C++ constness refers to the use of the `const` qualifier to indicate that a variable's value cannot be changed after its initialization, thereby enhancing code safety and clarity.
Here’s a code snippet demonstrating the usage of `const` in C++:
#include <iostream>
void printValue(const int num) {
// num cannot be modified due to constness
std::cout << "The value is: " << num << std::endl;
}
int main() {
const int myValue = 10;
printValue(myValue);
// myValue = 20; // This line would cause a compile-time error
return 0;
}
Understanding the Concept of Constness
What is const?
In C++, the `const` keyword is a powerful feature that indicates that a variable's value cannot be modified after it has been initialized. Declaring a variable as `const` ensures that any attempts to change its value will result in a compile-time error, thus preventing unintended modifications.
For example, when you create a constant variable:
const int MAX_VALUE = 100;
Here, `MAX_VALUE` is a constant and trying to do something like `MAX_VALUE = 200;` would lead to a compilation error. This allows you to enforce immutability in your code, significantly reducing the potential for bugs.
Why Use Const?
Using `const` in your code offers several critical benefits:
-
Preventing Accidental Modification: By marking variables as `const`, you protect them from unintentional changes throughout your program. This makes your code safer and eases debugging.
-
Enhancing Function Performance: Declaring parameters as `const` allows the compiler to optimize the code better, leading to potential performance improvements.
-
Improving Code Readability and Maintainability: It serves as a promise to the reader of the code. When they see `const`, they immediately know that the value will not change, which improves the understanding and clarity of your codebase.
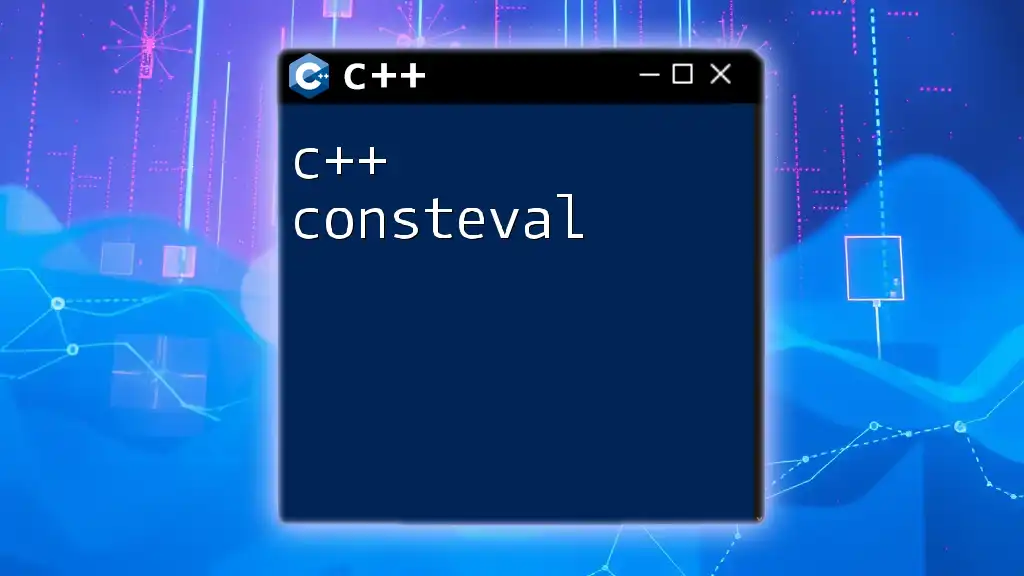
Different Forms of Constness in C++
Const Variables
Definition and Usage:
Constant variables play a pivotal role in a well-structured code. By declaring variables with `const`, you define them as immutable:
const int MAX_VALUE = 100;
The compiler enforces the constness, meaning any attempt to modify `MAX_VALUE` later in the code will result in an error.
Effects on Variables:
If you try to change a const variable, such as:
MAX_VALUE = 200; // This will raise a compilation error
This error occurs because the compiler knows that `MAX_VALUE` is not supposed to change.
Const Pointers
In C++, pointers can also be marked as `const`, resulting in different constness behaviors:
-
Pointer to const: This type of pointer allows you to point to a variable that should not be modified.
const int* ptr = &MAX_VALUE;
Here, `ptr` can be changed to point to another address, but the value it points to cannot be altered.
-
Const pointer: A constant pointer can only point to the memory address it was assigned initially, but the value it points to can be changed.
int* const ptr1 = &MY_VALUE;
`ptr1` must always point to `MY_VALUE`, but you can modify `MY_VALUE`.
-
Const pointer to const: This combination does not allow modifications to either the pointer itself or the value at the address it points to.
const int* const ptr2 = &MY_CONST_VALUE;
This is useful when you want to encapsulate both a variable and the pointer in a safe way, minimizing accidental changes.
Const Member Functions
Definition and Purpose:
In object-oriented programming, declaring member functions as `const` can be incredibly useful. A const member function promises not to modify the object on which it operates.
class MyClass {
void myFunction() const; // Declaration of a const member function
};
This is essential when implementing methods that shouldn't affect the object's state.
When to Use Const Member Functions:
By using const member functions, you indicate to other developers (and the compiler) that these functions won’t change any member variables of the class, which leads to clearer logic and expected behavior.
Const with Reference Parameters
Using const with reference parameters enhances performance and safety. Passing by reference avoids the overhead of copying large objects while preventing modification of the original object.
Example:
void myFunction(const MyClass& obj);
In this case, `obj` can be utilized as if it were a normal object, but any attempt to modify `obj` will result in a compilation error. This not only speeds up the code but also protects the integrity of the original object.
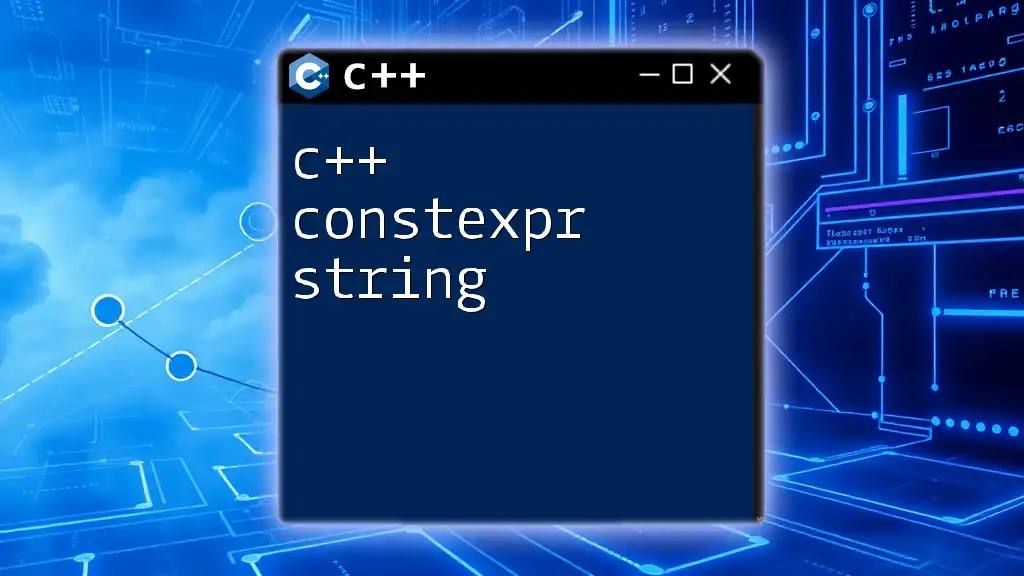
Best Practices for Using Constness
General Guidelines
- Declare variables and constants as const whenever possible. If their values don't change, they should be marked as const.
- Use const in function signatures for parameters that won’t be modified. This clarifies intent and aids compiler optimization.
Using Const for Function Parameters
It's essential to understand the performance implications between passing by value and passing by const reference. When you pass by value, a copy is created, which can be costly for large objects. However, passing by const reference avoids this overhead:
void passByValue(MyClass obj);
void passByConstReference(const MyClass& obj);
In practice, always favor passing by const reference for large classes or structures to avoid unnecessary overhead.
Avoiding Pitfalls
Preventing mistakes related to constness is vital for maintaining code reliability. Common missteps include:
-
Modifying const objects. Attempting to change a const variable or call a non-const member function on a const instance will lead to compilation errors, ensuring immutability.
-
Incorrect usage of const pointers. Always double-check the type of pointer you declare to make sure it aligns with your intent, as misunderstandings can lead to confusion or errors.
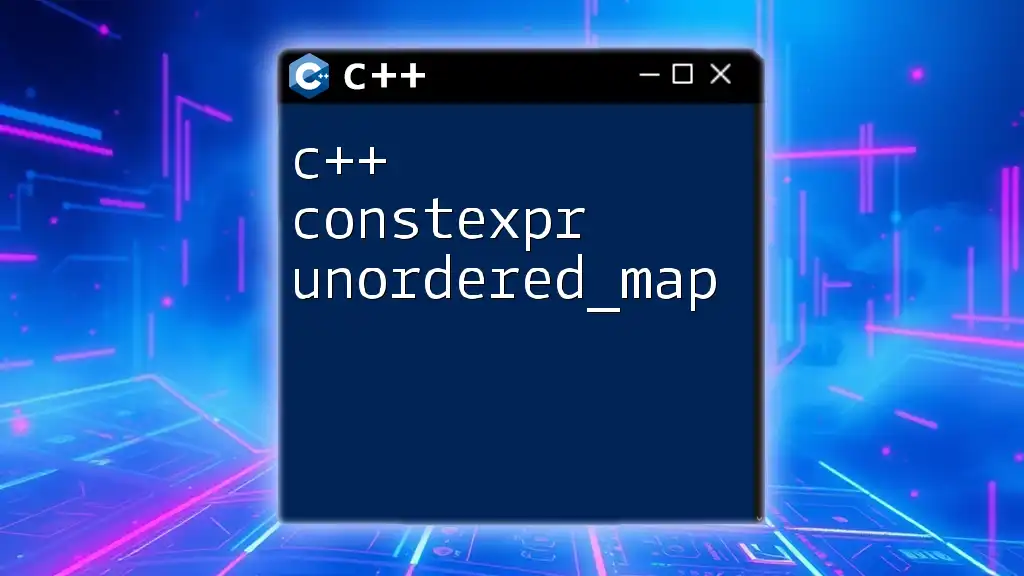
Advanced Constness Concepts
Constexpr
Introduction to Constexpr:
`constexpr` extends the flexibility of `const` by allowing you to declare variables, functions, and expressions that can be evaluated at compile time. This significantly optimizes performance as it enables the compiler to perform computations during compilation rather than runtime.
Example of Constexpr:
constexpr int factorial(int n) { return (n <= 1) ? 1 : n * factorial(n - 1); }
The `factorial` function can be used in contexts requiring a constant, such as array sizes, thereby enhancing efficiency.
Constness in Templates
When working with template functions, understanding constness becomes even more critical. The constness of template parameters allows for both flexibility and safety by ensuring that objects passed to templates can remain unchanged if needed.
Code Example Illustrating Templates with Const Types:
template <typename T>
void func(const T& value) {
// Operations that don't modify value
}
Using const with templates ensures that various types can be processed without the risk of modification, maintaining consistent behavior across different contexts.
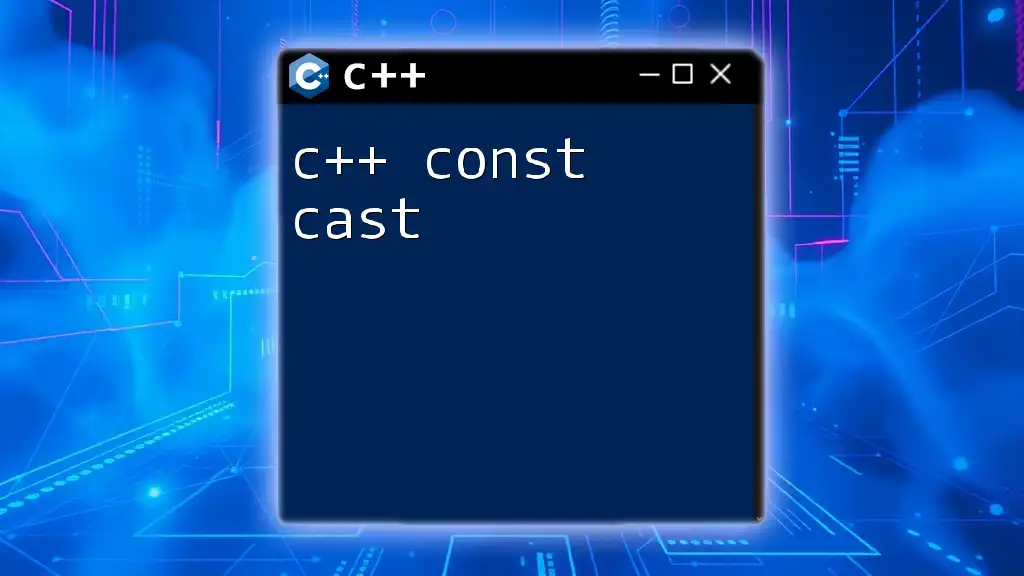
Conclusion
In summary, embracing c++ constness in your programming practices is invaluable in promoting safe, clear, and efficient code. Constness serves as a contract in your code, offering both security against accidental modifications and performance improvements through better compiler optimizations. By implementing const in variables, pointers, member functions, and templates, you ensure that your C++ code is robust, maintainable, and efficient.