An overloaded constructor in C++ allows a class to have multiple constructors with different parameter lists, enabling objects to be initialized in various ways.
Here’s a code snippet demonstrating overloaded constructors:
#include <iostream>
using namespace std;
class Rectangle {
public:
int width, height;
// Default constructor
Rectangle() {
width = 0;
height = 0;
}
// Overloaded constructor with parameters
Rectangle(int w, int h) {
width = w;
height = h;
}
void display() {
cout << "Width: " << width << ", Height: " << height << endl;
}
};
int main() {
Rectangle rect1; // Calls default constructor
Rectangle rect2(10, 5); // Calls overloaded constructor
rect1.display();
rect2.display();
return 0;
}
Understanding Constructors in C++
Constructors are special member functions in C++ that are automatically invoked when an object of a class is instantiated. Their primary purpose is to initialize the object’s attributes, setting up the necessary conditions through which the object can function correctly.
Every class can have a default constructor, which is called when an object is created without any arguments, initializing variables to default values. Constructors notably help in defining the state of an object at the moment of its creation, ensuring readiness for any methods that may be called upon it.
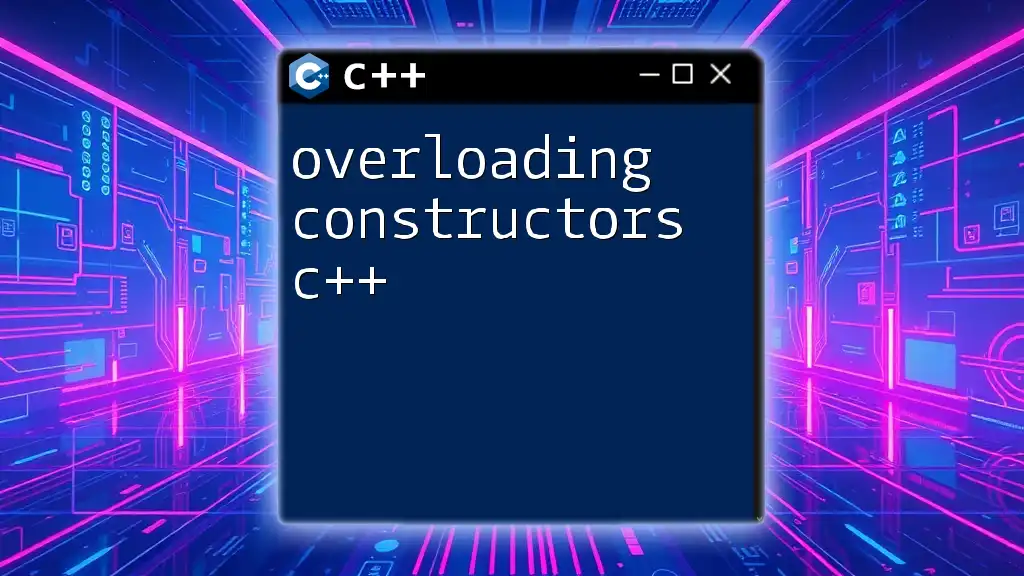
What is Constructor Overloading?
Constructor overloading refers to the practice of defining multiple constructors in a class, each having a different signature (i.e., differing in the number or type of parameters). This feature provides flexibility in instantiating objects using different sets of initial data, making the code more versatile.
Difference Between Constructor Overloading and Other Forms of Overloading
Unlike function overloading, which allows multiple functions with the same name but different parameters, constructor overloading specifically focuses on initializing class objects. The constructors allow for tailored instantiation based on specific needs, enhancing the functionality of a class.
Benefits of using constructor overloading include:
- Clarity: You can create objects in a way that’s relevant to their intended use.
- Simplicity: Developers can choose the most appropriate constructor as per necessity, leading to cleaner code.
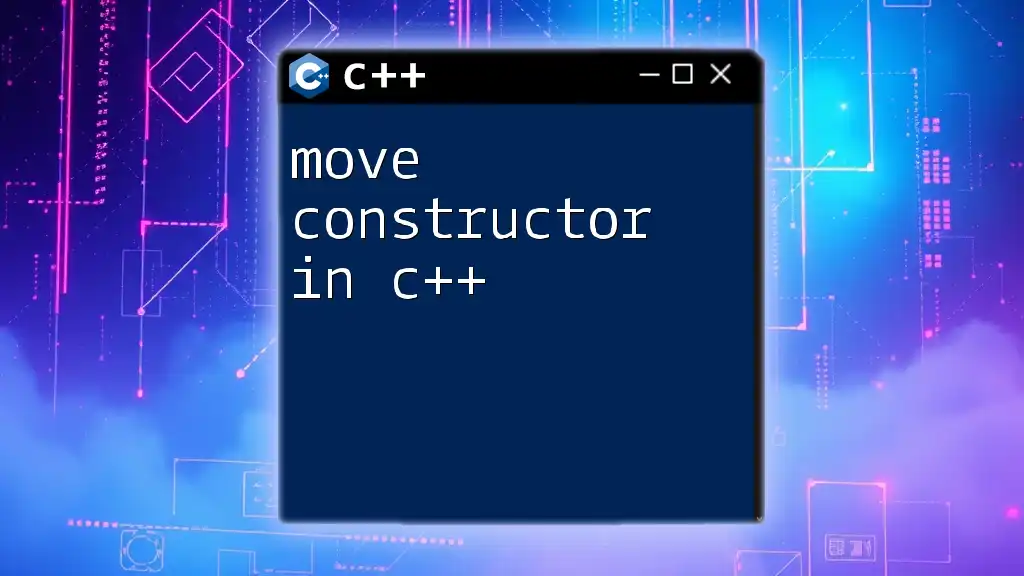
Syntax of Overloading Constructor in C++
Basic Syntax Structure
The syntax for defining overloaded constructors is straightforward. Here’s a basic format that illustrates the concept:
class Example {
public:
Example(); // Default constructor
Example(int a); // Overloaded constructor
Example(int a, double b); // Another overloaded constructor
};
How to Implement Multiple Constructors
To implement overloaded constructors effectively, each constructor must have a unique set of parameters. Here is a step-by-step guide along with an example:
- Define constructors with different numbers of parameters.
- Implement the constructors with appropriate logic based on the input parameters.
Example::Example() {
// Default constructor initializes variables to default values
}
Example::Example(int a) {
// This constructor might set a single attribute based on the provided parameter
}
Example::Example(int a, double b) {
// Here we might initialize two attributes using both parameters
}
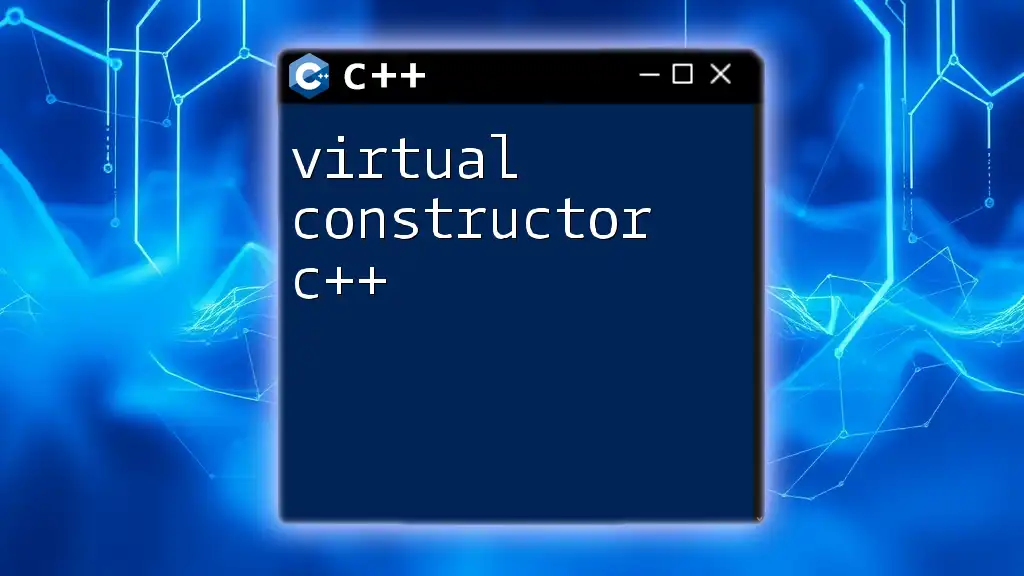
Use Cases for Overload Constructors in C++
Common Real-World Scenarios for Constructor Overloading
Constructor overloading is particularly useful in various real-world scenarios, where an object may need different initial conditions based on certain factors. For example, consider a program managing shapes. A `Rectangle` class might benefit from overloaded constructors to allow for both default and parameterized initialization of its dimensions.
class Rectangle {
public:
Rectangle(); // Default constructor
Rectangle(float width); // Single parameter constructor
Rectangle(float width, float height); // Two parameter constructor
};
Advantages of Overloaded Constructors
The advantages are manifold:
- Enhanced Readability: It’s clear what parameters are necessary for object creation, and the interface becomes self-documenting.
- Flexibility: The ability to initialize objects with varying data sets ensures more adaptability in real-world applications.
- Convenience: Developers can choose a constructor that best fits their circumstances without worrying about numerous class initialization methods.
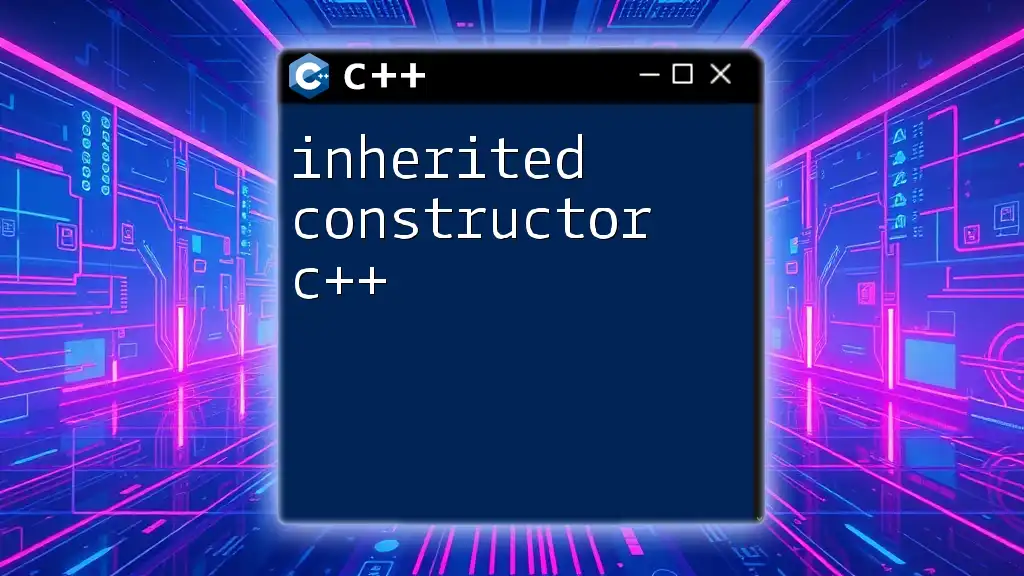
Practical Examples of Constructor Overloading
Creating a Class with Multiple Constructors
Consider the example of a `Book` class that can be instantiated in multiple ways:
class Book {
public:
Book(); // Default constructor
Book(string title); // Single parameter constructor
Book(string title, string author); // Two parameter constructor
};
In this implementation:
- The default constructor allows for a simple instantiation without parameters.
- The single parameter constructor initializes the book with a title only.
- The two-parameter constructor initializes both the title and author.
Demonstrating Constructor Overloading in Use
Here’s how you might utilize the overloaded constructors:
int main() {
Book book1; // Uses default constructor
Book book2("1984"); // Uses single parameter constructor
Book book3("Animal Farm", "Orwell"); // Uses two parameters constructor
}
In this example, three instances of the `Book` class are created with varying initial conditions. Each constructor demonstrates how overloading provides flexibility in instantiation.
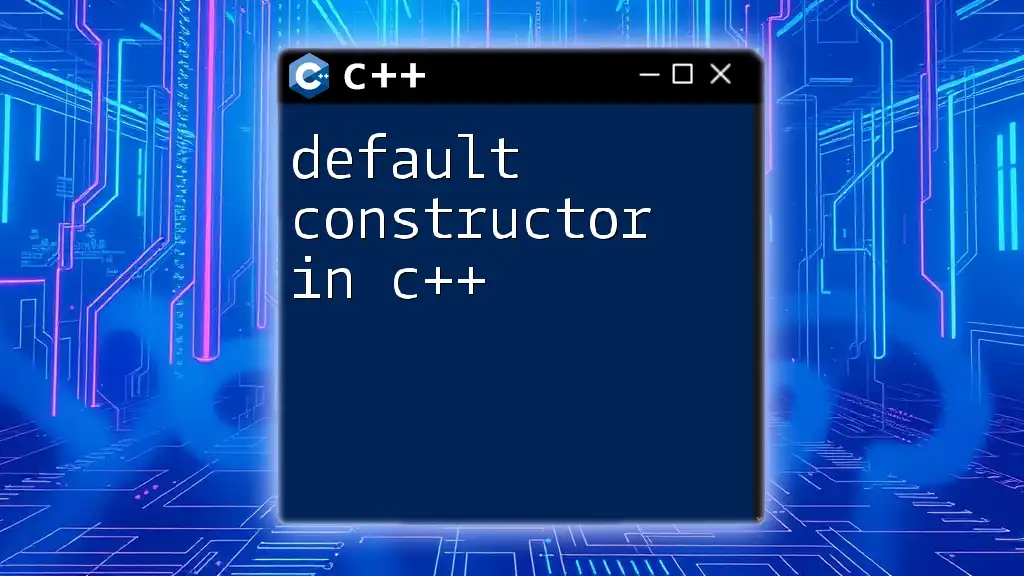
Best Practices for Constructor Overloading in C++
Tips to Follow When Overloading Constructors
When implementing overloaded constructors, adhering to best practices is essential to maintain clean and efficient code:
- Ensure Clarity: Constructor signatures should clearly communicate their purpose.
- Avoid Ambiguity: Ensure that no two constructors have the same signature to prevent confusion.
- Always Initialize Member Variables: Default values should be set to avoid uninitialized member variables, which can lead to errors.
When to Avoid Constructor Overloading
While constructor overloading is incredibly useful, there are circumstances where it might lead to complications:
- Excessive Constructors: Overloading too many constructors can lead to a confusing interface.
- Ambiguous Situations: If constructors are not distinct enough, the compiler may raise ambiguity errors, which can be very frustrating to debug.
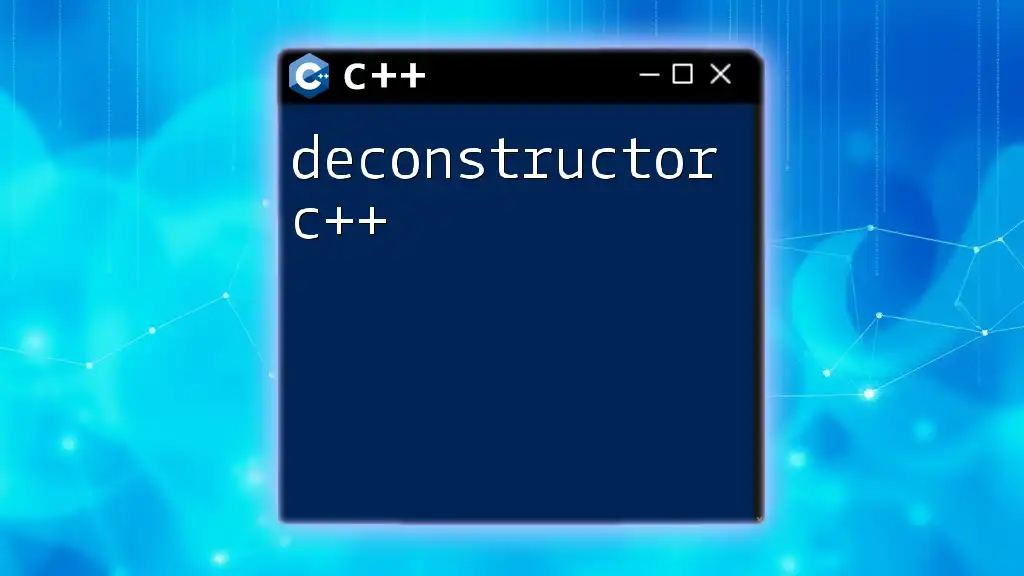
Troubleshooting Common Issues with Constructor Overloading
Common Mistakes and How to Fix Them
During implementation, developers may encounter various issues with constructor overloading. Here are some common problems along with solutions:
-
Ambiguous Overloads: When overloaded constructors accept parameters of similar types, the compiler can struggle to decide which constructor to use.
Example:
Book myBook("1984"); // If both constructors take a string, compiler may be unable to decide
Fix: Ensure constructors have unique signatures or types.
-
Default Arguments Causing Confusion: Using default parameters in overloaded constructors can also create ambiguity.
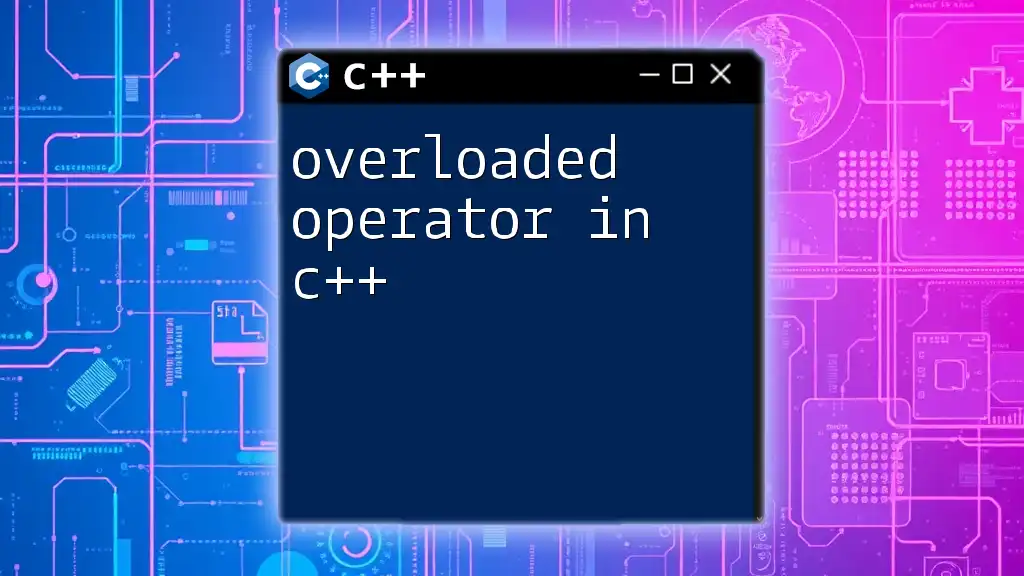
Conclusion
Constructor overloading in C++ is a powerful feature that allows developers to create versatile objects with different initialization scenarios. By using overloaded constructors, developers can ensure flexibility in their code while maintaining readability and clarity. Embracing best practices can lead to effective use of this capability, ensuring the robustness and reliability of your C++ applications.
Further Reading and Resources
For those interested in diving deeper into C++ and constructor overloading, consider exploring various programming books focused on C++ best practices and advanced topics. Online tutorials and forums are also excellent resources for practical insights and community support.