The `printf_s` function in C++ is a safer version of `printf` that helps prevent buffer overflows by ensuring the output buffer's size is specified.
#include <cstdio>
int main() {
const char* name = "World";
printf_s("Hello, %s!\n", name);
return 0;
}
Understanding the Need for printf_s
Why Use Secure Functions?
The digital landscape is fraught with security vulnerabilities, particularly when it comes to input and output operations in programming. Many traditional functions, including `printf`, are susceptible to issues such as buffer overflows, which can lead to serious security breaches, including code injection attacks or unauthorized access to sensitive data. This is where secure functions, like `printf_s`, come into play. They are designed to incorporate checks that prevent such vulnerabilities, making your applications more robust and secure.
Differences Between printf and printf_s
`printf_s` is an extension of the standard `printf` function that emphasizes safety. Here are some essential differences:
- Buffer Overflow Prevention: `printf_s` checks the format string against the provided arguments to ensure that they match in type and number, significantly reducing the risk of buffer overflows.
- Error Handling: Unlike traditional `printf`, which may fail silently or produce undesirable output, `printf_s` provides clear error codes, enabling easier debugging.
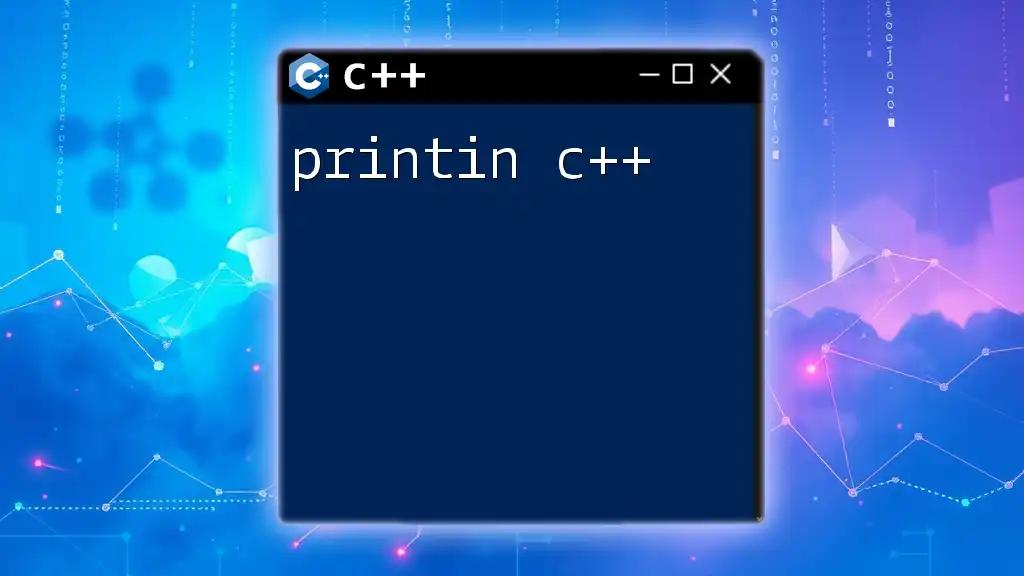
Syntax of printf_s
Basic Syntax Structure
The basic syntax of `printf_s` can be broken down as follows:
int printf_s(const char* format, ...);
- The first parameter, `format`, is a string that specifies how subsequent arguments are converted for output.
- The ellipsis (`...`) allows for a variable number of arguments to be passed, corresponding to the format specifiers present in the `format` string.
- The function returns the number of characters printed, or a negative value if an error occurs.
Format Specifiers
Format specifiers dictate how data will be formatted during output. Commonly used format specifiers include:
- %d - for integers
- %f - for floating-point numbers
- %s - for strings
These format specifiers serve as placeholders within the format string, which gets replaced by the corresponding argument.

Using printf_s in C++
Setting Up the Environment
To utilize `printf_s`, you need to ensure that your compiler supports secure functions. Typically, you'll need to include the necessary headers at the beginning of your code:
#include <cstdio>
Basic Example
Simple Output with printf_s
The simplest use case for `printf_s` is just to print a string.
#include <cstdio>
int main() {
printf_s("Hello, World!\n");
return 0;
}
In this example, running the program will output `Hello, World!` to the console. The function takes care of all the underlying processing, making it straightforward to display simple messages.
Advanced Usage
Formatting Strings
`printf_s` can also format strings dynamically based on variable values. For instance:
#include <cstdio>
int main() {
int age = 25;
printf_s("I am %d years old.\n", age);
return 0;
}
In this example, `%d` in the format string is replaced by the value of `age`, resulting in the console output: `I am 25 years old.`
Handling Multiple Variables
You can include multiple variables in your output with ease:
#include <cstdio>
int main() {
const char* name = "Alice";
int age = 30;
printf_s("Name: %s, Age: %d\n", name, age);
return 0;
}
In this snippet, the format string includes both a string and an integer. The output will read: `Name: Alice, Age: 30`. This flexibility makes `printf_s` an excellent choice for complex output scenarios.
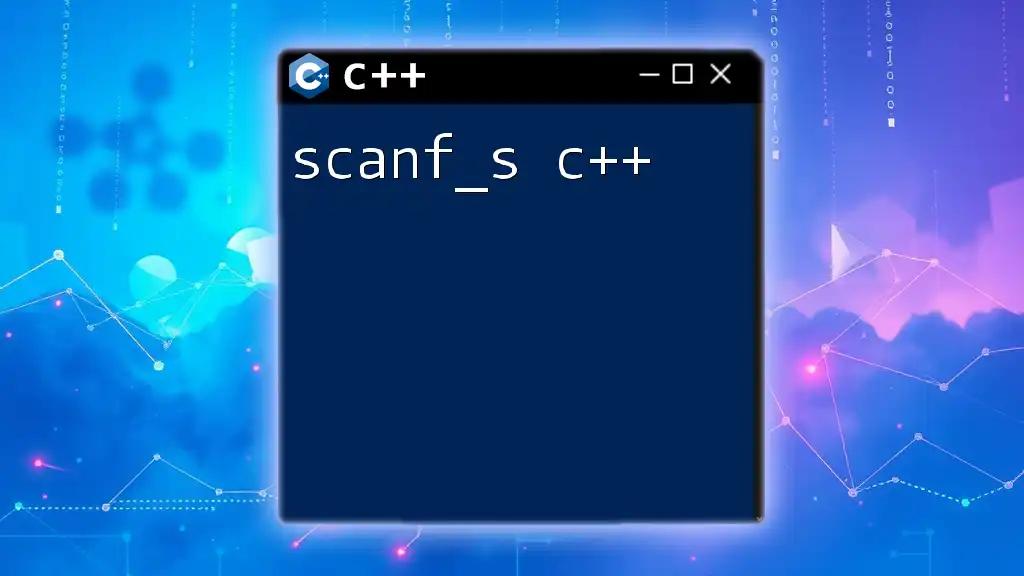
Error Handling in printf_s
Understanding Return Values
One of the key advantages of `printf_s` is its error handling capabilities. The return value of the function indicates the number of characters printed. If an error occurs, it returns a negative value, allowing you to react accordingly.
Example of Error Handling
Taking advantage of this feature can simplify debugging:
#include <cstdio>
int main() {
int result = printf_s("Hello\n");
if (result < 0) {
perror("Error: ");
}
return 0;
}
In this case, if `printf_s` encounters an error, the program will output an error message instead of failing silently. This clarity is invaluable when troubleshooting.
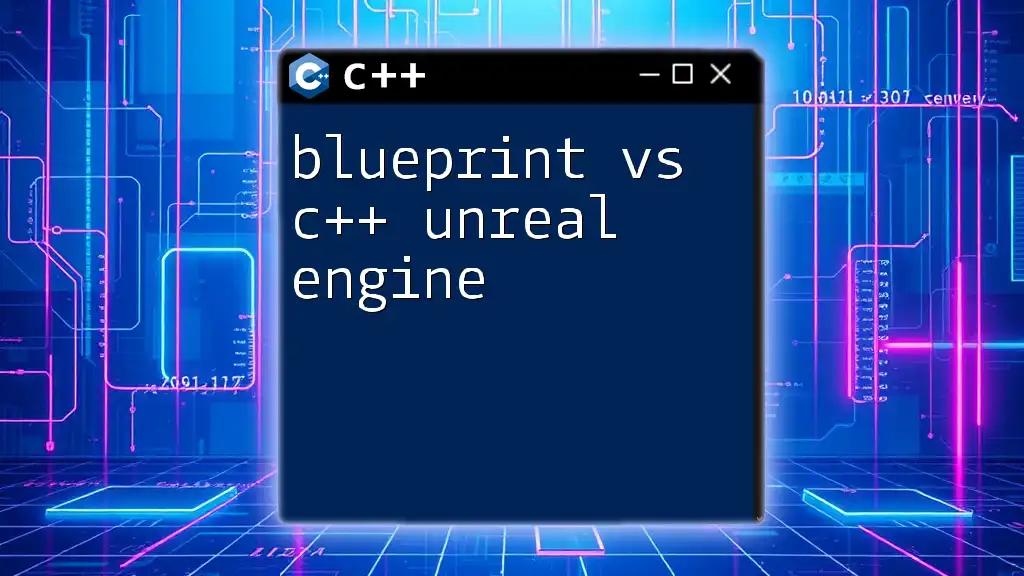
Best Practices for Using printf_s in C++
Always Validate Input
Ensuring your program can handle various inputs safely is crucial. Always validate user input before passing it to `printf_s`. This ensures that your program behaves predictably and securely.
Efficient Memory Management
While `printf_s` enhances safety, you still need to consider memory management. Avoid overly long strings or excessive variable formatting, which could still lead to performance issues. Understanding memory implications will enable you to utilize `printf_s` effectively.

Conclusion
In summary, `printf_s` provides a secure, efficient way to handle formatted output in C++. With built-in protections against common pitfalls, it is a smart choice for modern C++ programming. By incorporating `printf_s` into your coding practices, you’ll not only enhance the security of your applications but also make your code easier to read and maintain. Consider trying `printf_s` in your next C++ project to take advantage of its many benefits!
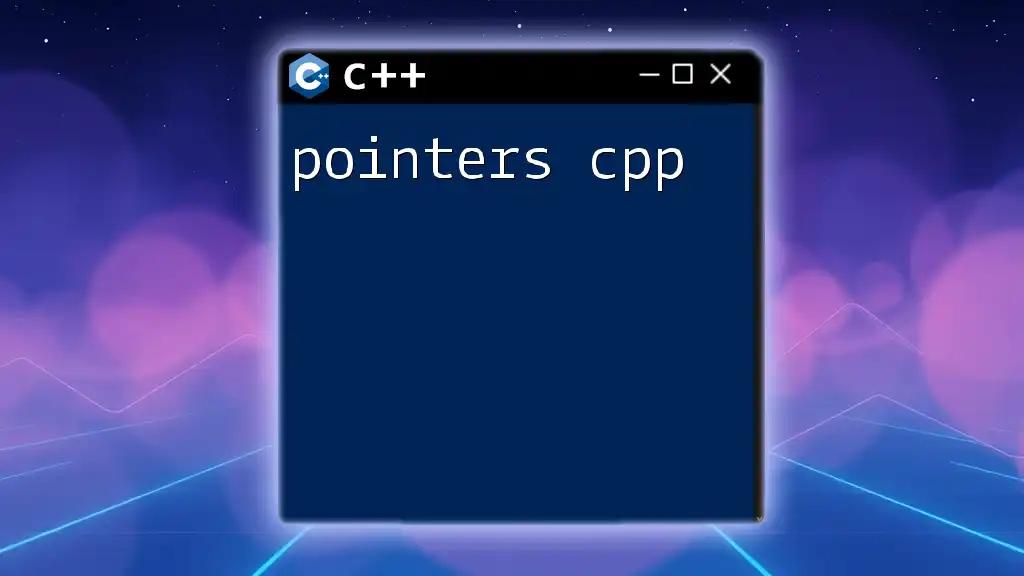
Additional Resources
For those looking to deepen their understanding of secure coding practices, consult additional resources such as the official C++ documentation concerning secure functions for further reading and best practices.