In C++, a template structure allows for the creation of generic data types that can operate with any data type, enhancing code reusability and flexibility.
#include <iostream>
template <typename T>
struct MyStruct {
T value;
void display() {
std::cout << "Value: " << value << std::endl;
}
};
int main() {
MyStruct<int> intStruct;
intStruct.value = 42;
intStruct.display();
MyStruct<std::string> stringStruct;
stringStruct.value = "Hello, Templates!";
stringStruct.display();
return 0;
}
Understanding Structure in C++
What is a Struct in C++?
A `struct` in C++ is a user-defined data type that allows you to group related variables together. It serves as a blueprint for creating complex data types that can hold multiple values of different types.
One key difference between struct and class is the default accessibility; members of a struct are public by default, while members of a class are private. Here’s a basic syntax example:
struct Person {
std::string name;
int age;
};
In this example, the `Person` struct holds two members: `name`, which is of type `string`, and `age`, which is an `int`.
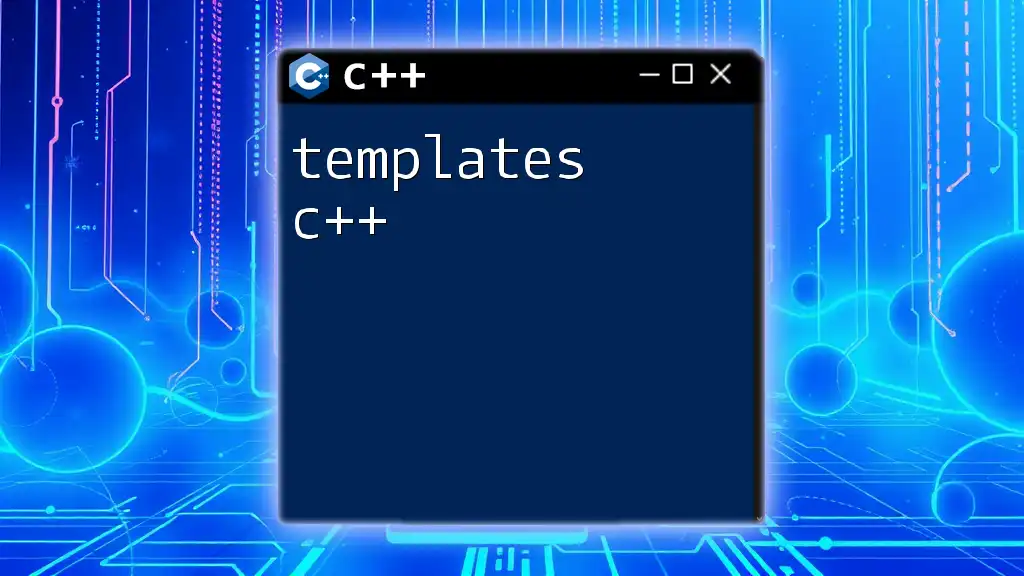
Introduction to Template Programming
What is a Template in C++?
A template in C++ is a powerful feature that allows you to write generic and reusable code. Templates can be applied to functions and classes, enabling developers to create algorithms and data structures that can work with any data type.
For instance, a simple function template for adding two numbers can be written as follows:
template <typename T>
T add(T a, T b) {
return a + b;
}
In this function template, T is a placeholder for any data type. When you call this function with integers, it behaves like an integer addition function; if you use it with floats, it operates as a float addition function.
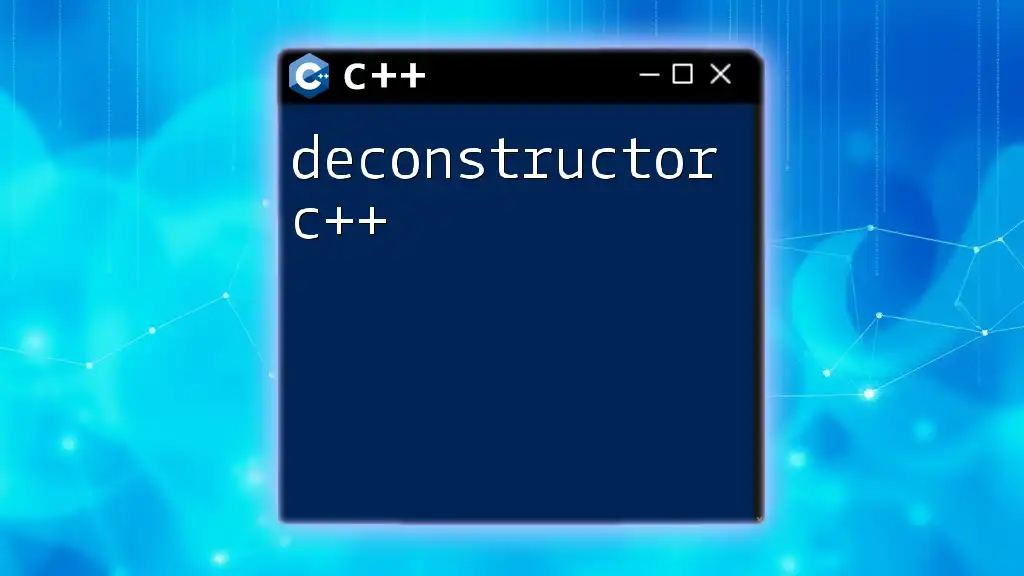
Combining Templates with Structures
What is a Template Struct in C++?
A template struct in C++ allows you to create a structure that can operate with any data type specified at compile time. This is particularly useful for writing generic data structures.
The following is an example of a template struct:
template <typename T>
struct Container {
T element;
void display() {
std::cout << "Element: " << element << std::endl;
}
};
In this `Container` struct, T can be replaced with any type (like `int`, `string`, etc.) when an instance of the structure is created. This gives you the flexibility to create containers for various data types without duplicating code.
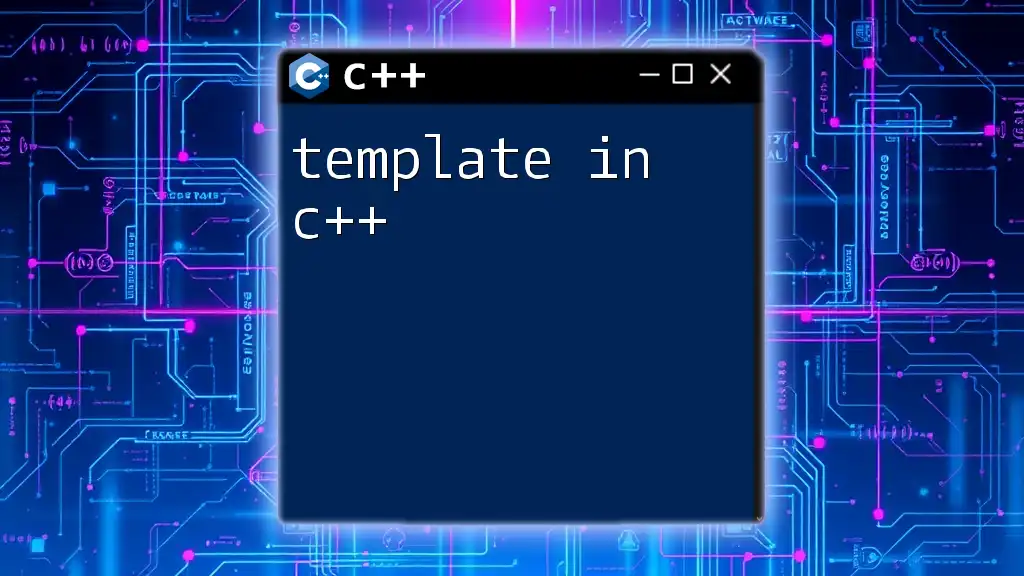
Implementing a Template Struct
Steps to Create a Template Struct
Creating a template struct involves defining the template with the `template` keyword followed by its parameter(s). Here’s a simple example of a template struct called `Box`:
template <typename T>
struct Box {
T content;
void showContent() {
std::cout << "Content: " << content << std::endl;
}
};
In this example, `Box` can hold any type of content, and the `showContent` method will display that content.
To create a `Box` for integers:
Box<int> intBox;
intBox.content = 5;
intBox.showContent(); // Displays: Content: 5
For a `Box` containing strings:
Box<std::string> strBox;
strBox.content = "Hello, C++!";
strBox.showContent(); // Displays: Content: Hello, C++!
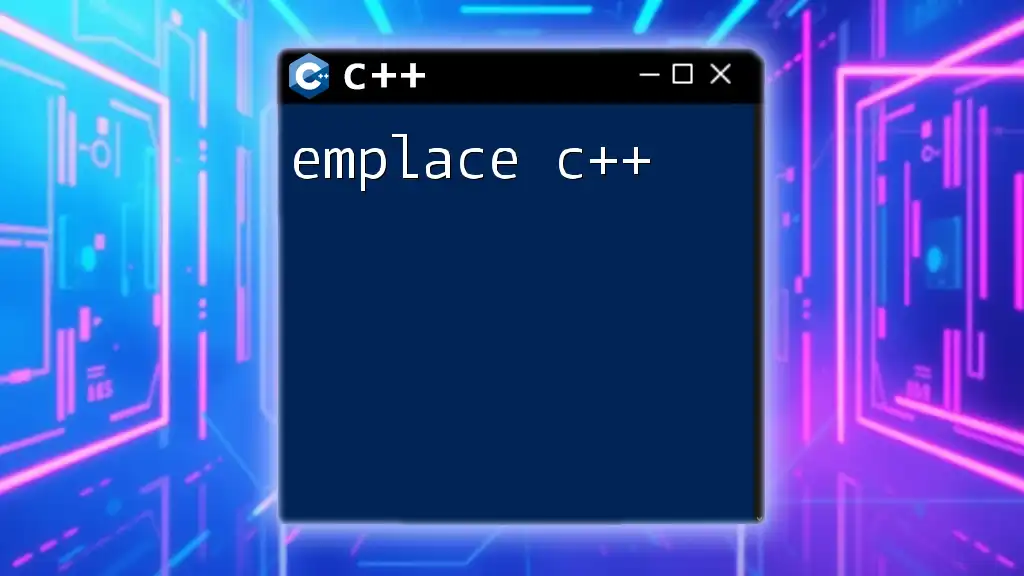
Specializing Template Structures
What is Template Specialization?
Template specialization allows you to define a specific implementation of a template struct for a specific data type. This can be particularly useful when you need different behavior for a specific type compared to the general template behavior.
For instance, you can specialize the `Box` struct for int types:
template <>
struct Box<int> {
int content;
void showContent() {
std::cout << "Integer Content: " << content << std::endl;
}
};
With this specialization, if you create a `Box<int>` instance, it will use the specialized version, which can provide functionality uniquely tailored for integers.
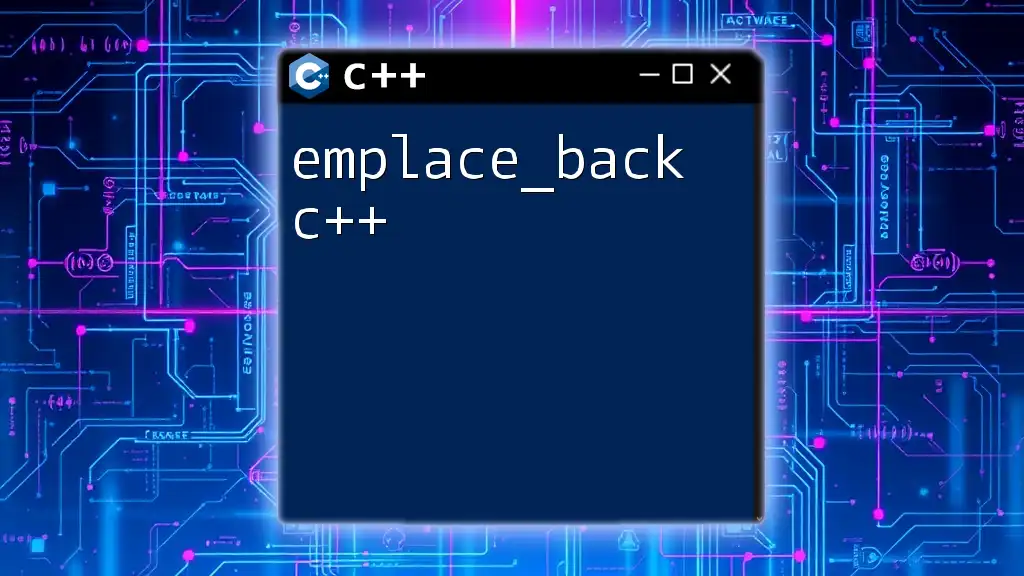
Use Cases for Template Structures
Real-World Applications
Template structs provide significant advantages in various programming scenarios where code reusability and flexibility are paramount. Here are a few common use cases:
- Data Structures: You can create linked lists or trees using template structs that can store any data type. Here is an example of a simple linked list node implemented as a template struct:
template <typename T>
struct Node {
T data;
Node* next;
};
This `Node` struct can be part of a linked list that holds integers, strings, or any other data type, thereby creating a highly reusable piece of code.
- Containers: Many standard containers in the STL (Standard Template Library) such as `std::vector` or `std::map` are essentially template structs that allow for storage of various data types.
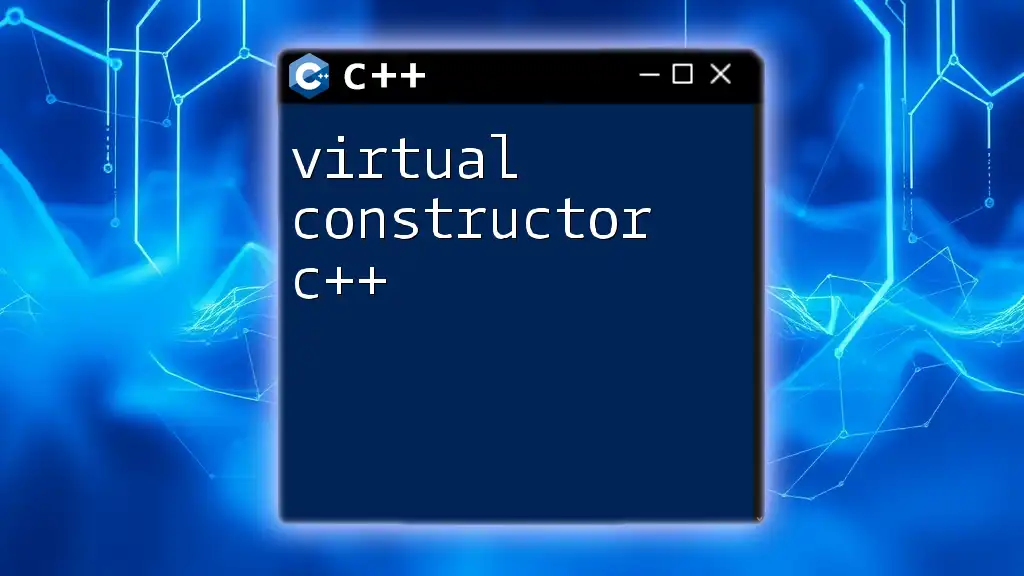
Performance Considerations
Are Template Structs Efficient?
Template structs in C++ can lead to significant performance benefits. Since templates are resolved at compile time, the compiler creates a separate instantiation of the template for each data type used. This results in optimized code tailored for specific types, thus offering greater performance than using a common base type combined with dynamic allocation or virtual functions.
However, there can also be concerns regarding code bloat, as each specific instance of a template results in a separate copy in the compiled binary. To mitigate this, it’s crucial to only use templates when necessary and to keep a close eye on your code’s structure.
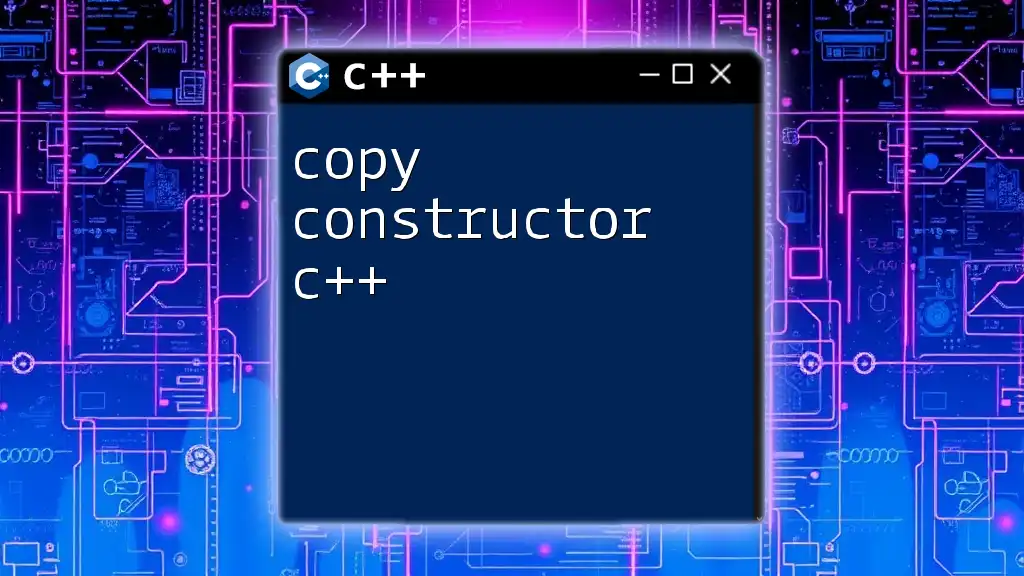
Common Mistakes and Pitfalls
Errors to Avoid with Template Structs
When working with template structures, developers can sometimes encounter issues that lead to compiler errors or unintended behavior. Here are a few common pitfalls:
- Recursive Template Definitions: Be cautious about defining template structs that contain themselves indefinitely. This can result in compilation errors due to exceeding template depth limits. For example:
template <typename T>
struct RecursiveStruct {
RecursiveStruct<T> nested; // This will lead to compilation errors!
};
- Overusing Template Specialization: While specialization can enhance functionality, overusing it can result in a codebase that is difficult to read and maintain. Aim to keep your templates as generic as possible unless specific specializations are genuinely necessary.
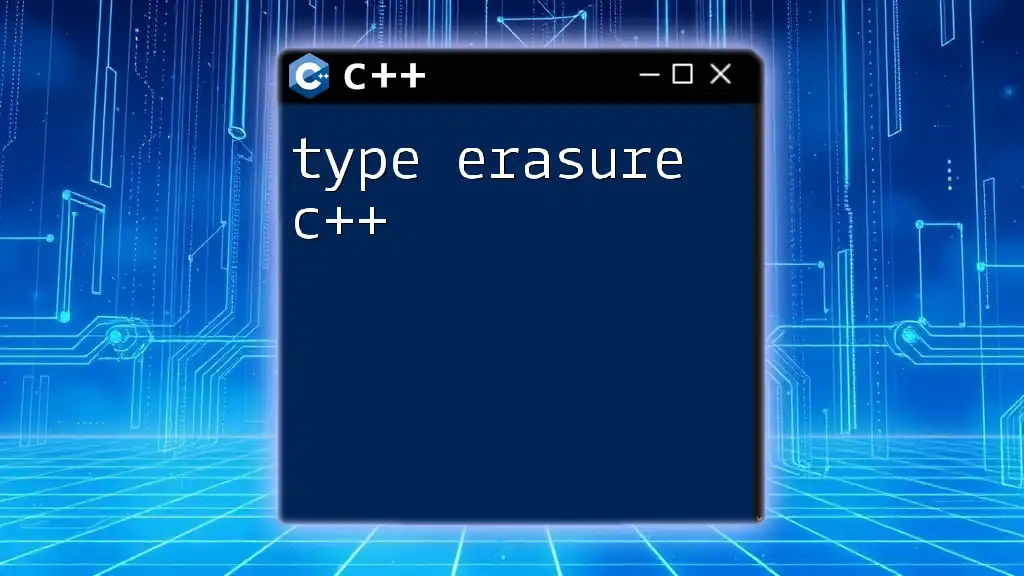
Conclusion
In conclusion, template structures in C++ provide a powerful mechanism to create flexible and reusable code. By understanding how to define and implement template structs, you can enhance your programming skills and improve the efficiency of your code. Don't hesitate to experiment with these concepts in your projects, as realizing the full potential of template programming can greatly benefit your development process.
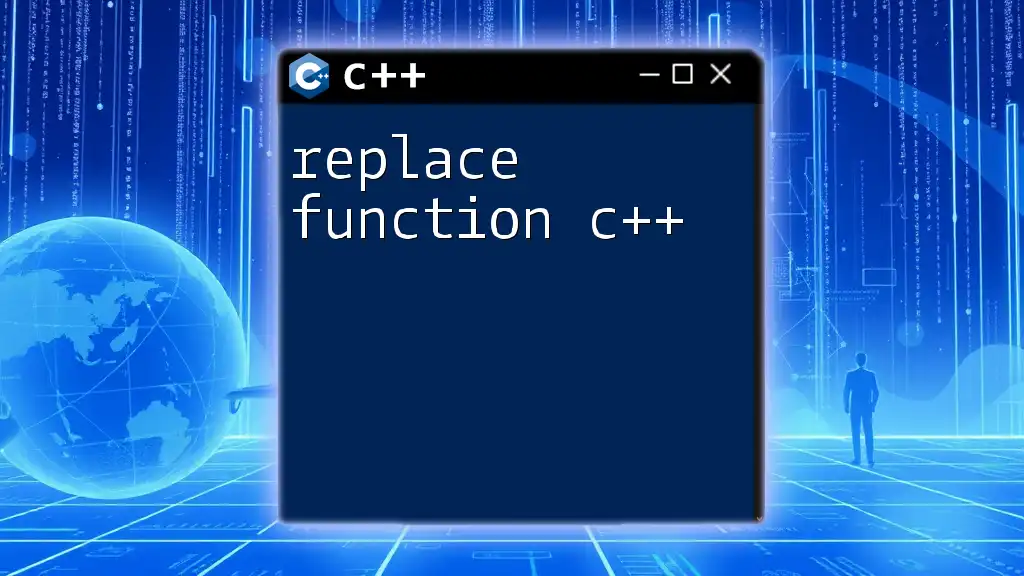
Call to Action
If you found this article helpful and are eager to learn more about C++ programming, be sure to follow us for ongoing insights, tips, and tutorials. We also invite you to share your experiences, questions, or topic suggestions in the comments below!