Inherited constructors in C++ allow a derived class to use the constructors of its base class, enabling efficient code reuse and initialization.
class Base {
public:
Base(int x) { /* initialization code */ }
};
class Derived : public Base {
public:
using Base::Base; // Inherit constructors
};
Derived obj(5); // Calls Base's constructor with 5
Understanding Inherited Constructors in C++
Inherited constructors are a powerful feature in C++ that simplifies the management of constructors for derived classes. Understanding how to use inherited constructors effectively can greatly enhance the quality of your object-oriented design.
Definition of Inherited Constructors:
An inherited constructor in C++ allows a derived class to inherit the constructors from its base class. This makes it easier to initialize objects of the derived class without having to redefine the same constructor logic, thereby promoting code reuse.
C++ Constructor Inheritance:
In C++, constructors are not inherited by default. However, with the introduction of C++11, you can explicitly inherit constructors from a base class into a derived class. This is important because it allows you to call base class constructors directly when creating an instance of the derived class. It enhances maintenance and reduces redundancy.
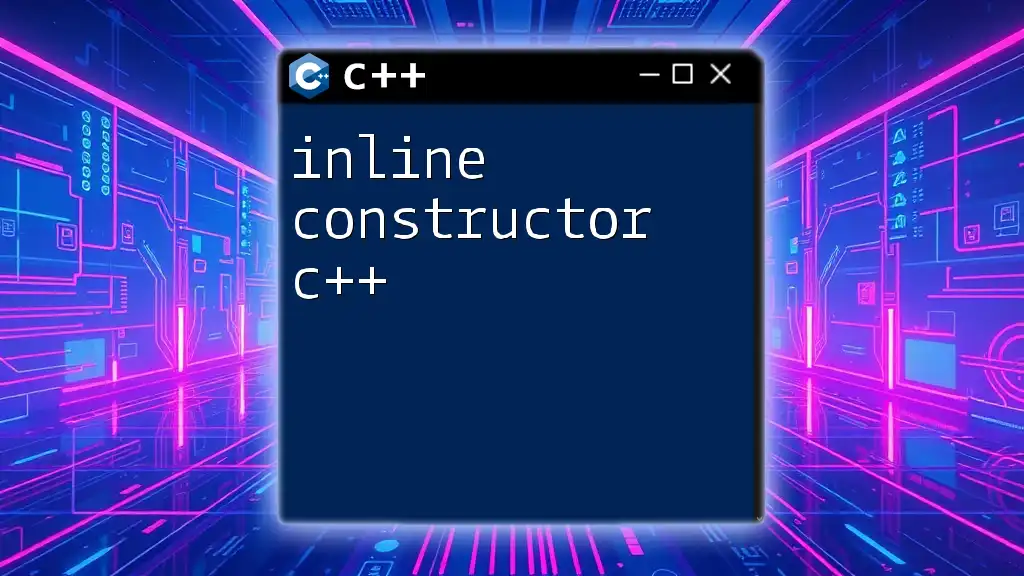
How to Use Inherited Constructors
Syntax of Inheriting Constructors in C++
Here’s the syntax to inherit constructors in C++:
using BaseClassName::ConstructorName;
This syntax tells the compiler that the derived class intends to make use of the constructors defined in the base class.
Enabling Constructor Inheritance
To enable constructor inheritance in a derived class, you utilize the `using` directive. Here’s an example to illustrate this:
class Base {
public:
Base(int x) { /* initialization code */ }
Base(double y) { /* initialization code */ }
};
class Derived : public Base {
public:
using Base::Base; // Inherit Base constructors
};
In this example, the `Derived` class can now utilize both constructors from the `Base` class. This means you can create instances of `Derived` using either an integer or double argument, directly invoking the corresponding base class constructor.
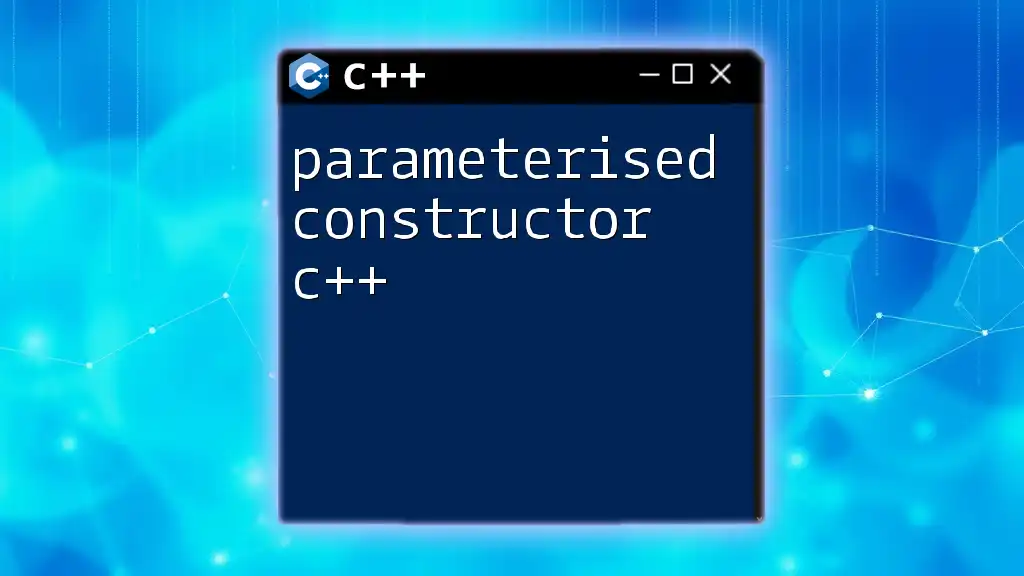
Practical Examples of Inherited Constructors
Example 1: Simple Inheritance of Constructors
Let’s start with a simple example where a derived class inherits a constructor from its base class:
class Animal {
public:
Animal(const std::string& name) {
// Initialization code
}
};
class Dog : public Animal {
public:
using Animal::Animal; // Inherit constructors
};
Here, the `Dog` class inherits the constructor from the `Animal` class. When you create an instance of `Dog`, you can directly use the constructor of `Animal`:
Dog myDog("Buddy");
This results in the `Animal` constructor being called with the string "Buddy".
Example 2: Multiple Constructors in Base Class
Let’s see how multiple constructors in a base class can be inherited:
class Vehicle {
public:
Vehicle(int wheels) { /* initialization code for wheels */ }
Vehicle(const std::string& type) { /* initialization code for vehicle type */ }
};
class Car : public Vehicle {
public:
using Vehicle::Vehicle; // Inherit both constructors
};
In this case, the `Car` class can be initialized with both types of constructors:
Car myCar(4); // Calls the Vehicle(int) constructor
Car anotherCar("Sedan"); // Calls the Vehicle(const std::string&) constructor
Example 3: Constructor Initialization Lists
Constructor initialization lists are essential for efficiently initializing class members. Here’s an example of how to inherit constructors while using initialization lists:
class Shape {
public:
Shape(int width, int height) {
// Shape initialization code
}
};
class Rectangle : public Shape {
public:
using Shape::Shape; // Inherit constructors
};
// Usage
Rectangle rect(10, 20); // Calls the Shape(int, int) constructor
In this example, the `Rectangle` class can also utilize the constructor from the `Shape` class to initialize its dimensions.
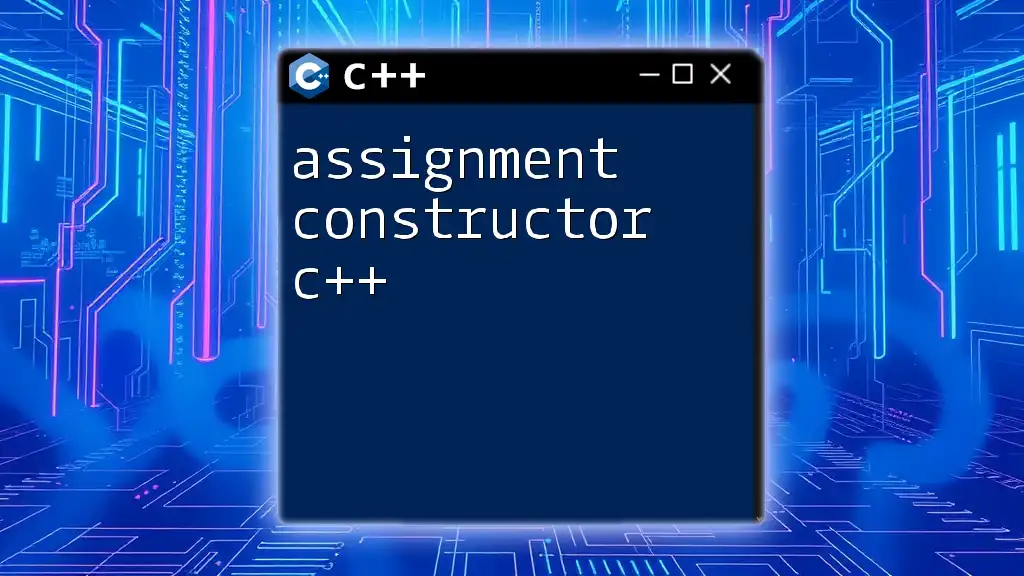
Limitations and Considerations
While inherited constructors streamline code, they come with certain considerations.
When Not to Use Inherited Constructors:
Inherited constructors are not always beneficial. If the derived class has unique initialization logic that doesn't apply to the base class, it's better to explicitly define a constructor in the derived class. This ensures clarity and proper encapsulation.
Overriding Inherited Constructors:
When you need to modify inherited constructors or introduce additional parameters, you can override them. This involves creating a new constructor in the derived class and delegating to the base class constructor. For example:
class Base {
public:
Base(int x) { /* initialization code */ }
};
class Derived : public Base {
public:
using Base::Base; // Inherit constructors
Derived(int x, int y) : Base(x) {
// Additional initialization code specific to Derived
}
};
In this case, the `Derived` class has a new constructor that takes two parameters. It calls the inherited constructor with one parameter, allowing it to incorporate its specific initialization logic.
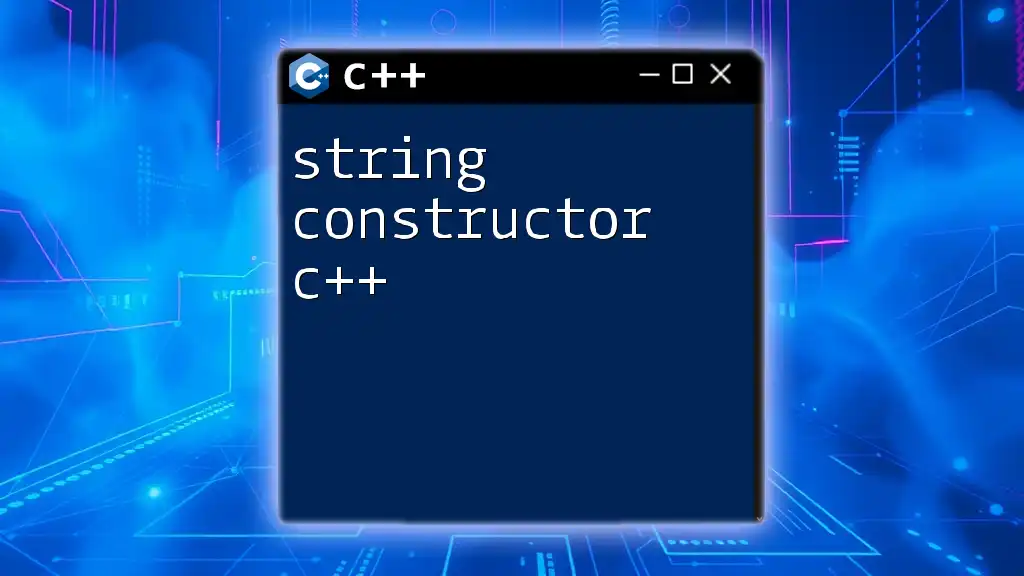
Best Practices for Using Inherited Constructors
To make the most of inherited constructors, consider the following best practices:
Keep Constructors Simple:
Simplicity enhances maintainability. Strive for constructors that focus on initializing objects without excessive complexity.
Documenting Inherited Constructors:
Proper comments and documentation are critical for helping future developers understand the architecture. Indicate which constructors are inherited and why they are necessary for clarity in collaboration.
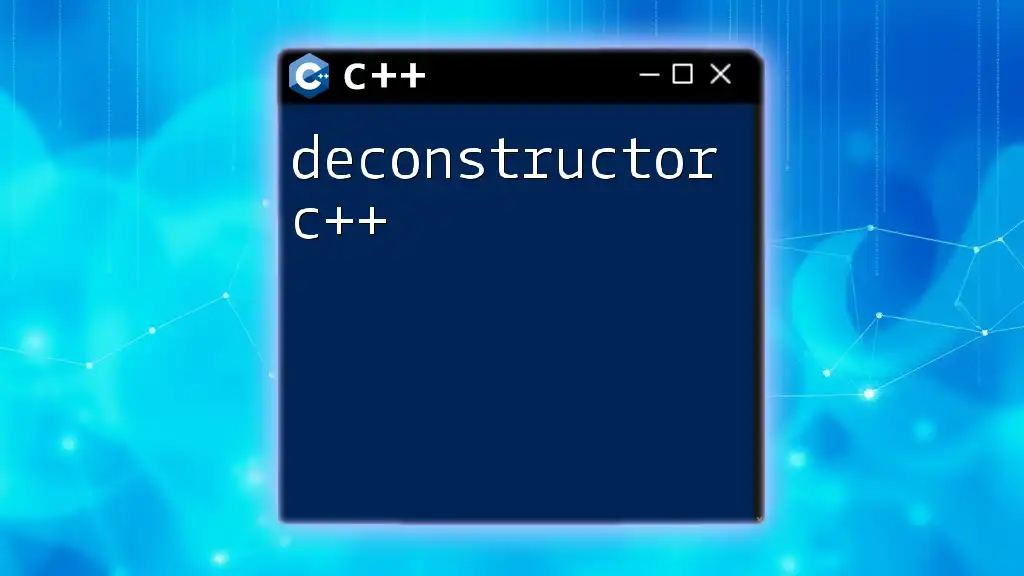
Conclusion
Inherited constructors in C++ can significantly enhance the reusability and efficiency of your code. Understanding how to implement and leverage these features will result in better-designed object-oriented systems. By recognizing when and how to use them, you can create code that is not only cleaner but also easier to maintain. As you develop your C++ skills, keep exploring the advanced concepts associated with inheritance and constructors to hone your programming expertise.
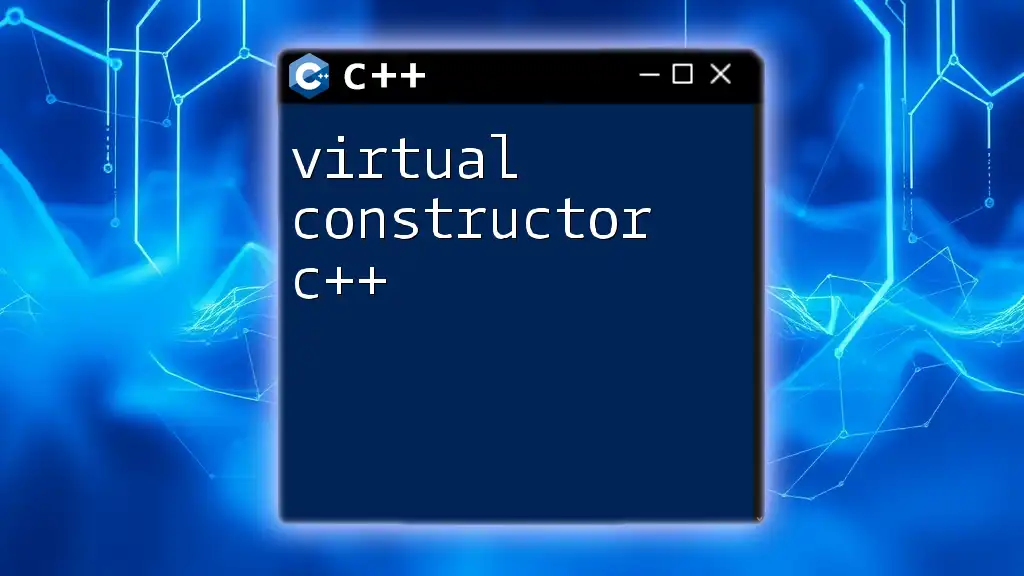
FAQs
What are inherited constructors in C++?
Inherited constructors allow a derived class to inherit constructors from its base class, enabling easy initialization during object creation.
How do I enable constructor inheritance in C++?
Constructor inheritance is enabled using the `using` directive followed by the base class name and constructor names.
Can I inherit multiple constructors?
Yes, you can inherit multiple constructors from a base class to a derived class using the `using` directive for each constructor.
What should I be cautious about when using inherited constructors?
Be careful when inherited constructors may not apply specifically to the derived class, or when unique initialization logic is required that differs from the base class.