A copy constructor in C++ is a special constructor that initializes a new object as a copy of an existing object, allowing for the transfer of data member values from one instance to another.
class MyClass {
public:
int data;
// Copy constructor
MyClass(const MyClass &obj) {
data = obj.data;
}
};
Understanding Copy Constructors in C++
What is a Copy Constructor?
A copy constructor in C++ is a special constructor that initializes a new object as a copy of an existing object of the same class. It serves a crucial role when it comes to the management of resources and memory. The main purpose of the copy constructor is to create a new instance of an object that duplicates the state of an existing instance. When you don't provide a copy constructor, C++ provides an implicit one, which may not handle resource management adequately, often leading to shallow copies.
Why Use Copy Constructors?
Copy constructors are essential for several reasons:
-
Managing Resources: When dealing with resources like dynamic memory allocation, files, or network connections, copy constructors help ensure that each object manages its own resources properly.
-
Avoiding Shallow Copy: A default shallow copy may lead to multiple objects trying to manage the same resource, resulting in undefined behavior. Copy constructors help avoid this by implementing deep copying when necessary.
For instance, if you have an object that manages a dynamic array, a shallow copy could lead to two objects trying to delete the same memory, which will cause crashes or memory leaks.
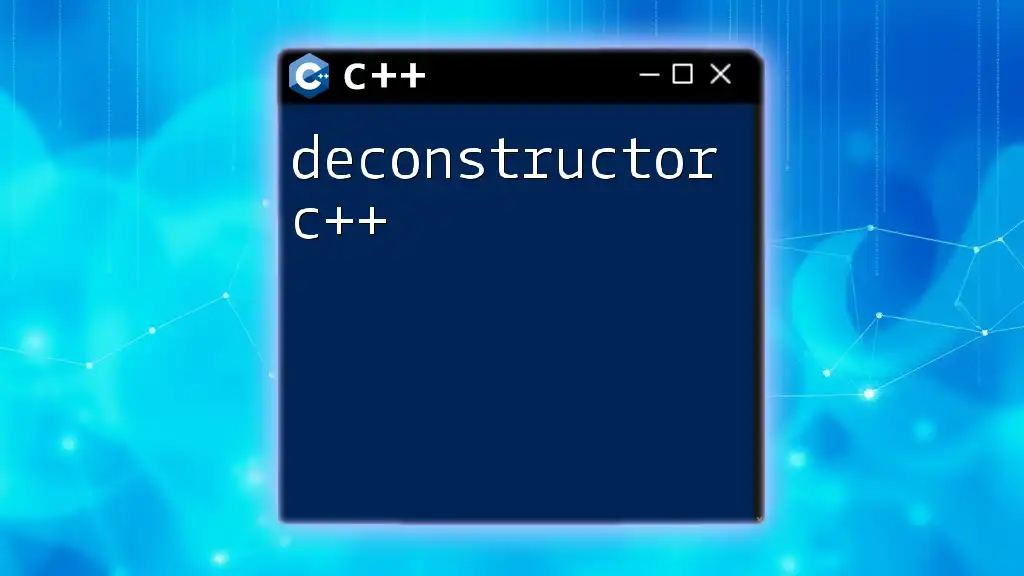
The Syntax of Copy Constructors
General Syntax
The syntax for defining a copy constructor follows this pattern:
ClassName(const ClassName &obj) { /* body */ }
Breaking down the components:
- ClassName: This represents the name of your class.
- const ClassName &obj: This parameter is a reference to an object of the same class, marked as `const` to prevent modification to the source object.
Example Code Snippet
class Sample {
public:
int data;
Sample(const Sample &obj) {
data = obj.data; // Copies the data
}
};
In this example, the `Sample` class has a copy constructor that initializes a new `Sample` object with the data from another `Sample` object.
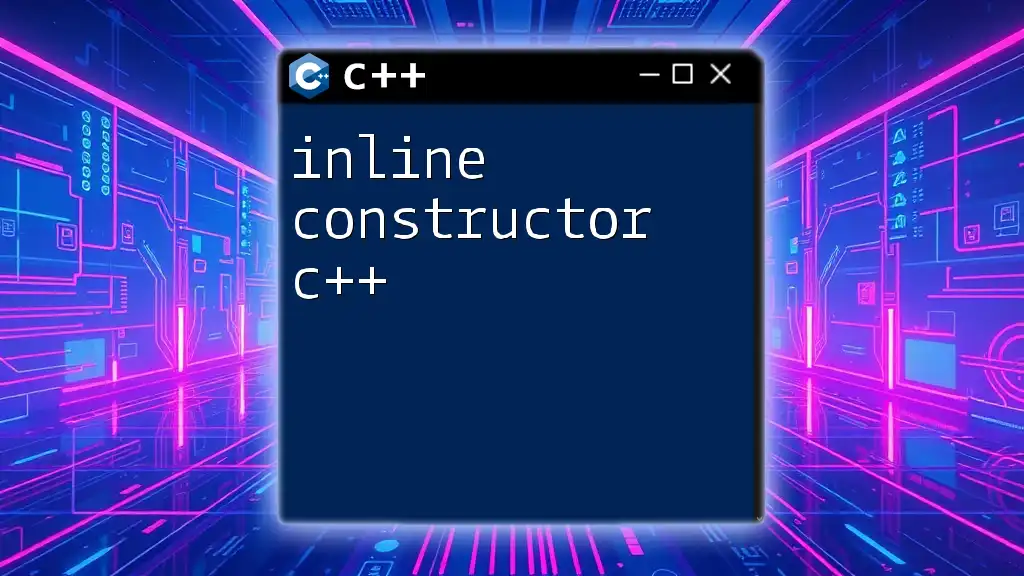
How Copy Constructors Work
Implicit vs. Explicit Copy Constructors
There are two types of copy constructors:
-
Implicit Copy Constructor: Automatically created by the compiler if you do not define your own. However, it performs a shallow copy.
-
Explicit Copy Constructor: This is a user-defined version. It should be created when your class manages resources to ensure proper copying.
Example of Implicit Copy Constructor
class Demo {
public:
int value;
Demo(int v) : value(v) {} // Parameterized constructor
};
void demoFunction() {
Demo obj1(10);
Demo obj2 = obj1; // Implicit copy constructor invoked
}
In this snippet, when `obj2` is initialized with `obj1`, the default implicit copy constructor is invoked, which results in a shallow copy of the `value` member.
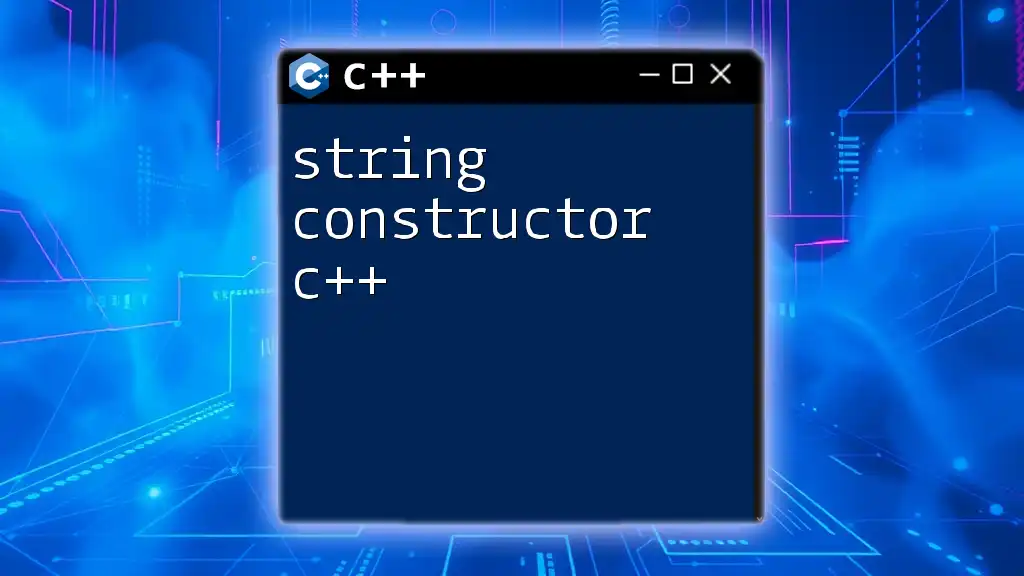
When is a Copy Constructor Called?
The copy constructor is called in several situations:
- Passing by Value: When an object is passed as an argument to a function.
- Return by Value: When an object is returned from a function.
- Object Initialization: Creating a new object from an existing one.
Example of Copy Constructor Call
Sample createSample(int val) {
Sample temp; // Default constructor
temp.data = val;
return temp; // Copy constructor is called here
}
In this example, when `createSample` returns `temp`, a copy of `temp` is created using the copy constructor.
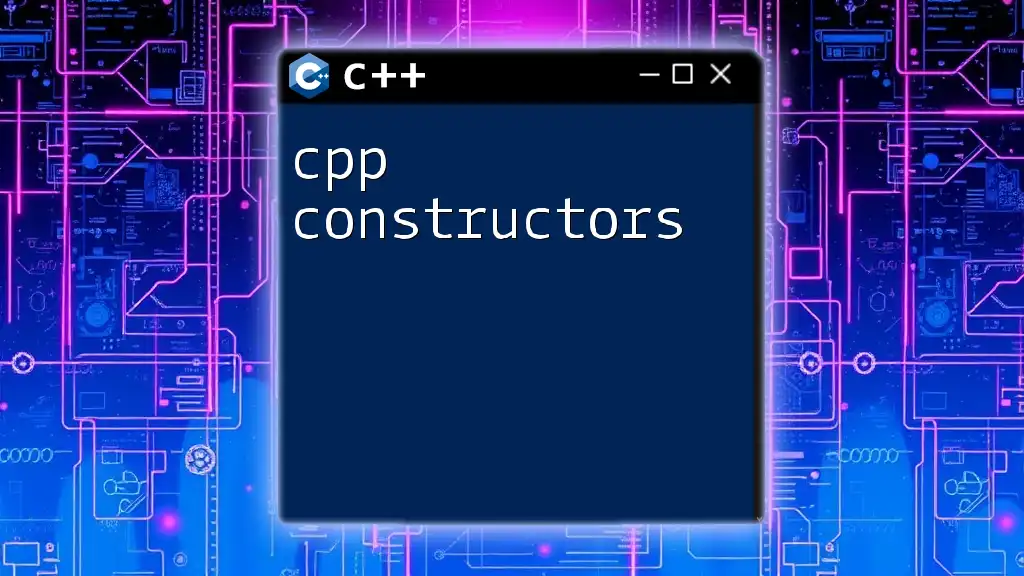
The Rule of Three in C++
Understanding the Rule of Three
The Rule of Three says that if your class defines one of the following, you should should consider defining all three:
- Destructor: Cleans up when the object goes out of scope.
- Copy Constructor: Manages resources for copying.
- Copy Assignment Operator: Handles assignment of one existing object to another.
This rule is vital, especially in classes that manage dynamic memory. If you neglect to implement this rule, you could end up with resource leaks or undefined behavior.
Example in Context
class RuleOfThree {
public:
int* data;
RuleOfThree(int size) {
data = new int[size];
}
// Copy Constructor
RuleOfThree(const RuleOfThree &obj) {
data = new int[sizeof(obj.data)/sizeof(int)]; // Allocating memory for new object
std::copy(obj.data, obj.data + sizeof(obj.data), data); // Deep copy
}
~RuleOfThree() {
delete[] data; // Cleanup memory
}
};
In this example, the copy constructor allocates new memory and copies data to ensure that each object has its own separate copy.
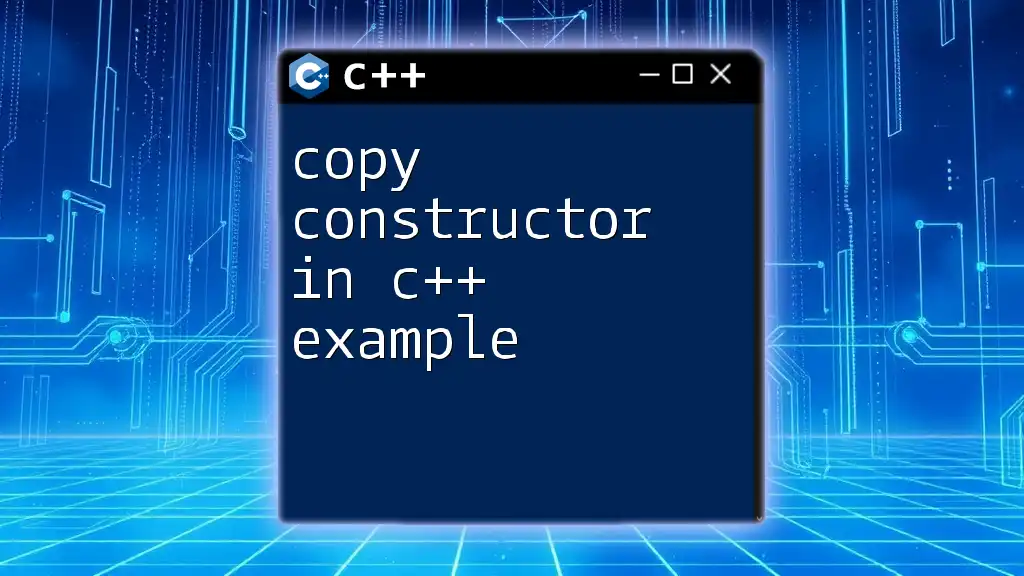
Implementing a Custom Copy Constructor
Steps to Create a Copy Constructor
- Define the Constructor: Create a constructor that takes an object of the same class as a parameter.
- Allocate Memory (if applicable): Ensure you allocate your resources for the new object.
- Copy Data/Values: Transfer the values from the source object to the new object.
Detailed Example
class MyClass {
private:
int* ptr;
public:
MyClass(int val) {
ptr = new int(val); // Dynamic allocation
}
// Custom Copy Constructor
MyClass(const MyClass &obj) {
ptr = new int(*(obj.ptr)); // Deep copy
}
~MyClass() {
delete ptr; // Free memory
}
};
In this example, the copy constructor allocates new memory for `ptr` and makes sure to copy the value from the source object `obj`, preventing any accidental resource sharing.
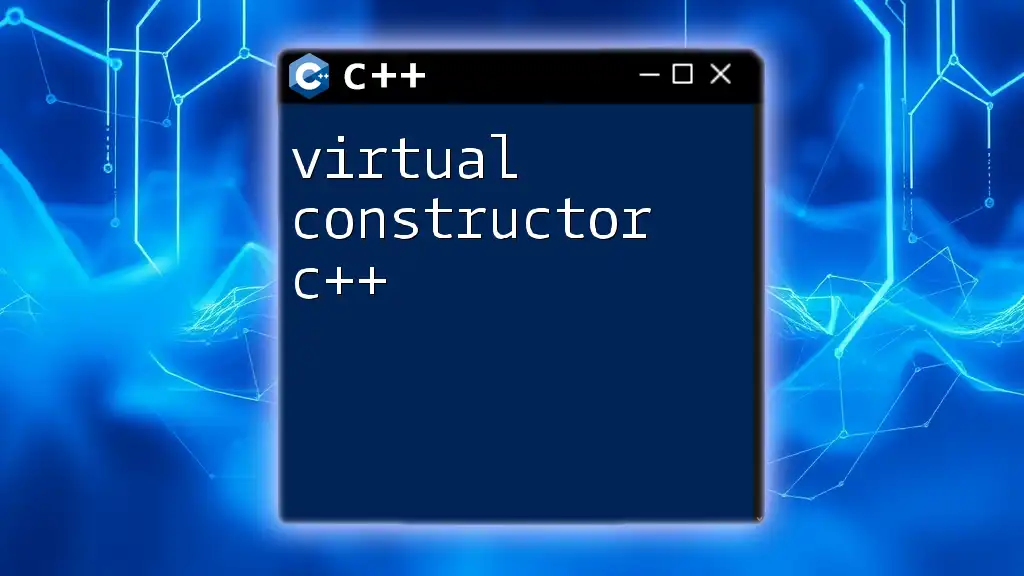
Common Pitfalls with Copy Constructors
Shallow Copy vs. Deep Copy
Understanding the difference between shallow and deep copy is crucial:
- Shallow Copy: Only copies the pointer values. If two objects are created this way, they will point to the same underlying resource.
- Deep Copy: Allocates new memory and copies the actual values into the new object. This avoids conflicts when managing resources.
Example of Pitfall
class InvalidCopy {
public:
int* arr;
InvalidCopy(int size) {
arr = new int[size]; // Dynamic allocation
}
InvalidCopy(const InvalidCopy &obj) { // Shallow Copy
arr = obj.arr; // Dangerous! Both objects now point to the same memory
}
~InvalidCopy() {
delete[] arr; // Potentially unsafe!
}
};
This scenario presents severe risks; if one object is destructed while the other is still in use, it will lead to undefined behavior—both trying to delete the same memory location.
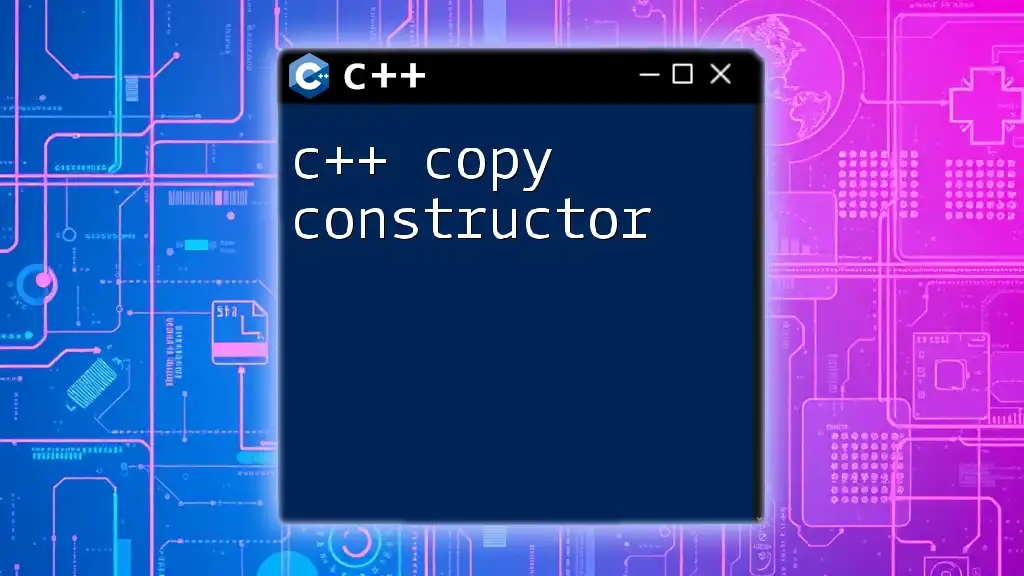
Conclusion
To summarize, copy constructors in C++ are indispensable for managing dynamic resources within classes. They help promote proper resource handling by implementing deep copies when necessary. The importance of understanding and applying the Rule of Three cannot be understated since omitting any of its components can lead to severe resource management issues. By taking care of how your classes are designed and making intentional decisions on resource management, you'll create more robust and error-free applications.
As you implement copy constructors in your applications, don’t hesitate to experiment and discover the nuances of proper resource handling. Your understanding will deepen with practice, leading to better software design. If you have any questions or need further clarification on specific points, feel free to reach out!