In C++, control structures are programming constructs that dictate the flow of execution based on specific conditions, enabling decision-making and looping within the code.
Here’s an example of a simple `if-else` statement and a `for` loop in C++:
#include <iostream>
using namespace std;
int main() {
int number = 10;
// Control structure: if-else
if (number > 0) {
cout << "Positive number" << endl;
} else {
cout << "Non-positive number" << endl;
}
// Control structure: for loop
for (int i = 0; i < 5; i++) {
cout << "Iteration " << i << endl;
}
return 0;
}
What Are Control Structures?
Control structures are foundational elements in programming that dictate the flow of execution based on certain conditions. They are essential for decision-making, looping through data, and controlling the execution path in a modular fashion. Understanding control structures in C++ is crucial for writing effective and logical programs.
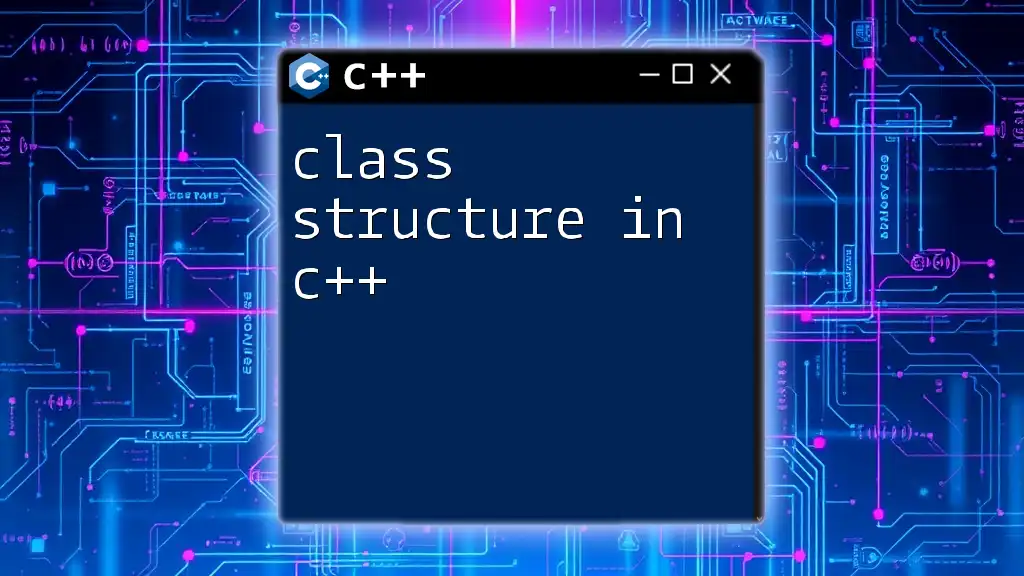
Types of Control Structures in C++
Conditional Control Structures
Conditional control structures are used to execute specific parts of code based on whether a certain condition is true or false. These structures allow for decision-making in the program's flow.
if Statements
The `if` statement is the simplest form of conditional execution. It checks a condition and executes a block of code if the condition evaluates to true.
Syntax:
if (condition) {
// code to be executed if condition is true
}
Example:
int x = 10;
if (x > 5) {
std::cout << "x is greater than 5";
}
In this example, since `x` is indeed greater than 5, the condition is fulfilled, and the message is printed.
if-else Statements
To handle alternative paths of execution, we use the `if-else` statement. This structure allows the program to choose between two possible paths based on a condition.
Syntax:
if (condition) {
// code for true condition
} else {
// code for false condition
}
Example:
int x = 3;
if (x > 5) {
std::cout << "x is greater than 5";
} else {
std::cout << "x is 5 or less";
}
If `x` is greater than 5, the first block executes; otherwise, the code within the `else` block runs.
else if Ladder
For scenarios requiring multiple conditions, the `else if` ladder is very useful. It allows for multiple checks to be performed sequentially.
Syntax:
if (condition1) {
// code for condition1
} else if (condition2) {
// code for condition2
} else {
// code if all conditions are false
}
Example:
int x = 7;
if (x > 10) {
std::cout << "x is greater than 10";
} else if (x > 5) {
std::cout << "x is greater than 5 but less than or equal to 10";
} else {
std::cout << "x is 5 or less";
}
This structure checks each condition in order, enabling complex decision-making.
Switch Statements
When you need to select one of many possible execution paths from a single variable, a `switch` statement can simplify the code.
Syntax:
switch (variable) {
case value1:
// code block for value1
break;
case value2:
// code block for value2
break;
default:
// code block for all other cases
}
Example:
int day = 4;
switch (day) {
case 1:
std::cout << "Monday";
break;
case 2:
std::cout << "Tuesday";
break;
default:
std::cout << "Another day";
break;
}
In this case, if `day` equals 1 or 2, it outputs the respective day. If it doesn't match either case, it defaults to printing "Another day".
Loop Control Structures
Loop control structures allow the program to execute a block of code multiple times, either for a specific number of iterations or until certain conditions are met.
for Loops
The `for` loop is commonly used when the number of iterations is known ahead of time. It includes initialization, a condition, and an increment expression.
Syntax:
for (initialization; condition; increment) {
// code to be executed
}
Example:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration " << i << std::endl;
}
This loop runs five times, outputting the current iteration number on each pass.
while Loops
A `while` loop continues executing its block as long as a specified condition remains true. This loop allows for dynamic iteration based on the runtime condition.
Syntax:
while (condition) {
// code to be executed
}
Example:
int i = 0;
while (i < 5) {
std::cout << "Iteration " << i << std::endl;
i++;
}
In this case, the loop will keep running until `i` reaches 5.
do-while Loops
The `do-while` loop is similar to the `while` loop but guarantees at least one execution of the block, as the condition is checked after the block has been executed.
Syntax:
do {
// code to be executed
} while (condition);
Example:
int i = 0;
do {
std::cout << "Iteration " << i << std::endl;
i++;
} while (i < 5);
This will output the iterations regardless of the initial condition value and will stop when `i` equals 5.
Jump Control Structures
Jump control structures allow for immediate changes in the flow of execution, enabling you to exit loops or skip iterations.
break Statement
The `break` statement is utilized to exit a loop or switch statement abruptly. This can be particularly useful in scenarios where the termination of the loop occurs under specific conditions.
Example:
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // Exit the loop when i equals 5
}
std::cout << i << std::endl; // Output 0 through 4
}
Here, the loop stops executing once `i` equals 5.
continue Statement
The `continue` statement is used to skip the current iteration of a loop and proceed with the next iteration, based on a specified condition.
Example:
for (int i = 0; i < 10; i++) {
if (i % 2 == 0) {
continue; // Skip even numbers
}
std::cout << i << std::endl; // Outputs only odd numbers
}
In this example, all even numbers are skipped, resulting in only odd numbers being printed.
return Statement
The `return` statement exits from the current function and can also return a value. This is crucial in functions that perform computations and need to provide output.
Example:
int add(int a, int b) {
return a + b; // Returns the sum of a and b
}
The `return` keyword effectively ends the function and sends the result back to the caller.
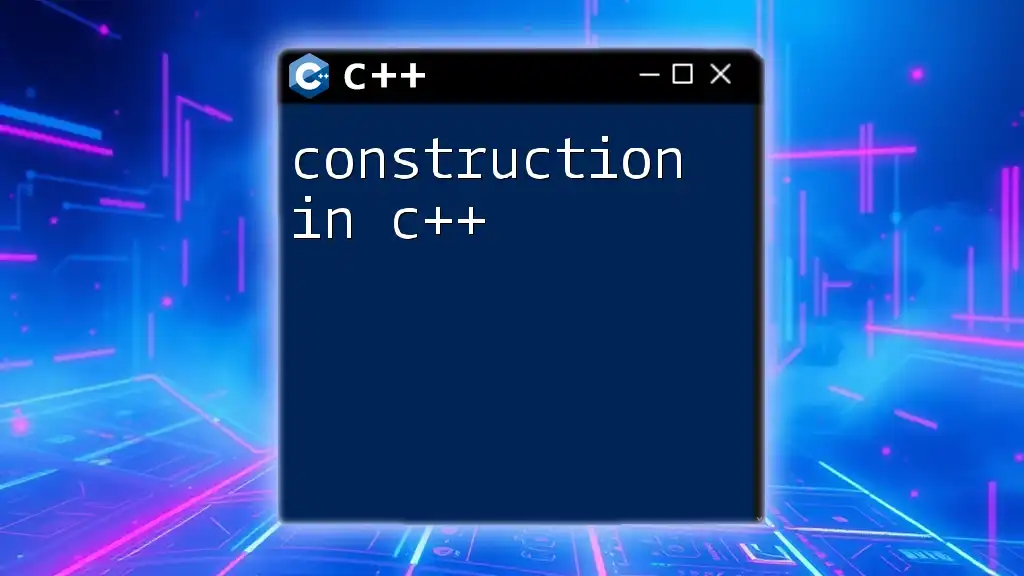
Best Practices for Using Control Structures
-
Clarity over Cleverness: Always prioritize making your code clear and readable over writing clever, convoluted logic. Often the most straightforward approach is the best choice.
-
Avoid Deep Nesting: Deeply nested structures can complicate your code and make it prone to errors. If you find yourself nesting too deeply, consider refactoring into smaller functions or changing the logic flow.
-
Use Comments: Documenting your code will help others (or even yourself later) understand the intent behind your control structures. When the logic is complex, explanations can prove invaluable.
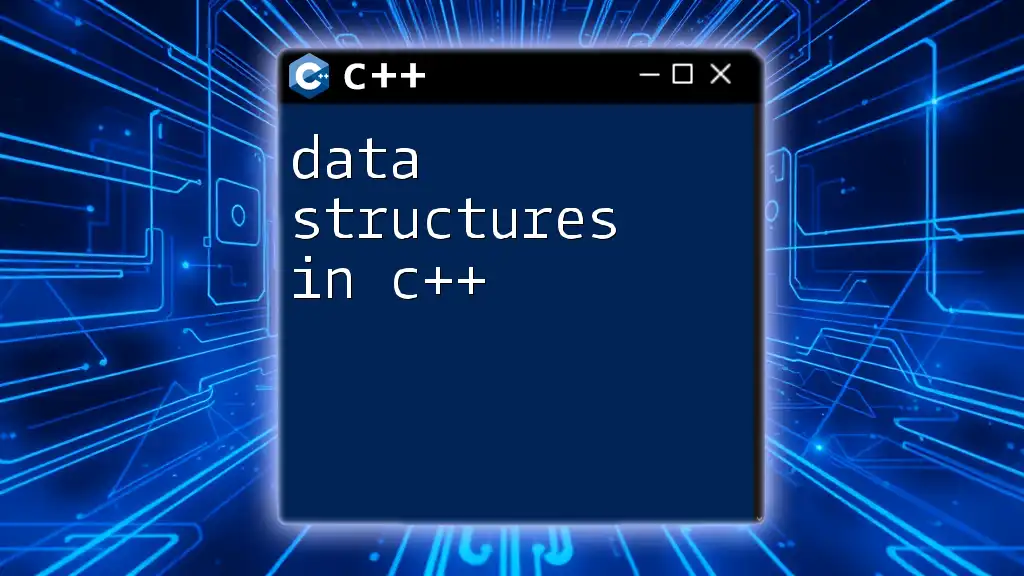
Conclusion
Control structures in C++ are essential for directing the flow of execution in a program. With a strong grasp of these structures, you will be able to implement complex logic and transform input into meaningful output. Mastering control structures empowers you to write efficient and effective code, laying a solid foundation for your programming journey.