In C++, the stack is a data structure that follows the Last In First Out (LIFO) principle, allowing for the efficient management of data through push and pop operations.
Here's a simple code snippet demonstrating how to use a stack in C++:
#include <iostream>
#include <stack>
int main() {
std::stack<int> myStack;
myStack.push(10); // Add 10 to the stack
myStack.push(20); // Add 20 to the stack
myStack.pop(); // Remove the top element (20)
std::cout << "Top element: " << myStack.top() << std::endl; // Outputs 10
return 0;
}
What is Stack?
A stack in computer science is a data structure that follows the Last In, First Out (LIFO) principle. This means that the last element added to the stack is the first one to be removed. Stacks are essential in programming for managing data in a systematic way, enabling functionalities like undo mechanisms, function call management, and expression evaluation.
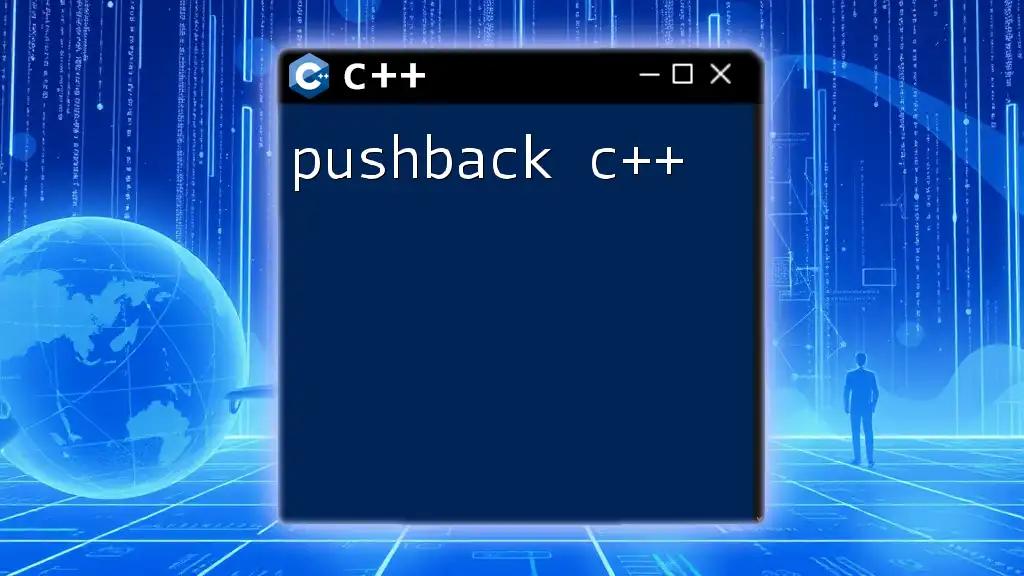
Why Use Stack in C++?
Stacks are incredibly useful in various programming scenarios. They provide a clear structure for handling behavior that requires strict ordering. Key advantages of using stacks in C++ include:
- Efficient memory management: Stacks operate quickly due to the built-in operations that can efficiently handle memory allocation and deallocation.
- Organized data flow: Maintaining a sequence of operations or function calls can help avoid confusion and errors, making debugging easier.
- Recursive function handling: Stacks play a critical role in managing function calls, especially recursive functions, by storing return addresses and local variables.
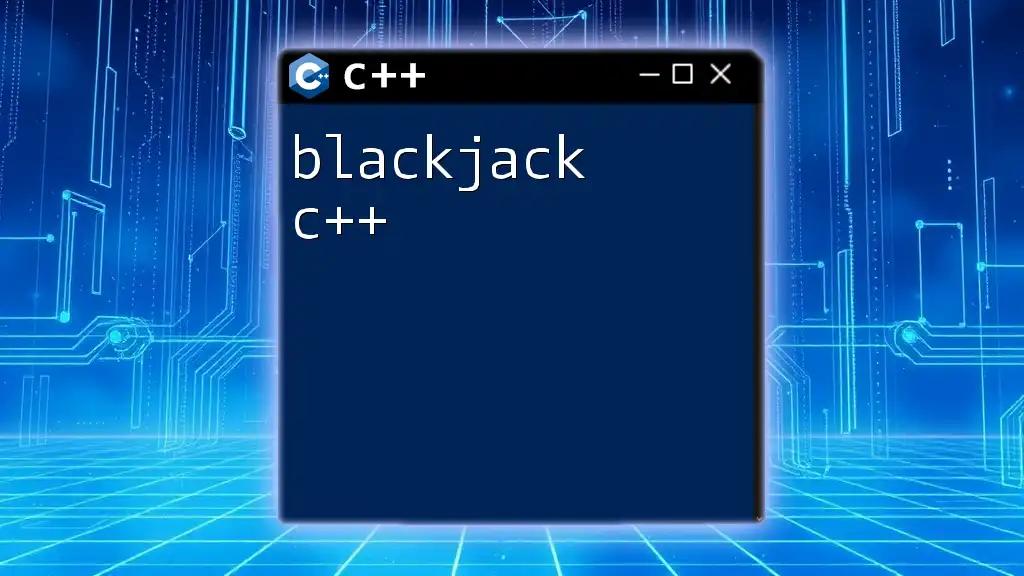
Understanding Stack Fundamentals
What is a Stack Data Structure?
A stack is a collection of elements that supports basic operations such as push, pop, and peek.
Stack Operations
- Push: This operation adds an element to the top of the stack.
- Pop: This operation removes the top element from the stack.
- Peek/Top: This operation retrieves the top element without modifying the stack.
- IsEmpty: This operation checks whether the stack contains any elements.
Visual Representation of Stack
While a visual representation is not included here, imagine a vertical stack of plates with the newest plate at the top. When you add a plate, you always do it at the top. When you remove a plate, you only take the one sitting on top, illustrating the LIFO principle perfectly.
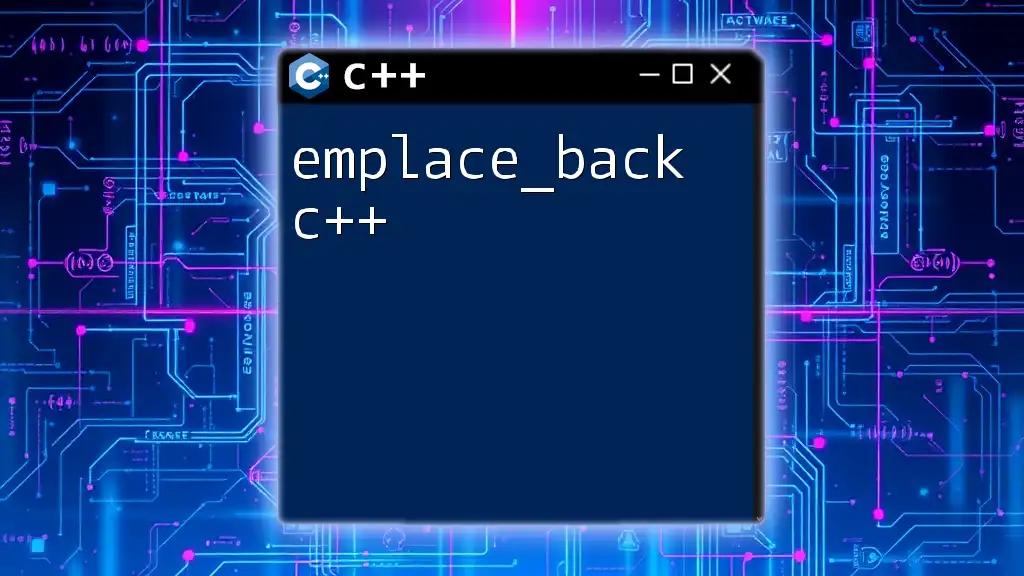
Implementation of Stack in C++
Using Arrays to Implement Stack
A common way to create a stack is by using arrays. Below is a straightforward implementation of a stack utilizing an array.
Code Example: Stack Implementation Using Arrays
#include <iostream>
#define MAX 100
class Stack {
private:
int arr[MAX];
int top;
public:
Stack() { top = -1; }
void push(int x);
void pop();
int peek();
bool isEmpty();
};
void Stack::push(int x) {
if (top >= (MAX - 1)) {
std::cout << "Stack Overflow" << std::endl;
} else {
arr[++top] = x;
}
}
void Stack::pop() {
if (top < 0) {
std::cout << "Stack Underflow" << std::endl;
} else {
top--;
}
}
int Stack::peek() {
if (top < 0) {
std::cout << "Stack is Empty" << std::endl;
return -1; // Assuming -1 signifies empty
} else {
return arr[top];
}
}
bool Stack::isEmpty() {
return top < 0;
}
In this code, the Stack class maintains an array of integers and a variable top to track the top of the stack. The push method handles overflow, preventing the stack from exceeding its maximum size. The pop method manages underflow scenarios, ensuring an element can only be removed if the stack is not empty.
Using Linked Lists to Implement Stack
An alternative to arrays is using linked lists, which can dynamically allocate memory and thus overcome the limitations of a fixed array size.
Code Example: Stack Implementation Using Linked Lists
#include <iostream>
class Node {
public:
int data;
Node* next;
};
class Stack {
private:
Node* top;
public:
Stack() { top = nullptr; }
void push(int x);
void pop();
int peek();
bool isEmpty();
};
void Stack::push(int x) {
Node* newNode = new Node();
newNode->data = x;
newNode->next = top;
top = newNode;
}
void Stack::pop() {
if (top == nullptr) {
std::cout << "Stack Underflow" << std::endl;
return;
}
Node* temp = top;
top = top->next;
delete temp;
}
int Stack::peek() {
if (top == nullptr) {
std::cout << "Stack is Empty" << std::endl;
return -1; // Assuming -1 signifies empty
} else {
return top->data;
}
}
bool Stack::isEmpty() {
return top == nullptr;
}
In this implementation, each stack element is represented as a Node. New elements are added to the top node, which points to the previous node. This structure allows for greater flexibility, as the stack can grow or shrink according to the needs of the program.
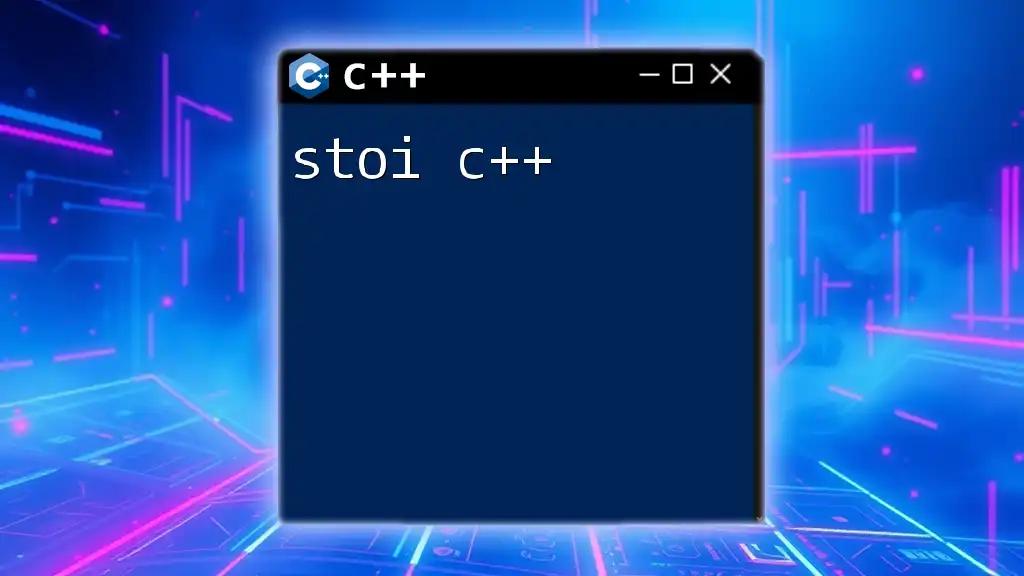
Real-World Applications of Stack
Function Call Management
Stacks are crucial for managing function calls in C++. Every time a function is called, its execution context—including local variables and return addresses—is pushed onto the call stack. When the function execution completes, the top of the stack is popped, restoring the previous execution context.
Example with Recursion
void recursiveFunction(int n) {
if (n > 0) {
std::cout << n << " ";
recursiveFunction(n - 1);
}
}
// Calling recursiveFunction(5) will push multiple calls onto the stack.
Expression Evaluation and Syntax Parsing
Stacks can also be utilized to evaluate expressions. For example, converting infix expressions to postfix expressions (Reverse Polish Notation) and evaluating them can be efficiently managed using a stack.
Example of Postfix Evaluation
#include <iostream>
#include <stack>
#include <sstream>
int evaluatePostfix(std::string expression) {
std::stack<int> stack;
std::istringstream tokens(expression);
std::string token;
while (tokens >> token) {
if (isdigit(token[0])) {
stack.push(std::stoi(token));
} else {
int right = stack.top(); stack.pop();
int left = stack.top(); stack.pop();
switch (token[0]) {
case '+':
stack.push(left + right);
break;
case '-':
stack.push(left - right);
break;
case '*':
stack.push(left * right);
break;
case '/':
stack.push(left / right);
break;
}
}
}
return stack.top();
}
// For example, evaluatePostfix("5 2 + 3 *"); evaluates to 21
Undo Mechanism in Applications
Many applications, such as text editors, provide an undo feature that relies on stacks. Each action the user takes can be pushed onto a stack, and when the user requests an undo operation, the latest action can be popped from the stack, reverting the state of the application.
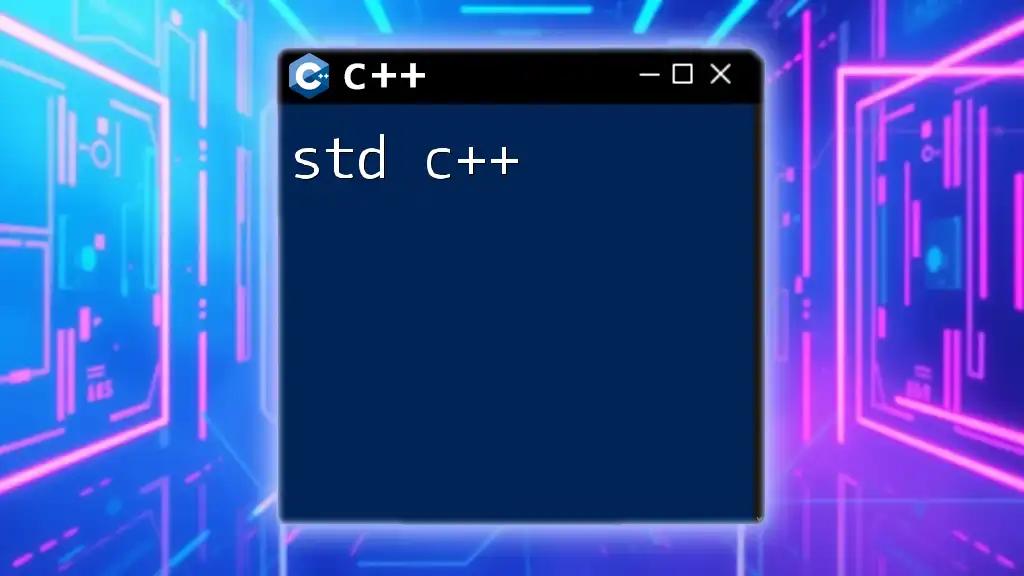
Common Challenges and Solutions
Stack Overflow
Stack overflow occurs when there is an attempt to push an element onto a stack that has reached its maximum capacity. To prevent this, always check if an operation will exceed the maximum size before execution.
Stack Underflow
Stack underflow happens when an attempt is made to pop an element from an empty stack. Implementing checks in the pop method can help manage this issue gracefully, allowing the error to be reported before attempting the operation.
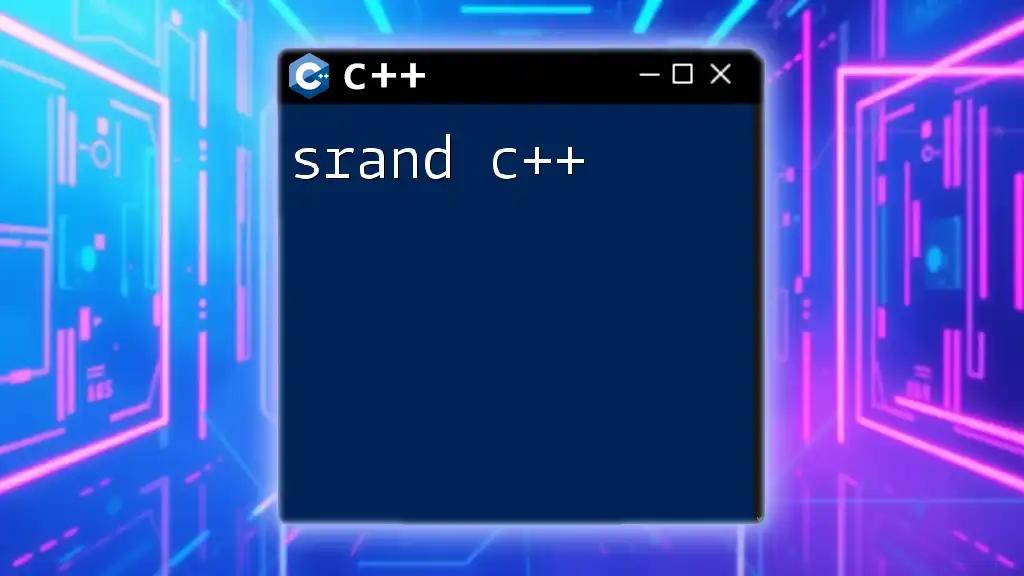
Summary and Best Practices
In this guide to stack c++, we explored the definition and importance of the stack data structure, along with implementation techniques—both with arrays and linked lists. Key takeaways include the stack's relevance in function call management, expression evaluation, and undo functionalities.
When using stacks, always ensure to handle overflow and underflow errors properly. Keep experimenting with various stack implementations and discover innovative ways to leverage stacks in your C++ projects.
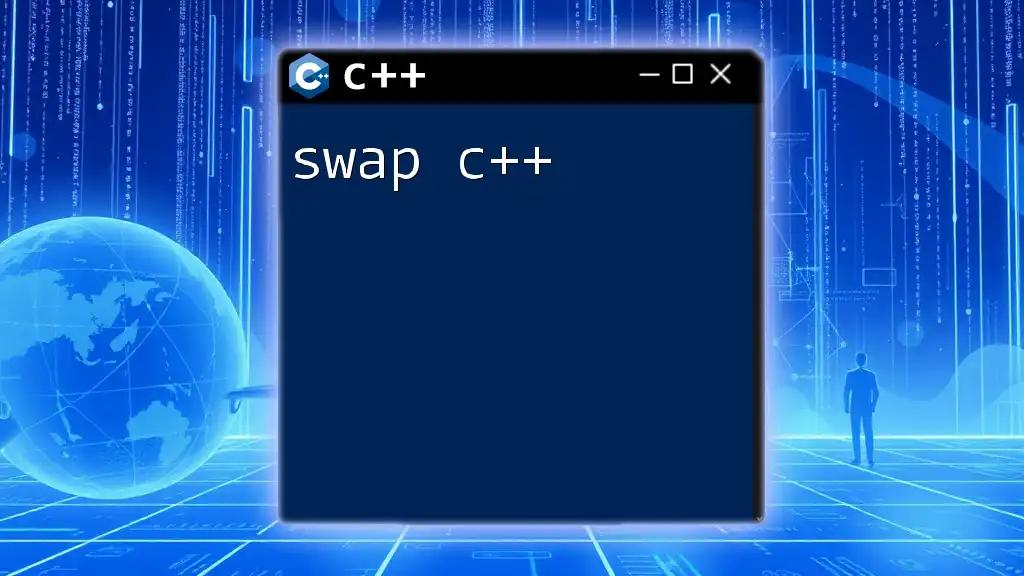
Conclusion
By understanding and utilizing stacks in your C++ programming, you can enhance your ability to manage data efficiently and create robust applications. Share your experiences and thoughts on stack implementations, and don't hesitate to explore further into advanced applications of this foundational data structure!