Pointers in C++ are variables that store the memory address of another variable, allowing for direct manipulation of data in memory.
Here's a simple code snippet demonstrating the use of pointers in C++:
#include <iostream>
int main() {
int var = 20; // declare an integer variable
int *ptr = &var; // declare a pointer and assign it the address of var
std::cout << "Value of var: " << var << std::endl; // Output: 20
std::cout << "Value at ptr: " << *ptr << std::endl; // Output: 20
std::cout << "Address of var: " << &var << std::endl; // Address of var
std::cout << "Address stored in ptr: " << ptr << std::endl; // Address of var
return 0;
}
What is a Pointer in C++?
A pointer is a variable that stores the memory address of another variable. In C++, pointers are essential for dynamic memory management, enabling developers to allocate memory at runtime and manage resources effectively. Understanding pointers cpp is vital for using C++ efficiently, especially in complex applications.
In the context of C++, a pointer can be thought of as a reference to a location in memory where data is stored. This allows you to manipulate memory directly, which can lead to optimized performance and effective resource management.
Syntax of Pointers in C++
Declaring a pointer involves specifying the data type it points to followed by an asterisk (*) and then the pointer variable name. Here’s the basic syntax:
int* ptr; // Declaration of a pointer to an integer
This declaration means that `ptr` can hold the address of an integer variable.
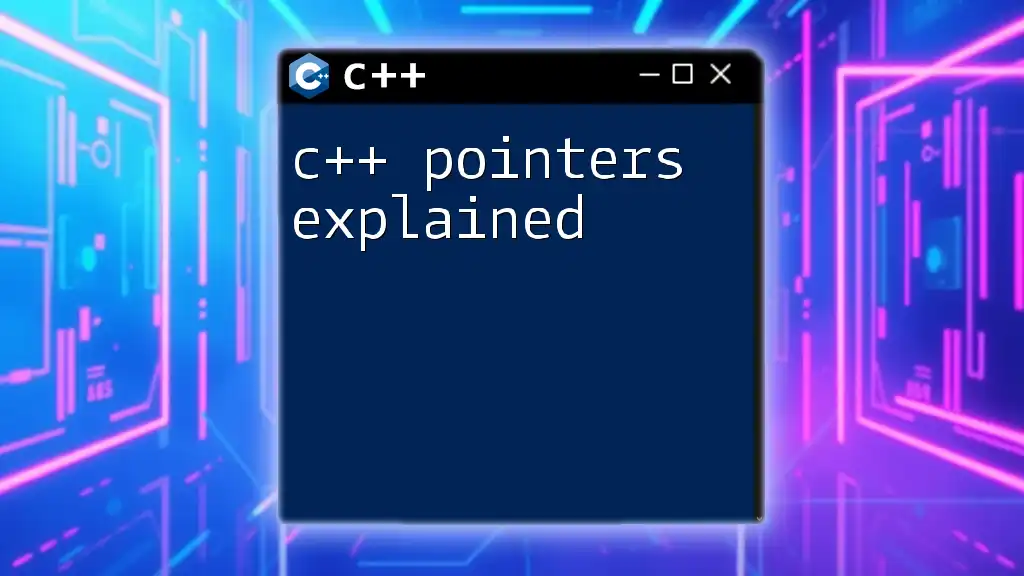
Memory Management and Pointers
Understanding Memory in C++
In C++, memory is divided into two main regions: stack and heap. Understanding this difference is crucial for effective memory management:
-
Stack Memory: This is used for static memory allocation. Variables declared inside a function are stored here, and the memory is automatically managed (allocated and deallocated) by the compiler when the function exits.
-
Heap Memory: This is used for dynamic memory allocation. Memory here must be managed explicitly using operators like `new` and `delete`. This allows flexibility at the cost of requiring careful memory management to avoid leaks and fragmentation.
Using `new` and `delete` Operators
Dynamic memory allocation in C++ is done using `new` and is handled using `delete` to prevent memory leaks. For instance:
int* num = new int; // allocate memory for an integer
*num = 5; // assign value 5 to the allocated memory
delete num; // free the allocated memory
Here's what happens in the above code:
- A new integer is allocated on the heap.
- This integer is accessed via the pointer `num`.
- After it's no longer needed, the memory is freed with `delete`.
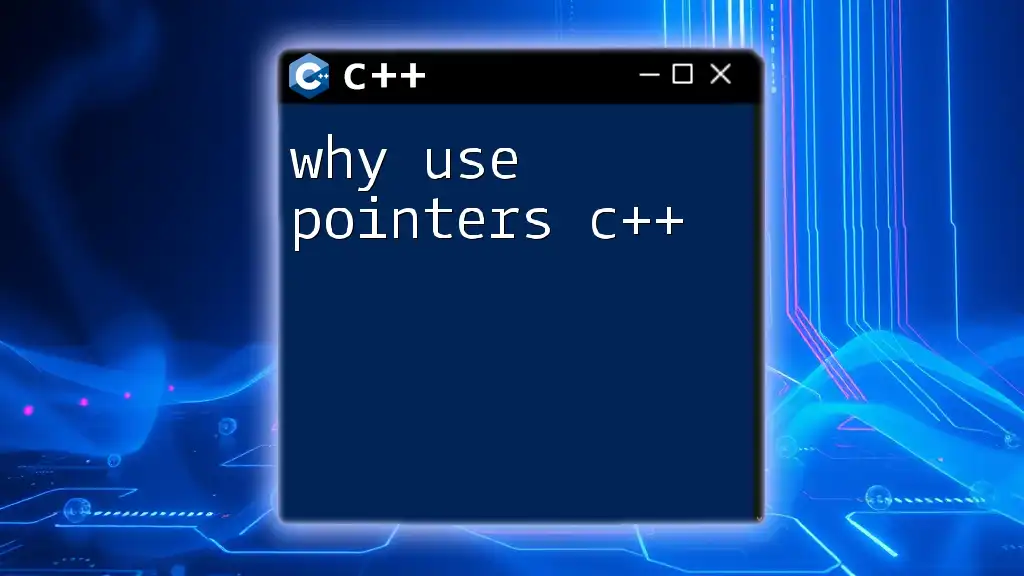
Working with Pointers
Pointer Initialization
Pointers should be initialized to either point to a valid memory address or set to `nullptr` to avoid dangling pointers. Here's an illustrated example:
int x = 10;
int* ptr = &x; // ptr points to the address of variable x
In this case, `ptr` holds the address of `x`, allowing you to modify or read `x` directly through the pointer.
Dereferencing Pointers
Dereferencing allows you to access or modify the value contained at the address pointed to by a pointer. The dereference operator (*) is used for this:
int value = *ptr; // accesses the value of x using pointer
Here, `*ptr` fetches the value of `x`, which is `10`. Similarly, you can modify it:
*ptr = 20; // changes the value of x to 20
Pointer Arithmetic
Pointer arithmetic is a powerful feature that allows you to navigate through memory efficiently. For example, you can increment a pointer to point to subsequent memory locations:
int arr[] = {10, 20, 30};
int* p = arr; // pointer to first element
p++; // now p points to arr[1], which is 20
This code demonstrates moving the pointer to access different elements of an array.
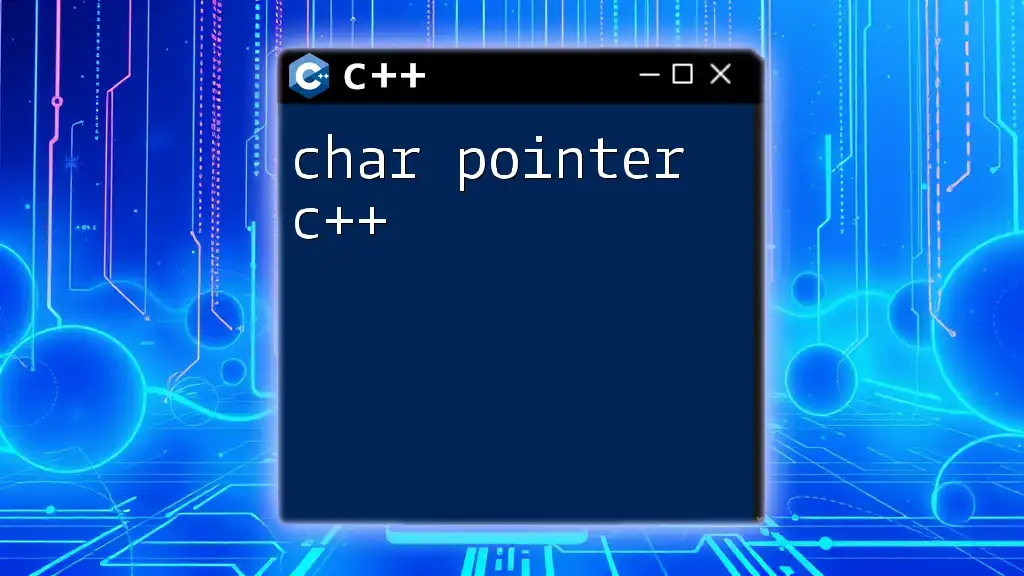
Types of Pointers
Null Pointer
A null pointer does not point to any valid memory address and is often used to indicate that the pointer is not in use. In modern C++, it is often defined as follows:
int* ptr = nullptr; // using nullptr in C++, safe initialization
Using `nullptr` helps prevent potential issues caused by uninitialized pointers, making your code more robust.
Wild Pointers
Wild pointers refer to pointers that have not been initialized and may point to random memory locations. Using wild pointers can lead to undefined behavior. Always ensure pointers are either initialized to `nullptr` or assigned a valid address before use.
Smart Pointers
Smart pointers are part of C++'s STL (Standard Template Library) and provide automatic memory management through RAII (Resource Acquisition Is Initialization). Key types include:
- `unique_ptr`: A unique ownership model where only one pointer can own the memory.
- `shared_ptr`: Allows multiple pointers to share ownership of the same memory resource.
Example using `unique_ptr`:
#include <memory>
std::unique_ptr<int> smartPtr(new int(10));
In this case, `smartPtr` automatically deallocates the memory it holds when it goes out of scope, preventing memory leaks.
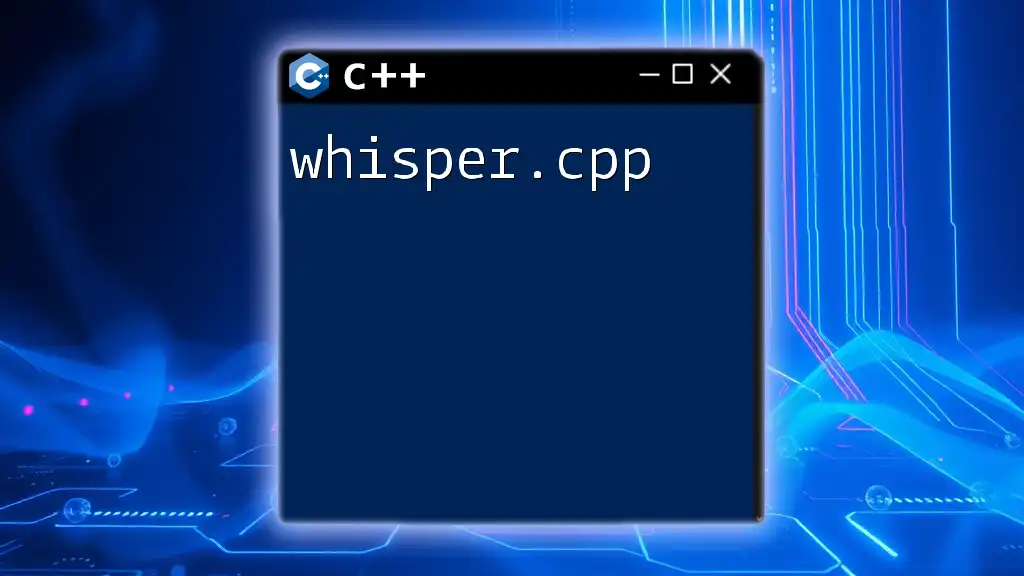
Common Use Cases for Pointers in C++
Dynamic Arrays
Pointers are crucial when working with dynamic arrays. This offers flexibility in creating arrays whose size can be determined at runtime:
int* arr = new int[5]; // allocate memory for 5 integers
In this snippet, an array of integers is allocated on the heap, allowing dynamic size allocation.
Passing Pointers to Functions
Pointers are also commonly used to pass variables by reference to functions, enabling modifications to be reflected outside the function scope:
void modify(int* ptr) {
*ptr = 20; // modifies the original variable's value
}
Calling this function with a pointer will change the original variable's value, showcasing how pointers allow functions to affect variables directly.
Multi-dimensional Arrays
Working with multi-dimensional arrays is made easier through pointers. For example:
int** matrix = new int*[3]; // create a pointer array for 3 rows
for (int i = 0; i < 3; i++) {
matrix[i] = new int[4]; // allocate columns for each row
}
This code segment allows for the creation of a 3x4 matrix dynamically, providing flexibility with memory allocation.
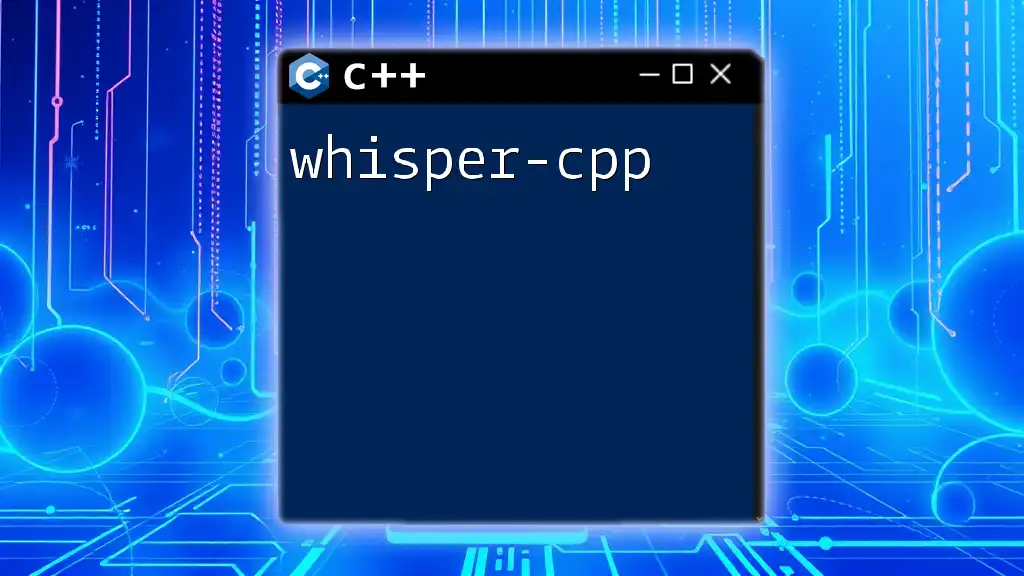
Common Pitfalls When Working with Pointers
Memory Leaks
Memory leaks occur when allocated memory is not deallocated. This can lead to performance issues and can consume all available memory over time. It’s crucial to always pair `new` with `delete` and `new[]` with `delete[]` to avoid memory leaks.
Dangling Pointers
Dangling pointers are pointers that refer to memory locations that have been freed or were never allocated. Dereferencing them can lead to undefined behavior. It's important always to nullify pointers after freeing memory:
delete ptr;
ptr = nullptr; // prevent dangling pointers
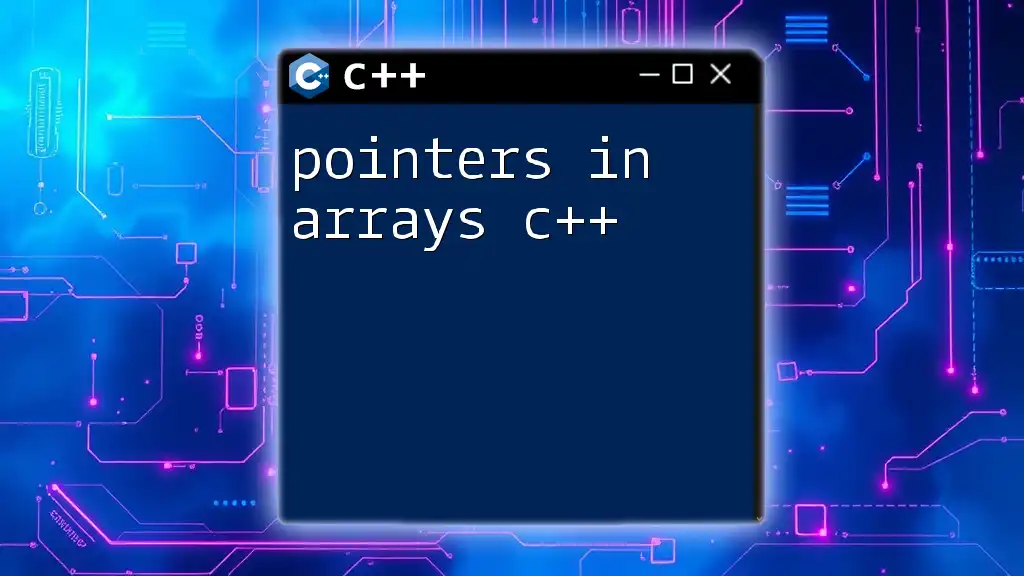
Conclusion
In summary, understanding pointers cpp is crucial for mastering C++ programming, particularly in the realms of dynamic memory management and performance optimization. By mastering concepts such as pointer initialization, arithmetic, dynamic memory allocation, and the use of smart pointers, you will be able to harness the full power of C++. Remember, with great power comes great responsibility—always manage your memory wisely!