Aliasing in C++ allows you to create an alternative name for an existing variable or type, which can improve code readability and maintainability.
Here’s an example of aliasing using type definitions and reference variables:
#include <iostream>
using namespace std;
int main() {
int originalVariable = 42;
int& aliasVariable = originalVariable; // Creating an alias using a reference
cout << "Original: " << originalVariable << endl; // Outputs: Original: 42
aliasVariable = 100; // Modifying the alias affects the original
cout << "Modified Original: " << originalVariable << endl; // Outputs: Modified Original: 100
return 0;
}
What is Aliasing?
Aliasing in C++ refers to the phenomenon where two or more variables refer to the same memory location. When aliasing occurs, changes made through one variable affect all other aliases. Understanding aliasing is crucial for any C++ programmer, as it plays a significant role in memory management, debugging, and code efficiency.

Why is Aliasing Important?
In C++, aliasing offers several benefits, such as:
- Memory efficiency: By using aliases, programmers can save memory as multiple references can point to the same data rather than creating copies.
- Code readability: Aliases can help make the code clearer by giving meaningful names to references.
- Impact on performance: Understanding aliasing can enable better optimizations, leading to faster-running code.

Types of Aliasing in C++
Definition Aliasing
Definition aliasing occurs when two variables directly refer to the same memory location. This is a common practice when using references.
Example:
int a = 5;
int& b = a; // b is an alias for a
In this example, both `a` and `b` point to the same integer. If you modify `b`, `a` will also reflect that change, demonstrating direct interaction between aliases.
Assignment Aliasing
Assignment aliasing is when a variable is assigned the value of another variable, creating a copy rather than an alias.
Example:
int a = 10;
int b = a; // Assignment creates a copy, not an alias
In this scenario, changing `b` will not affect `a`, as they are stored in different memory locations.
Pointer Aliasing
Pointer aliasing occurs when two pointers point to the same memory address. This type of aliasing is crucial in dynamic memory management.
Example:
int a = 20;
int* p = &a; // p points to a
Here, `p` is an alias for `a`, since it stores the address of `a`. Any modifications done through `p` will directly affect `a`.
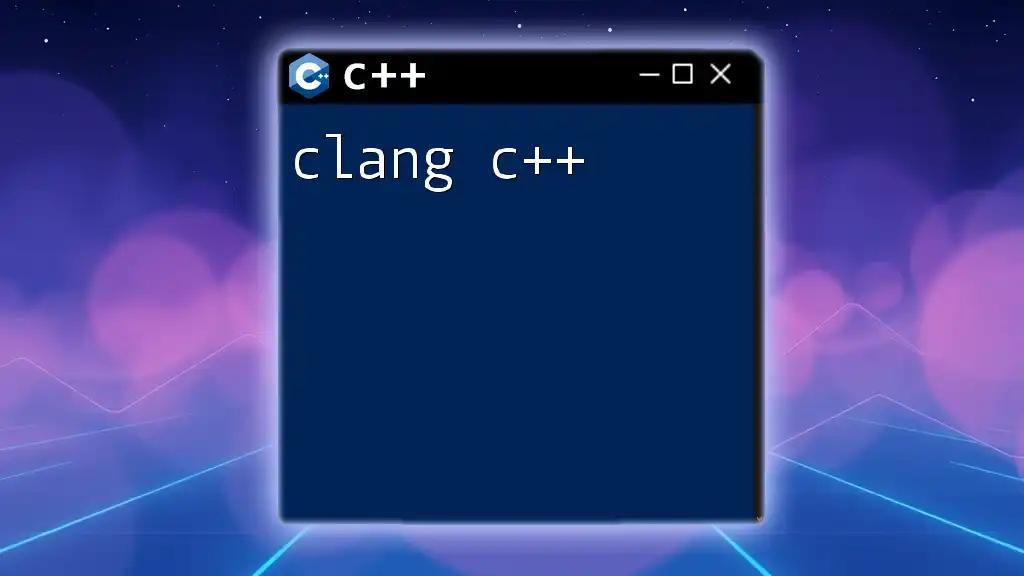
Consequences of Aliasing
Performance Considerations
Aliasing can have significant implications for performance. In many cases, compilers perform optimizations, assuming that distinct variables are independent. When aliasing is present, these assumptions may be violated, leading to less efficient code execution.
Additionally, cache performance can be compromised. When multiple aliases reference the same data, cache coherence may become an issue, potentially slowing down access times.
Undefined Behavior
Aliasing can also lead to undefined behavior, especially if care is not taken. One common issue arises when aliasing is used alongside mutable and immutable data types.
Example:
int a = 1;
int* p = &a;
*p = 2; // Alters a
a = 3;
In this example, `a` is modified through the pointer `p`, and due to how compilers might optimize this code, it could lead to unpredictable results.
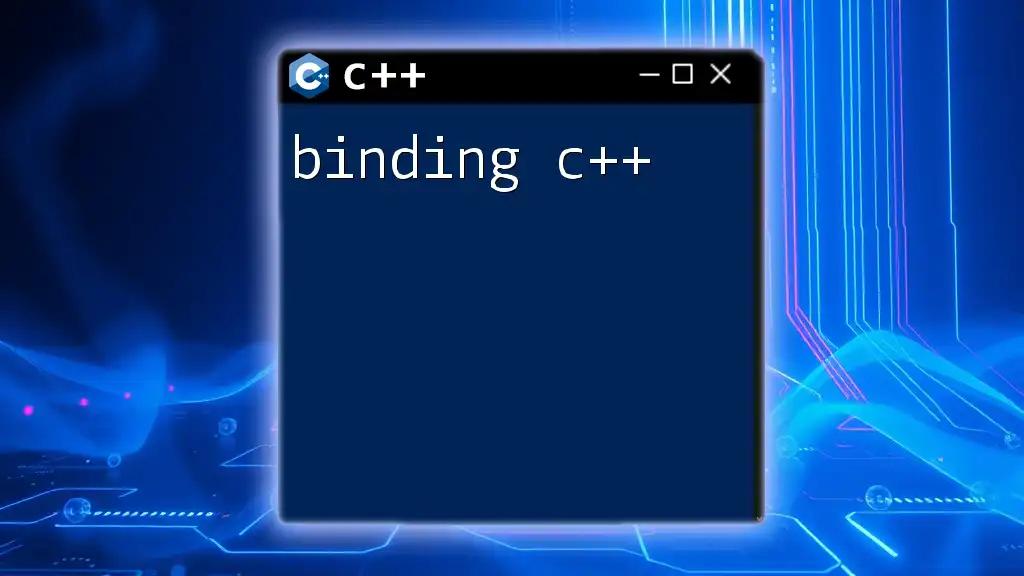
Best Practices to Avoid Issues with Aliasing
Use `const` References
By using `const` references, you limit the possibility of unintended modifications, which is particularly useful when passing large data structures or complex objects to functions.
Example:
void func(const int& x) {
// Cannot change x here
}
This practice enhances code safety and clarity.
Avoiding Global Variables
Global variables are a frequent source of aliasing issues, particularly in multithreaded contexts. Instead of globals, prefer using encapsulation through classes or namespaces to manage state.
Employing Smart Pointers
Smart pointers manage ownership and lifetime of dynamically allocated memory, thereby reducing aliasing-related bugs.
Example:
std::shared_ptr<int> ptr1 = std::make_shared<int>(10);
auto ptr2 = ptr1; // ptr2 is another alias
Using smart pointers like `std::shared_ptr` ensures better memory management and minimizes risks associated with raw pointers.
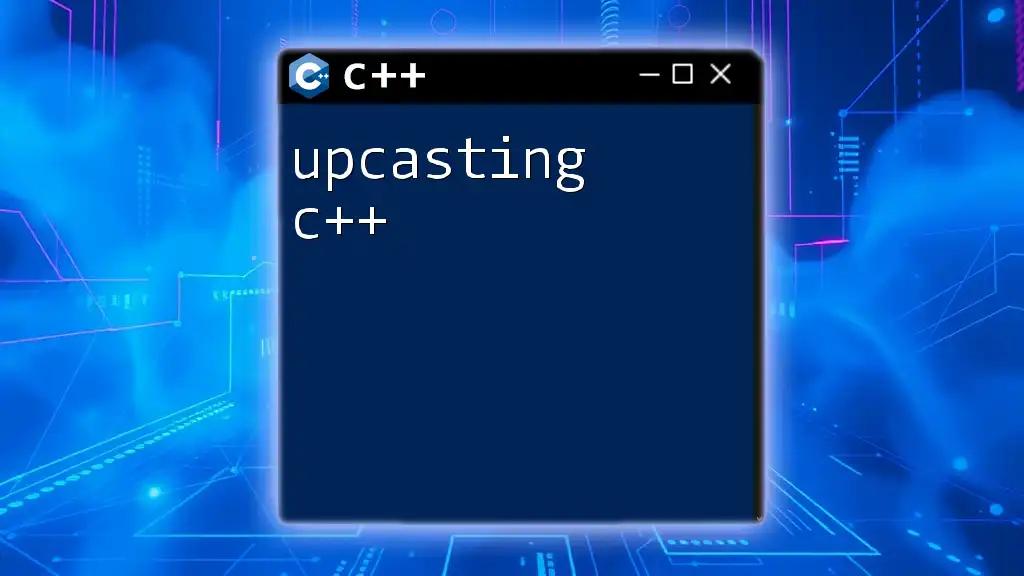
Common Mistakes with Aliasing
Using Aliases in Function Parameters
One common pitfall arises when using non-const references as function parameters. If the function modifies the parameter, changes will propagate to the argument used to call it.
Example:
void func(int& x) {
x = 5; // Modified outside scope
}
This could lead to unexpected side effects, making code harder to read and debug.
Pointer Arithmetic
Pointer arithmetic can complicate aliasing further, especially when used in conjunction with arrays. Miscalculating bounds or modifying pointers can result in significant errors.
Example:
int arr[5] = {1, 2, 3, 4, 5};
int* p = arr;
p += 3; // p points to arr[3], which changes its meaning
In this case, `p` does not just point to the first element, and misinterpretation can lead to unwanted behavior.
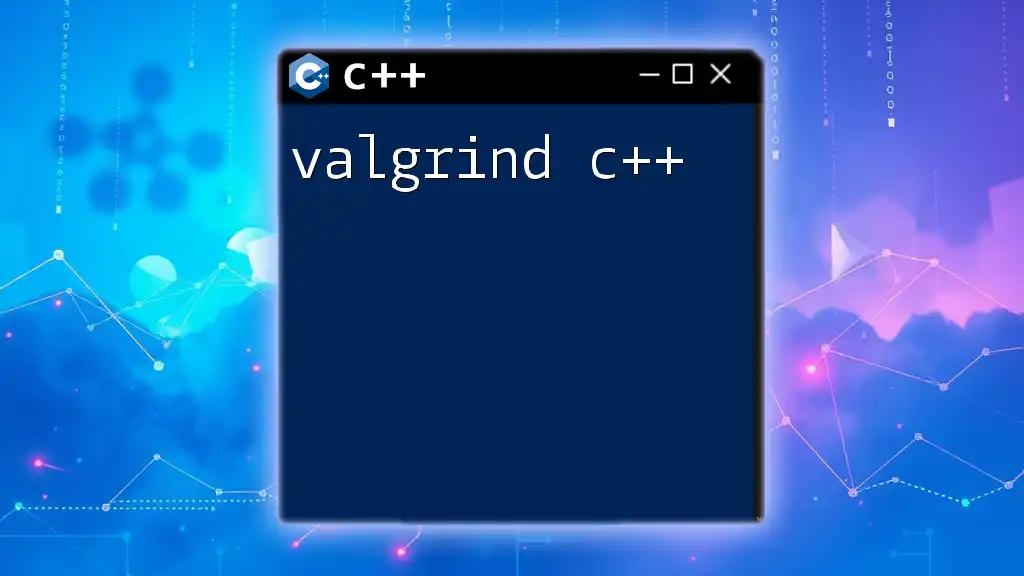
Aliasing in Templates
Template Parameters and Aliasing
When using templates, aliasing can complicate the behavior of both function and class templates. If parameters are passed by reference without `const`, the original data can be altered unintentionally.
Example:
template<typename T>
void process(T& x) {
// x is an alias and changes the original object
}
It’s crucial to be mindful of how aliasing works in templates to prevent issues stemming from unintended modifications.
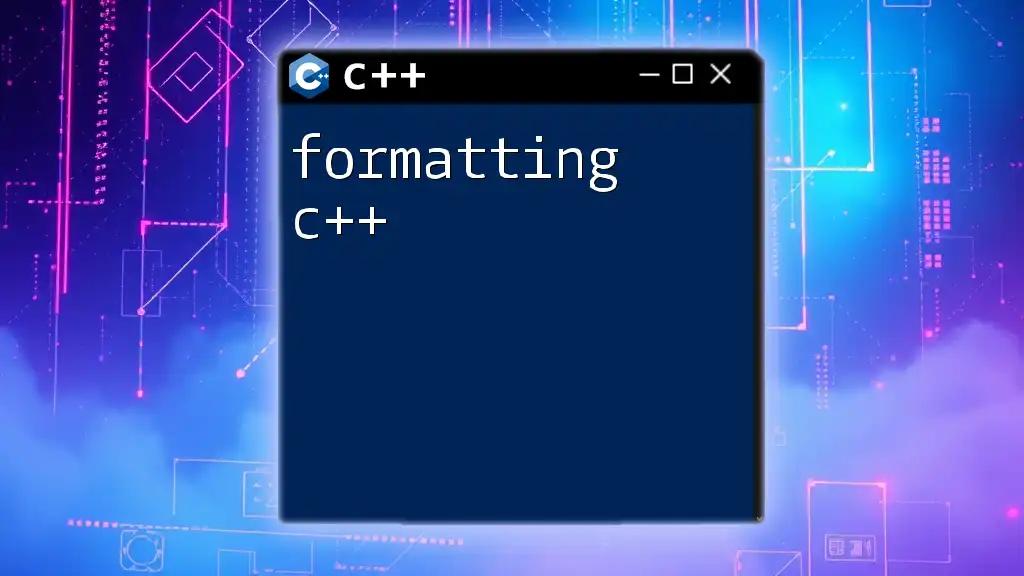
Conclusion
Understanding aliasing in C++ is essential for both performance and code reliability. By recognizing its various forms, consequences, and best practices, you can write more effective and clearer code. This comprehension will not only mitigate bugs but also optimize code performance.
Further Reading and Resources
For those looking to deepen their knowledge on effective C++ programming, consider exploring advanced topics such as memory management, multithreading, and C++ design patterns. Books, online courses, and coding forums are excellent resources for continued learning.