In C++, assignment is the process of storing a value in a variable using the assignment operator `=`, which can be demonstrated with the following example:
int x = 5; // Assigns the value 5 to the variable x
Understanding Assignment in C++
What is Assignment?
Assignment in programming refers to the process of storing a value in a variable. It’s a fundamental operation in C++ that allows you to allocate data to a memory location so it can be used later in your program. In C++, the assignment operation is crucial because it allows programmers to manipulate data effectively, making it an essential component for writing dynamic and functional code.
How Assignment Works in C++
When you assign a value to a variable in C++, it is important to understand how the value is stored in memory. The variable acts as a placeholder, and the assignment operation associates the value with that placeholder. Each data type has a specific memory requirement, and it’s essential to use compatible types during assignment.
Data Types and Assignment Compatibility:
C++ is a statically typed language, meaning the type of every variable must be declared before it can be used. When assigning a value, you must ensure that the value's type matches or is compatible with the variable's type. Assigning incompatible types can lead to data loss or compile-time errors.
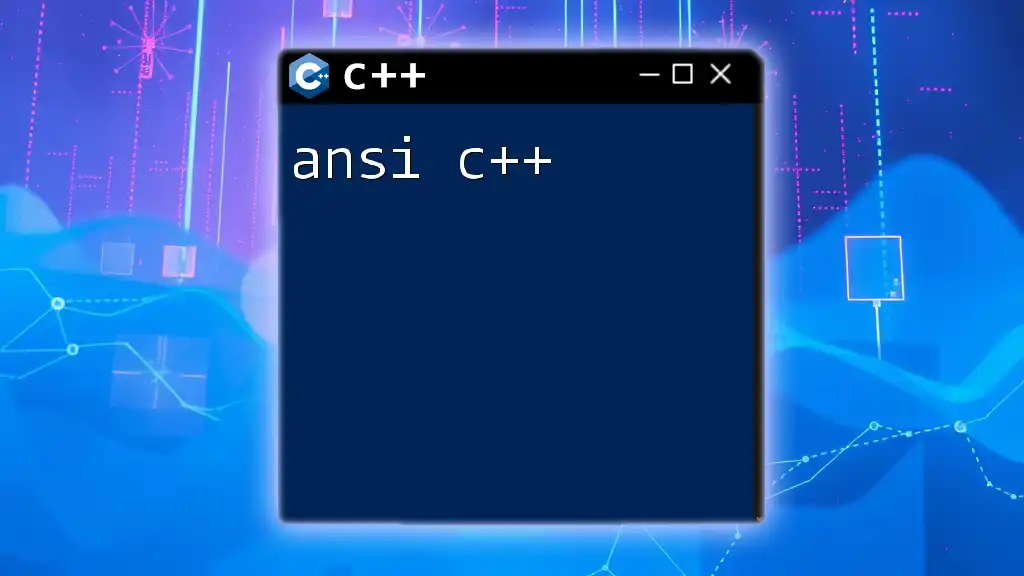
The Assignment Operator in C++
The Simple Assignment Operator `=`
The simplest way to assign a value to a variable in C++ is by using the assignment operator `=`.
int a = 5;
In this code, the variable `a` is initialized with the value `5`. The assignment operator takes the value on the right (in this case, `5`) and stores it in the variable on the left (`a`). Understanding this fundamental behavior is crucial for effective programming.
Compound Assignment Operators
C++ offers several compound assignment operators, which allow you to perform operations and assignments in a single statement. These operators not only modify the variable but also help concise your code.
Types of Compound Operators:
- `+=` (Addition assignment): Adds the right operand to the left operand and assigns the result to the left operand.
- `-=` (Subtraction assignment): Subtracts the right operand from the left operand and assigns the result to the left operand.
- `*=` (Multiplication assignment): Multiplies the right operand with the left operand and assigns the result to the left operand.
- `/=` (Division assignment): Divides the left operand by the right operand and assigns the result to the left operand.
- `%=` (Modulus assignment): Computes the modulus of the left operand by the right operand and assigns it to the left operand.
Examples of Compound Assignments
Here’s a simple example using the `+=` operator:
int a = 10;
a += 5; // a now equals 15
In this case, `5` is added to the current value of `a`, which updates `a` to `15`. Each compound operator works similarly, allowing for succinct and efficient code.
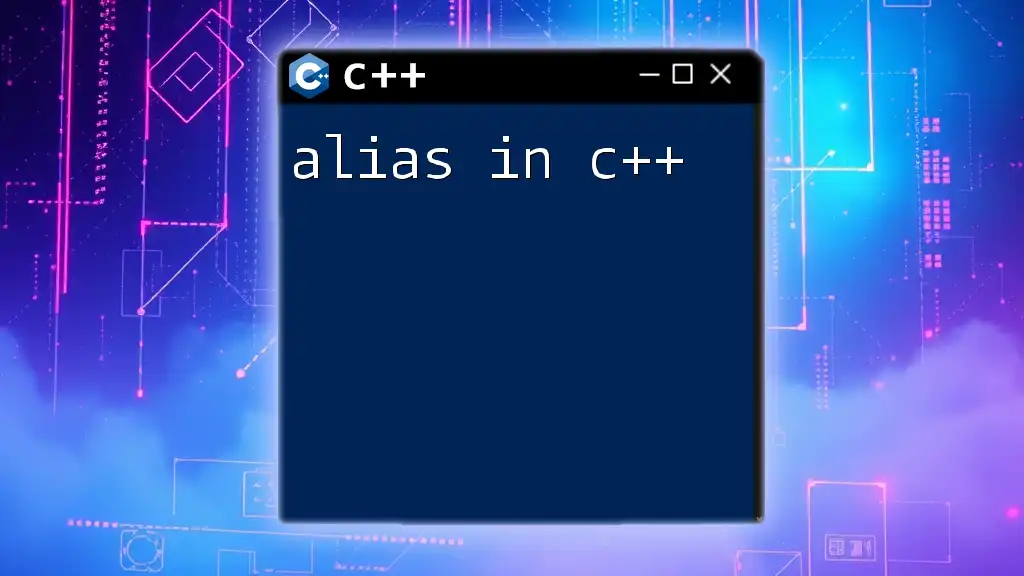
Assignment and Data Types
Integers, Floats, and Doubles
C++ supports a variety of numeric types, with integer, float, and double being the most common.
int intVar = 10;
float floatVar = 10.5f;
double doubleVar = 20.25;
Type Safety and Conversion: When assigning values of different types, it’s important to be aware of potential issues. For instance, if you attempt to assign a float like `10.5` to an integer variable, C++ will truncate the decimal portion, leading to potential data loss.
Character and String Assignments
Character and string types also utilize assignment:
char charVar = 'A';
For strings, using the C++ standard library:
#include <string>
std::string strVar = "Hello, World!";
Handling String vs. Character Arrays: C-style strings, which are arrays of characters, behave differently than `std::string` in terms of assignment and manipulation. Understanding these differences ensures better memory management and manipulation of string data.
User-Defined Data Types
C++ allows users to define their own data types such as structures and classes:
struct Person {
std::string name;
int age;
};
Person p;
p.name = "John";
p.age = 30;
When assigning values to user-defined data types, consider deep and shallow copies. A shallow copy refers to copying the reference of an object, while a deep copy creates an entirely new object with its own copies of the data. This distinction becomes crucial when dealing with dynamic memory allocation, as failing to properly manage memory can lead to leaks or undefined behavior.
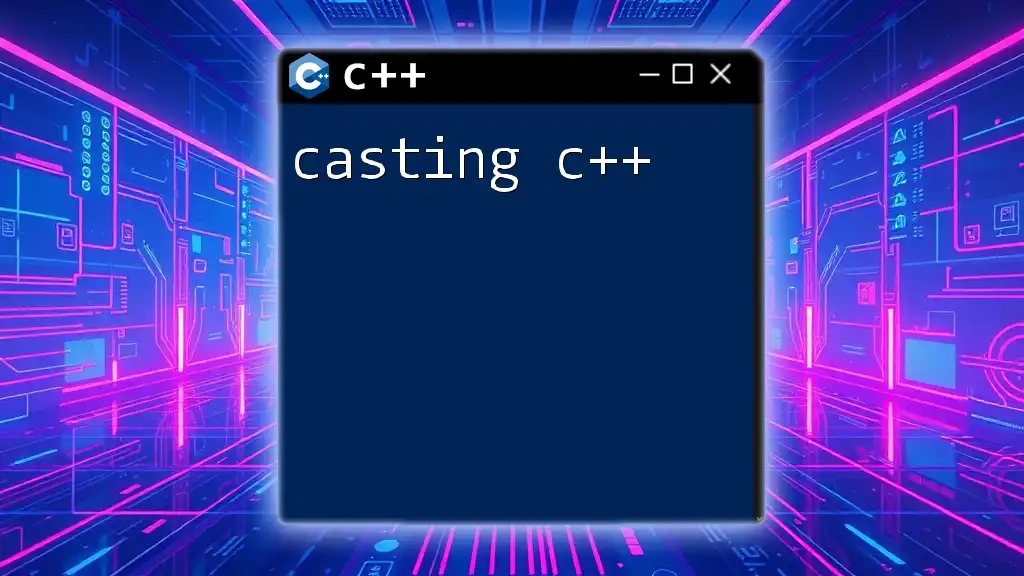
Common Pitfalls in Assignment
Uninitialized Variables
One common mistake in C++ programming is attempting to use uninitialized variables. When a variable is declared but not initialized, it contains an unpredictable value, which can lead to erratic behavior in your programs.
Overwriting Data
Care must be taken to avoid unintentional data loss when reassigning variables. For instance, if you reassign a variable without saving its current value somewhere else, that information is lost.
Implicit Type Conversions
C++ performs implicit type conversions when assigning values to variables. While this feature can be handy, it can also introduce errors if you aren't careful. For example, assigning a larger numeric type to a smaller one can cause value truncation, leading to unexpected outcomes.
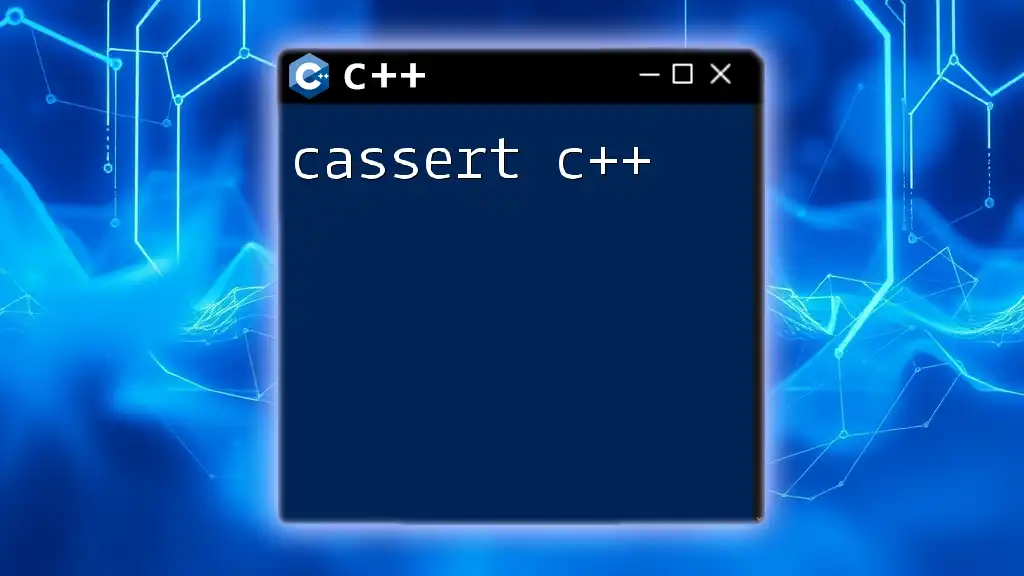
Best Practices for Assignment in C++
Clear and Meaningful Variable Names
Using clear and descriptive variable names increases the readability of your code. This practice prevents confusion when assigning values, helping others (or even yourself in the future) understand what each variable represents.
Avoiding Magic Numbers
Instead of using arbitrary numbers within your code (often referred to as "magic numbers"), assign descriptive constants. For example:
const int maxUsers = 100;
This approach not only increases clarity but also makes it easier to modify the program later.
Comments and Documentation
Effective use of comments is crucial in coding. Comment your assignment statements to indicate their purpose and any important details, making your code more maintainable.
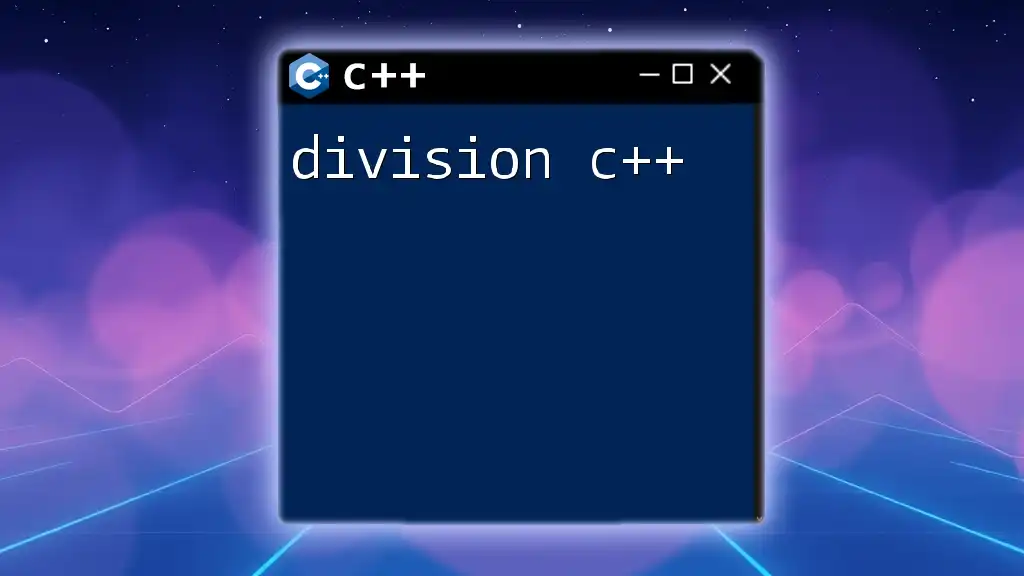
Conclusion
Assignment is a fundamental concept in C++ programming that merits attention and understanding. From the simple assignment operator to the nuances of user-defined data types, mastering assignments will significantly enhance your programming skills. By adhering to best practices and being aware of common pitfalls, programmers can ensure clean, efficient, and error-free code.
Remember, programming is a continuous learning journey. Practice assigning values in different contexts to strengthen your understanding, and explore additional resources to expand your knowledge further.