The Llama C++ REST API allows developers to interact with the Llama C++ model using HTTP requests for tasks such as text generation and processing.
Here’s a simple example of how to make a POST request to the Llama C++ REST API using C++ with the `libcurl` library:
#include <curl/curl.h>
int main() {
CURL *curl;
CURLcode res;
curl_global_init(CURL_GLOBAL_ALL);
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://your-llama-cpp-api-endpoint");
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "{\"input\":\"Hello, Llama!\"}");
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, "Content-Type: application/json");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
What is Llama CPP?
Llama CPP is a powerful framework tailored for C++ developers interested in building web applications and services, specifically RESTful APIs. Its core innovation lies in its simplicity and efficiency, making the development process less cumbersome while allowing developers to leverage the full potential of C++.
The characteristics of Llama CPP include:
- High performance: Llama CPP is designed to handle multiple requests efficiently.
- Ease of use: The framework's syntax is simplified, lowering the barrier to entry for developers new to C++.
- Modular architecture: It offers modular components that enable developers to build scalable and maintainable applications quickly.
Understanding Llama CPP’s capabilities is crucial for anyone looking to create robust web services or APIs.
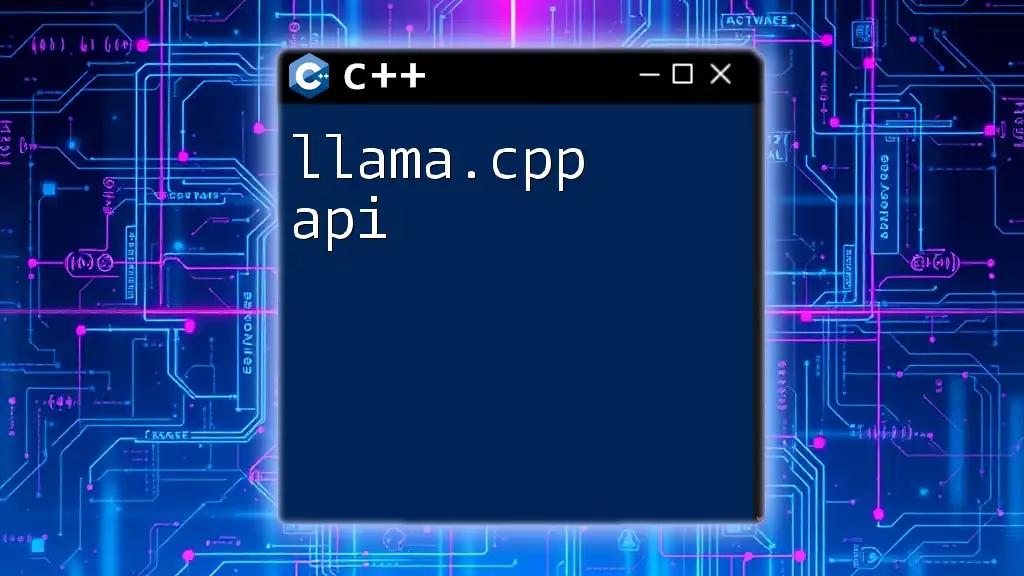
Understanding REST API
Explanation of REST
REST (Representational State Transfer) is an architectural style that outlines a set of constraints for creating web services. It emphasizes a stateless communication protocol, typically HTTP, and relies on standard methods such as GET, POST, PUT, and DELETE to operate on resources.
The core principles of REST include:
- Stateless: Each API call from a client contains all the information needed to process the request. The server does not store any state about the client session on its side.
- Client-Server Separation: The client and server operate independently, allowing each to evolve separately.
- Uniform Interface: A uniform method of communication, which simplifies the architecture and decouples the implementation from the service.
Advantages of Using REST APIs
REST APIs offer numerous benefits, making them a popular choice for developers:
- Scalability: The stateless nature of REST allows for easy scalability, as servers can manage requests independently.
- Language-agnostic: Clients can interact with REST APIs written in different programming languages, making them versatile for various applications.
Common Terminologies in REST
Understanding the basic terminologies is vital for anyone working with REST APIs:
- Resources: These are the objects or representations managed by the API, such as user data or product details.
- Endpoints: Specific URLs through which resources can be accessed.
- HTTP methods: The commands used to retrieve or manipulate resources, including GET (retrieve), POST (create), PUT (update), and DELETE (remove).
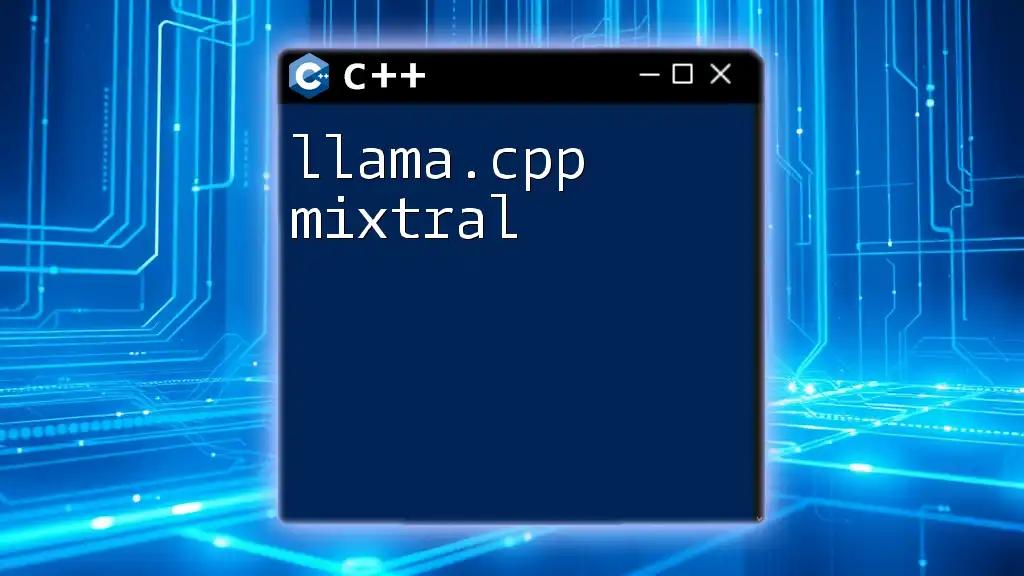
Setting Up Llama CPP for REST API Development
System Requirements
Before diving into Llama CPP, ensure your system meets the hardware and software prerequisites:
- Hardware requirements: A machine capable of running a modern C++ compiler and has sufficient RAM.
- Software requirements: The latest version of a C++ compiler, along with libraries like Boost for advanced features.
Installation Steps
- Downloading Llama CPP: Visit the official Llama CPP repository and clone or download the framework.
- Setting up your development environment: Choose a suitable IDE such as Visual Studio or CLion.
- Required libraries and dependencies: Ensure you have all necessary libraries installed, particularly those specified in the Llama CPP documentation.
Basic Configuration
Once everything is set up, it's time to create your new Llama CPP project. Organize your directory structure effectively, including essential files such as `main.cpp`, configuration files, and any resource directories. This will help maintain clarity as your project grows.

Creating a Simple REST API with Llama CPP
Step 1: Project Structure
A well-organized project structure enhances maintainability. Essential components include:
- `src/` folder: Contains source files.
- `include/` folder: Header files defining function interfaces.
- `CMakeLists.txt` file: For project configuration and dependencies.
Step 2: Implementing RESTful Endpoints
Creating Endpoints
Llama CPP simplifies the process of defining endpoints. Here's how you can create a simple `GET` endpoint:
void getEndpoint() {
llama::api::addRoute("/api/data", http::GET, [](auto req, auto res) {
res.send("{\"msg\": \"Hello, world!\"}");
});
}
In this code snippet, we define an endpoint at `/api/data` that returns a JSON message when a client makes a GET request.
Handling Multiple Methods
Handling multiple HTTP methods within Llama CPP is straightforward. For instance, to implement a `POST` endpoint, you can write:
void addDataEndpoint() {
llama::api::addRoute("/api/data", http::POST, [](auto req, auto res) {
auto data = req.body(); // Parsing body data
// Process your data here
res.send("{\"msg\": \"Data added!\"}");
});
}
This example captures the body data from the request and responds with a confirmation.
Step 3: Testing Your API
Testing is a crucial part of API development. Using tools such as Postman or curl allows you to make requests to your endpoint and verify responses. Here’s an example of a curl command to test your endpoint:
curl -X GET http://localhost:8000/api/data
This command sends a GET request to your running API and should return the set JSON message.
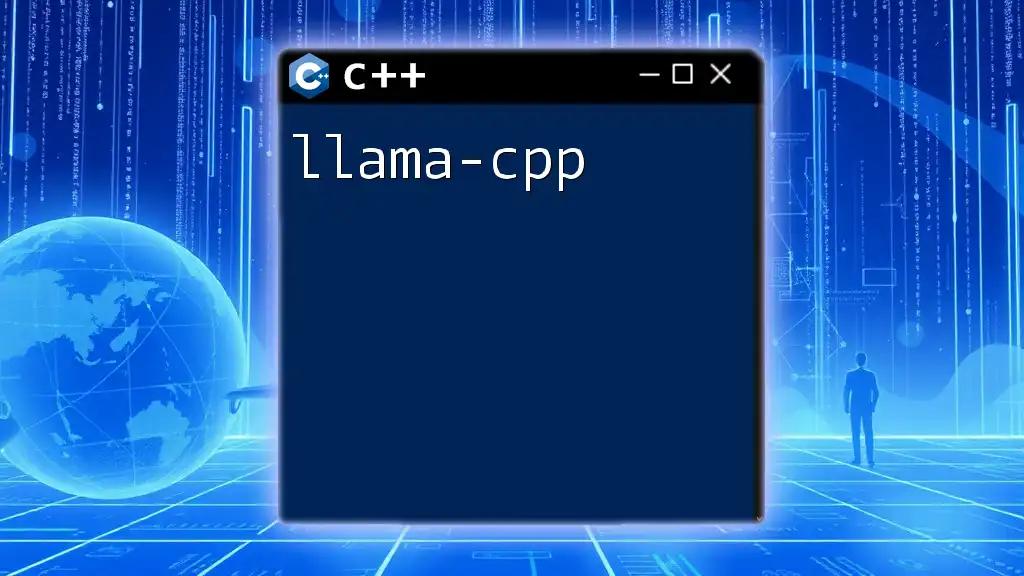
Consuming REST APIs with Llama CPP
Making API Calls
Not only can Llama CPP be used to create REST APIs, but it can also consume them. To make a `GET` request to an external API, you could write:
llama::http::get("http://api.example.com/data", [](auto response) {
std::cout << response.body(); // Handling response
});
In this snippet, we make a GET request to an external API, and upon receiving the response, we print its body to the console.
Error Handling Best Practices
When working with REST APIs, effective error handling is essential. Consider the various HTTP status codes your API might encounter. Here’s a simple error-handling strategy:
if (response.status() != 200) {
std::cerr << "Error: " << response.status() << std::endl;
}
By implementing structured error handling, you can provide meaningful feedback to clients regarding issues they might encounter while interacting with your API.
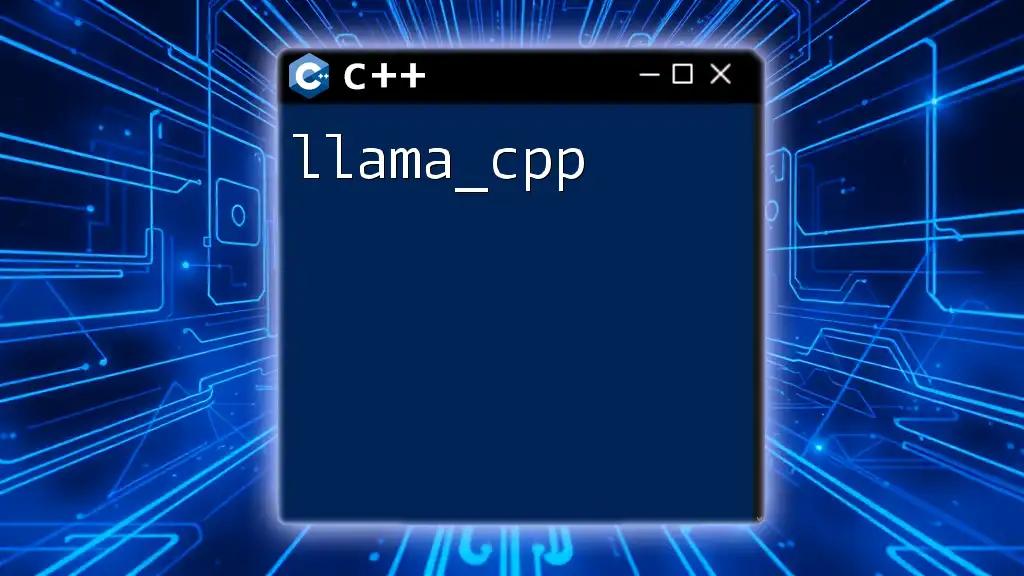
Advanced Features of Llama CPP for REST APIs
Middleware Support
Middleware is an essential component of modern APIs, allowing you to pause requests for tasks like authentication, logging, and rate limiting. In Llama CPP, you can implement middleware easily:
llama::api::use([](auto req, auto res, auto next) {
std::cout << "Middleware triggered!" << std::endl;
next(); // Call next middleware or endpoint
});
This code defines a middleware function that logs when a request reaches the server and then proceeds to the next step in the chain.
Authentication and Security
Incorporating authentication mechanisms like API keys or JWT tokens enhances security. Secure your endpoints by validating tokens for requests. For instance, check for a valid API key before processing any endpoint:
if (req.headers["Authorization"] != "Bearer YOUR_API_KEY") {
res.status(401).send("Unauthorized");
}
This conditional checks if the API key is present and valid before allowing access to the resource.
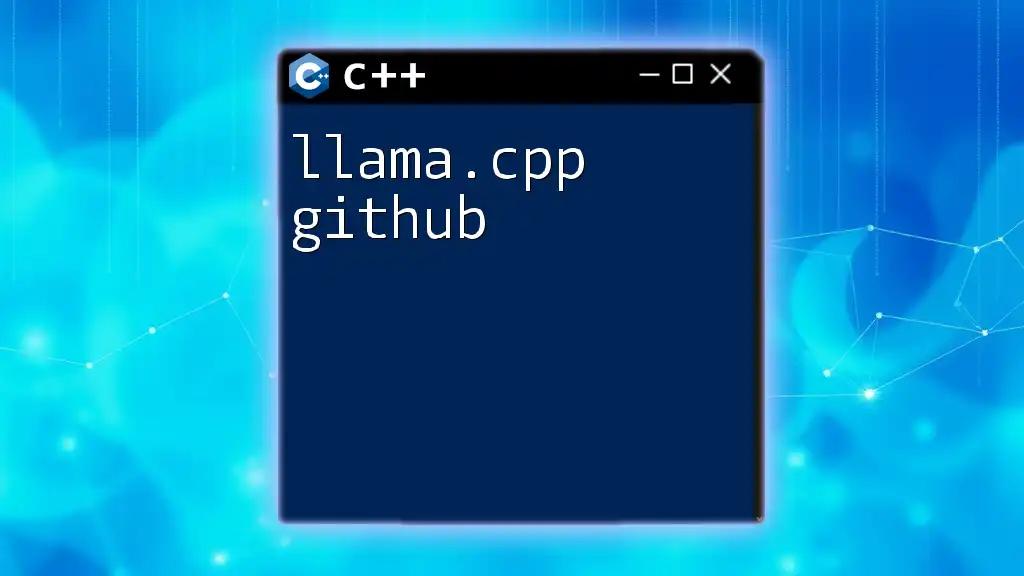
Debugging and Troubleshooting REST APIs
Common Issues
While working with REST APIs, you may encounter common issues such as:
- Connection timeouts
- Invalid endpoints
- Parsing errors in request bodies
Identifying and eliminating these issues early in your development process is paramount.
Debugging Tools
Utilize debugging tools and logs effectively. Log all requests and responses to analyze the flow of data through your API:
std::cout << "Received Request: " << req.method() << " " << req.path() << std::endl;
By adding logging at strategic points in your application, you’ll have better visibility into its operation.
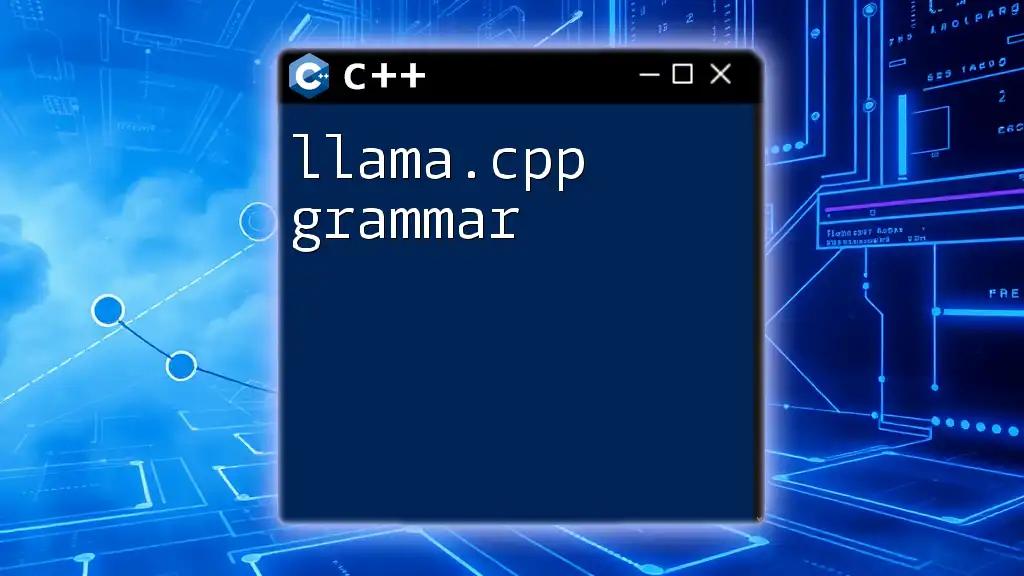
Conclusion
In this comprehensive guide, we've explored the Llama CPP framework's capabilities in creating and consuming REST APIs. From setting up your development environment to implementing endpoints and error handling, mastering these concepts will significantly enhance your API development skills.
With Llama CPP, the potential for crafting efficient and powerful web services in C++ is at your fingertips. Don’t hesitate to experiment with different features and advanced practices as you build your projects. For further learning, explore additional resources, documentation, and community forums dedicated to Llama CPP.