Valgrind is a powerful programming tool in C++ that helps detect memory leaks and memory-related errors, improving the performance and reliability of your applications.
Here's a simple example of how to use Valgrind to check for memory leaks in a C++ program:
#include <iostream>
int main() {
int* arr = new int[10]; // allocate memory for an array of 10 integers
// Memory leak occurs here if we forget to free the allocated memory
return 0;
}
To run Valgrind, you would compile your program and then execute it using Valgrind like this:
g++ -o example example.cpp
valgrind --leak-check=full ./example
What is Valgrind?
Valgrind is a programming tool used primarily for memory debugging, memory leak detection, and profiling in C and C++ applications. As C++ developers, understanding Valgrind is crucial because managing memory efficiently is one of the distinct challenges of programming in languages like C++. Valgrind helps you identify issues that could lead to crashes or unexpected behavior in your applications.
Why Use Valgrind?
Using Valgrind is essential in ensuring that your C++ code is robust and free of memory-related errors. Here are some reasons why you should incorporate Valgrind into your development workflow:
- Debugging Memory Issues: Many common issues, such as memory leaks and access violations, can be difficult to diagnose. Valgrind provides insights that can lead you directly to the source of the problem.
- Improving Application Performance: Understanding how memory is allocated and used enables you to optimize your application, leading to performance improvements.
- Enhancing Code Quality: Regular use of Valgrind can foster good coding practices and help maintain high standards in memory management.
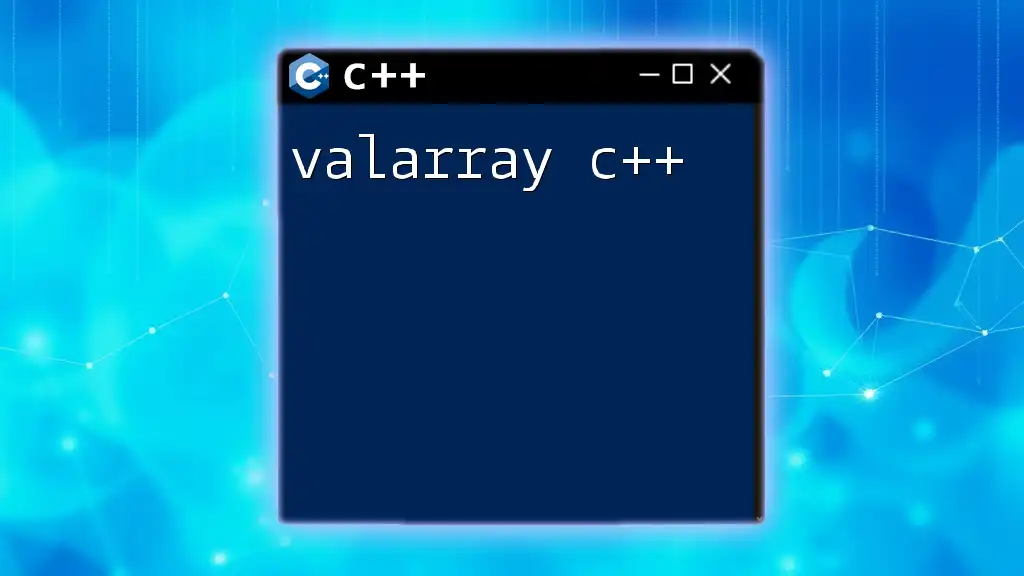
Getting Started with Valgrind
Installation of Valgrind
To start using Valgrind, you'll first need to install it. It is available on most Unix-like operating systems, including Linux and macOS. Here’s how you can install Valgrind:
-
Linux: You can typically install Valgrind using the package manager. For example, on Ubuntu, you would run:
sudo apt install valgrind
-
macOS: If you use Homebrew, you can install it by running:
brew install valgrind
Basic Usage of Valgrind
Once Valgrind is installed, using it is straightforward. The primary command syntax for running Valgrind is:
valgrind ./your_program
This command will start Valgrind and execute your program. By default, Valgrind will run in a mode that detects memory leaks and memory access violations.
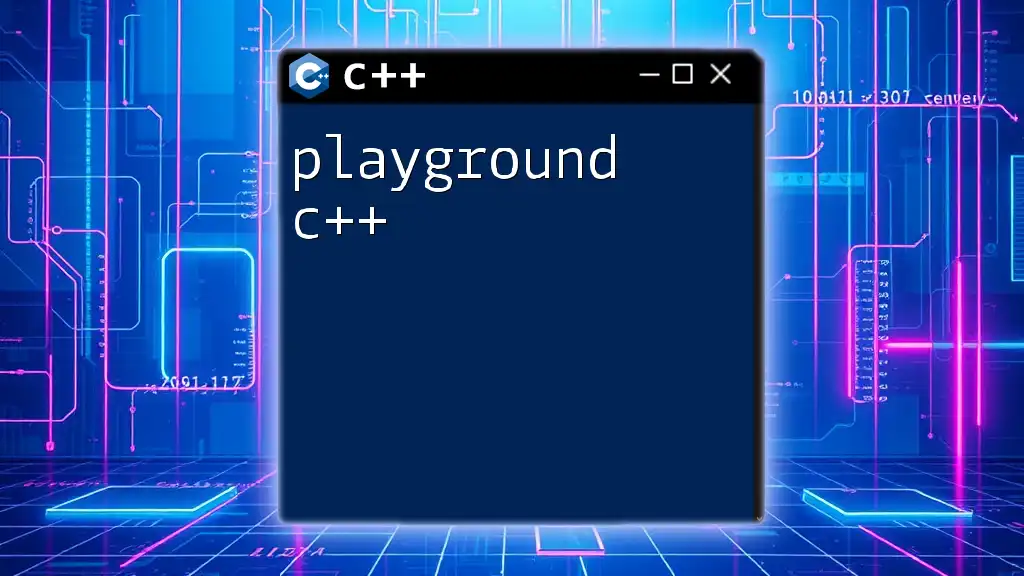
Understanding Valgrind’s Output
Types of Memory Issues Detected by Valgrind
Valgrind can identify several types of memory issues, each of which can lead to serious bugs if overlooked:
Memory Leaks
A memory leak occurs when allocated memory is not freed, which can lead to increased memory usage over time. Memory leaks can negatively affect application performance and stability. Consider the following example:
void createMemoryLeak() {
int* leak = new int[10]; // Memory is allocated
// Memory is never released
}
Running this code through Valgrind would indicate that there is a memory leak.
Invalid Memory Access
Accessing memory that your program does not own results in undefined behavior, which may cause crashes or corrupted data. For instance:
void invalidAccess() {
int* ptr = nullptr;
*ptr = 100; // Attempting to write to a null pointer
}
Valgrind would output errors indicating that invalid memory was accessed.
Uninitialized Memory Reads
Reading from memory that has not been initialized can lead to unpredictable results. Here's an example:
void uninitializedRead() {
int x;
std::cout << x; // x is uninitialized
}
In this instance, running Valgrind will produce warnings about reading uninitialized memory.
Reading Valgrind Reports
Understanding the output produced by Valgrind is essential for diagnosing issues. Valgrind reports will include the type of error detected, the location in your code, and a stack trace to help you find the root cause.
For example, a typical Valgrind output for a memory leak might look like this:
==1234== LEAK SUMMARY:
==1234== definitely lost: 40 bytes in 1 blocks
==1234== indirectly lost: 0 bytes in 0 blocks
Look for the “definitely lost” statement to identify memory leaks and trace it back to the relevant parts of your code.
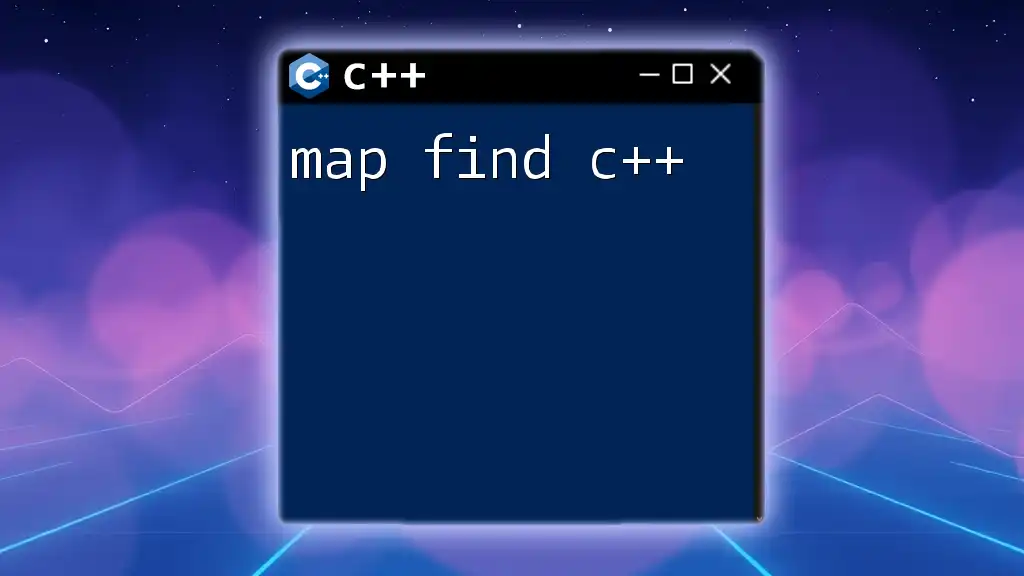
Common Valgrind Commands and Options
Key Command-Line Options
Valgrind offers a variety of command-line options that enhance its functionality:
`--leak-check=full`
This option causes Valgrind to provide detailed information about memory leaks:
valgrind --leak-check=full ./your_program
`--track-origins=yes`
This useful flag helps track down the origins of uninitialized values, making it easier to debug:
valgrind --track-origins=yes ./your_program
`--error-exitcode=1`
When you want Valgrind to exit with an error code on detecting memory issues, use:
valgrind --error-exitcode=1 ./your_program
Example Command-line Usages
Combining Valgrind options can provide deeper insights. Here’s a full command example that uses multiple flags:
valgrind --leak-check=full --track-origins=yes ./your_program
This command runs your program while enabling comprehensive leak checking and debugging uninitialized reads.
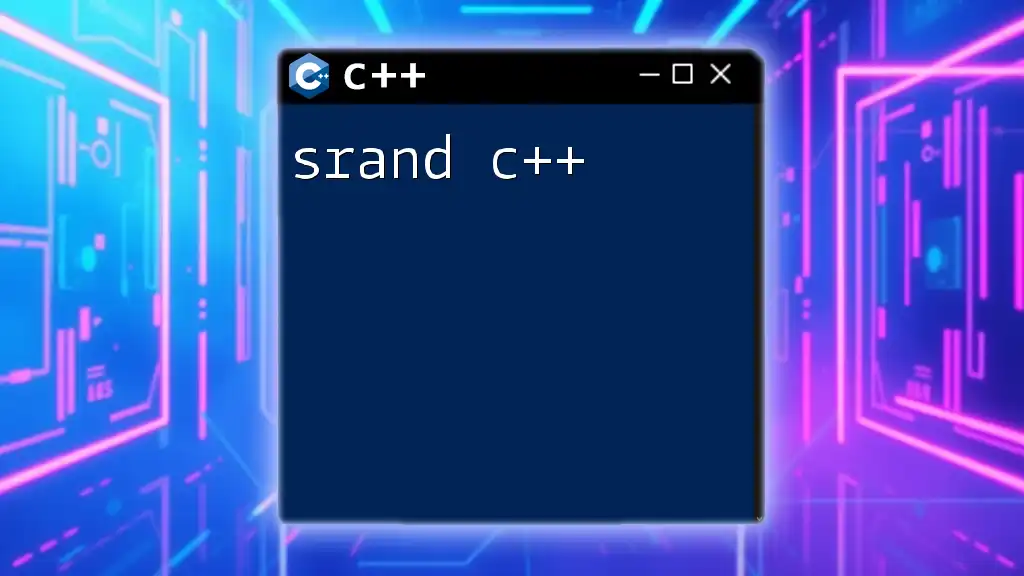
Advanced Valgrind Features
Suppression Files
Sometimes, certain errors may not impact your program's execution but can clutter the output from Valgrind. In such cases, suppression files can be used to ignore known issues:
- Identify the output that you want to suppress.
- Create a suppression file (e.g., `suppressions.txt`) with content similar to:
{
MemCheck:Leak
MemCheck:Leak:OBJ
}
- Use the file with Valgrind by running:
valgrind --suppressions=suppressions.txt ./your_program
Using Valgrind with Multi-threaded Applications
Debugging multi-threaded applications can be more complex due to concurrent memory access. Valgrind has ways to help:
- Use the `--track-fds=yes` option to track file descriptors.
- Be aware of race conditions, as multiple threads can create unique memory access patterns.
Analyzing thread interactions can be invaluable for identifying subtle bugs.
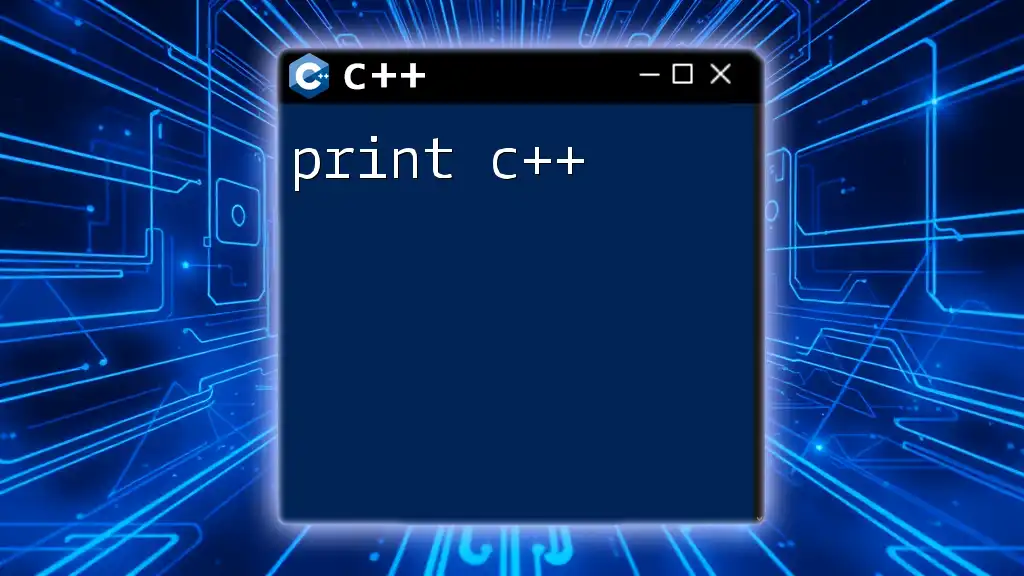
Best Practices When Using Valgrind
Integrating Valgrind into Your Development Workflow
Make Valgrind part of your regular testing cycle. Run Valgrind against your applications before major releases or during significant changes. Regular usage will lead to faster debugging as you become accustomed to identifying issues.
Interpreting Results Wisely
Remember that not all reported issues may be critical. Use your judgment to determine the significance of the reported problems and prioritize fixes based on their impact.
Combining Valgrind with Other Tools
Valgrind is a powerful tool, but it works best when combined with other debugging tools. Pair it with GDB for more comprehensive debugging sessions, or consider using sanitizers like AddressSanitizer alongside Valgrind for even greater code safety.
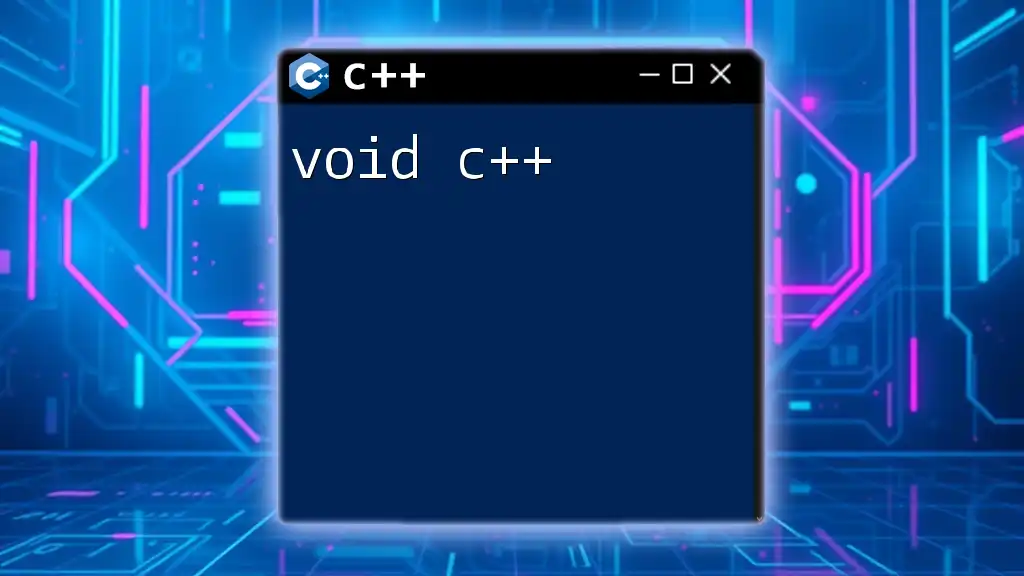
Conclusion
Incorporating Valgrind into your development process significantly enhances your ability to manage memory effectively in C++. With its robust toolset for diagnosing memory leaks, invalid accesses, and other problems, Valgrind is an indispensable asset in ensuring the quality and reliability of your code.
As you gain experience using Valgrind, don't forget the importance of practice. Creating sample projects and testing them with Valgrind will enhance your skills and deepen your understanding of memory management.
Further Resources
For more in-depth information on Valgrind, consider exploring the official Valgrind documentation, online tutorials, and community forums that focus on C++ memory management. These resources can help you further refine your skills and tackle complex issues as you continue your programming journey.