The `llama.cpp` interactive mode allows users to engage with the LLaMA model in a real-time, command-line environment for generating predictions or responses based on user input.
Here's a simple example of entering interactive mode and making a query:
// Start the interactive mode
./llama.cpp interactive
// Sample command to prompt the model
> Generate a short story about a time traveler.
Setting Up Your Environment for llama.cpp
To make full use of llama.cpp interactive mode, you first need to ensure that your development environment is properly set up.
System Requirements
Before diving in, it's essential to understand the system requirements. The software is primarily supported on various operating systems, including Windows, macOS, and Linux.
Make sure you have the following software installations:
- A compatible C++ compiler (GCC, Clang, or MSVC).
- Necessary libraries that might be required for higher-level functions.
Installing llama.cpp
Installing llama.cpp can be straightforward when following these steps:
- Download the latest release from the official GitHub repository.
- Extract the files to a directory of your choice.
- Open your terminal or command prompt and navigate to the directory where you extracted the files.
- Compile the source code by running:
make
After the compilation is complete, you can verify the installation by running a simple command:
./llama --version
This command should return the version of llama.cpp, confirming that you have successfully installed it.
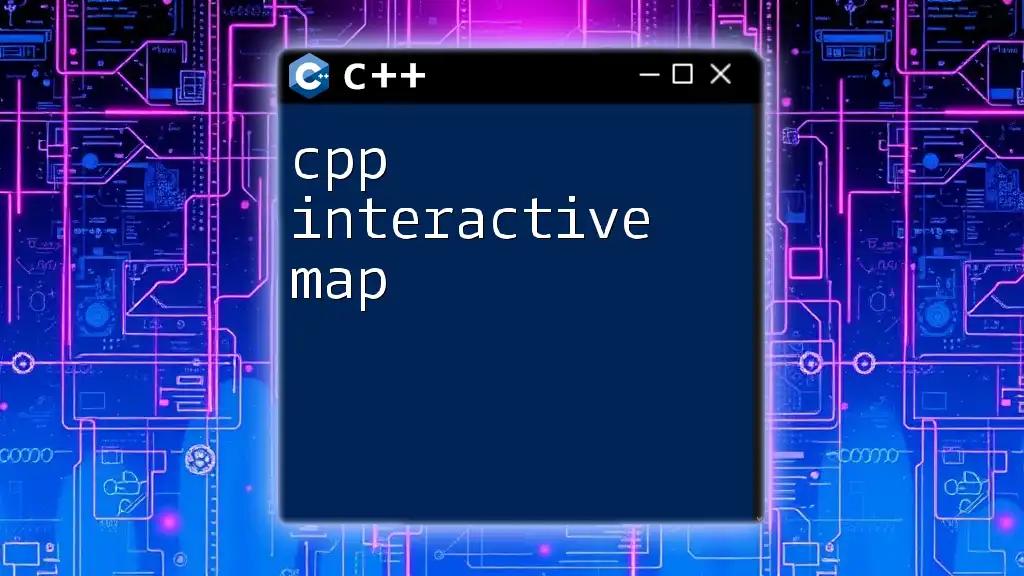
Getting Started with llama.cpp Interactive Mode
Launching Interactive Mode
Once the setup is complete, entering the interactive mode is the next step. This can be achieved by executing the following command in your terminal:
./llama
Upon launching, you’ll be greeted with an interactive prompt that indicates you are ready to start executing commands.
Basic Commands in Interactive Mode
In llama.cpp interactive mode, there are a few basic commands that every user should know:
- `help`: This command provides a list of all the available commands you can use.
- `exit`: Use this command to gracefully exit the interactive mode.
For example, typing `help` will yield:
Available commands:
- help
- exit
- <other commands>
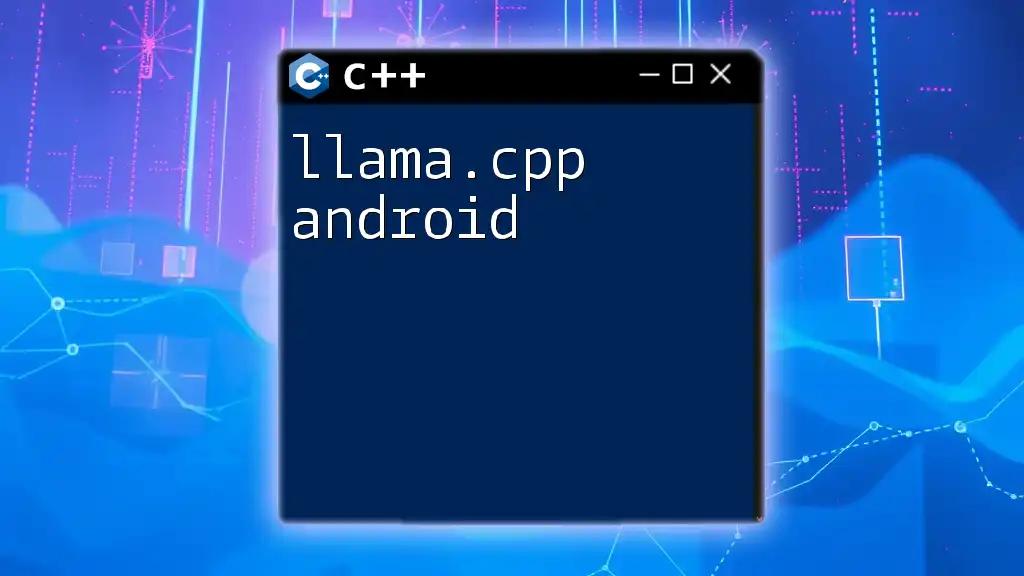
Essential Features of llama.cpp Interactive Mode
Executing Commands
Executing commands in llama.cpp interactive mode is intuitive. You simply type the command and hit enter.
For instance, to print "Hello, World!" you would enter:
print("Hello, World!");
This directly outputs:
Hello, World!
Variables and Data Types
Variables play a crucial role in C++ programming. In llama.cpp, you can define variables as follows:
int myVariable = 10;
float myFloat = 2.5;
string myString = "Llama";
These commands set up three different types of variables, and you can manipulate them as needed throughout your session.
Functions in Interactive Mode
Defining functions in the interactive mode is possible and allows you to streamline repetitive tasks. Here’s a simple example of how to define and use a function:
void greet() {
print("Hello from llama.cpp!");
}
Calling `greet();` will execute the function and output:
Hello from llama.cpp!
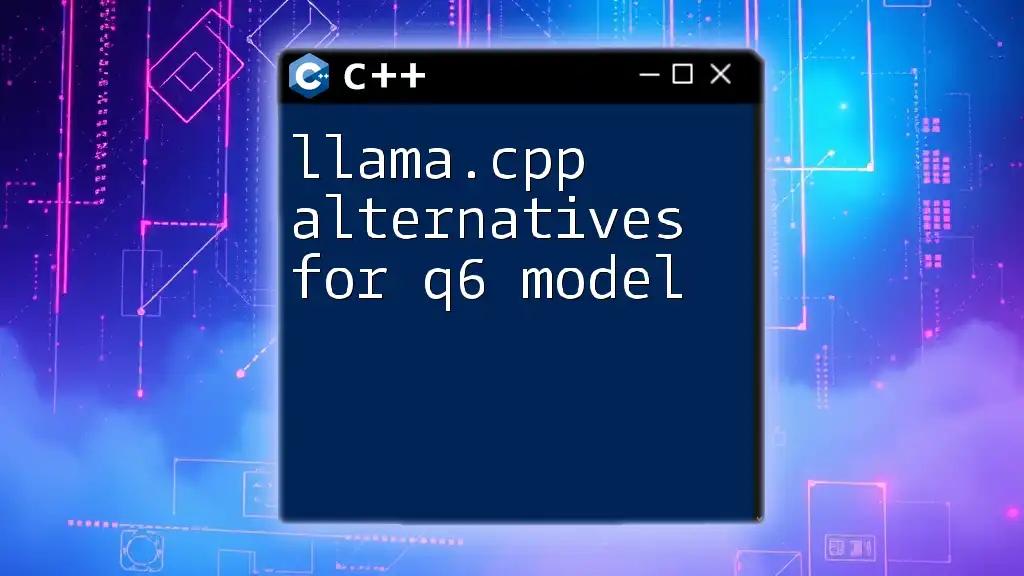
Advanced Interactive Features
Using Loops and Conditional Statements
For automation and logic control, loops and conditional statements are vital. In llama.cpp interactive mode, you can implement constructs like `for`, `while`, and `if` statements.
Here’s a simple demonstration using a `for` loop:
for(int i = 0; i < 5; i++) {
print("Current iteration: " + to_string(i));
}
This loop will output:
Current iteration: 0
Current iteration: 1
Current iteration: 2
Current iteration: 3
Current iteration: 4
Error Handling
While working in llama.cpp interactive mode, you may encounter several common errors. For instance, a syntax error might look like:
Error: 'unexpected token'
Understanding and resolving these errors requires careful observation of the code you input. Utilizing simple debugging techniques—like isolating problematic code—can save you time and effort.
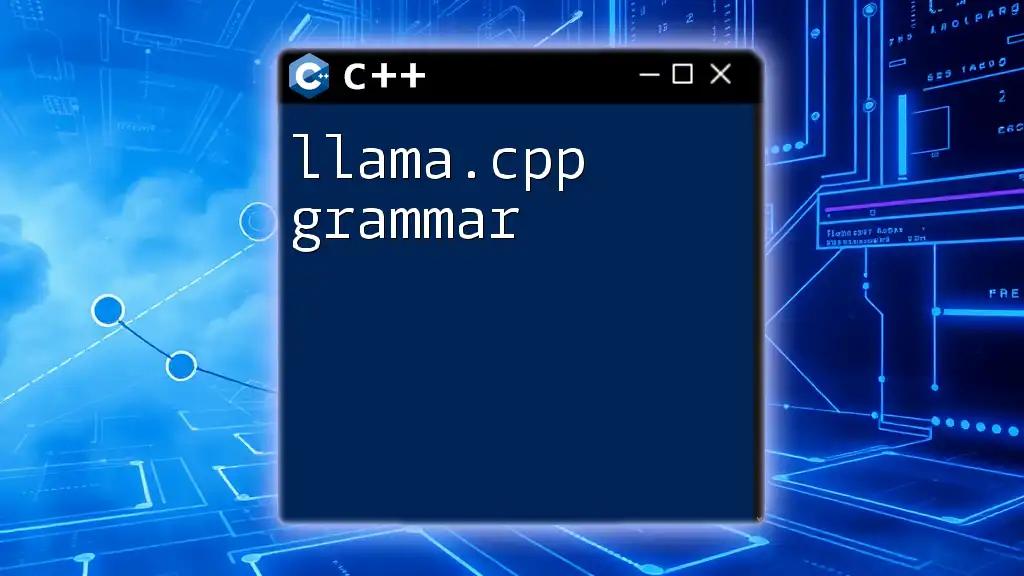
Tips and Tricks for Effective Use
Optimizing Your Experience in Interactive Mode
To fully optimize your experience, consider familiarizing yourself with shortcuts and command inputs. For example, using the up and down arrow keys allows you to scroll through your command history, which can significantly streamline workflow.
Common Pitfalls to Avoid
While interacting with the command mode, avoid frequent use of complex structures immediately. Start simple, and incrementally test your code. This approach will help you identify issues early and avoid frustration.
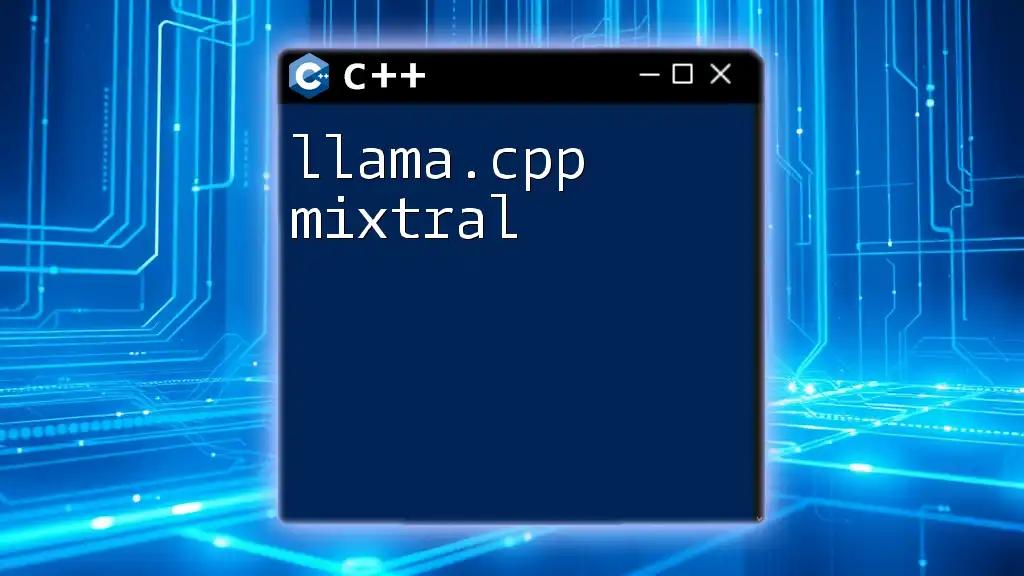
Real-World Applications of llama.cpp Interactive Mode
Project Scenarios
Utilizing llama.cpp interactive mode can enhance productivity across various projects. For instance, during rapid prototyping, you can quickly test snippets of code for algorithms or ideas without the overhead of compiling a full application.
Success Stories
Many users have found success through llama.cpp interactive mode, reporting enhanced learning experiences and expedited project developments. It has allowed beginners to instantly grasp concepts and seasoned developers to iterate faster on their ideas.
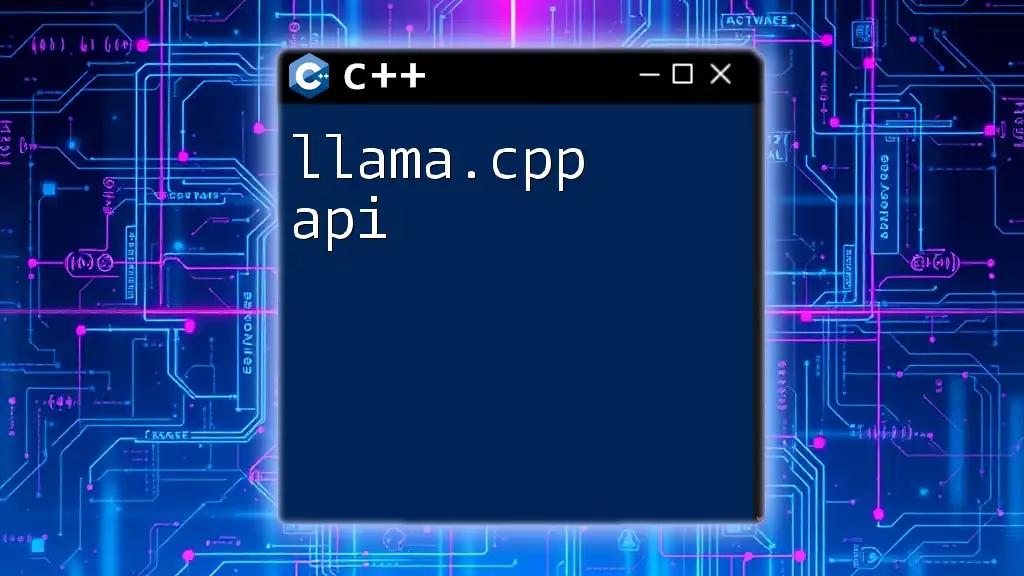
Conclusion
Engaging with llama.cpp interactive mode is an inviting gateway into the powerful potential of C++ programming. By leveraging the commands and features detailed above, you can refine your skills, experiment, and potentially create incredible applications. Don't hesitate to immerse yourself in this interactive environment and explore all the possibilities it offers.
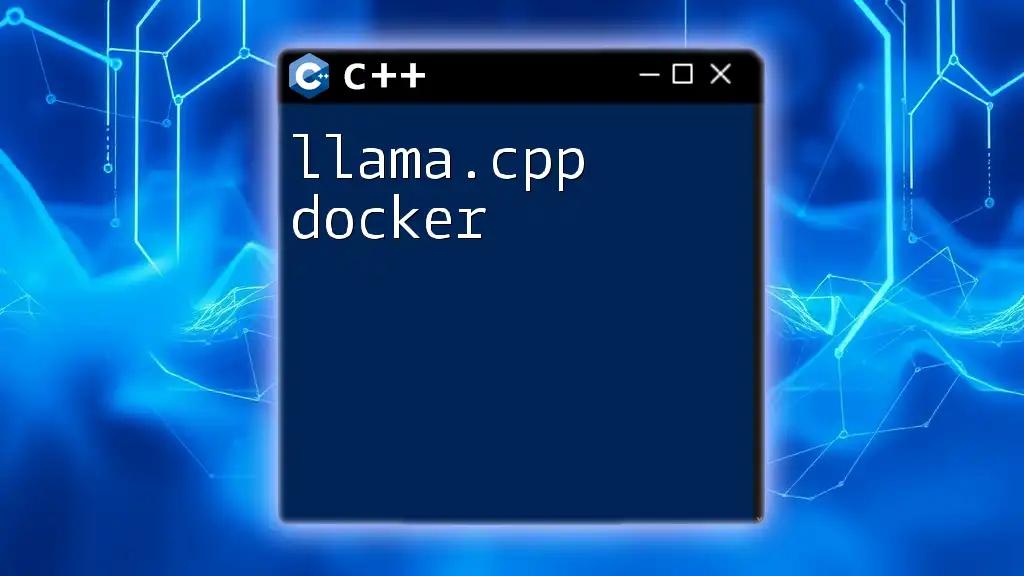
Additional Resources
To further enrich your learning journey, consider exploring additional resources, from books to online tutorials, and join community forums to connect with other llama.cpp enthusiasts. This engagement will not only enhance your learning curve but also provide a support network for troubleshooting and sharing ideas.