A `constexpr` constructor in C++ allows the creation of objects at compile time, enabling the use of those objects in constant expressions, thereby improving performance and ensuring immutability.
class Point {
public:
constexpr Point(int x, int y) : x_(x), y_(y) {}
constexpr int getX() const { return x_; }
constexpr int getY() const { return y_; }
private:
int x_;
int y_;
};
constexpr Point p(3, 4); // Can be evaluated at compile time
Understanding Constexpr
What is `constexpr`?
`constexpr` is a keyword in C++ that specifies that a value can be evaluated at compile time. The primary advantage of using `constexpr` is improved performance, as it allows certain computations to be determined during compilation rather than at runtime. This leads to faster execution and reduces overhead, making your applications more efficient.
Why use `constexpr`? Using `constexpr` enhances your code by making it safer and more optimal. It allows you to eliminate redundant computations and supports more expressive code.
History of `constexpr` in C++
The `constexpr` feature was introduced in C++11 and has evolved significantly with subsequent standards:
- C++11: Initial introduction of `constexpr`, which allowed for basic constant expressions.
- C++14: Expanded functionality to include more complex functions and use-case scenarios.
- C++17: Introduced `if constexpr`, allowing conditional compile-time expressions.
- C++20: Further improvements, including the ability to use `constexpr` with more standard library features.
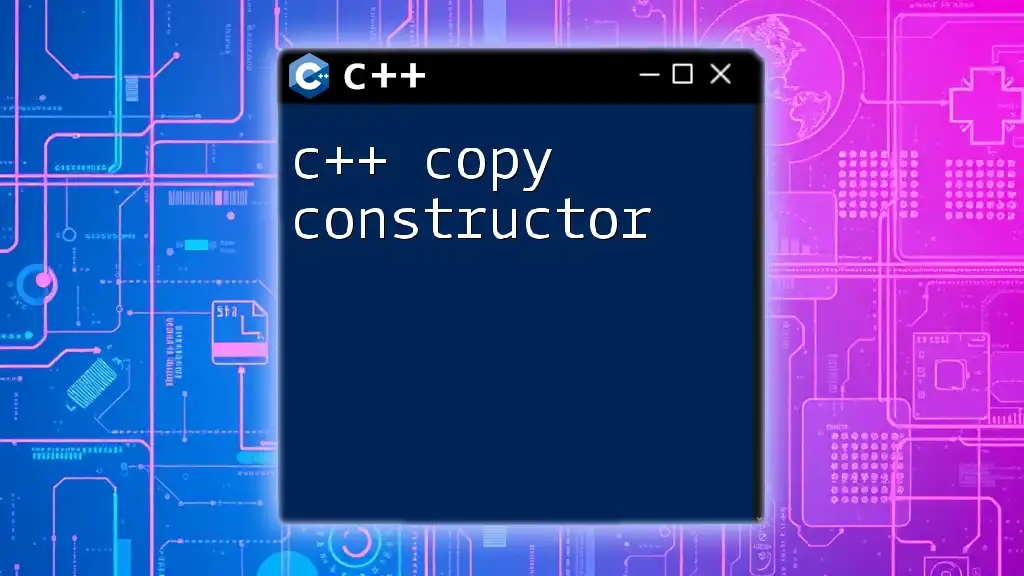
`constexpr` Constructors Explained
What is a `constexpr` Constructor?
A `constexpr` constructor is a special type of constructor that allows the initialization of class objects at compile time. This feature is particularly useful when objects are used in constant expressions, leading to improved performance through pre-calculated results.
Understanding the differences between regular constructors and `constexpr` constructors is crucial:
- Regular constructors are evaluated at runtime.
- `constexpr` constructors are evaluated at compile time, under certain conditions.
Syntax and Structure of Constexpr Constructors
The syntax of a `constexpr` constructor is quite straightforward. It defines the constructor with the keyword `constexpr` preceding it. To declare a `constexpr` constructor, you need to follow these guidelines:
- Return type must be a `class` or `struct`.
- The body must consist of statements that are valid in a constant expression.
- Only literals, references to constants, and calls to `constexpr` functions are allowed in the body.
Example Code Snippet
class Point {
public:
constexpr Point(int x, int y) : x_(x), y_(y) {}
private:
int x_;
int y_;
};
In the example above, the `Point` class has a `constexpr` constructor that initializes the values of `x_` and `y_`. This object can now be created at compile time, enabling its use in other compile-time constructs.
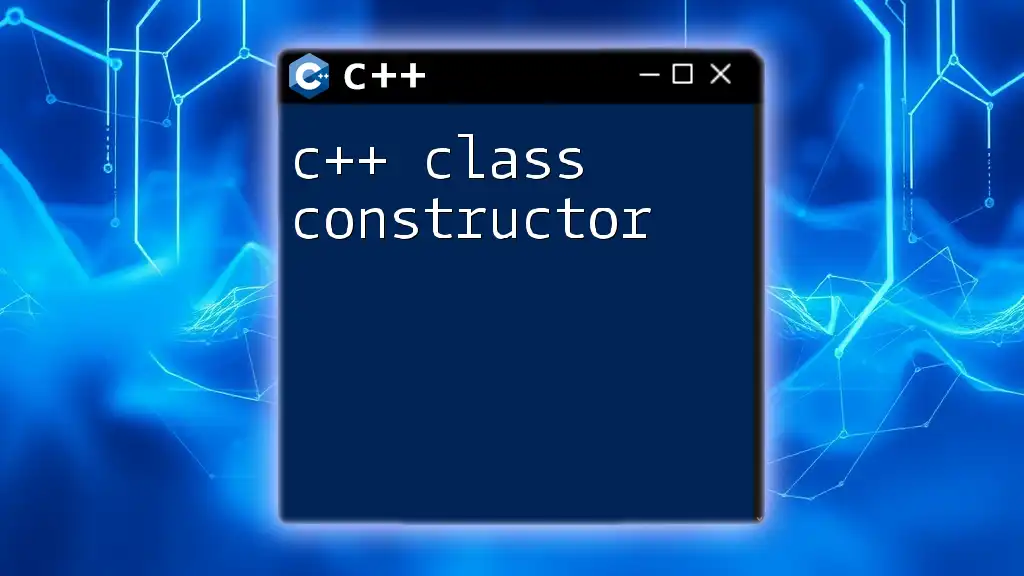
Key Features of `constexpr` Constructors
Compile-time Evaluation
One of the most significant advantages of `constexpr` constructors is their ability to enable compile-time evaluation. This means that the C++ compiler can evaluate expressions and calculations during compilation rather than at runtime.
To illustrate this concept, consider a simple function that calculates the square of a number:
Example Code Snippet
constexpr int square(int n) {
return n * n;
}
constexpr int result = square(5);
In the above example, the square of 5 is computed during compilation, significantly reducing runtime computation efforts.
Limitations and Considerations
When using `constexpr` constructors, there are specific limitations to keep in mind:
- You cannot use non-`constexpr` functions within a `constexpr` constructor.
- Dynamic memory allocation is not allowed. Objects initialized with `new` cannot be declared `constexpr`.
- Data members must be of literal types.
As a best practice, remember to keep your `constexpr` constructors simple and expressive, minimizing the complexity of any computations within them.
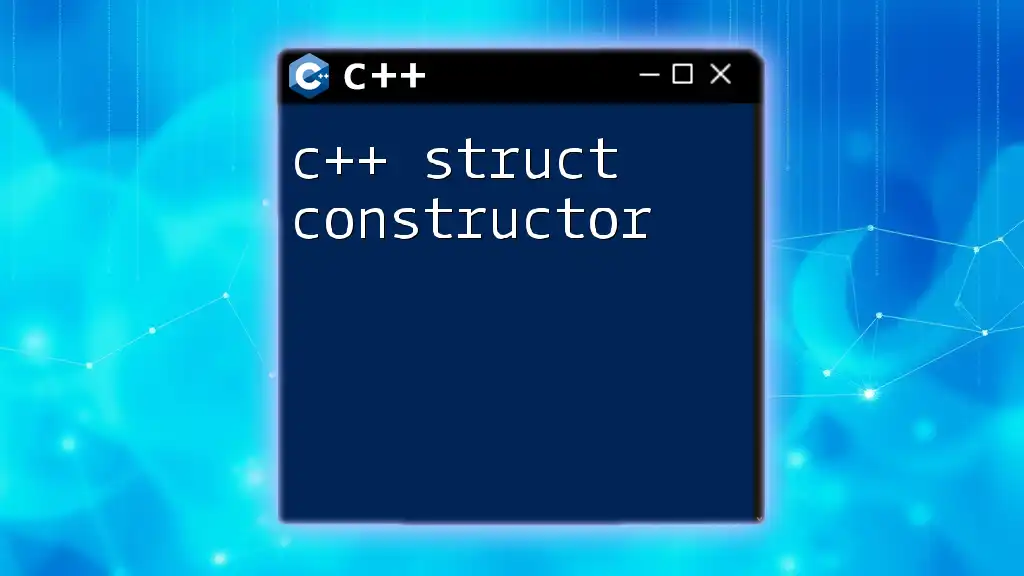
Use Cases for `constexpr` Constructors
When to Use `constexpr` Constructors
Using `constexpr` constructors is especially beneficial in scenarios where the object’s value will never change and will be required for compile-time calculations. Typical use cases include:
- Designing immutable data types.
- Building complex compile-time data structures, such as vectors or matrices.
Example Code Snippet
class Circle {
public:
constexpr Circle(double radius) : radius_(radius) {}
constexpr double area() const {
return 3.14159 * square(radius_);
}
private:
double radius_;
};
This `Circle` class not only allows the creation of objects at compile time but also includes a `constexpr` method for calculating the area, ensuring that both the radius and output can be determined during compilation.
Practical Applications
In real-world applications, the use of `constexpr` constructors enables developers to create highly efficient libraries, frameworks, and utilities where compile-time optimizations can lead to significant performance gains, especially in environments with limited resources.
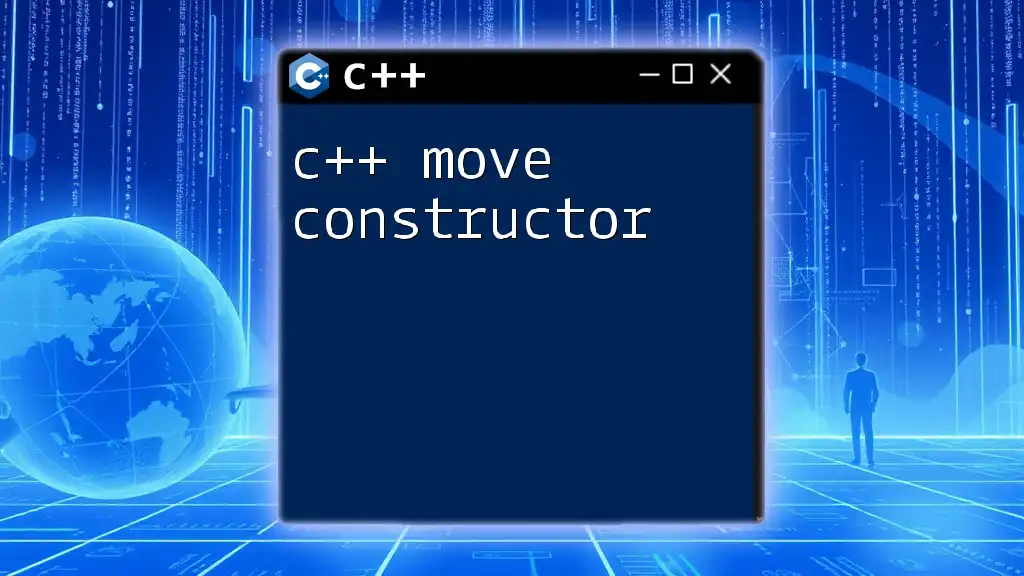
Advanced Topics
Constexpr and Templates
Another fascinating aspect of `constexpr` is its compatibility with C++ templates. This integration allows for highly flexible and reusable code.
Example Code Snippet
template <typename T>
constexpr T max(T a, T b) {
return (a > b) ? a : b;
}
The template function above can be utilized in various scenarios, demonstrating how `constexpr` can enhance the utility of generic programming.
Combining Constexpr with Other Features
`constexpr` can be combined with other C++ features such as `inline` variables, `constexpr` if statements, and `constexpr` lambdas to create robust compile-time computations. This ability opens doors for writing cleaner, more efficient code.
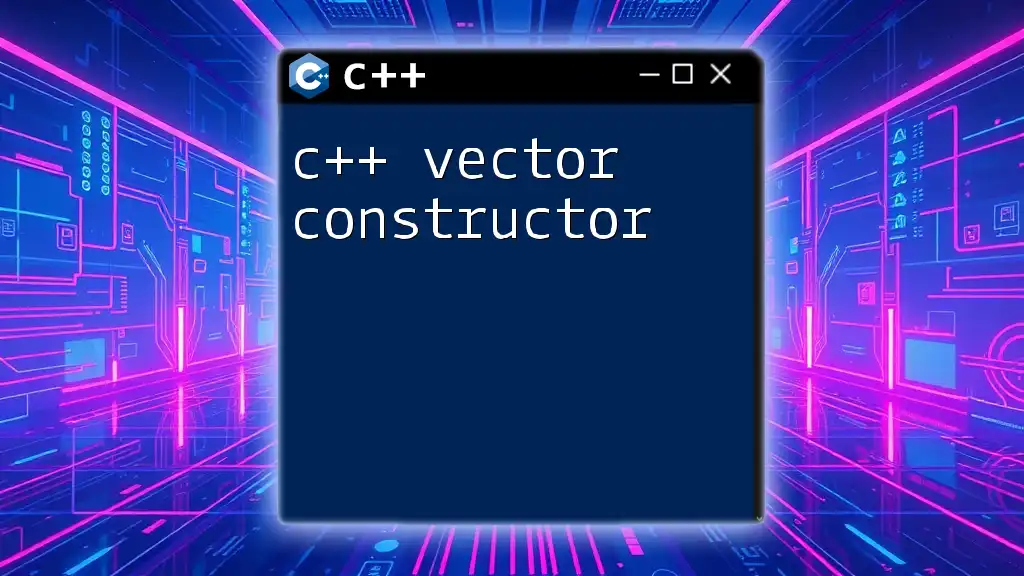
Performance Considerations
Benchmarking `constexpr` vs Regular Constructors
To illustrate the benefits of `constexpr` constructors, benchmarking is often needed. Analyzing the performance might reveal that `constexpr` constructors lead to reduced runtime overhead due to pre-computed values.
Recommendations for Optimizing Constexpr Usage
To maximize the benefits of `constexpr`, consider the following tips:
- Keep computations used in `constexpr` constructors simple and within the constraints outlined for compile-time evaluation.
- Employ `constexpr` functions to encapsulate more complex logic while maintaining the construct's compile-time evaluations.
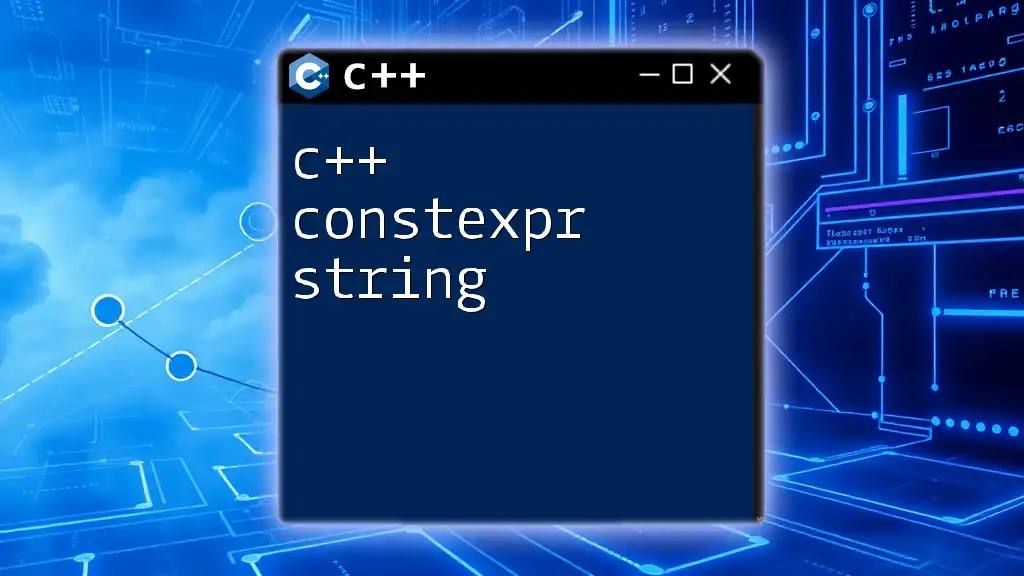
Conclusion
In summary, mastering the use of C++ `constexpr constructors` can lead to significantly improved performance and safety in your C++ applications. They enable compile-time evaluations that can eliminate unnecessary runtime overheads, providing both speed and efficiency to your code.
Embrace your newfound knowledge of `constexpr` constructors and experiment with implementing them in your own projects. Explore the potential of compile-time computations and join our community for more tutorials on advanced C++ topics!
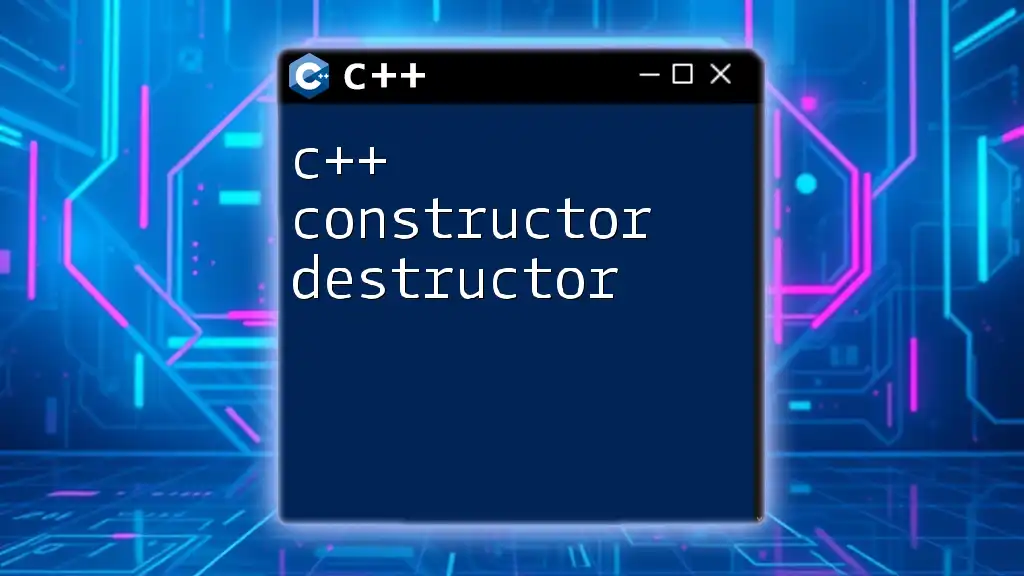
Additional Resources
For further reading on `constexpr` and C++ programming, consider reviewing the official C++ documentation, relevant books, and online tutorials that delve deeper into the advanced features of C++ and how to effectively utilize them to enhance your coding skillset.