In C++, you can create a two-dimensional array to represent a table of rows and columns, where each element can be accessed using its corresponding indices.
#include <iostream>
int main() {
const int rows = 3;
const int cols = 4;
int table[rows][cols] = { {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} };
// Display the table
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
std::cout << table[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
Understanding Tables in C++
Definition of Tables
In programming, a table can generally be understood as a structured arrangement of data in rows and columns. This format helps developers organize information logically, making it easier to access and manipulate. When discussing C++ table rows and columns, we often refer to the various ways we can store, retrieve, and manage data in a tabular format.
The Concept of Rows and Columns
Rows and columns serve as the fundamental components of a table. Each row typically represents a record or an entry, while each column signifies an attribute or field of that entry. This organization allows for efficient data manipulation, as one can easily traverse, modify, add, or remove entries.
To use a real-life analogy, consider a spreadsheet: each row can be seen as a distinct subject (like a student), whereas columns would represent different properties of the subject (such as name, age, and grade). Having structured data in this manner not only improves readability but also enhances the efficiency of data processing.
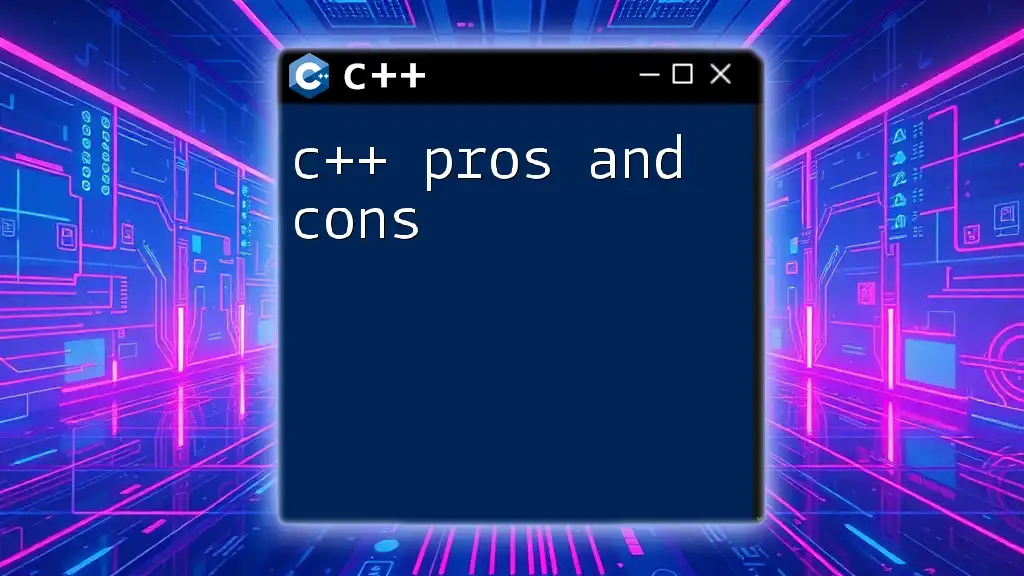
Data Structures for Managing Tables
Arrays
Arrays are one of the simplest data structures used in C++ for handling tables.
One-Dimensional Arrays
A one-dimensional array can represent a single row of data. For instance, to store student grades in a single row, you can define an array as follows:
#include <iostream>
using namespace std;
int main() {
int row[5] = {10, 20, 30, 40, 50};
for (int i = 0; i < 5; i++) {
cout << "Row Value: " << row[i] << endl;
}
return 0;
}
This simple program prints the values stored in the row array, where each element corresponds to a student's grade.
Two-Dimensional Arrays
To create a more complex table, a two-dimensional array is utilized. This structure allows for the representation of rows and columns, effectively forming a grid.
Here’s an example of a simple 2D table:
#include <iostream>
using namespace std;
int main() {
int table[2][3] = { {1, 2, 3}, {4, 5, 6} };
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
cout << table[i][j] << " ";
}
cout << endl;
}
return 0;
}
This code initializes a table with 2 rows and 3 columns, outputting the values neatly arranged like a table.
Structs and Classes
For more organized data, structs and classes can be used in C++.
Using Structs to Create a Table Row
Structs enable the grouping of related data types, making it possible to define a table-like structure. For example, if each row represents a student’s data, you could create a struct like this:
#include <iostream>
using namespace std;
struct Student {
string name;
int age;
float grade;
};
int main() {
Student row1 = {"Alice", 20, 89.5};
cout << row1.name << " " << row1.age << " " << row1.grade << endl;
return 0;
}
In this example, the Student struct holds the name, age, and grade of a student as attributes, creating a single entity or row.
Using Classes for More Complex Tables
When the requirement grows complex, using classes can provide significant benefits, such as encapsulation and methods for managing data. Here’s how a class can be employed to manage a dynamic 2D table:
#include <iostream>
#include <vector>
using namespace std;
class Table {
public:
vector<vector<int>> data;
void addRow(const vector<int>& row) {
data.push_back(row);
}
void display() {
for (const auto& row : data) {
for (const auto& item : row) {
cout << item << " ";
}
cout << endl;
}
}
};
int main() {
Table myTable;
myTable.addRow({1, 2, 3});
myTable.addRow({4, 5, 6});
myTable.display();
return 0;
}
This class manages a table of integers, allowing for dynamic addition of rows and the ability to display the entire table.
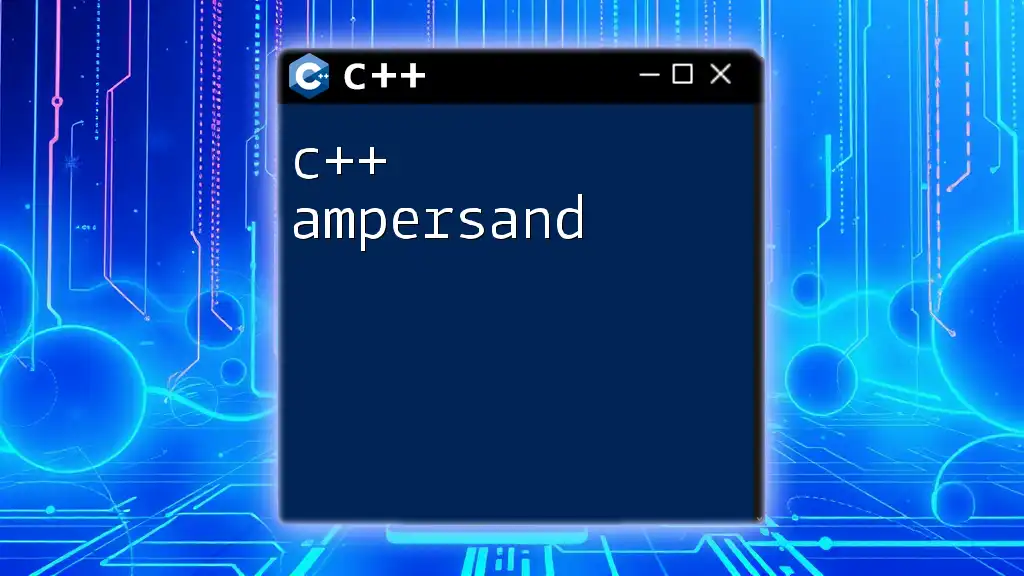
Common Operations on Tables
Inserting Data
Inserting new rows or columns into your table can be straightforward. For example, when managing students, adding a new row with their information is simply a matter of calling an insertion method.
Accessing Data
Retrieving data from specific cells in your table is an essential skill. You can access values using their respective indices, similar to arrays:
int grade = table[0][1]; // Accessing the second element in the first row
Deleting Data
Removing entries from a table is also a core operation. You can delete a specific row by adjusting the underlying data structure, noting that this may involve shifting data around.
Modifying Data
Updating values in your data structure is a constant necessity. Using the same access pattern, you can easily assign a new value to an existing element:
table[1][0] = 10; // Change the value in the second row, first column
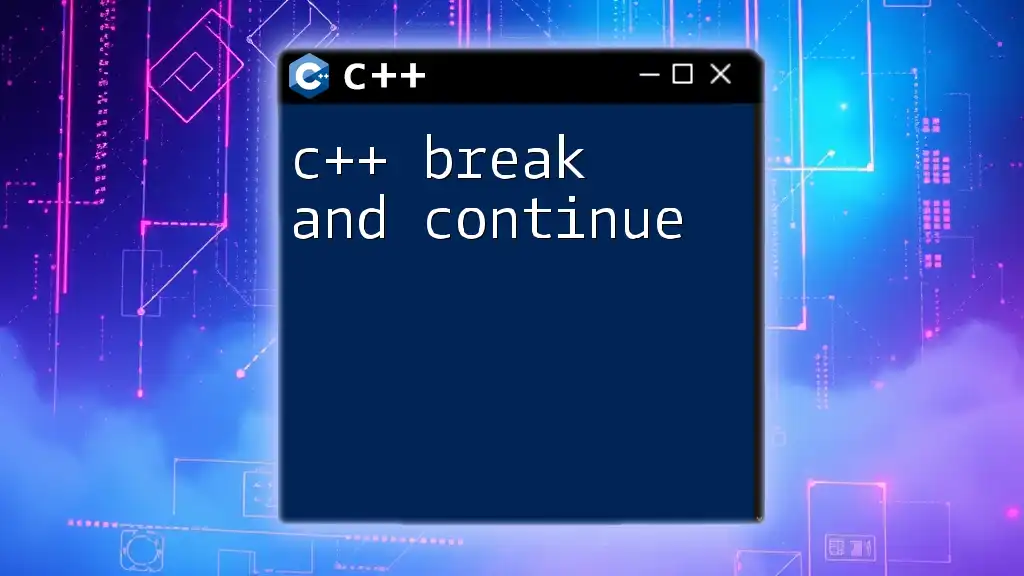
Dynamic vs Static Tables
Static Tables
Static tables are defined with a fixed size at compile time. While easy to implement, they have clear limitations, particularly in flexibility and scalability. If you need to add or remove data frequently, static tables might not be appropriate.
Dynamic Tables
Dynamic tables, often implemented with the use of std::vector in C++, offer a powerful alternative. They allow for resizing and provide greater flexibility:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<vector<int>> dynamicTable;
dynamicTable.push_back({1, 2, 3});
dynamicTable.push_back({4, 5}); // Table automatically resizes
for (const auto& row : dynamicTable) {
for (const auto& item : row) {
cout << item << " ";
}
cout << endl;
}
return 0;
}
In this example, adding rows dynamically adjusts the size of the table, making it adaptable to changing needs.
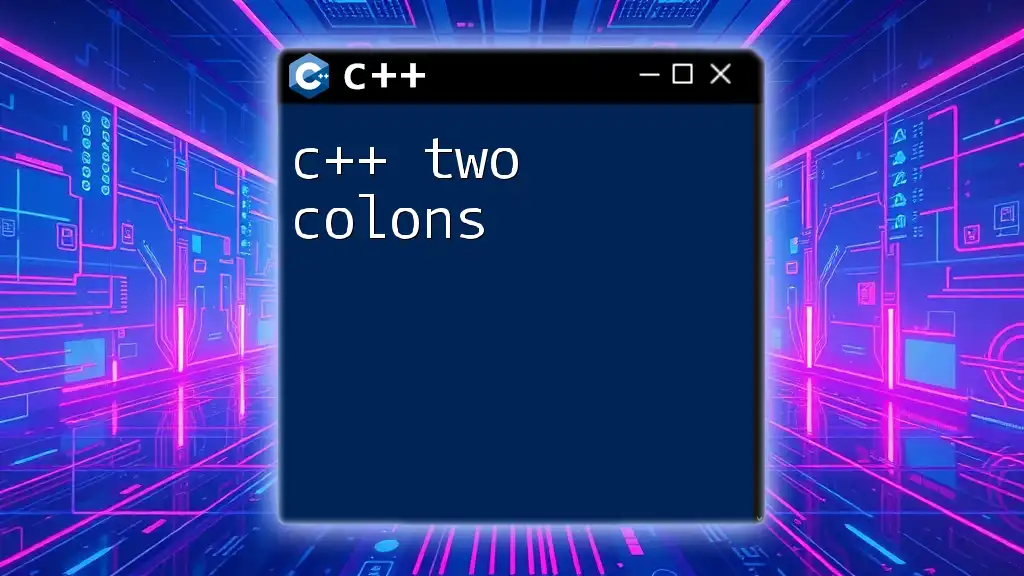
Conclusion
Understanding how to effectively manage C++ table rows and columns is crucial for any developer looking to manipulate data efficiently. By utilizing arrays, structs, and classes, you can create organized data structures that simplify both the coding process and data management. Remember to choose between static and dynamic structures based on your application’s needs and complexity.
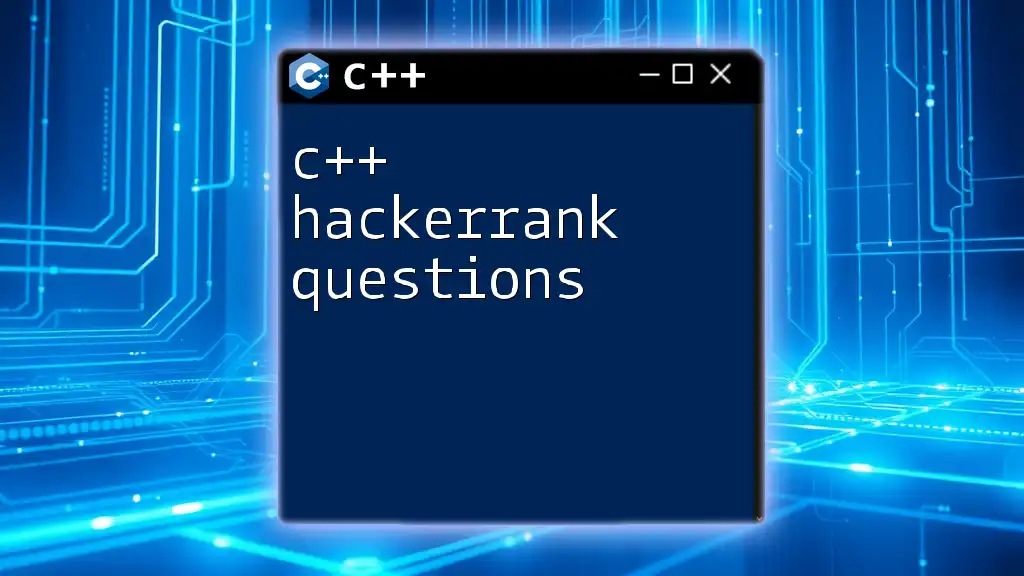
Additional Resources
To further your learning and refine your skills in handling C++ tables, consider exploring the official C++ documentation on arrays, structs, and classes, along with other recommended tutorials and textbooks that delve deeper into advanced data manipulation techniques.