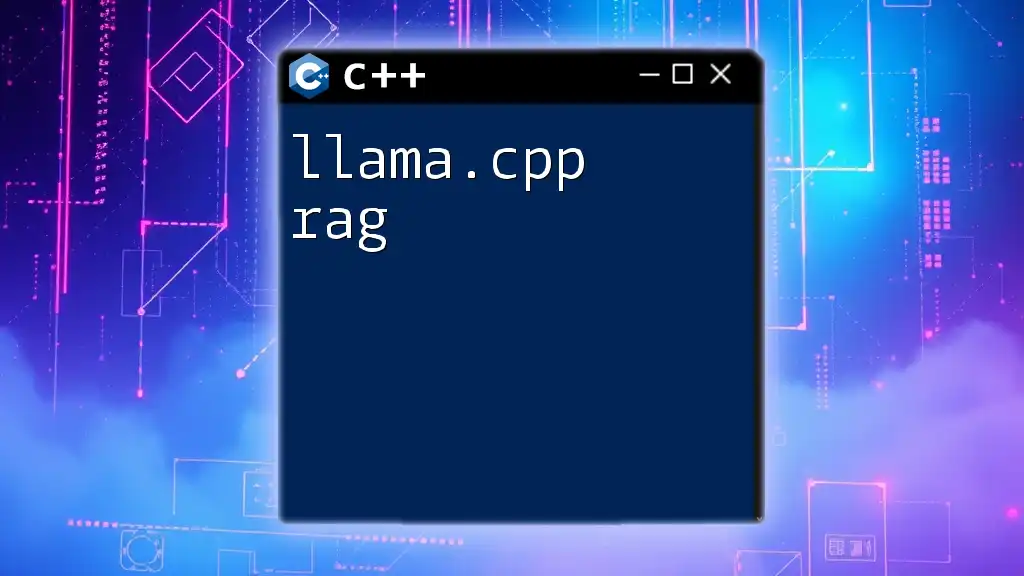
Mixtral's Features in Action
Utilizing Mixtral’s Command Management
Mixtral excels in command management, offering an intuitive way to create and manipulate commands.
Example: Custom Command Creation
mixtral::addCommand("greet", []() {
std::cout << "Hello from Mixtral" << std::endl;
});
In this example, we create a custom command `greet` that outputs a message. This highlights how Mixtral allows for creating tailored functionalities that enhance user interaction with programming commands.
Debugging with Mixtral
Debugging is made easier with Mixtral's built-in features.
Example: Error Handling
try {
// Code that might throw an error
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
This snippet showcases how to implement basic error handling. By wrapping your code in a try-catch block, you can manage exceptions more gracefully, providing better feedback when issues arise during execution.
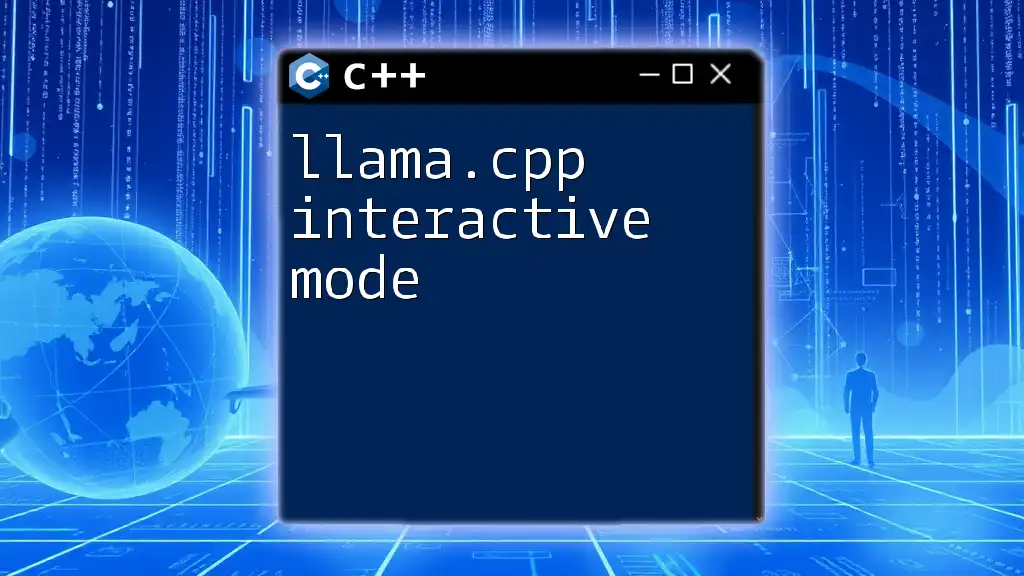
Best Practices for Using `llama.cpp` with Mixtral
Code Quality and Style
Maintaining high code quality is vital. Clean and well-styled code promotes better understanding and collaboration. Here are a few tips:
- Use meaningful variable names that describe their purpose.
- Follow consistent indentation and formatting guidelines.
- Comment your code where necessary to provide clarity on complex sections.
Optimizing Performance
Performance optimization can dramatically affect the efficiency of your program. Techniques to enhance execution speed include:
- Avoiding unnecessary calculations inside loops where values can be computed beforehand.
- Minimizing memory allocation in tight loops to alleviate performance bottlenecks.
- Leveraging Mixtral’s features to analyze and optimize resource usage.
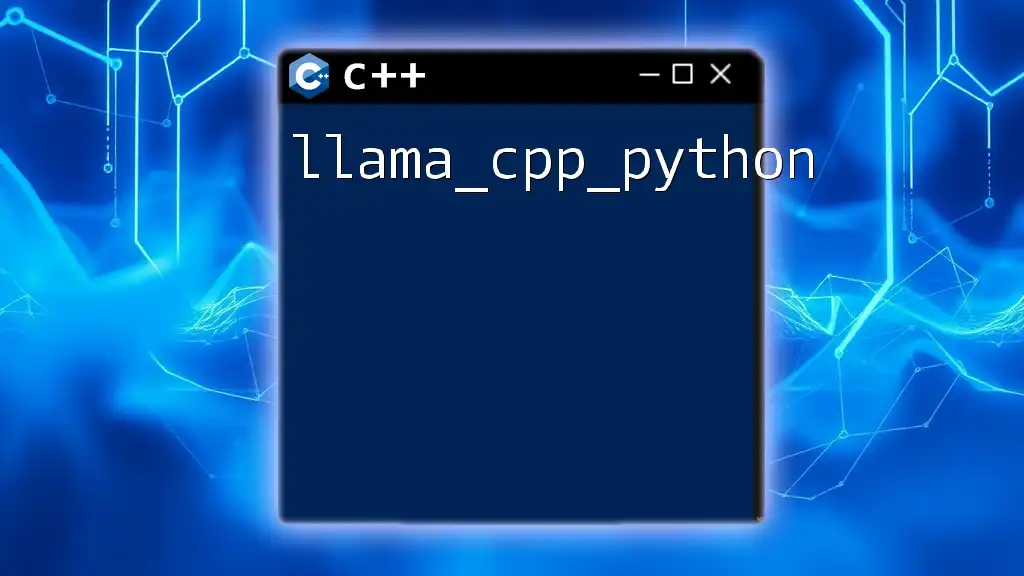
Conclusion
As we've explored, using `llama.cpp mixtral` empowers developers to harness a robust C++ library in conjunction with an efficient command management interface. Understanding these tools greatly enhances your programming capabilities, allowing for efficient coding practices and simplified debugging processes.
As you delve deeper into `llama.cpp`, practice executing commands and experimenting with various functionalities. Joining the community can provide even more resources to support your learning journey. Happy coding!
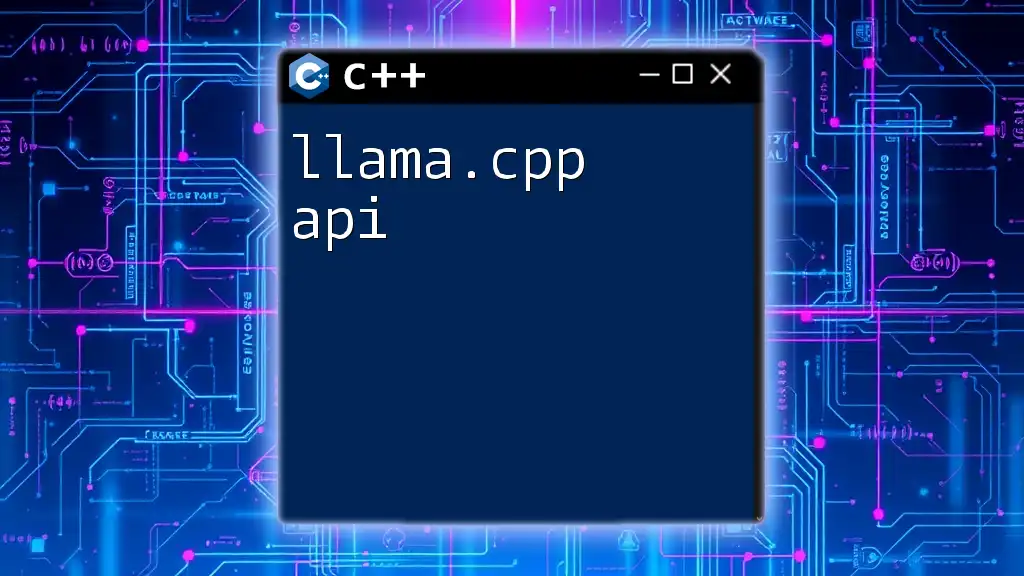
Additional Resources
Recommended Reading
Explore online tutorials, official documentation, and related books that deepen your understanding of `llama.cpp`.
Forums and Communities
Get connected with fellow learners and experts in programming forums to exchange knowledge and solutions.
Tools and Plugins
Identify tools that enhance the user experience when working with `llama.cpp` and Mixtral to bolster your learning and functional capacity.