The `if constexpr` statement in C++ allows for compile-time conditional branching, enabling the compiler to eliminate branches of code that are never used, which is especially useful in templated programming.
Here’s a code snippet demonstrating its use:
#include <iostream>
#include <type_traits>
template<typename T>
void printType(T value) {
if constexpr (std::is_integral_v<T>) {
std::cout << value << " is an integer." << std::endl;
} else {
std::cout << value << " is not an integer." << std::endl;
}
}
int main() {
printType(42); // Output: 42 is an integer.
printType(3.14); // Output: 3.14 is not an integer.
return 0;
}
Understanding `if constexpr`
What is `if constexpr`?
`if constexpr` is a feature introduced in C++17 that allows for compile-time conditional statements. Unlike traditional `if` statements that evaluate conditions at run-time, `if constexpr` evaluates the condition during compilation. This means that certain branches of your code might never exist in the final binary if their conditions aren't met, leading to potentially safer and more efficient code.
Purpose of `if constexpr`
The primary purpose of `if constexpr` is to facilitate compile-time decision making. This is particularly useful in the context of template metaprogramming, where you may need to execute different logic based on the type passed as a template parameter. By separating compile-time logic from run-time checks, you can improve both performance and readability of your C++ code.
Syntax of `if constexpr`
The syntax is straightforward and closely resembles that of a traditional `if` statement:
if constexpr (condition) {
// code to execute if condition is true
} else {
// code to execute if condition is false
}
This clear structure allows you to maintain readability while adding the benefits of compile-time evaluations.
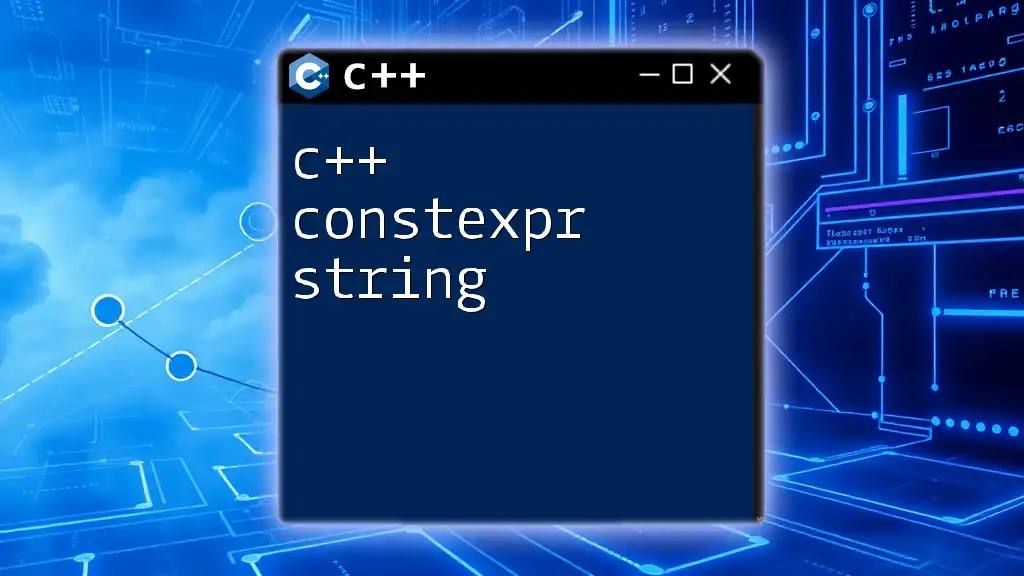
When to Use `if constexpr`
Template Specialization
Template specialization is a powerful feature in C++ that allows specialization of templates based on the type parameters. `if constexpr` can significantly simplify this process. Instead of creating multiple template specializations, you can use a single template and employ `if constexpr` to handle various types differently.
Performance Optimization
Using `if constexpr` can lead to performance optimizations. Since the compiler can discard branches that are not applicable, this prevents unnecessary code from being generated. By reducing the code instantiation at compile time, it ultimately decreases compile times and potentially runtime overhead.
Examples of Usage
Example 1: Basic Condition
Below is a simple example that demonstrates how `if constexpr` can be used to differentiate between integral and non-integral types:
template<typename T>
void checkType(T value) {
if constexpr (std::is_integral_v<T>) {
std::cout << "Integral type." << std::endl;
} else {
std::cout << "Non-integral type." << std::endl;
}
}
In this example, if `T` is an integral type, the message "Integral type." will be printed. If not, "Non-integral type." is executed. The compile-time evaluation ensures that the code for the non-matching case isn't included in the final binary when `T` is an integral type.
Example 2: Complex Conditions
You can also branch based on multiple conditions using `if constexpr`. Here’s how you handle more complex checks:
template<typename T>
void process(T value) {
if constexpr (std::is_same_v<T, std::string>) {
std::cout << "You provided a string." << std::endl;
// Handle string processing
} else if constexpr (std::is_integral_v<T>) {
std::cout << "You provided an integral type." << std::endl;
// Handle integral processing
} else {
std::cout << "Default case for other types." << std::endl;
// Handle default case
}
}
In this expanded example, we have three separate code paths based on the type of `T`. This flexibility greatly enhances code clarity while ensuring that only the necessary code is compiled.
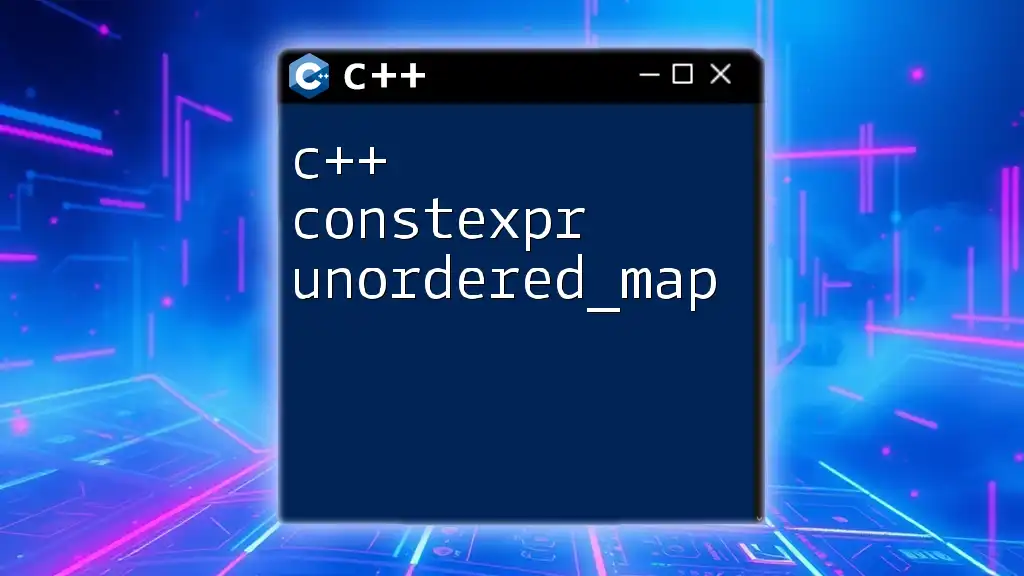
Advantages of Using `if constexpr`
Enhanced Readability
One of the standout benefits of `if constexpr` is improved readability. Instead of scattering separate template specializations throughout your code, you can write a single function that is easier to follow. This leads to code that is more maintainable and easier for others (or yourself in the future) to understand.
Reduced Compilation Time
Reducing compile-time errors is another significant advantage. Because conditions are evaluated at compile time, unnecessary branches that would produce errors won't be included during compilation. This minimizes the risk of encountering template instantiation errors, thus streamlining the development process.
Type Safety
The use of `if constexpr` allows for stronger type safety. Since the code that's invalid for a type won't even be compiled, you avoid common pitfalls associated with template programming, leading to fewer run-time errors.
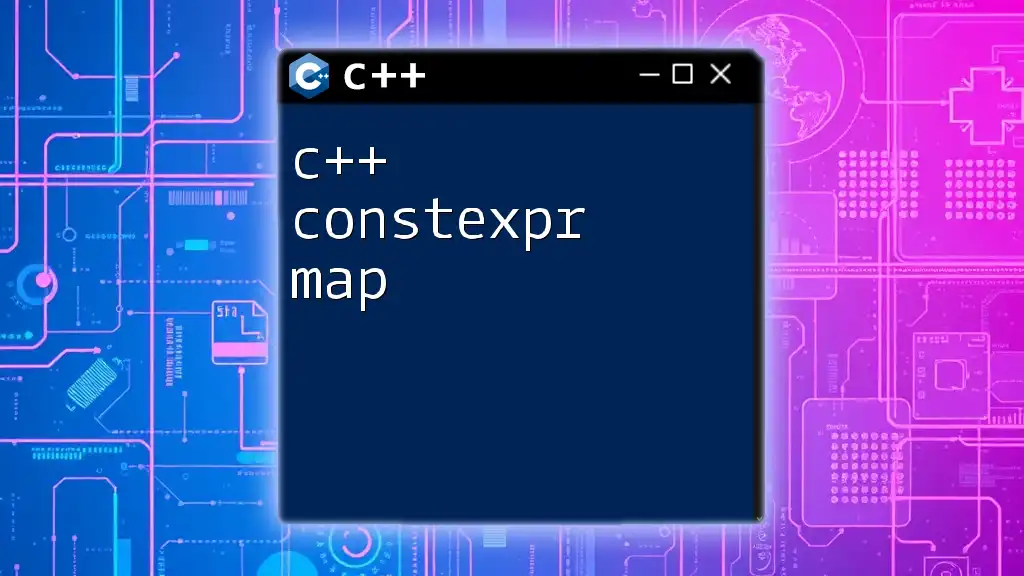
Common Pitfalls
Misuse of `if constexpr`
Despite its advantages, developers should be cautious not to overuse `if constexpr` inappropriately. Using it excessively can lead to code that's more complex than necessary. It's crucial to strike a balance between clarity and compactness in your code.
Confusion with Runtime `if` Statements
Many newcomers might confuse `if constexpr` with traditional run-time `if` statements. While `if` checks conditions at run-time, `if constexpr` is strictly evaluated during compilation. Understanding this difference is critical for leveraging the power of `if constexpr` effectively.
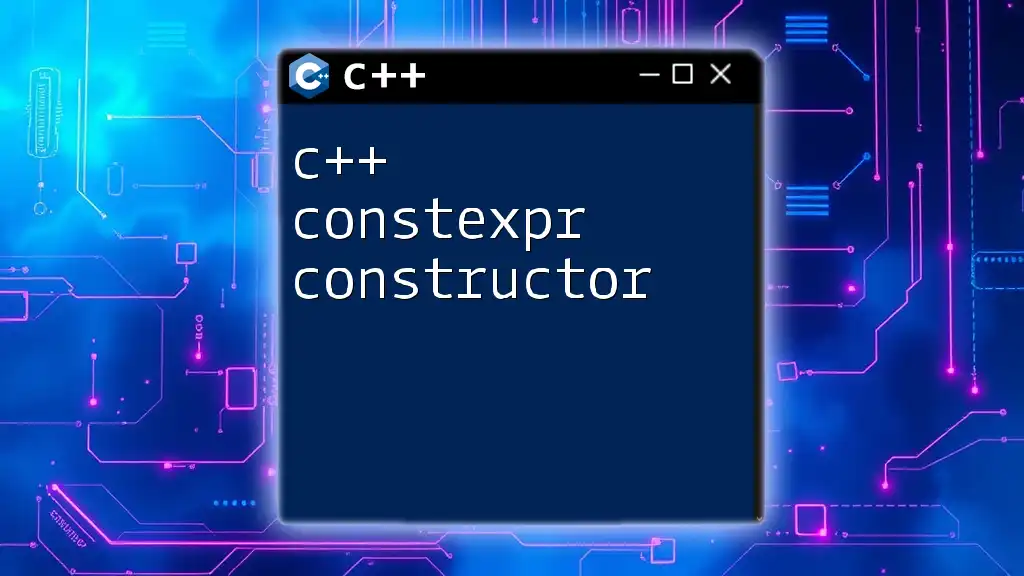
Real-World Applications
Libraries Utilizing `if constexpr`
Several C++ libraries, especially modern ones, have begun integrating `if constexpr` for enhanced type handling and generic programming. Libraries such as Boost and STL showcase examples of template metaprogramming where `if constexpr` simplifies the complexity.
Case Study: A C++ Template Library
Consider a hypothetical scenario with a C++ template library designed for mathematical operations. This library could implement various algorithms for different numeric types. Instead of specializing each operation for each numeric type, `if constexpr` could be employed, significantly reducing code duplication and improving maintainability.
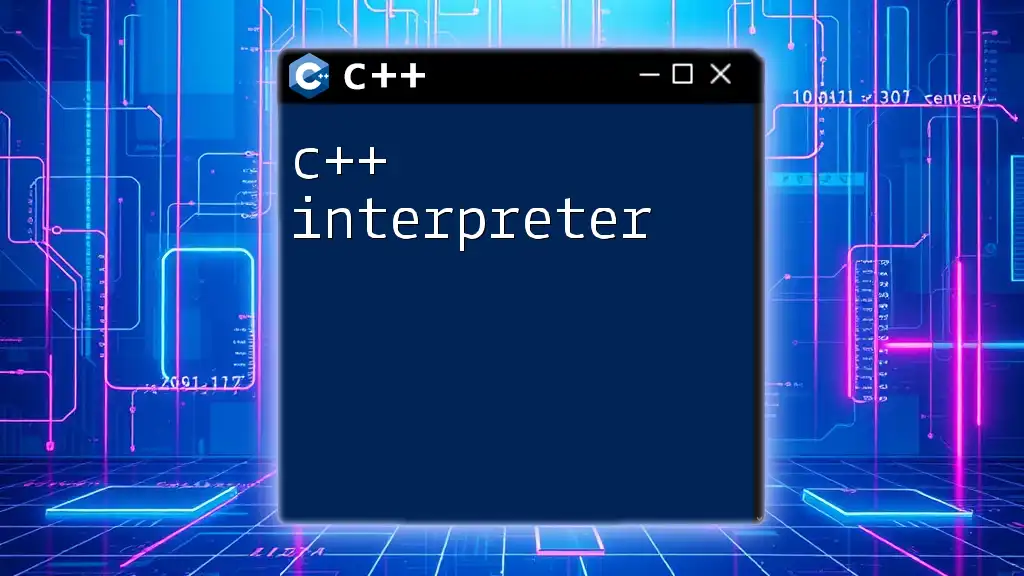
Conclusion
The introduction of `if constexpr` in C++17 offers a compelling tool for developers aiming to write clean, efficient, and type-safe code. Its ability to evaluate conditions at compile time not only enhances performance but also makes the code easier to read and maintain. For anyone working with C++, mastering the use of `if constexpr` is highly beneficial.
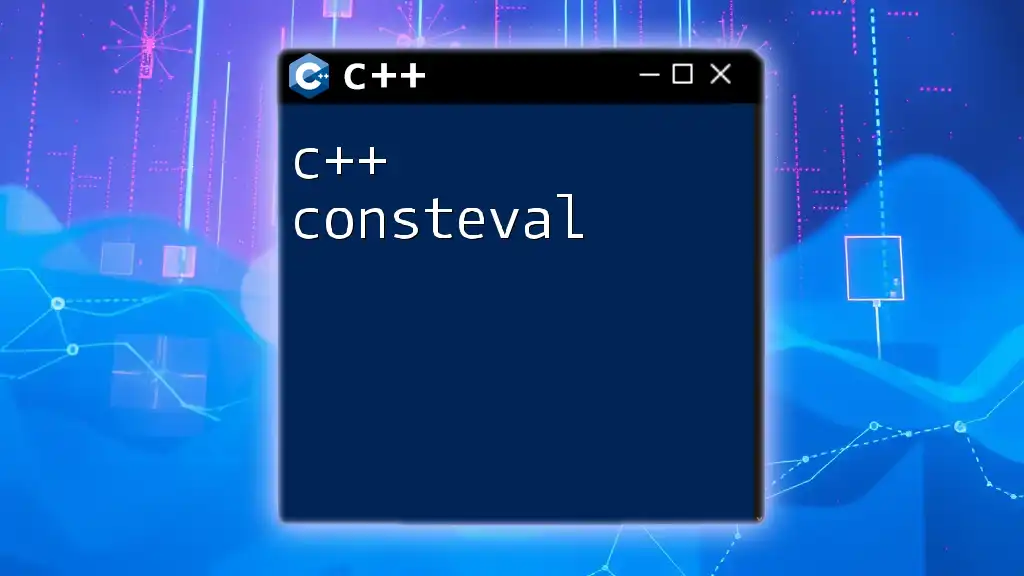
Further Reading and Resources
Explore the official C++ documentation and established C++ programming books that delve deeper into advanced features like `if constexpr`. Consider online resources as well that provide hands-on tutorials on template programming and metaprogramming.
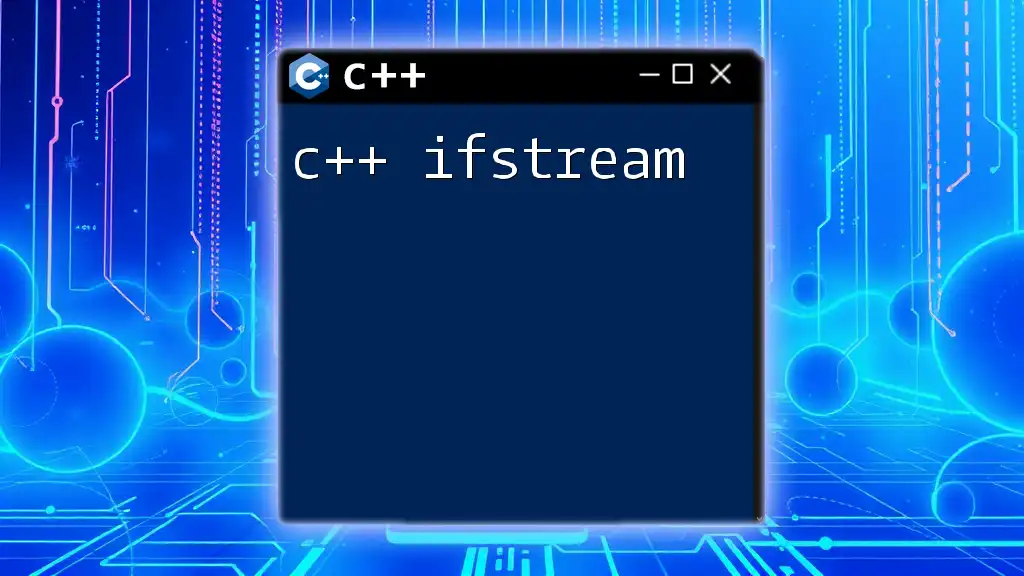
Call to Action
We invite you to share your insights or examples of using `if constexpr` in your projects. Follow our company for more engaging content on C++ commands and best practices. Your experience can help others in the community grow and excel!