The `break` statement in C++ is used to terminate the nearest enclosing loop or switch statement, allowing control to transfer to the statement immediately following that loop or switch.
#include <iostream>
int main() {
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // exit the loop when i equals 5
}
std::cout << i << " ";
}
return 0;
}
Understanding the `break` Statement
What is the `break` Statement?
The `break` statement in C++ serves as a control mechanism that allows you to exit a loop or switch statement prematurely. This can be particularly useful in situations where you want to halt iteration based on a specific condition without having to run through all iterations of the loop or all cases of a switch statement.
Basic Syntax of `break`
The syntax for using the `break` statement is simple and can be summarized as:
break;
When this statement is encountered within a loop (such as `for`, `while`, or `do-while`) or a switch case, it immediately terminates that block of code, transferring control to the next statement following the loop or switch.
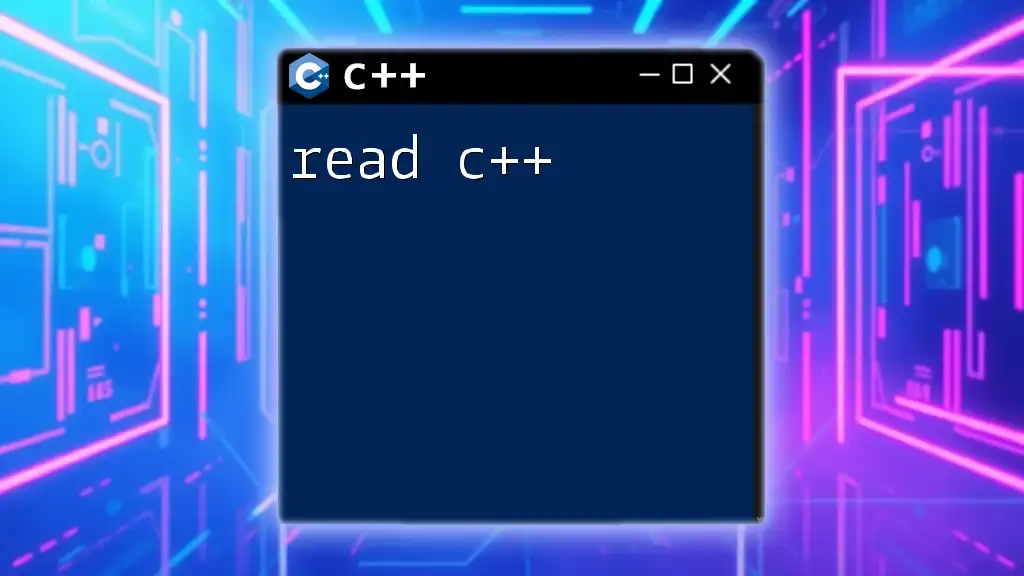
Where to Use `break`
Inside Loops
For Loops
When using the `break` statement within a for loop, it provides a clear exit strategy from the loop based on a specified condition.
Example: Simple for loop demonstration
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // Terminates the loop when i is 5
}
std::cout << i << " ";
}
In this example, the loop runs from 0 to 9, but once `i` equals 5, the `break` statement halts the loop, resulting in the output: `0 1 2 3 4`.
While Loops
In a while loop, the `break` statement functions in the same way—allowing exit when a specific condition is met:
Example: Using break in a while loop
int j = 0;
while (j < 10) {
if (j == 3) {
break; // Terminates the loop when j is 3
}
std::cout << j << " ";
j++;
}
Here, once `j` reaches 3, the loop stops executing. The output will be `0 1 2`.
Inside Switch Statements
The switch statement allows the execution of specific cases based on variable values. Using the `break` statement here is crucial to prevent what is known as fall-through behavior:
Example: Using break to control execution flow
int choice = 2;
switch (choice) {
case 1:
std::cout << "Option 1 selected.\n";
break; // Stops execution after this case
case 2:
std::cout << "Option 2 selected.\n";
break;
default:
std::cout << "No valid option selected.\n";
}
In this example, if `choice` equals 2, the output will be `Option 2 selected.` The `break` statement prevents subsequent cases from executing.
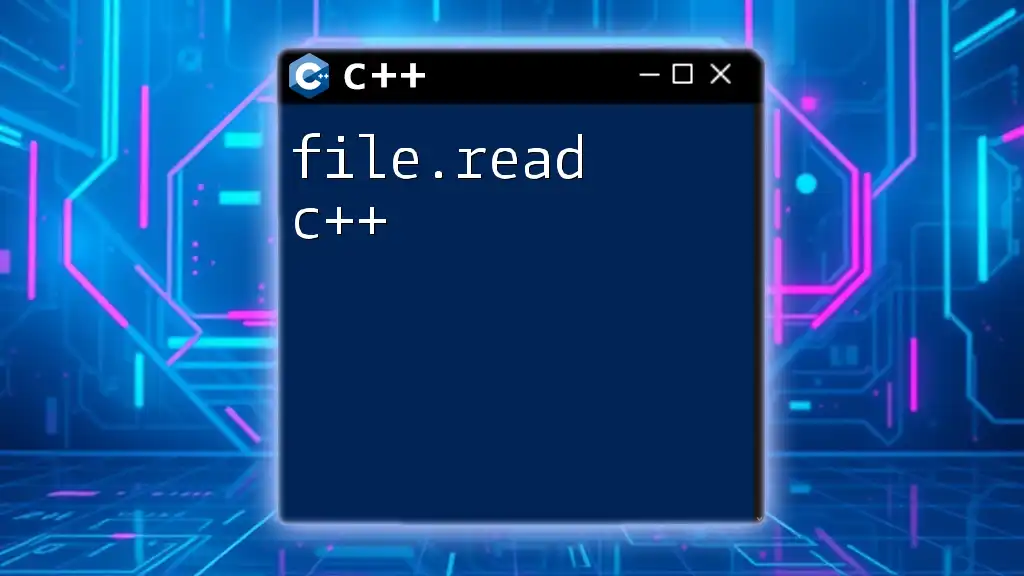
Practical Applications of `break`
Exiting Nested Loops
When working with nested loops, the `break` statement can be pivotal in managing flow control effectively.
Example: Break from inner loop
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 5; j++) {
if (j == 2) {
break; // Breaks the inner loop
}
std::cout << "(" << i << ", " << j << ") ";
}
}
In this code, when `j` equals 2, the inner loop will stop, but the outer loop continues. The output would indicate several pairs until the `j` loop breaks.
Control Flow in Error Handling
Using `break` in error loops can simplify your program's flow:
Example: Simplifying error-checking code
while (true) {
std::cout << "Please enter a number (0 to exit): ";
int number;
std::cin >> number;
if (number == 0) {
break; // Exiting the loop
}
std::cout << "You entered: " << number << std::endl;
}
In this example, the loop continues indefinitely until the user enters `0`, at which point the `break` statement is executed, and the loop exits cleanly.
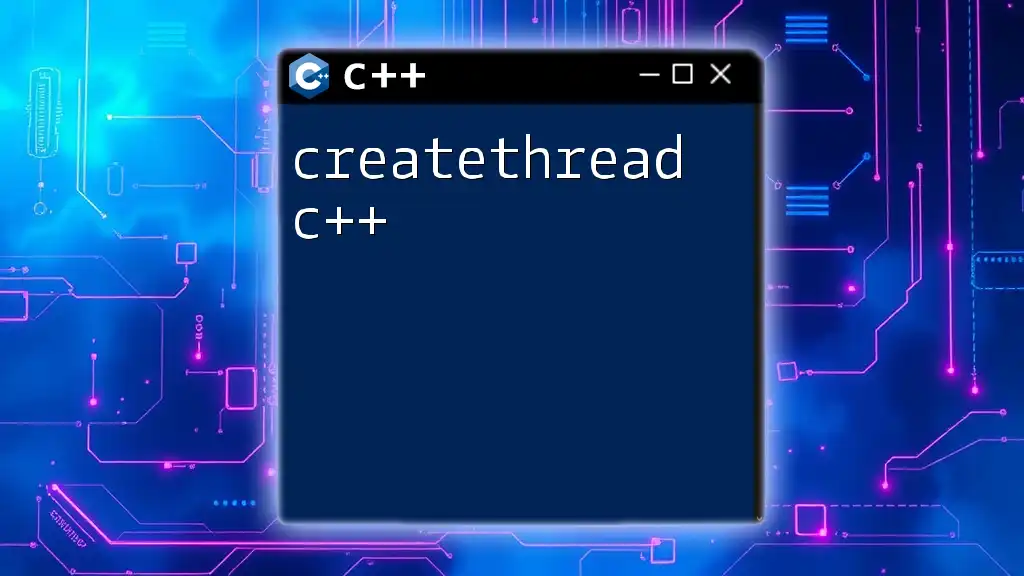
Common Mistakes
Misuse of `break` in Unintended Contexts
One common mistake is placing `break` statements where they don’t belong, such as outside of loops or switch statements. This will cause compilation errors and lead to confusion.
Overusing `break`
Excessive use of `break` can lead to spaghetti code, making your program difficult to read and maintain. Aim for clarity; when the purpose of the loop or switch is compromised, it may be beneficial to consider other structuring methods rather than relying heavily on `break`.
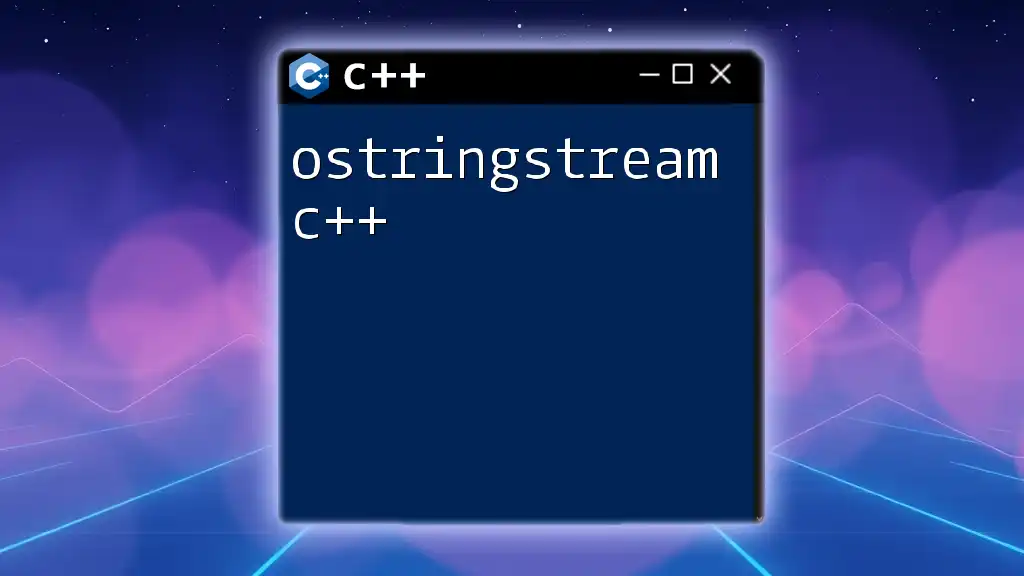
Best Practices
When to Use `break`
Effective usage of `break` is key. Use it judiciously to enhance code clarity. For instance, exiting a loop under specific conditions can often make your code cleaner and more understandable.
Alternatives to `break`
Using Flags
An alternative to `break` can be the implementation of flags that can indicate when to stop the loop. This approach keeps the looping structure intact, which can enhance readability:
bool keepRunning = true;
while (keepRunning) {
// Some logic...
if (condition) {
keepRunning = false; // Instead of using break
}
}
Return Statement
In functions, consider using the return statement to exit early. This adds clarity by specifying that the function will terminate:
void checkValue(int value) {
if (value < 0) {
return; // Exits the function early
}
// Further logic...
}
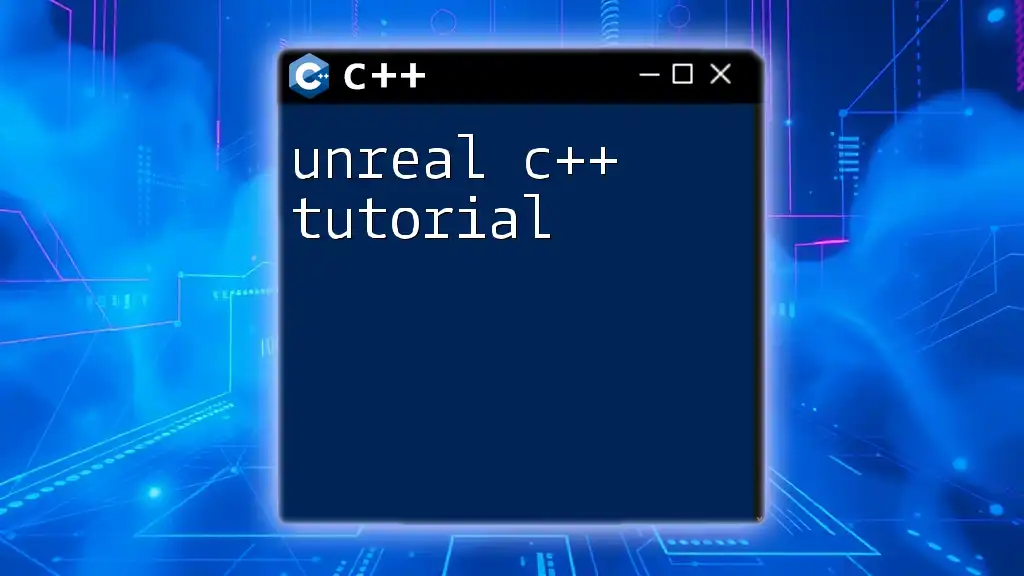
Conclusion
The `break c++` statement is a powerful tool in your programming arsenal. By understanding its use and best practices, you can enhance both the functionality and readability of your code. It’s crucial to practice with examples and identify where `break` can offer the most benefit. Explore further control statement topics in C++ to expand your expertise.