In C++, you can read data from a file using the `ifstream` class along with the `read()` function to directly read binary data or the extraction operator (`>>`) for formatted input.
Here's a code snippet demonstrating how to read from a text file:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt"); // Open the file
std::string line;
if (file.is_open()) { // Check if the file is opened successfully
while (getline(file, line)) { // Read data line by line
std::cout << line << std::endl; // Output each line
}
file.close(); // Close the file
} else {
std::cout << "Unable to open file." << std::endl;
}
return 0;
}
Understanding File Input/Output in C++
File I/O refers to the process of reading data from and writing data to a file, which is a fundamental aspect of C++ programming. Understanding how to work with files is crucial for tasks such as persistent data storage, configuration management, and log analysis.
C++ primarily supports two types of files:
- Text Files: Human-readable files that store data in the form of characters. Examples include `.txt` and `.csv` files.
- Binary Files: Non-human-readable files that store data in a format that is specific to the system or application. Binary files may include executable files or serialized objects.
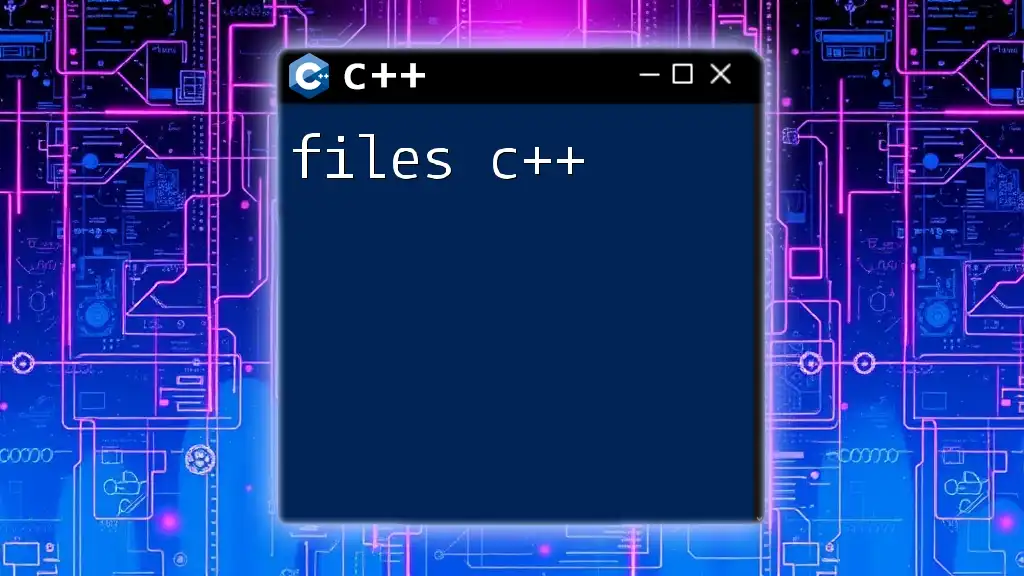
Setting Up for Reading Files in C++
To begin working with file reading in C++, you need to include the necessary libraries that provide file handling functionalities.
Including Necessary Libraries
The essential libraries for file operations are found in the `<fstream>` header, which is part of the standard library.
#include <fstream>
#include <iostream>
#include <string>
By including these libraries, you gain access to the functionalities required to create file streams that can read from files.
Creating a File Stream
To open and read from a file, you will use the `ifstream` class, which stands for "input file stream." This class is used specifically for reading data from files.
std::ifstream inputFile("example.txt");
In this example, an `ifstream` object named `inputFile` is created to read from `example.txt`.
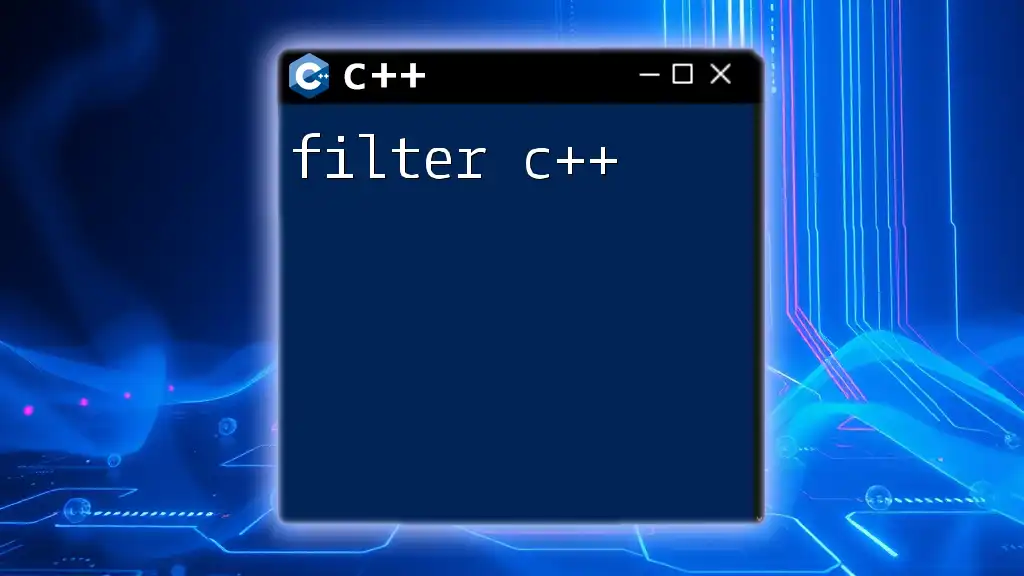
How to Read Data from a File in C++
Basic File Reading Process
Reading data from a file typically involves three steps: opening the file, reading content, and closing the file. A basic example of reading a file is as follows:
std::ifstream inputFile("example.txt");
if (!inputFile) {
std::cerr << "File could not be opened!" << std::endl;
return 1;
}
std::string line;
while (getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
This snippet demonstrates how to open a file and read it line by line until no more lines are available.
Opening and Closing Files
When opening a file, it is essential to verify whether the file opened successfully to avoid runtime errors. The `!inputFile` check validates the stream's state. If the file is not found or cannot be accessed, you should handle the error gracefully.
Always remember to close your file using `inputFile.close()` to free up system resources.
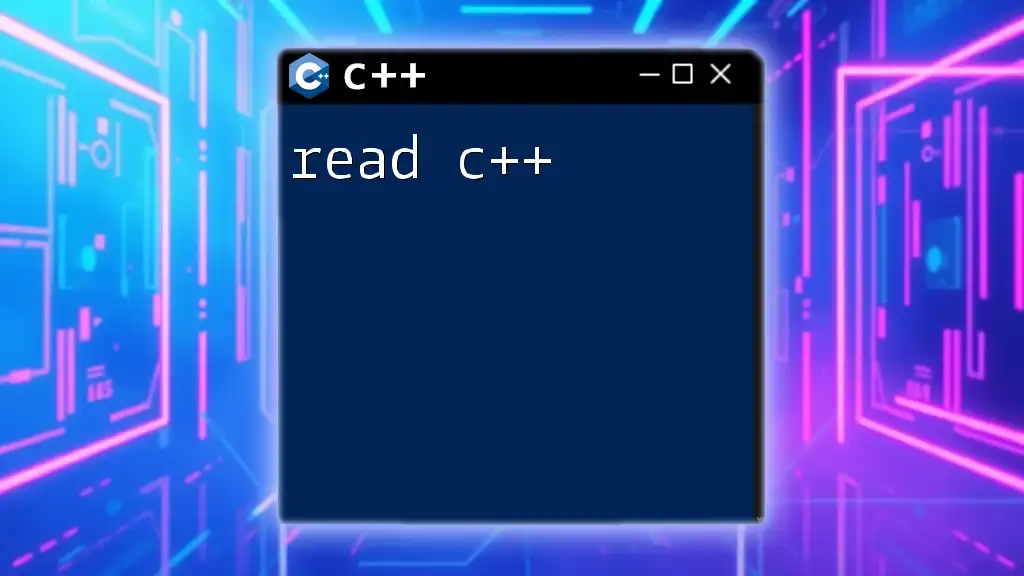
Reading Different Types of Data
Reading Text Files
To read lines from a text file, the `getline()` function is particularly effective, as demonstrated in the earlier code snippet. It reads an entire line from the file and stores it into a string variable.
Reading Numbers from a File
If your file contains numerical data, you can read integers or floating-point numbers easily using the extraction operator (`>>`).
std::ifstream numFile("numbers.txt");
int number;
while (numFile >> number) {
std::cout << number << std::endl;
}
In this example, `numFile >> number` reads integers from `numbers.txt`.
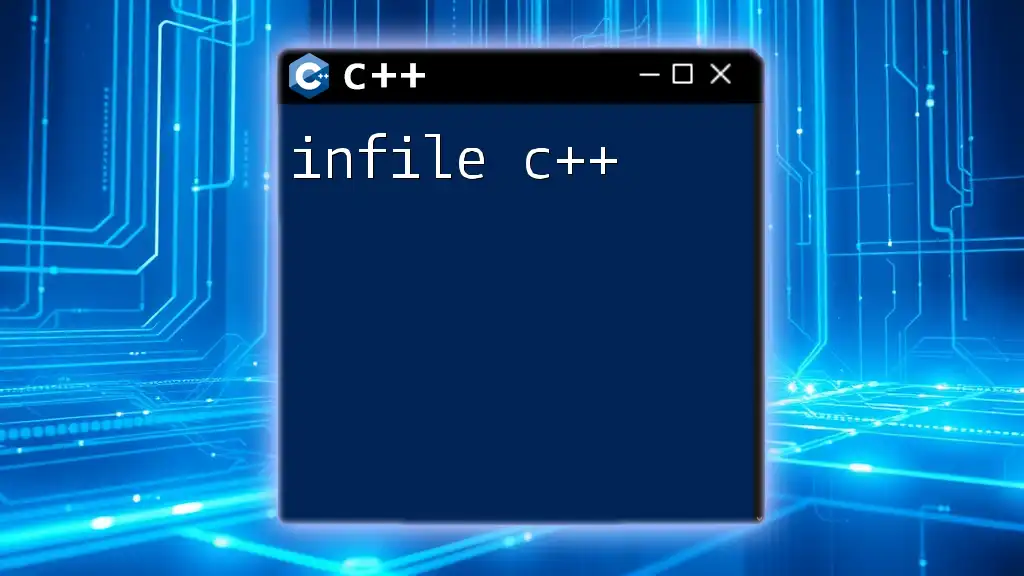
Advanced Techniques for Reading Files
Reading Word by Word
If you need to extract data word by word, you can achieve this with the extraction operator as well.
std::ifstream wordFile("words.txt");
std::string word;
while (wordFile >> word) {
std::cout << word << std::endl;
}
This reads each word from the file `words.txt` and prints it out.
Reading with a Loop
When reading data from a file, it is common to use a loop to process each line or item until the end of the file (EOF) is reached. Using a loop ensures you don’t miss any data.
std::ifstream inputFile("sample.txt");
std::string line;
while (getline(inputFile, line)) {
// Do something with the line
}
This structure is robust and should be used whenever reading lines from text files.
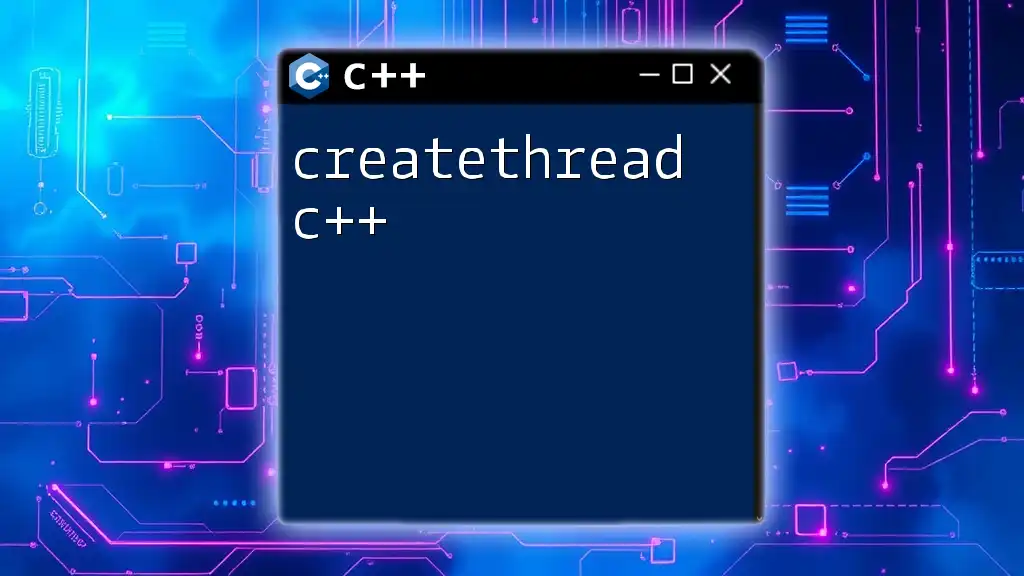
Error Handling While Reading Files
Common File Read Errors
While working with files, you may encounter common errors such as:
- File not found: If the specified file does not exist.
- Permission denied: If the file is not accessible due to permission issues.
Using `fail()` and `eof()`
C++ provides functions that help in error handling during file reading.
if (inputFile.fail()) {
std::cerr << "Failed to read the file!" << std::endl;
}
if (inputFile.eof()) {
std::cout << "End of file reached." << std::endl;
}
The `.fail()` function detects if an error occurred in the stream, whereas `.eof()` checks if the end of the file has been reached.
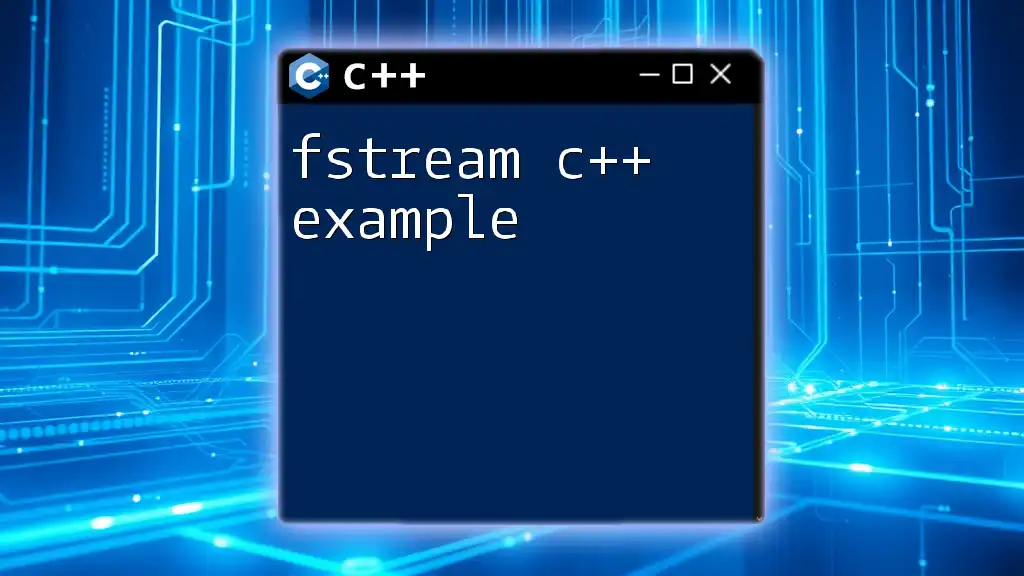
Real-World Applications
Storing User Input and Configuration Data
File reading is often used to store user configurations or preferences, allowing applications to load settings during startup.
Data Analysis and Log File Processing
Another common application is analyzing large datasets or processing log files. By reading data from these files, you can extract insights or investigate user behavior.
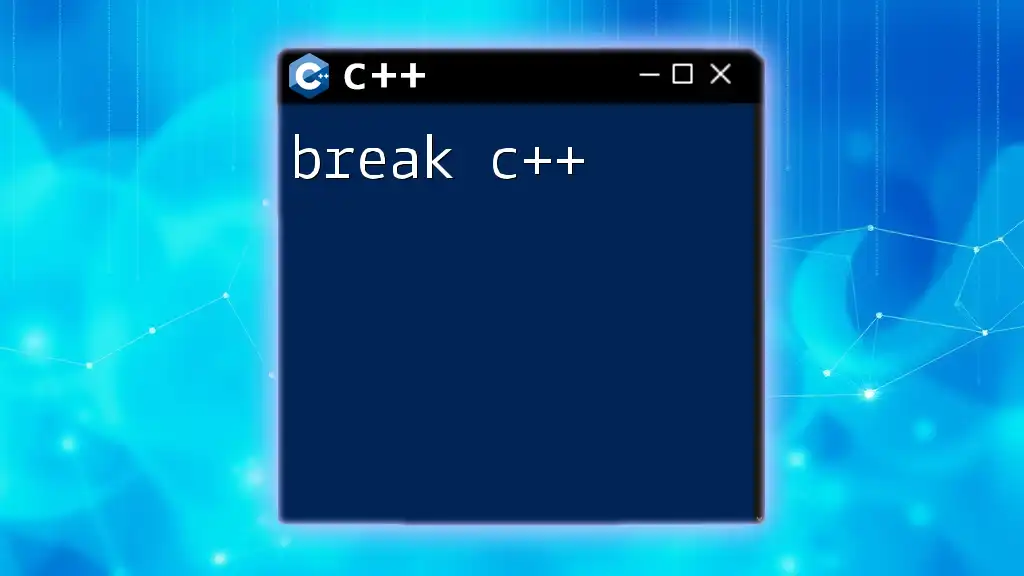
Best Practices in File Reading in C++
Always Check File Open Status
Before attempting to read from a file, always check if the file opened successfully. This practice prevents unnecessary exceptions or crashes.
Use Smart Pointers for Resource Management
Utilize smart pointers wherever possible. For instance, employing `std::unique_ptr` can help manage file resource lifetimes automatically, reducing the risk of memory leaks.
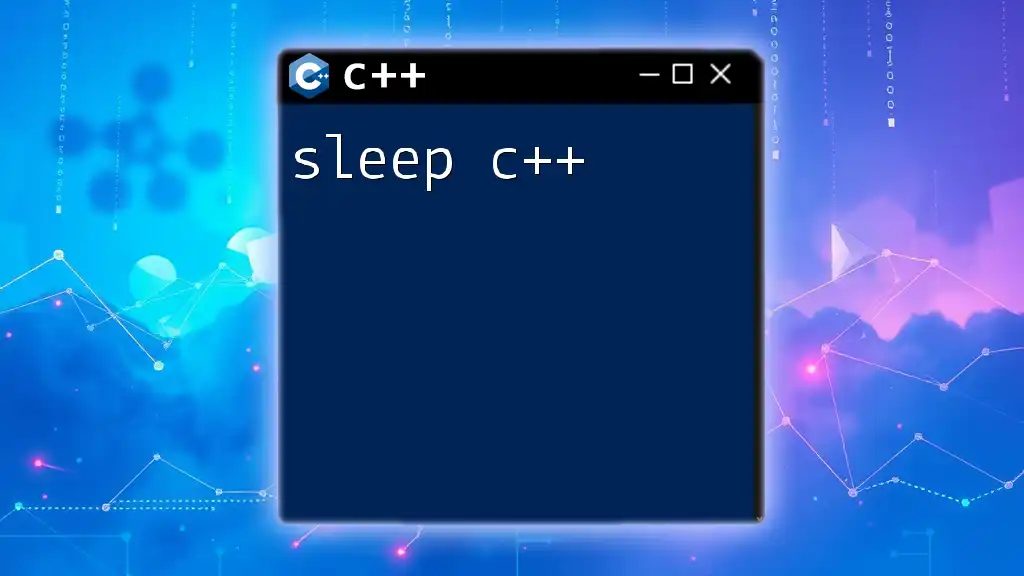
Conclusion
File reading in C++ is a fundamental skill that every programmer should master. Understanding how to read various data formats and handle potential errors will significantly enhance your programming capabilities. Practice with different types of files and use the best practices discussed to ensure smooth operations in your applications.
![Effortless Memory Management: Delete[] in C++ Explained](/images/posts/d/delete-cpp.webp)
Additional Resources
Recommended Books and Online Resources
- Consider exploring classic C++ programming books or online tutorials that delve deeper into file handling and standard library usage.
Commonly Asked Questions
- Don't hesitate to explore FAQs or forums for troubleshooting common issues related to file reading in C++. This will enhance your understanding even further.