The `scanf_s` function in C++ is a safer version of `scanf` that includes additional security checks, specifically for buffer overflows, making it suitable for reading formatted input from standard input.
Here's a simple code snippet demonstrating its usage:
#include <stdio.h>
int main() {
char name[50];
printf("Enter your name: ");
scanf_s("%49s", name, (unsigned)_countof(name)); // Using _countof for buffer size
printf("Hello, %s!\n", name);
return 0;
}
Understanding `scanf_s`
What is `scanf_s`?
`scanf_s` is a secure version of the traditional `scanf` function, designed to improve the safety of user input handling in C++. It is particularly beneficial for preventing buffer overflow vulnerabilities, which can lead to program crashes or security exploits. This function is part of the C standard library and is primarily used in Microsoft compilers as a safer alternative to `scanf`.
Why Use `scanf_s`?
Using `scanf_s` provides enhanced security against common pitfalls associated with user input. Unlike `scanf`, which does not require the programmer to specify buffer sizes, `scanf_s` mandates it, effectively preventing the chance of writing data outside the allocated memory. This additional layer of security is especially critical in C++ programming, where manual memory management is common. The function is often considered best practice, especially in applications where user input can vary in size and could potentially expose the program to vulnerabilities.
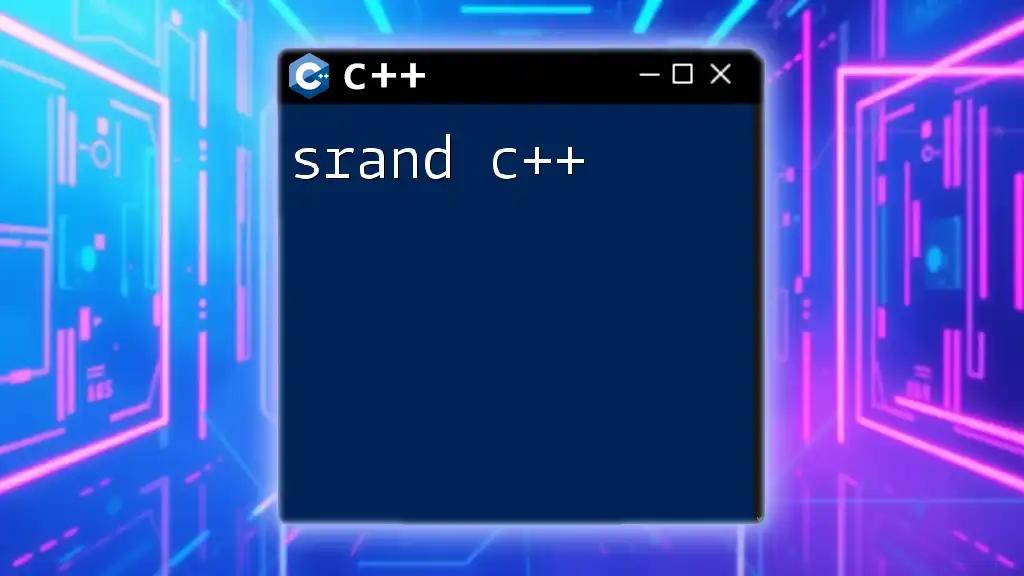
How to Use `scanf_s`
Basic Syntax
The basic syntax of `scanf_s` is somewhat similar to `scanf`, but with additional parameters for specifying buffer sizes. Here’s a breakdown of the syntax:
scanf_s(format-string, argument1, size1, argument2, size2, ...);
Each argument corresponds to a variable where the input will be stored, and each size specifies the size of the buffer being used for input.
Here’s a simple example:
#include <cstdio>
int main() {
char name[50]; // Buffer size of 50
printf("Enter your name: ");
scanf_s("%s", name, (unsigned)_countof(name)); // _countof returns the size of the array
printf("Hello, %s!\n", name);
return 0;
}
Format Specifiers
Format specifiers in `scanf_s` operate similarly to those in `scanf`. Here are some commonly used format specifiers:
- `%d`: for integers
- `%f`: for floating-point numbers
- `%s`: for strings
Here's an example that shows how to read multiple types of input:
int age;
float height;
printf("Enter age and height: ");
scanf_s("%d %f", &age, &height); // Notice the lack of size argument here, since int and float don’t require it
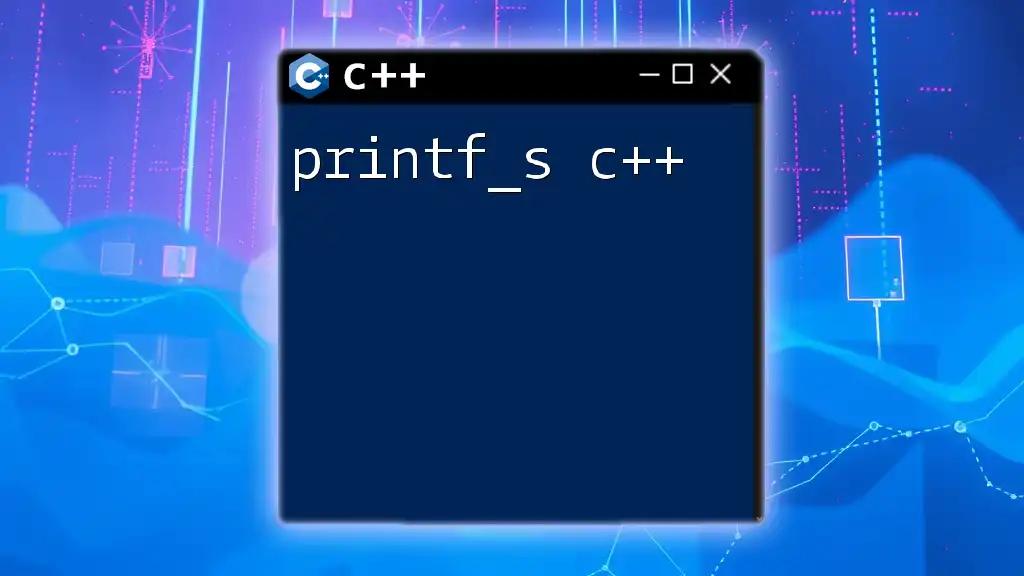
Working with Strings and Buffers
Importance of Buffer Size in `scanf_s`
A critical aspect of `scanf_s` is that it requires you to specify the size of the buffer you're using for strings. This prevents buffer overflows, a common source of security vulnerabilities. When using `scanf_s`, it's essential to allocate enough space for the string data being read.
Here’s an example demonstrating how to correctly utilize buffer size:
char buffer[20]; // Buffer can hold 19 characters + 1 for null terminator
printf("Enter a string (max 19 characters): ");
scanf_s("%19s", buffer, (unsigned)_countof(buffer)); // %19s ensures we only read 19 characters
Using `scanf_s` with Strings
When dealing with strings in C++, it's critical to manage buffer sizes to avoid potential overflow issues. Here's another functioning example:
char input[100]; // Allocate sufficient space for user input
printf("Input a string: ");
scanf_s("%99s", input, (unsigned)_countof(input)); // Prevent overflow by specifying max input
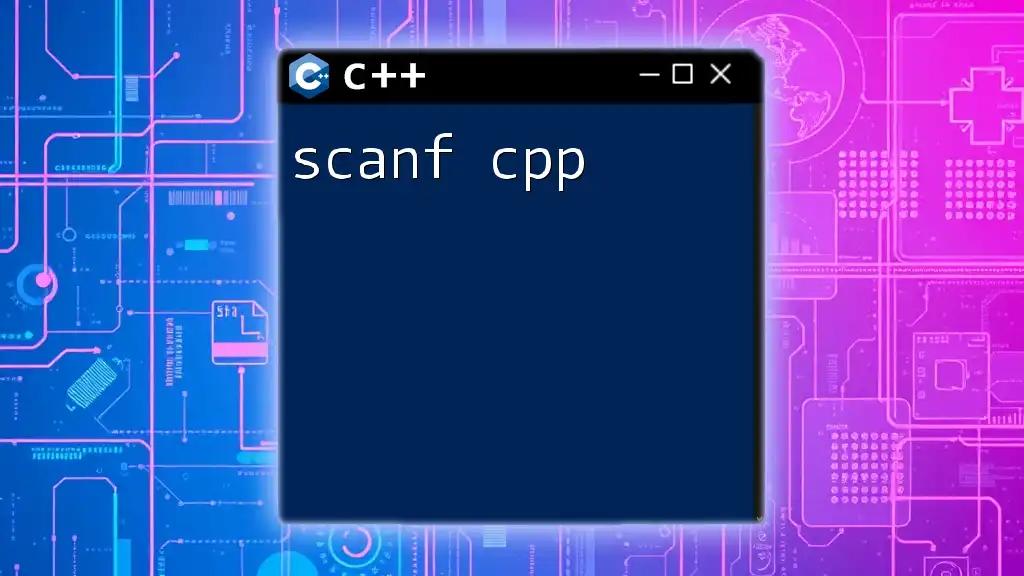
Best Practices for Using `scanf_s`
Safety and Error Checking
When using `scanf_s`, it's always a good practice to check the return value. This allows you to verify if the input was read correctly and take necessary action if it fails. It’s a safeguard that ensures your program behaves predictably.
Consider the following example:
int number;
if (scanf_s("%d", &number) != 1) {
printf("Invalid input!\n"); // Handle invalid input
}
Input Validation Techniques
You should also validate user inputs by leveraging loops. This encourages users to enter data until they provide valid input. Below is an example of using a loop for safety:
int number;
while (true) {
printf("Enter a valid number: ");
if (scanf_s("%d", &number) == 1) {
break; // Exit loop if valid input is provided
}
printf("Please try again.\n");
while (getchar() != '\n'); // Clear the input buffer
}
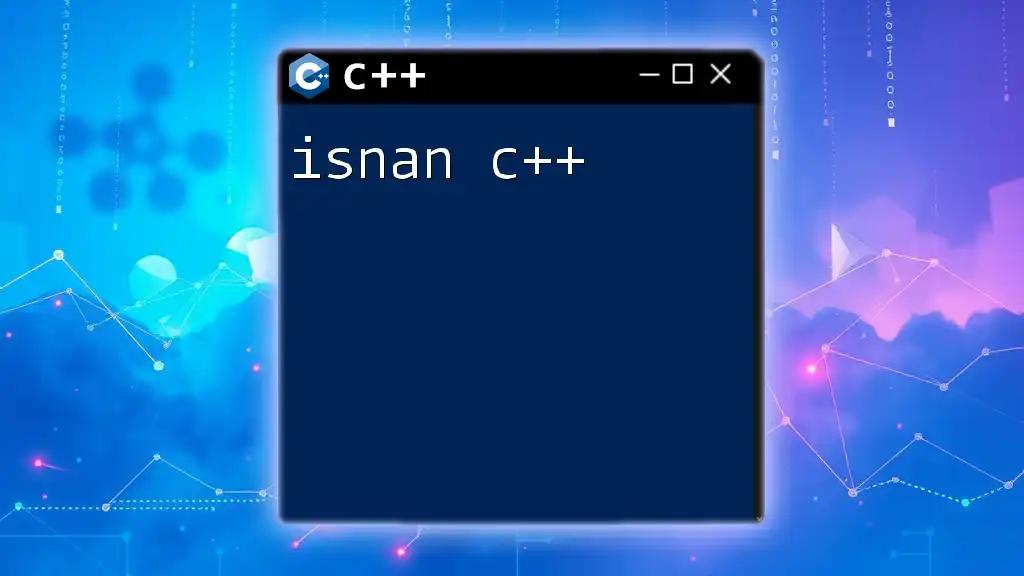
Common Mistakes
Misunderstandings with Input Size
A common mistake programmers make is neglecting the size specifications for buffers. Relying on `scanf` in a no-size context can lead to buffer overflows. Take the following example:
char unsafeBuffer[10];
scanf_s("%s", unsafeBuffer); // Unsafe and can lead to buffer overflow
The above code could easily cause unwanted memory corruption. Always remember to specify buffer sizes carefully when using `scanf_s`.
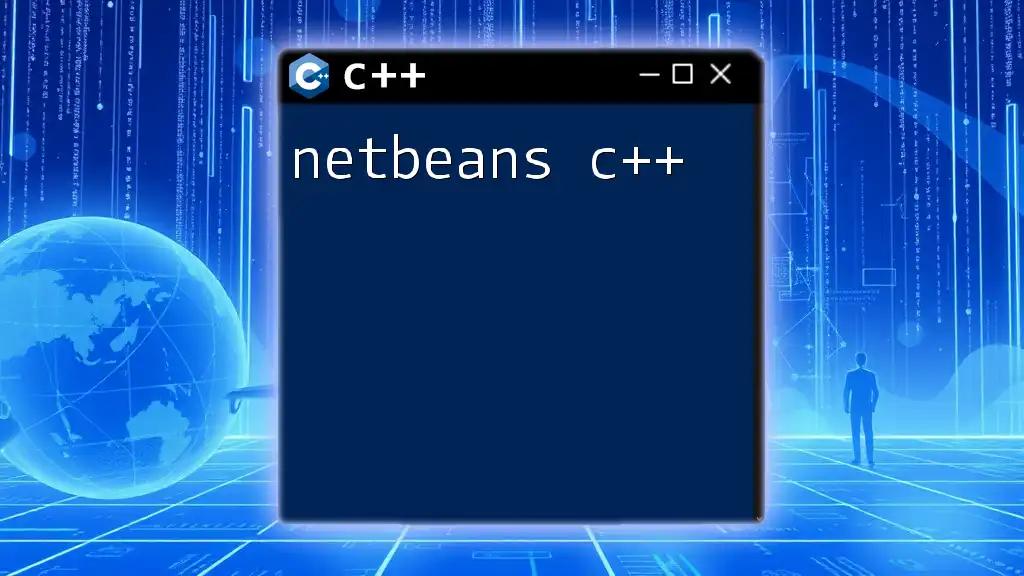
Key Takeaways
Using `scanf_s` enhances the safety of user input operations in C++. Not only does it help prevent buffer overflow, but it also encourages cleaner and more reliable code when accepting user data. It's vital to follow best practices, ensure proper buffer allocations, and always validate input to shield your applications from potential vulnerabilities.

Conclusion
In summary, `scanf_s` is an invaluable tool in handling user input in C++. Its design to require buffer sizes dramatically reduces the risk of common programming issues, making it the preferred method in secure C++ programming. By practicing its usage, you can ensure that your programs handle input in a safe and efficient manner.