A linked list destructor in C++ is a special member function that deallocates memory for each node in the linked list to prevent memory leaks when the list goes out of scope. Here's a simple example:
#include <iostream>
struct Node {
int data;
Node* next;
Node(int val) : data(val), next(nullptr) {}
};
class LinkedList {
public:
Node* head;
LinkedList() : head(nullptr) {}
~LinkedList() { // Destructor
Node* current = head;
while (current != nullptr) {
Node* nextNode = current->next;
delete current; // Deallocate memory
current = nextNode;
}
}
};
Understanding Linked Lists
What is a Linked List?
A linked list is a fundamental data structure where each element, known as a node, contains two essential components: data and a pointer (or link) to the next node in the sequence. Unlike arrays, linked lists allow for dynamic resizing, making them more flexible when it comes to memory allocation. Each node can be allocated and deallocated independently, which is particularly advantageous when the size of your dataset fluctuates.
Types of Linked Lists
When working with linked lists, it's important to understand the variations available:
-
Singly Linked List: Each node points to the next, forming a chain. This structure allows for efficient insertions and deletions at the cost of reverse traversal.
-
Doubly Linked List: Nodes contain two pointers, one for the next node and another for the previous node. This creation allows bi-directional traversal but requires additional memory for the extra pointer.
-
Circular Linked List: In this structure, the last node points back to the first node, creating a circular chain. This is ideal for applications requiring cyclical traversal.
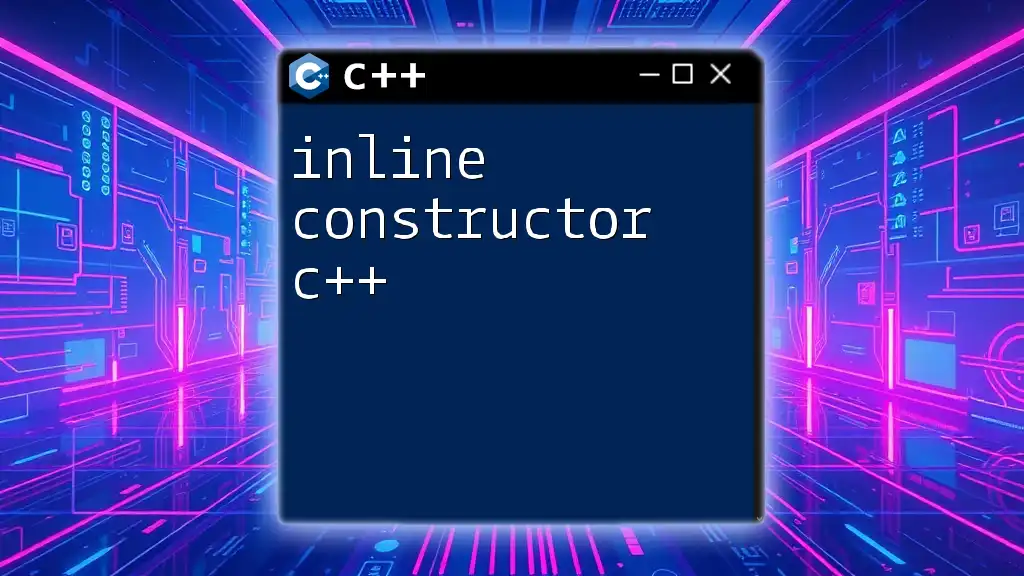
Destructor in C++
What is a Destructor?
In C++, a destructor is a special member function that is invoked when an object goes out of scope or is explicitly deleted. The primary role of a destructor is to release resources that were allocated during the lifetime of the object, ensuring that no memory leaks occur. It is crucial for managing dynamic memory, especially in linked lists where nodes are frequently added and removed.
Syntax of Destructor
Defining a destructor in C++ is straightforward:
class Node {
public:
int data;
Node* next;
// Destructor
~Node() {
// clean up resources
}
};
In this example, the destructor is indicated by the tilde (`~`) followed by the class name. It is called automatically when an instance of the class is destroyed.
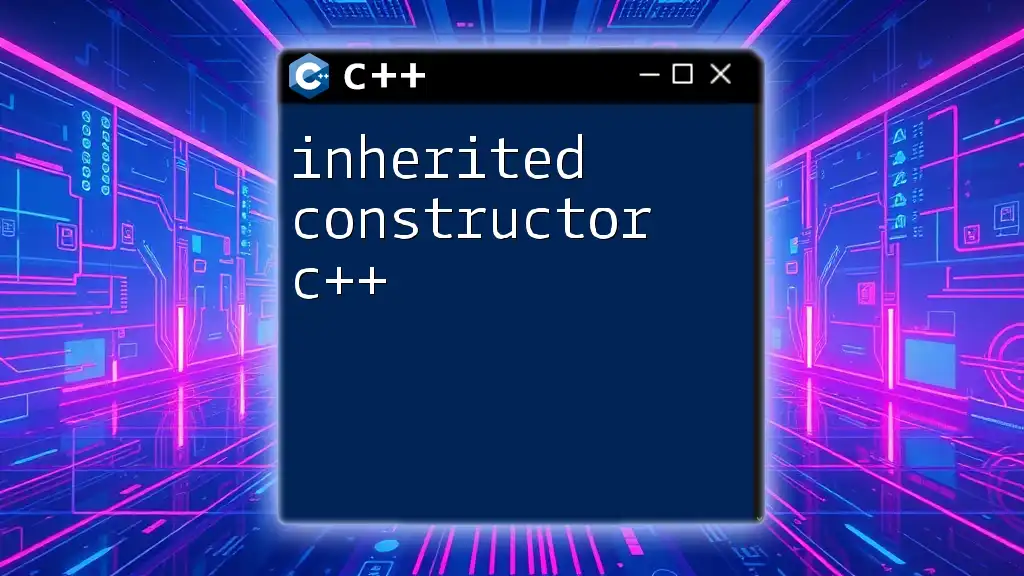
Linked List Destructor: Implementation
The Need for a Linked List Destructor
Properly managing memory in C++ is critical, especially when using dynamic data structures like linked lists. If you do not implement a destructor for your linked list, you run the risk of memory leaks, where allocated memory remains inaccessible and unreturned to the system, leading to inefficient use of resources.
Implementing the Linked List Destructor
Creating a destructor for a linked list involves traversing through each node and deleting it to free memory. Let’s explore how to implement this for a singly linked list.
Example Code: Singly Linked List Destructor
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(nullptr) {}
// Destructor
~LinkedList() {
Node* current = head;
Node* next;
while (current != nullptr) {
next = current->next;
delete current; // Free memory
current = next; // Move to the next node
}
}
};
Explanation of the Destructor Code
-
Initialization: The destructor initializes a pointer, `current`, to `head`, which points to the first node in the linked list.
-
Traversal: A loop continues as long as `current` is not `nullptr`. During each iteration, the next node is stored in the `next` pointer.
-
Memory Deallocation: The `delete` operator is used on `current`, which frees the memory allocated for that node. The pointer then advances to the next node until all nodes have been deleted.
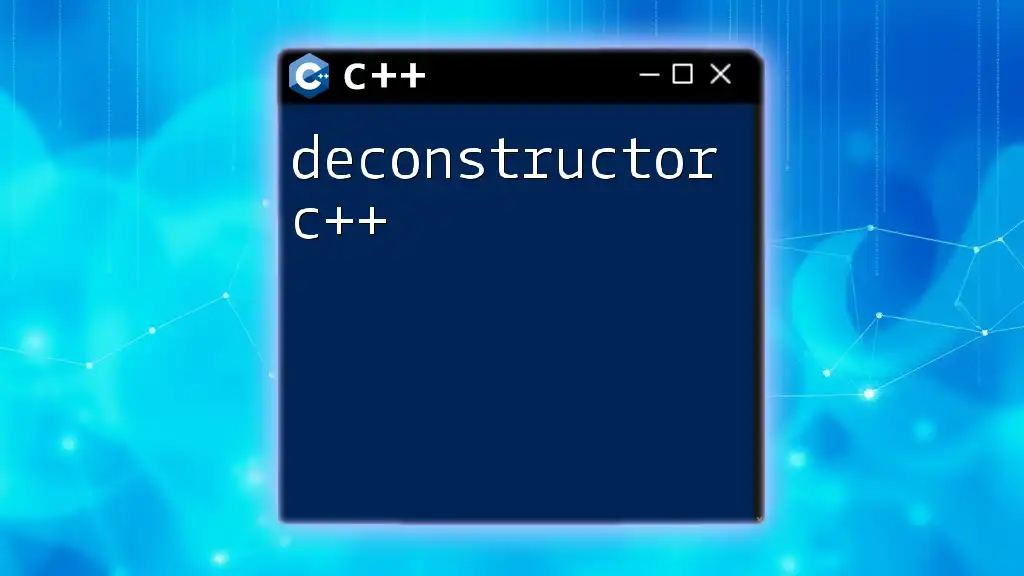
Best Practices for Implementing Destructors
Consistency in Resource Management
To maintain efficient memory management, it is essential to ensure that every allocation using `new` has a corresponding deallocation using `delete`. This practice prevents memory from being "lost" and ensures that your application runs smoothly.
Avoiding Memory Leaks
Utilize tools such as Valgrind to analyze your code for memory leaks. Regular testing can identify leaks early in the development process, helping you maintain clean and efficient code.
Use of Smart Pointers
Consider employing smart pointers such as `std::shared_ptr` and `std::unique_ptr`. These modern C++ features automatically handle memory deallocation, significantly reducing the chances of memory leaks. They can lead to cleaner, safer code by managing the lifecycle of resources effectively.
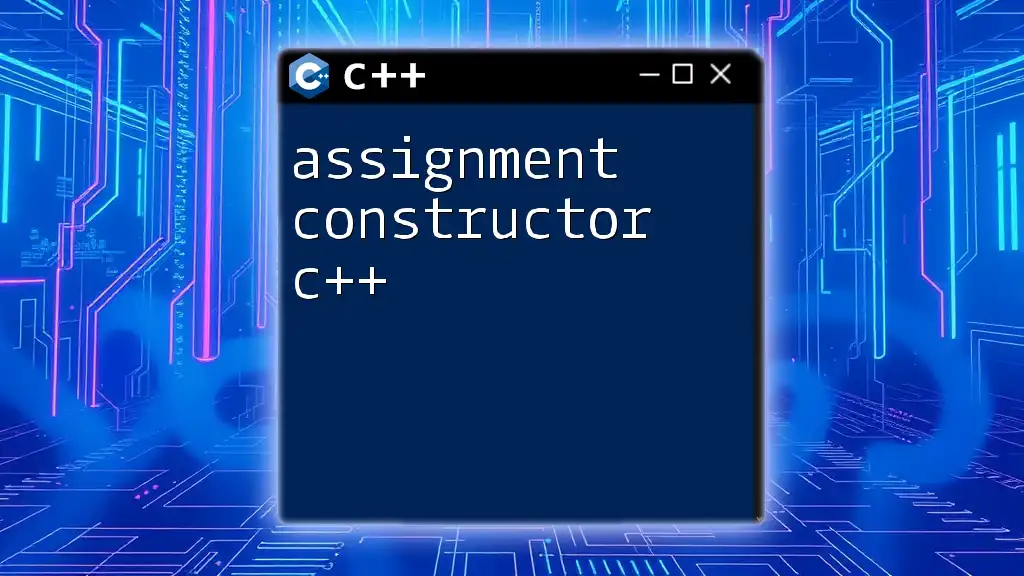
Common Mistakes to Avoid with Linked List Destructors
Forgetting to Implement a Destructor
Neglecting to implement a destructor can lead to substantial memory leaks, causing your application to consume more memory than necessary over time. This oversight can severely impact performance, especially in long-running applications.
Incorrect Node Deletion
Errors can occur if you attempt to delete nodes that haven’t been properly initialized or are malformed. Always ensure that your pointers are valid before attempting to delete any nodes.
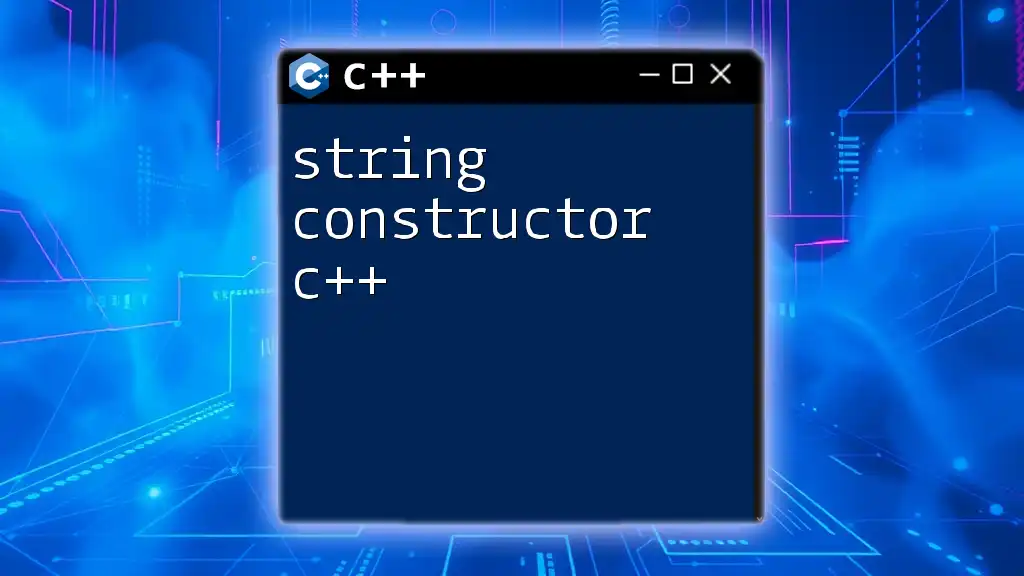
Conclusion
In summary, the linked list destructor in C++ is a vital component of managing memory effectively in dynamic data structures. Proper implementation of destructors ensures that resources are released, preventing memory leaks and maintaining application performance. As we work with linked lists, adhering to best practices and avoiding common pitfalls can help us achieve efficient and reliable code.
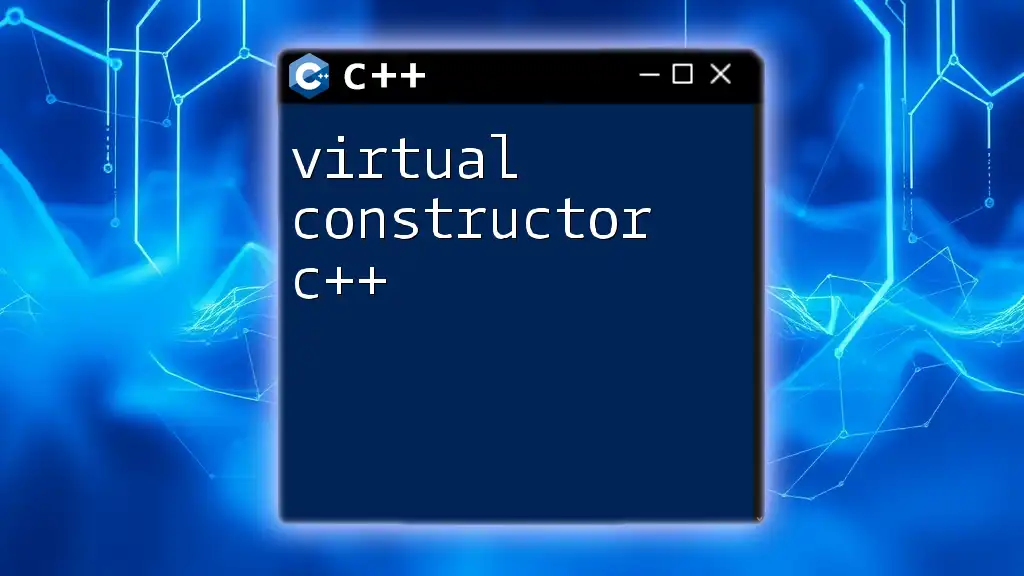
Additional Resources
Explore further reading on linked lists, memory management techniques, and advanced C++ features to enhance your understanding and improve your programming skills.
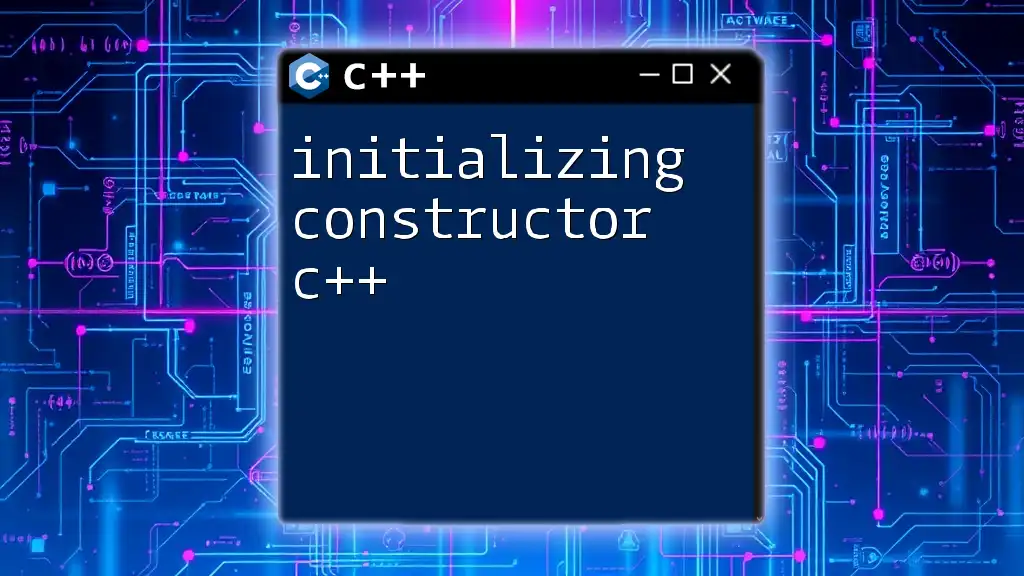
FAQs
Why is a Destructor Necessary for Linked Lists?
A destructor is necessary to free the dynamically allocated memory for each node in a linked list. Without it, the memory remains allocated and inaccessible, leading to leaks.
Can I Use Shared Pointers Instead of Raw Pointers in Linked Lists?
While it is possible to implement linked lists using smart pointers, it's important to understand the trade-offs. Smart pointers manage memory automatically but may incur additional overhead, making them unsuitable for all scenarios.
What Happens if I Forget to Define a Destructor?
If you forget to define a destructor, any dynamically allocated memory will not be released when the linked list goes out of scope, leading to memory leaks and inefficient memory usage.