The "cpp interactive map" refers to a feature in C++ that allows for the use of a map container to store data in key-value pairs, providing an efficient way to associate and retrieve values based on keys.
Here's a code snippet demonstrating how to create and manipulate a simple `std::map` in C++:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
// Inserting data into the map
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
ageMap["Charlie"] = 35;
// Accessing data from the map
std::cout << "Alice's age: " << ageMap["Alice"] << std::endl;
return 0;
}
What are Interactive Maps?
Definition of Interactive Maps
Interactive maps are dynamic representations of geographical data that allow users to interact with the displayed information. Unlike traditional static maps, interactive maps enable users to zoom in and out, pan across different areas, and even click on specific features to retrieve additional data or trigger actions. They are often utilized in web applications, mobile apps, and game development, enhancing user experience through real-time engagement.
Importance of Interactive Maps in C++
In the world of C++, interactive maps find application across various domains, including:
- Game Development: Often used for environments in 2D and 3D games, allowing for player navigation and exploration.
- Geographic Information Systems (GIS): Functions as a fundamental tool for visualizing spatial data, aiding in analysis and decision-making processes.
- Data Analysis: Helps in displaying large datasets geographically, allowing users to identify trends and patterns easily.
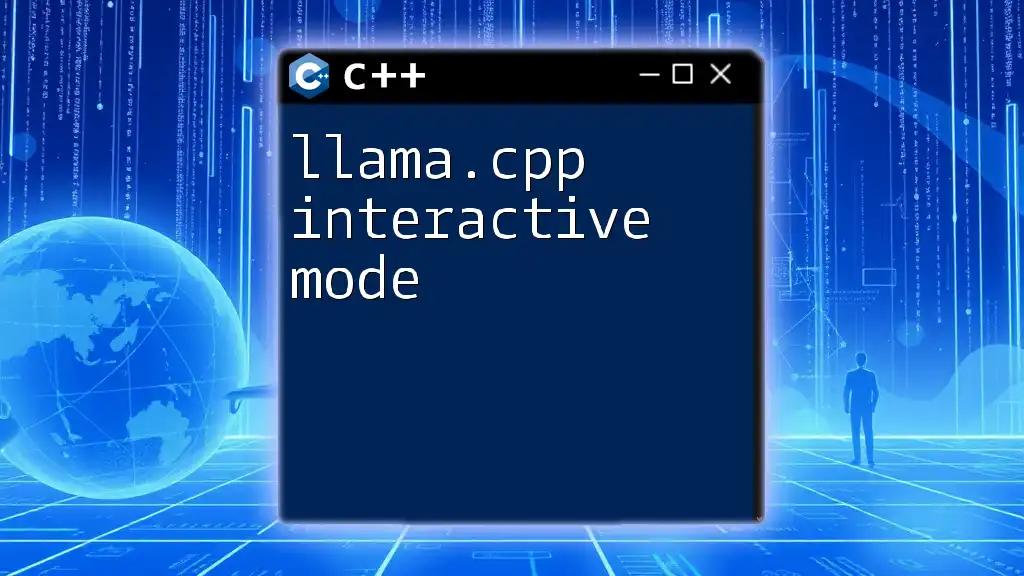
Setting Up the Environment
Required Software and Tools
When working with C++ for creating interactive maps, it is important to select the right compiler and IDE. Popular options include:
- Compilers:
- GCC (GNU Compiler Collection)
- Clang
- Integrated Development Environments (IDEs):
- Visual Studio: Rich features for debugging and code management.
- Code::Blocks: Lightweight and user-friendly for beginners.
Libraries and Frameworks
The power of C++ must be enhanced with libraries to manage graphics and interface. Here are two noteworthy choices:
- SFML (Simple and Fast Multimedia Library): SFML simplifies the process of creating graphics and handling user input, making it a favorite among game developers for creating interactive maps.
- Qt Framework: A robust framework for developing GUI applications, Qt provides tools that streamline interactive map creation, with a focus on user experience.
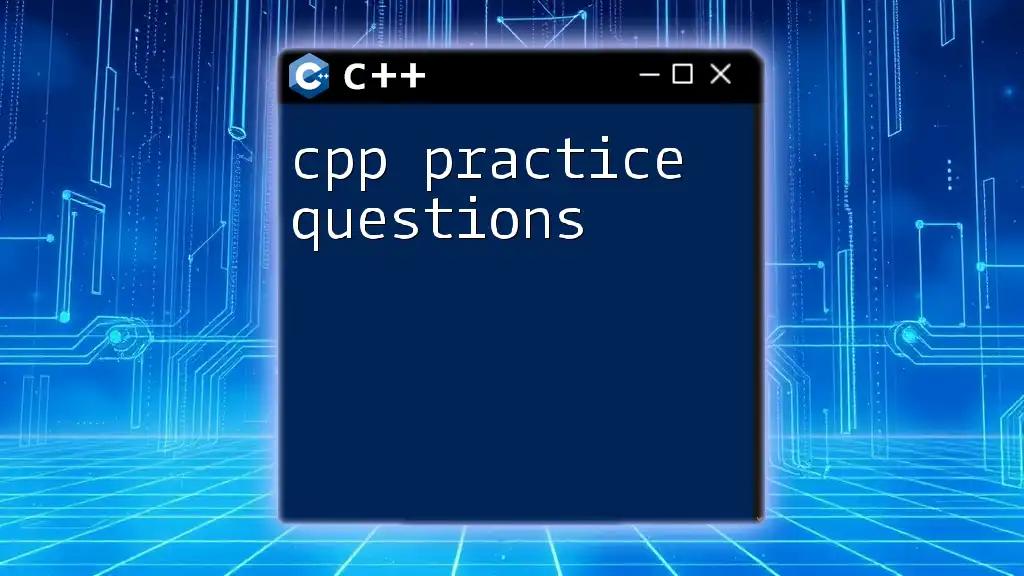
Creating an Interactive Map: Step-by-Step Guide
Initializing Your Project
To kickstart your cpp interactive map, begin with setting up a simple project structure. Here’s a basic code snippet illustrating how to create a window using SFML:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Interactive Map");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
// Insert map drawing logic here
window.display();
}
return 0;
}
Drawing the Map
Map Image and Texture
To display a map image, you need to load a texture that represents the map. Below is an example of how to do this in SFML:
sf::Texture mapTexture;
if (!mapTexture.loadFromFile("map.png")) {
// Handle error
}
sf::Sprite mapSprite(mapTexture);
Displaying the Map
After loading the texture, you can render the map image in the window. Simply add the drawing logic within the main loop:
window.draw(mapSprite);
Making the Map Interactive
Handling User Input
To make your cpp interactive map functional, handling user input is crucial. The following snippet details how to manage mouse clicks:
if (event.type == sf::Event::MouseButtonPressed) {
if (event.mouseButton.button == sf::Mouse::Left) {
// Get mouse position
sf::Vector2i mousePos = sf::Mouse::getPosition(window);
// Process interaction (e.g., display info, change states)
}
}
Adding Interactivity Features
Features like panning and zooming enhance the map's interactivity. For example, to implement a basic zooming mechanism:
float zoomFactor = 1.1f;
if (event.type == sf::Event::MouseWheelScrolled) {
if (event.mouseWheelScroll.delta > 0) {
// Zoom in code
} else {
// Zoom out code
}
}
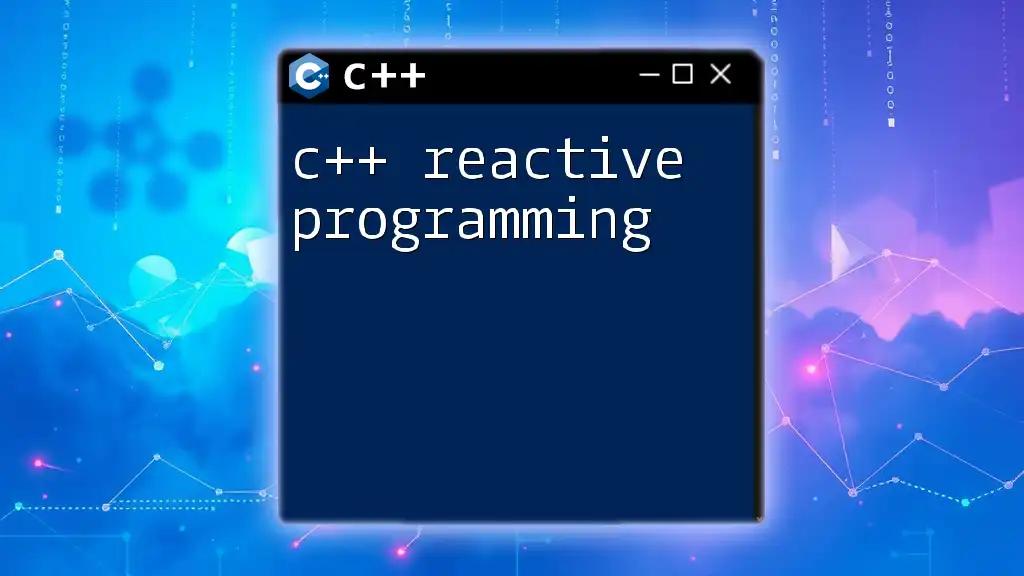
Examples of Interactive Maps in C++
Sample Projects
Game Development
In the context of game development, an interactive map might feature a mini-map that allows players to navigate through the game world. A practical example could involve setting waypoints that can be clicked to display information or trigger specific events.
GIS Applications
For a Geographic Information System, an interactive map may visualize geolocations such as restaurants or parks, where each feature on the map provides users with detailed statistics upon clicking.
Code Snippets
An example for adding markers on these locations might look like:
sf::CircleShape marker(5);
marker.setFillColor(sf::Color::Red);
marker.setPosition(x, y); // Coordinates of the location
window.draw(marker);
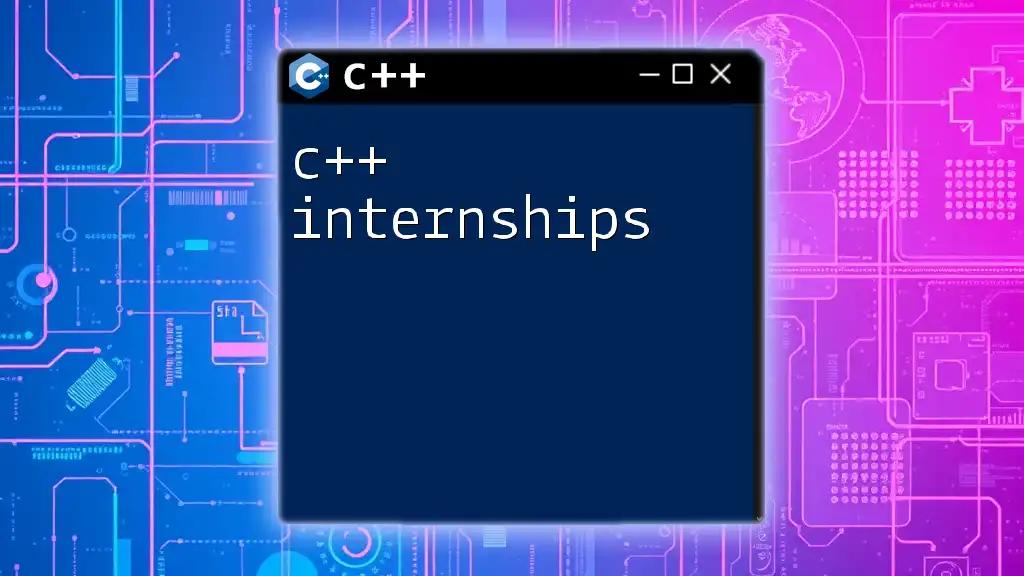
Best Practices for Smoother Interaction
Performance Optimization
To ensure a fluid experience, optimize performance by minimizing unnecessary calculations, using efficient data structures, and ensuring that graphic rendering occurs efficiently. This may include techniques such as:
- Culling: Only render objects within the viewable area.
- Layering: Divide the map into layers to manage rendering better.
User Experience
An engaging map design is essential. Consider:
- Providing clear visual cues and intuitive controls.
- Using a consistent color scheme that foster user engagement without overwhelming.
- Implementing smooth transitions during zooming and panning to enhance the user experience.
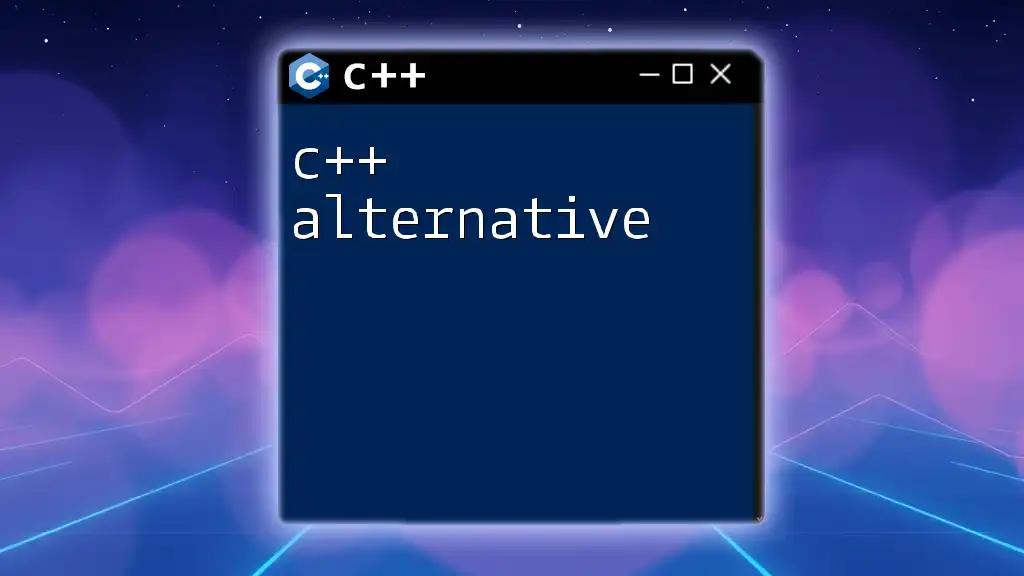
Troubleshooting Common Issues
Common Errors and Fixes
It's common to encounter issues like loading errors, rendering issues, or unresponsive event handling. Ensure you:
- Validate file paths when loading textures.
- Check for allocated resources and memory leaks after usage.
Helpful Debugging Tips
Effective debugging is key to resolving issues. Implementing debug messages throughout your code to track variable states can be incredibly helpful. The use of tools such as GDB or integrated debugger in your IDE can simplify the debugging process.
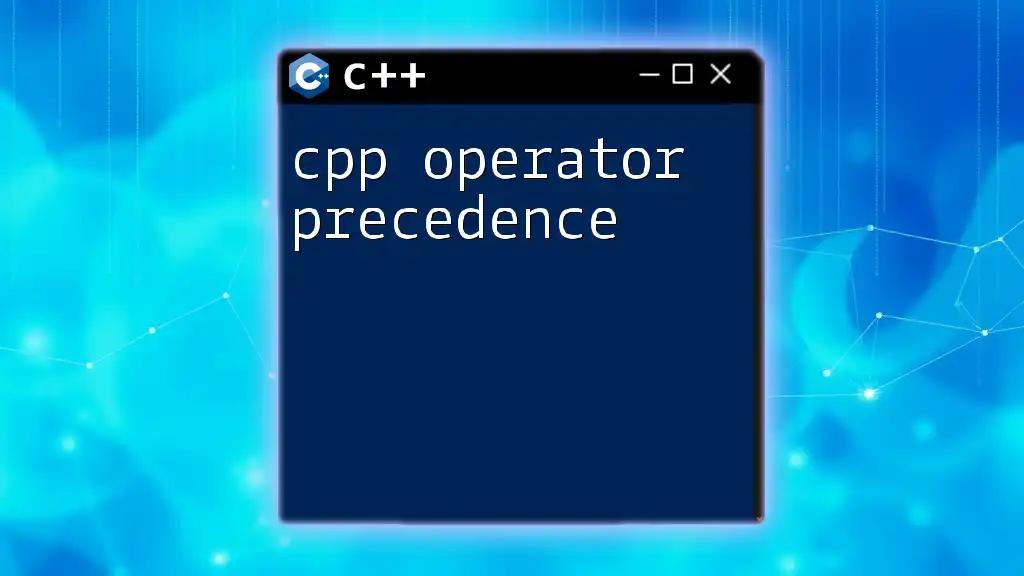
Conclusion
In summary, creating a cpp interactive map can be both an enriching learning experience and a valuable skill. By utilizing libraries such as SFML or Qt, you can effectively implement dynamic features that enhance user engagement. Experiment with the concepts discussed, and don't hesitate to explore further, as the world of interactive mapping is broad and filled with opportunities for innovation.
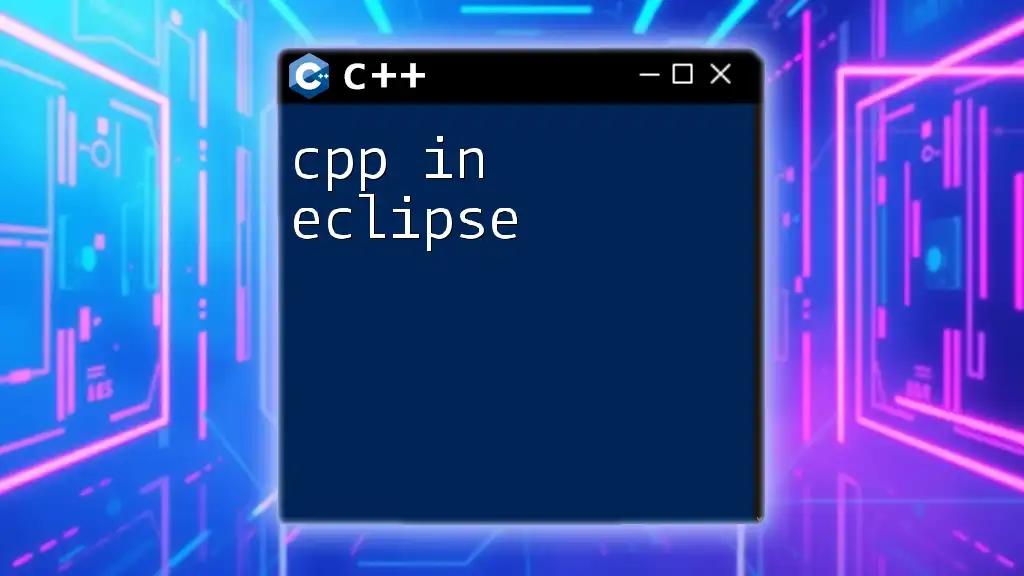
Additional Resources
To further develop your skills, consider exploring books focused on C++ graphics programming, online tutorials, and active communities such as forums or discussion groups. Engaging with others working on similar projects can provide insights and encouragement.
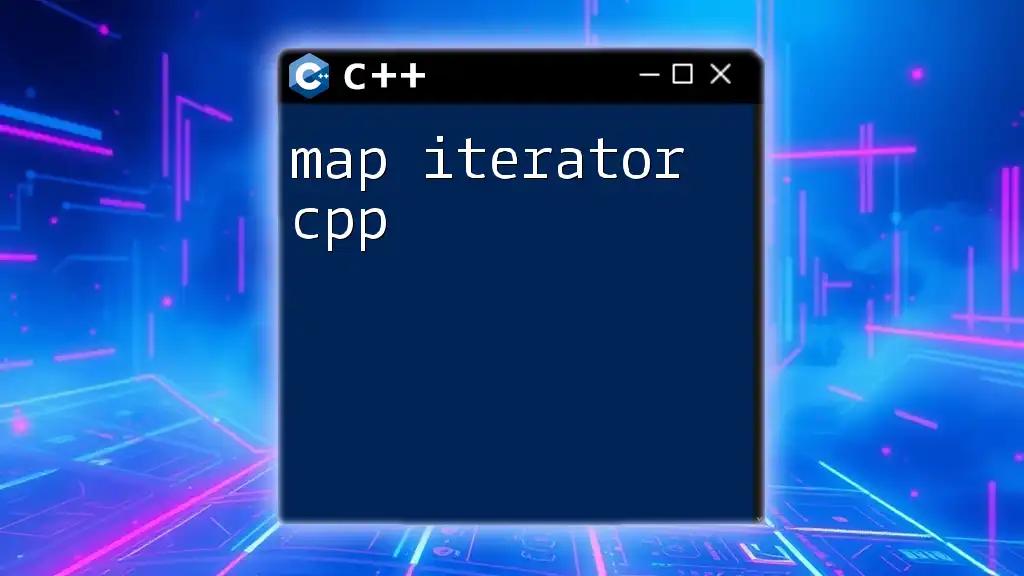
Call to Action
As you delve into crafting your own interactive maps, remember to share your experiences and learnings. Join our community to explore additional resources and connect with others passionate about learning C++ commands and enhancing their programming journey.