An ASCII C++ table is a representation of ASCII characters along with their corresponding integer values that can be generated using C++ code, making it easier for developers to reference character encoding.
Here’s a simple code snippet to display an ASCII table in C++:
#include <iostream>
int main() {
std::cout << "ASCII Table:\n";
std::cout << "Char\tInt\n";
for (int i = 32; i < 127; ++i) {
std::cout << static_cast<char>(i) << '\t' << i << '\n';
}
return 0;
}
What is ASCII?
ASCII, which stands for American Standard Code for Information Interchange, is a character encoding standard used for representing text in computers and other devices that use text. Developed in the early 1960s, it assigns each letter, digit, and symbol a unique number from 0 to 127. This representation is crucial for data exchange and storage, making ASCII fundamental to programming and computer science.
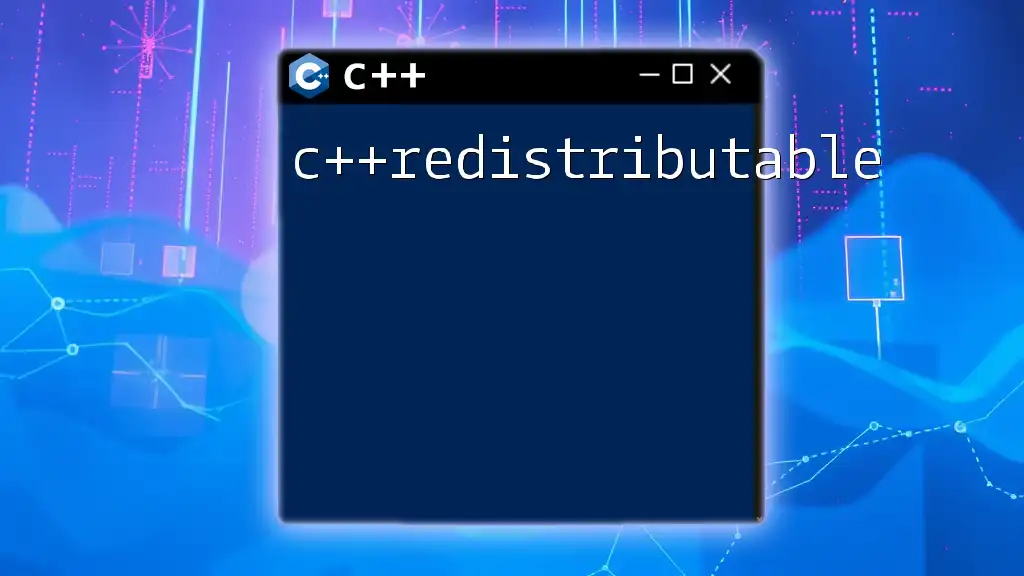
Why Use ASCII in C++?
In C++, understanding ASCII is essential for several reasons:
- Data Representation: ASCII allows programmers to easily work with character data, enabling text processing and manipulation.
- Interoperability: ASCII is widely supported, ensuring compatibility across various systems and platforms.
- Debugging: Knowing ASCII values can assist in debugging, especially when dealing with file I/O and character handling issues.
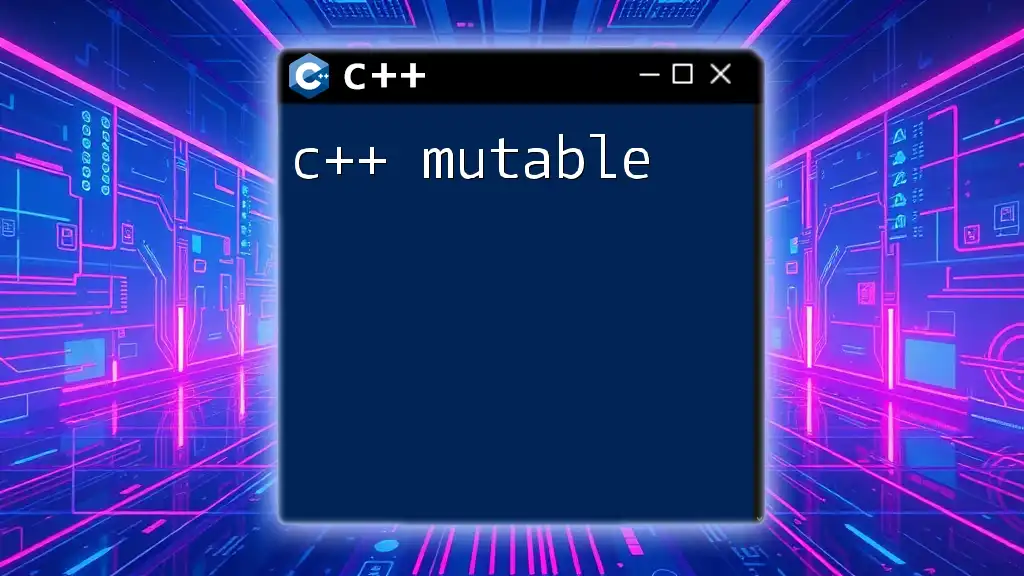
Understanding ASCII Values
Range of ASCII Values
ASCII encompasses 128 characters, including:
- Control Characters (0-31): Non-printable characters used for control in devices (like Carriage Return).
- Printable Characters (32-126): Visible characters including letters, digits, punctuation, and symbols.
For example:
- Space is ASCII 32
- A is ASCII 65
- a is ASCII 97
ASCII Character Set
The standard ASCII character set includes:
- Digits: 0-9 (ASCII 48-57)
- Uppercase Letters: A-Z (ASCII 65-90)
- Lowercase Letters: a-z (ASCII 97-122)
- Special Characters: !, @, #, $, etc. (ASCII 33-47, 58-64, 91-96, 123-126)
This comprehensive set of characters makes ASCII integral for text processing in C++.
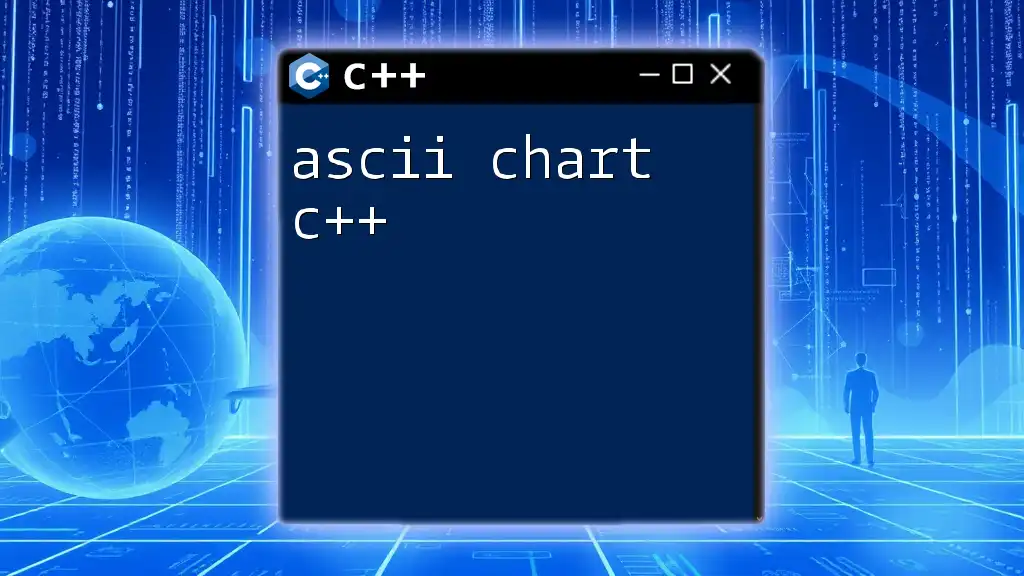
Working with ASCII in C++
Basic C++ Syntax for ASCII
C++ allows you to work with ASCII values seamlessly. You can use integer values to represent characters and vice versa. Here’s an example of how to print ASCII values:
#include <iostream>
int main() {
for (int i = 0; i < 128; i++) {
std::cout << "ASCII " << i << ": " << char(i) << std::endl;
}
return 0;
}
In this code snippet, we loop through the first 128 ASCII values and display each corresponding character. The `char(i)` converts the integer value to its corresponding ASCII character.
Manipulating ASCII Characters
Converting characters to their ASCII values is also straightforward. Here’s a simple example:
#include <iostream>
int main() {
char myChar = 'A'; // Character to be converted
int asciiValue = myChar; // Implicit conversion
std::cout << "The ASCII value of " << myChar << " is " << asciiValue << std::endl;
return 0;
}
In this example, the character 'A' is implicitly converted to its ASCII equivalent, 65. Understanding this conversion process gives programmers greater control over character handling.
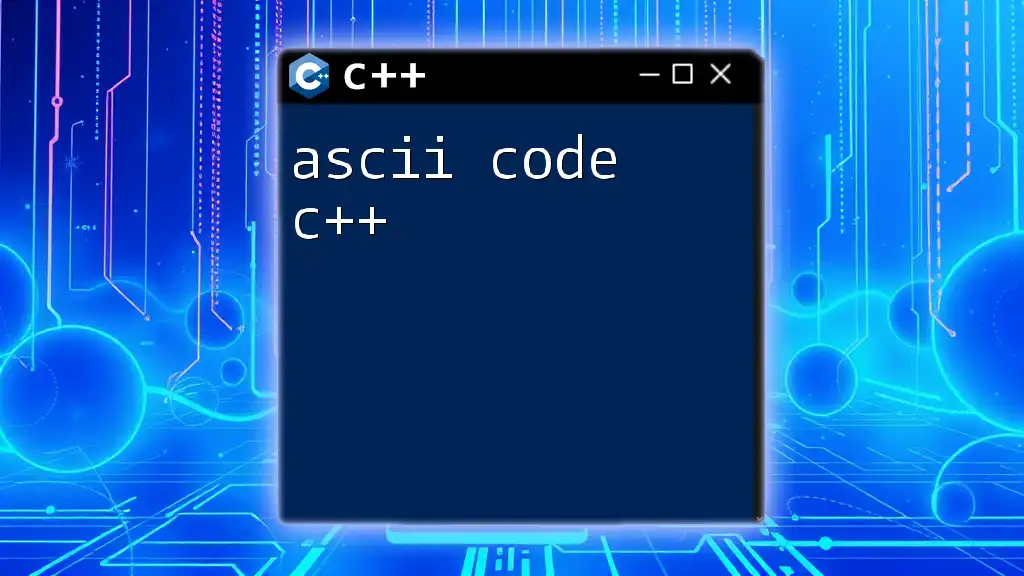
Building an ASCII Table in C++
Creating an ASCII table helps visualize the character set and their corresponding values. Here's how to construct one in C++:
#include <iostream>
#include <iomanip>
int main() {
std::cout << std::setw(10) << "ASCII" << std::setw(10) << "Character" << std::endl;
for (int i = 0; i < 128; i++) {
std::cout << std::setw(10) << i << std::setw(10) << char(i) << std::endl;
}
return 0;
}
This snippet outputs a formatted table showing ASCII values alongside their characters. The `setw()` function ensures proper alignment, enhancing readability.
Formatting and Presentation
When creating an ASCII table, clarity is paramount. Here are some tips for effective presentation:
- Alignment: Use `setw()` to align columns neatly.
- Headers: Clearly label your columns (e.g., "ASCII" and "Character") for easy reference.
- Spacing: Adequate spacing between rows prevents a cluttered appearance.
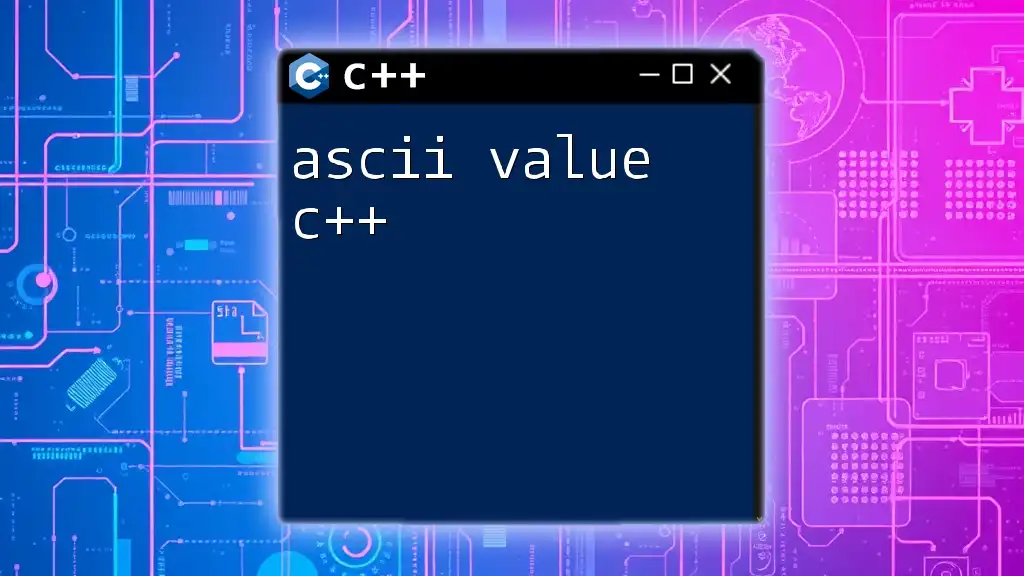
ASCII in Advanced C++ Applications
Using ASCII in Strings
ASCII significantly influences how strings are represented and manipulated in C++. For example, here’s how to extract ASCII values from a string:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
for (char c : str) {
std::cout << "Character: " << c << ", ASCII: " << int(c) << std::endl;
}
return 0;
}
This code iterates over each character in the string “Hello,” printing both the character and its ASCII value. Understanding this enables powerful string manipulation techniques.
ASCII in File Handling
File input/output operations often involve ASCII characters. Below is an example of writing ASCII values to a text file:
#include <iostream>
#include <fstream>
int main() {
std::ofstream outFile("ascii_output.txt");
for (int i = 0; i < 128; i++) {
outFile << "ASCII " << i << ": " << char(i) << std::endl;
}
outFile.close();
return 0;
}
This snippet demonstrates how to write ASCII character representations to a file, making data easy to store and retrieve.
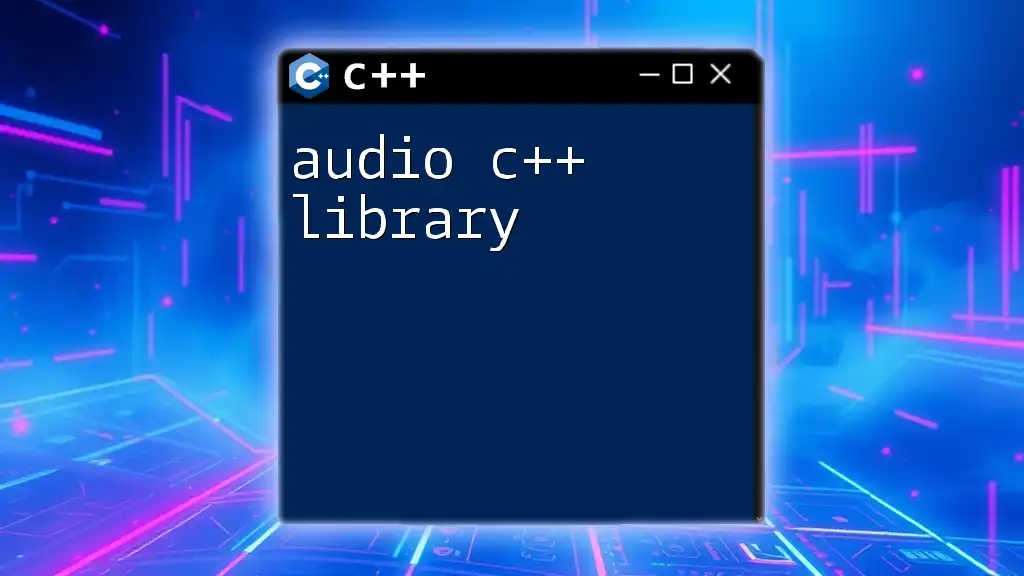
Common Issues and Troubleshooting
Encoding Issues
Sometimes, you may encounter encoding problems, especially when dealing with files created in different environments. To mitigate this:
- Use UTF-8 Encoding: Ensure that your files are saved with UTF-8 encoding to avoid compatibility issues.
- Check Locale Settings: Pay attention to locale settings in your C++ programs, as they can affect character representation.
Debugging ASCII Related Errors
Errors can arise in character handling due to misunderstanding ASCII values. Here are common pitfalls and their solutions:
- Off-by-One Errors: Ensure you are within the correct range (0-127) when accessing ASCII values to avoid unexpected behavior.
- Type Mismatches: Always ensure you correctly convert between characters and integers.
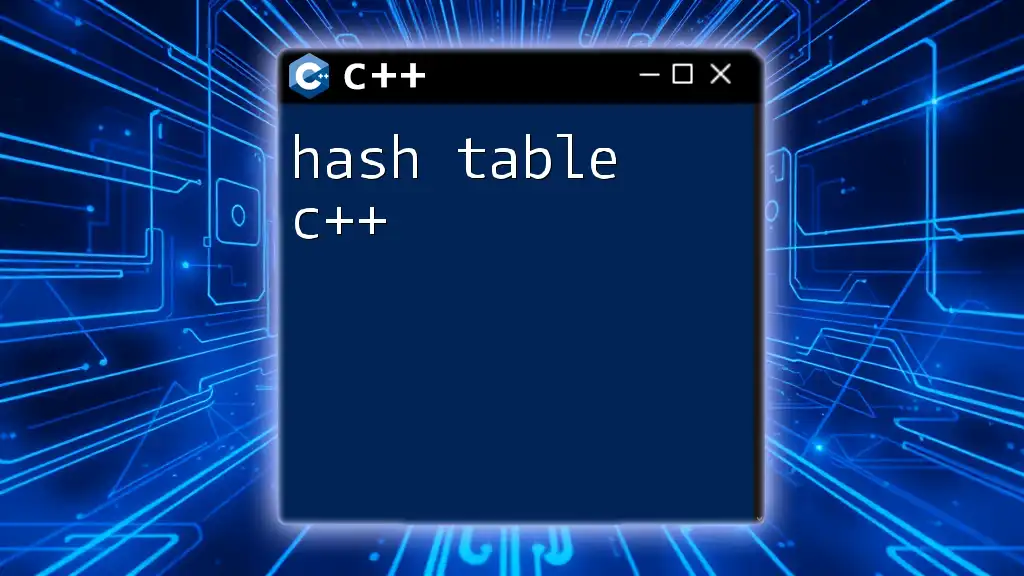
Conclusion
ASCII plays a pivotal role in C++ programming, influencing how we handle text and data. By utilizing the ASCII C++ table, developers can create, manipulate, and debug character data effectively. Understanding ASCII will undoubtedly enhance your C++ programming journey, opening up further avenues for exploration in text processing and data exchange.
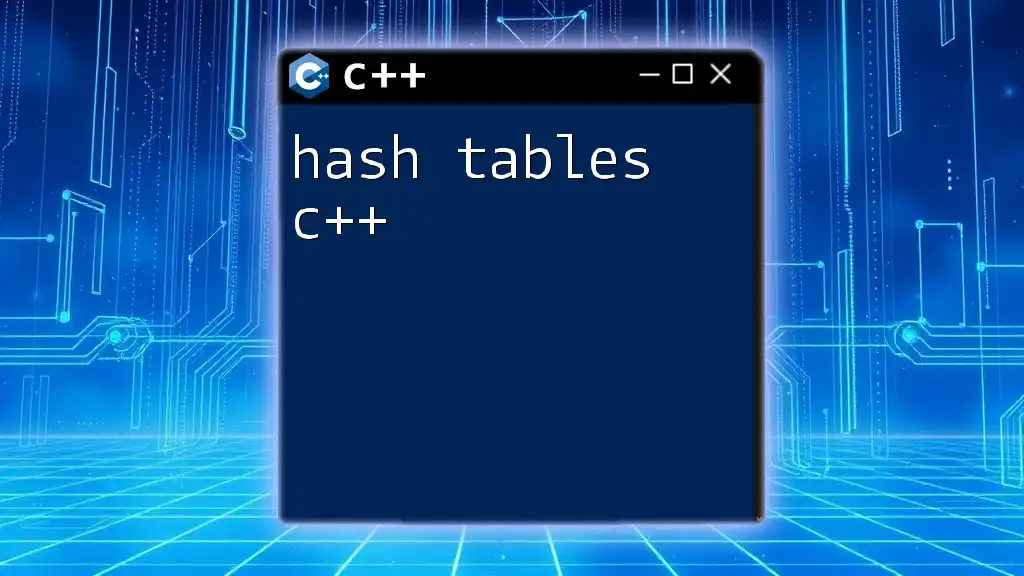
Call to Action
Engage with us! We invite you to share your own ASCII-related projects or questions in the comments. Stay tuned for more resources that can help elevate your C++ programming skills!