In C++, the two colons `::` are known as the scope resolution operator, used to define the context in which an identifier (such as a variable or function) is defined, allowing access to class members and global variables.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
class MyClass {
public:
static void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
int main() {
MyClass::display(); // Using the scope resolution operator
return 0;
}
What are C++ Two Colons?
The double colon `::` operator is a powerful feature within C++ that serves multiple purposes, each critical for effective programming. It primarily allows programmers to specify the scope in which a variable or function resides, thus providing clarity and control in your code.
Understanding the Syntax
In C++, the double colon is fundamentally a scope resolution operator. It enables programmers to distinctly specify where a variable or function is defined, whether it be within a class, namespace, or even within a global context. This specificity helps in organizing code and prevents naming collisions, which are common in larger programs.
Significance of the Double Colon
The double colon is vital in differentiating between various scopes in your program. Without this operator, one could easily run into conflicts, especially when dealing with multiple namespaces or inherited classes. By employing the `::` operator, you can effectively manage where your functions and variables are accessed from, making your code much more maintainable and understandable.
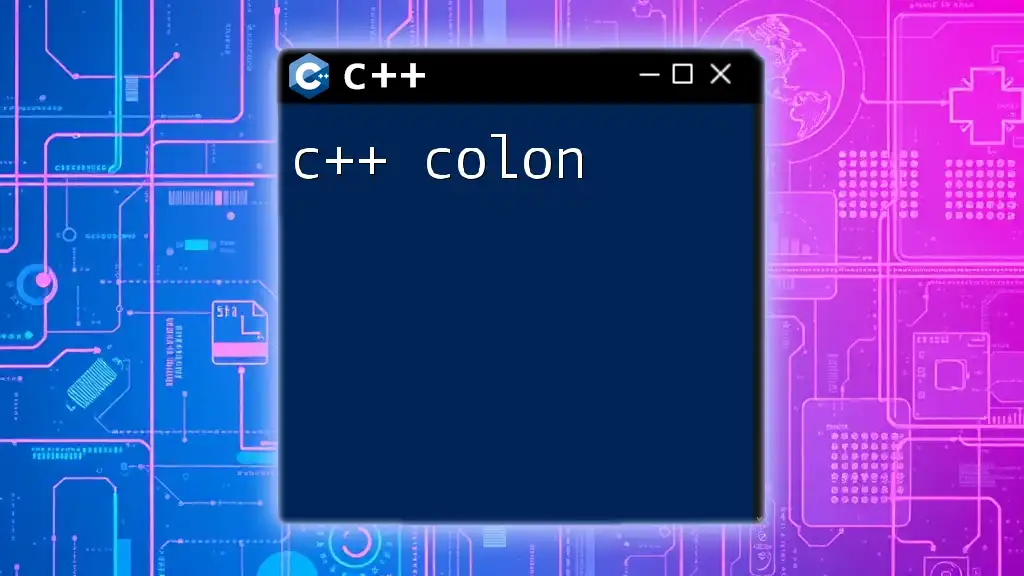
The Scope Resolution Operator
Explanation of the Scope Resolution in C++
In C++, scope resolution is the process of determining which variable or function to use when multiple definitions exist. This is particularly useful in larger projects where multiple files may define the same variable. The scope resolution operator helps clarify which definition is intended to be used.
Using the Scope Resolution Operator
Syntax: The general syntax for the scope resolution operator looks like this: `namespace::name`, `ClassName::methodName`, or simply `::variableName`.
Example #1: Accessing a variable within a namespace:
namespace MyNamespace {
int myVariable = 42;
}
int main() {
std::cout << MyNamespace::myVariable; // Output: 42
return 0;
}
In the example above, the variable `myVariable` is defined within `MyNamespace`. By using `MyNamespace::myVariable`, we avoid any potential naming conflicts with other variables in our program.
Example #2: Class members and functions:
class MyClass {
public:
static int myStaticVar;
void show() {
std::cout << "Showing MyClass" << std::endl;
}
};
int MyClass::myStaticVar = 100;
int main() {
MyClass obj;
obj.show(); // Output: Showing MyClass
std::cout << MyClass::myStaticVar; // Output: 100
return 0;
}
Here, `myStaticVar` is explicitly accessed via the class name, showcasing how double colons can help access static members from a class.
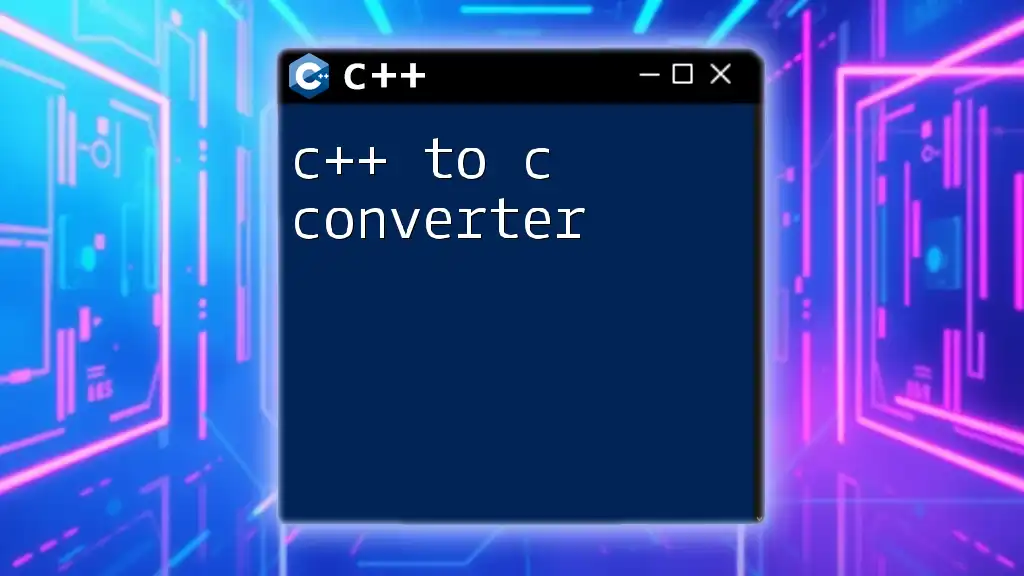
Double Colon in Inheritance
How the Scope Resolution Operator is used in Inheritance
Inheritance allows a class to derive properties and behaviors from another class. The double colon plays a crucial role in specifying which features belong to the base class versus those in the derived class.
Example illustrating base class scope resolution:
class Base {
public:
void greet() {
std::cout << "Hello from Base" << std::endl;
}
};
class Derived : public Base {
public:
void greetDerived() {
std::cout << "Hello from Derived" << std::endl;
}
};
int main() {
Derived obj;
obj.greet(); // Accessing Base class method
obj.greetDerived(); // Accessing Derived class method
return 0;
}
In this example, the derived class `Derived` inherits from `Base`, and we can call methods from both classes seamlessly, showcasing the utility of the double colon in inheritance.
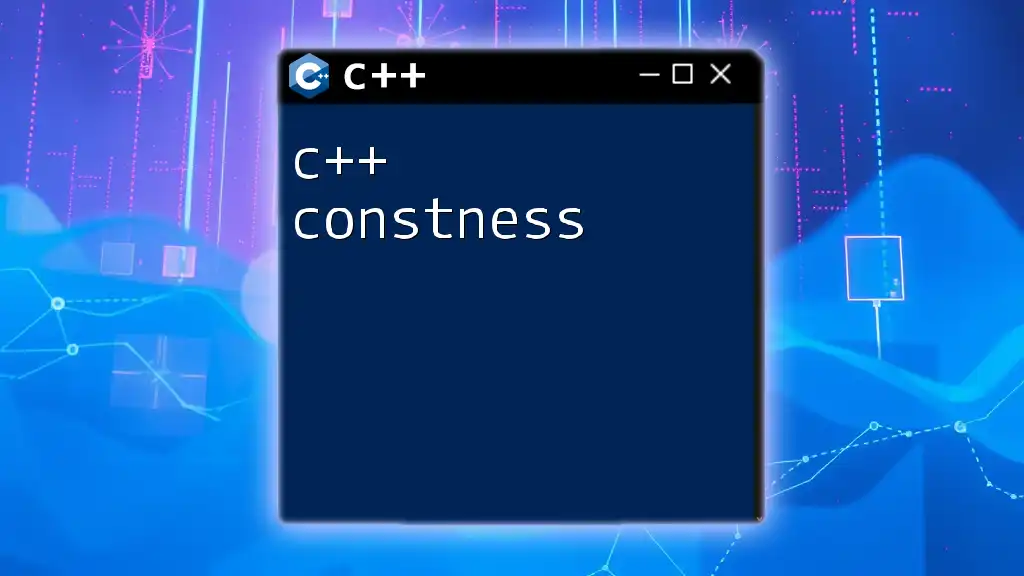
Using the Outer Class Scope
The Role of Double Colon in Nested Classes
Nested classes are classes defined within the body of another class. The double colon is vital for accessing methods and members from these nested structures.
Example of using the scope resolution operator with nested classes:
class Outer {
public:
class Inner {
public:
void display() {
std::cout << "Inside Inner Class" << std::endl;
}
};
};
int main() {
Outer::Inner obj; // Accessing nested class
obj.display(); // Output: Inside Inner Class
return 0;
}
In the above code, the `Inner` class is nested within the `Outer` class. The double colon is used to instantiate an object of `Inner`, ensuring clarity in how we access class features.
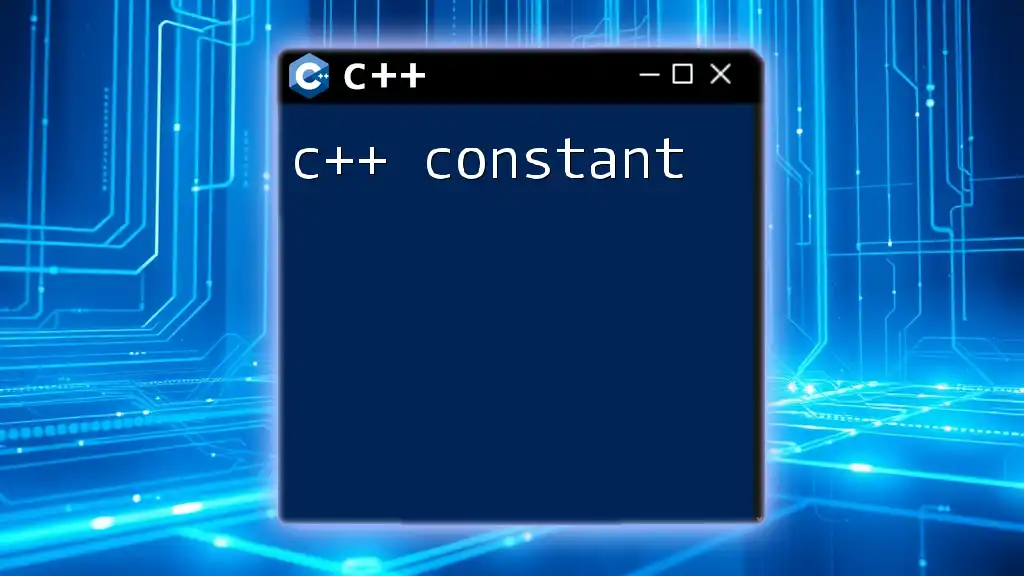
Accessing Global Variables
How to Use Double Colons to Access Global Variables
The double colon operator can also be employed to resolve the scope when dealing with global variables, especially when local variables carry the same name.
Example demonstrating access to global variables:
int globalVar = 10;
int main() {
int globalVar = 20; // local variable
std::cout << ::globalVar; // Accessing global variable
return 0;
}
In this example, the local variable `globalVar` shadows the global variable of the same name. Utilizing `::globalVar` allows us to specify that we want to access the global version.
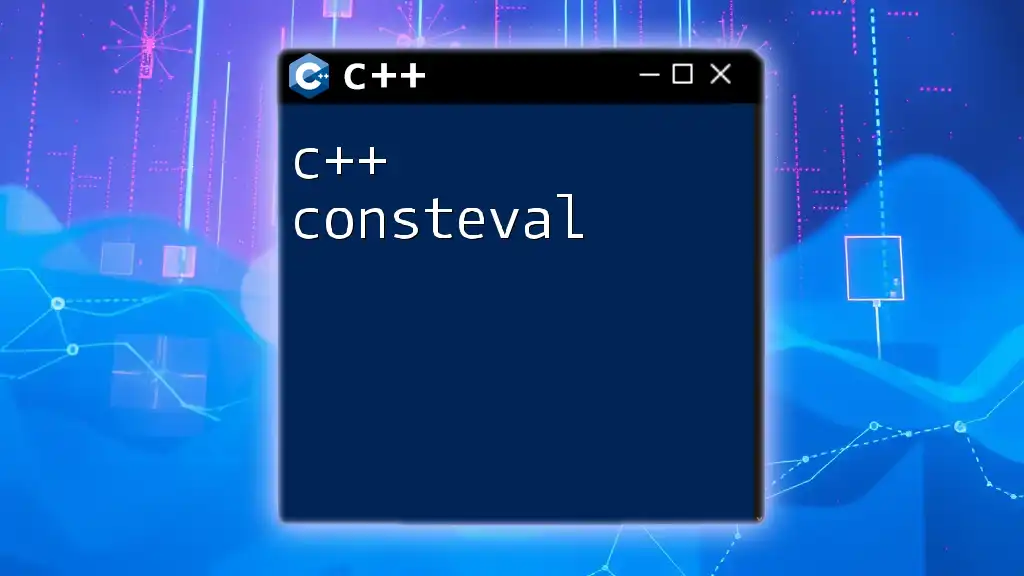
Namespace Management
The Importance of Namespaces in C++
Namespaces help avoid naming conflicts by organizing code into logical groups. The double colon operator is essential for properly referencing variables and functions within these namespaces.
Practical Examples of Namespaces
namespace First {
void func() {
std::cout << "In First Namespace" << std::endl;
}
}
namespace Second {
void func() {
std::cout << "In Second Namespace" << std::endl;
}
}
int main() {
First::func(); // Output: In First Namespace
Second::func(); // Output: In Second Namespace
return 0;
}
This example highlights how the double colon distinguishes between two functions named `func` residing in different namespaces.
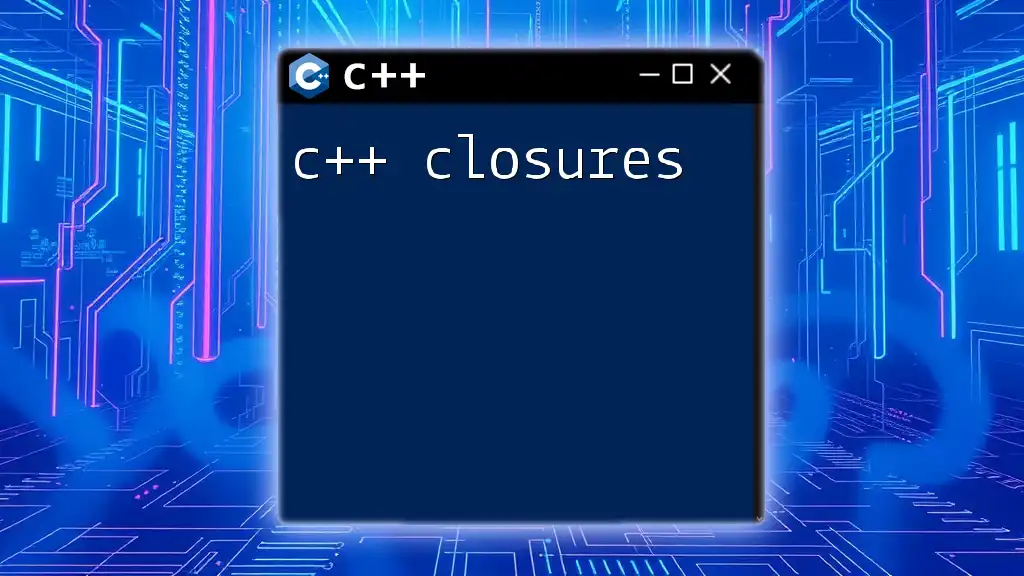
Best Practices Using the C++ Double Colon
Tips for Effective Use of the Double Colon
To fully leverage the power of the two colons, consider the following best practices:
-
Clarity: Always use the double colon to specify scope clearly. This minimizes confusion and helps in maintaining code comprehensibility.
-
Consistency: Stick to a pattern in your naming conventions to avoid potential collisions, especially in large-scale projects.
Code Organization
Organizing your code with a clear structure encourages better collaboration and code quality. Use namespaces liberally to group related functionalities, and make good use of the double colon to access these groupings easily.
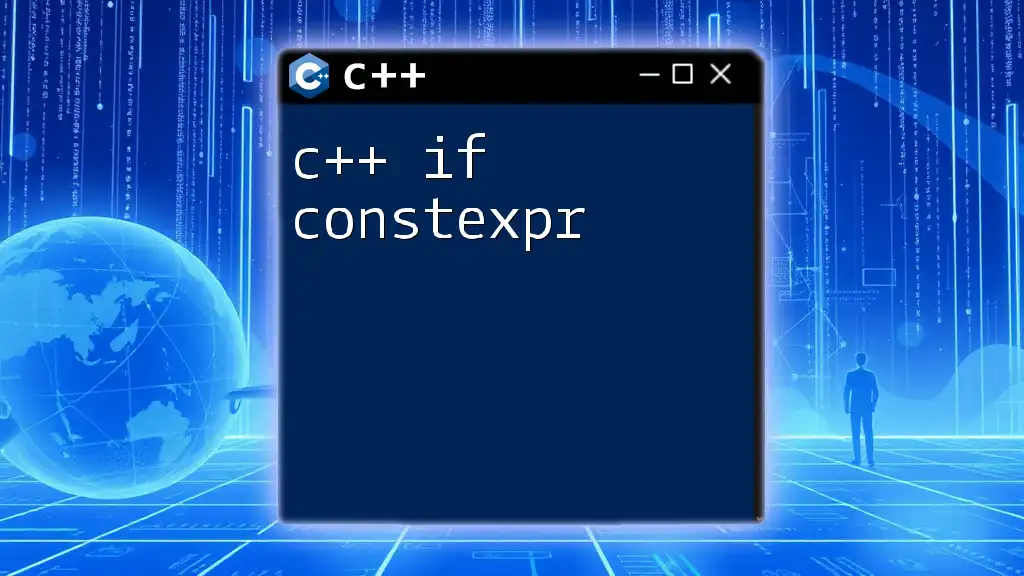
Conclusion
Understanding how to work with C++ two colons is essential for any C++ programmer. By mastering the scope resolution operator, programmers can write more effective, organized, and maintainable code. Focus on practicing with various examples and use the double colon thoughtfully to manage how you access variables and functions in your applications. Happy coding!
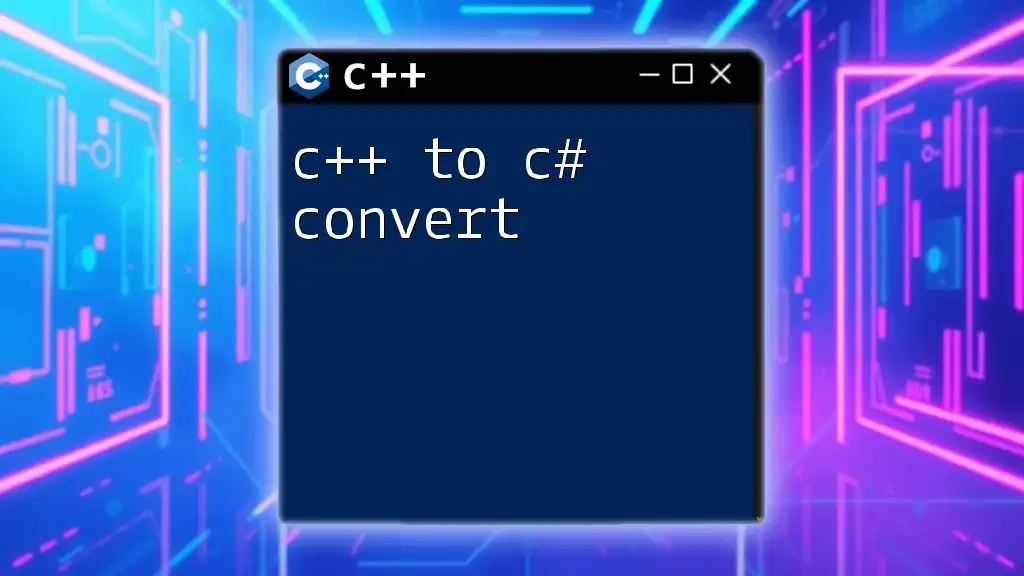
Additional Resources
For further reading, consider exploring additional resources and engaging in community forums to connect with other developers who share an interest in C++. These avenues can provide valuable insights and opportunities for learning and growth in your programming journey.