A default constructor in C++ is a special type of constructor that initializes objects without any parameters, either by providing default values or leaving them uninitialized.
Here's a simple example:
class MyClass {
public:
MyClass() { // Default constructor
// Initialization code here
}
};
What is a Default Constructor?
A default constructor is a special type of constructor in C++ that can be called without any parameters. It provides a way to initialize an object to a default state. In the context of the C++ programming language, the term "default" signifies that this constructor will automatically initialize an instance of a class without requiring any arguments from the user.
Key characteristics of a default constructor include:
- No Parameters: A default constructor does not take any parameters. This allows for implicit creation of objects.
- Public Access: It is generally defined as a public member function, enabling objects to be instantiated freely.
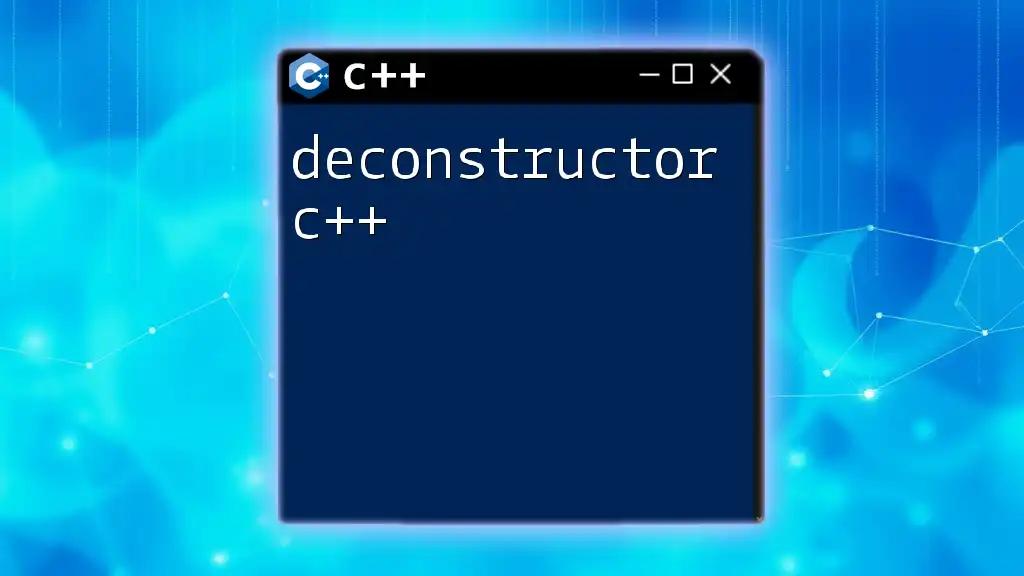
Types of Constructors
C++ Constructors Overview
C++ supports various types of constructors to cater to different initialization needs.
- Default Constructor: Initializes an object with no parameters.
- Parameterized Constructors: Takes parameters to initialize an object with specific values.
- Copy Constructors: Creates a new object as a copy of an existing object.

Syntax of a Default Constructor
Basic Structure
The syntax for declaring a default constructor is straightforward:
class MyClass {
public:
MyClass() {
// Default constructor code
}
};
In this example, `MyClass` has a default constructor which simply contains a block of code that executes when an object of `MyClass` is instantiated.

How Default Constructors Work
Invocation of the Default Constructor
A default constructor is called automatically when an object is created without supplying arguments. For instance:
MyClass obj; // Default constructor called
Upon creation of `obj`, the default constructor will be executed to initialize the object with default values.
Implicit and Explicit Default Constructors
C++ handles default constructors in two ways:
- Implicit Default Constructor: If no constructors are defined, the C++ compiler provides a default constructor that performs no initialization. For example:
class Implicit {
public:
int x; // Automatically initialized to 0 if no explicit constructors are defined
};
- Explicit Default Constructor: This is user-defined and can contain initialization logic. Here's an example:
class Explicit {
public:
Explicit() {
x = 0; // Initializes x to 0
}
};
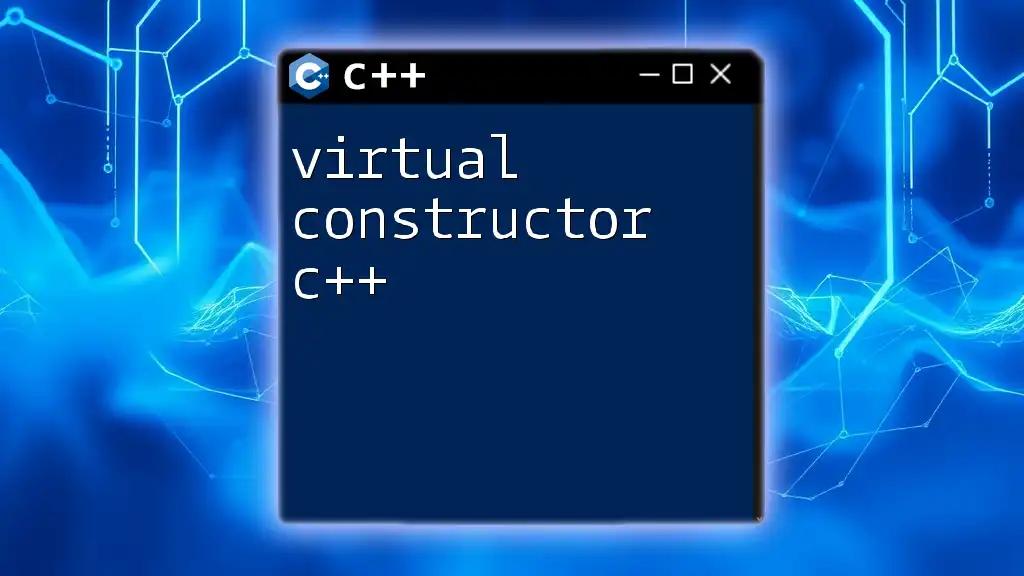
Default Constructor with Class Members
Initializing Member Variables
Using a default constructor is crucial for initializing member variables. Without proper initialization, these variables may hold garbage values, leading to unpredictable program behavior.
Consider this class where the member variables are explicitly initialized in the constructor:
class MyClass {
private:
int x;
double y;
public:
MyClass() : x(0), y(0.0) {
// Member variables initialized
}
};
Here, both `x` and `y` are initialized to `0` and `0.0`, respectively, ensuring the object's state is defined.

Benefits of Using Default Constructors
Simplified Object Creation
One of the principal advantages of a default constructor is the simplification it brings to object creation. It allows programmers to create instances without the need for any parameters, making code cleaner and easier to read.
Enhanced Readability and Maintenance
When certain initialization tasks are consolidated into a default constructor, it significantly enhances the readability of the code. Future maintainers of the code will also find it easy to grasp the defined behavior of objects without diving deeply into initialization details.

Challenges and Limitations of Default Constructors
Overhead in Automatic Initialization
While default constructors simplify object creation, there can be performance overhead when they perform unnecessary automatic initialization. This is particularly true if a class contains many member variables that require initialization.
Handling Complex Classes
In classes dealing with pointers or dynamic memory, careful construction is necessary to avoid memory leaks. A default constructor in such cases might need specific handling for memory management.
For example, consider the following class:
class ComplexClass {
private:
int* data;
public:
ComplexClass() : data(nullptr) {
// Pointer initialization to avoid dangling pointers
}
~ComplexClass() {
delete data; // Destructor to avoid memory leaks
}
};
Here, the default constructor sets `data` to `nullptr`, ensuring that it starts with a safe state, and the destructor ensures proper memory management.

When to Use Default Constructors
Default constructors are particularly useful in scenarios where you want to create objects with a generic state or when objects are expected to be initialized later. They are also recommended when designing classes that interact closely with containers like vectors or lists, ensuring that a collection of objects can be easily instantiated.
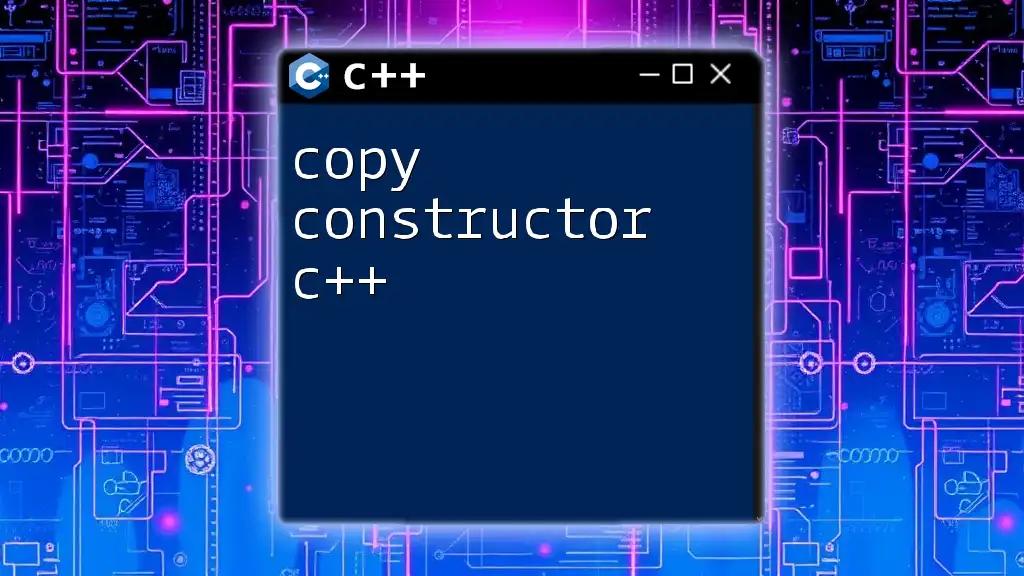
Conclusion
The default constructor in C++ plays a pivotal role in ensuring that objects are automatically initialized to a default state. Understanding default constructors empowers C++ programmers to write cleaner, more maintainable code and effectively handle the complexities associated with modern programming.
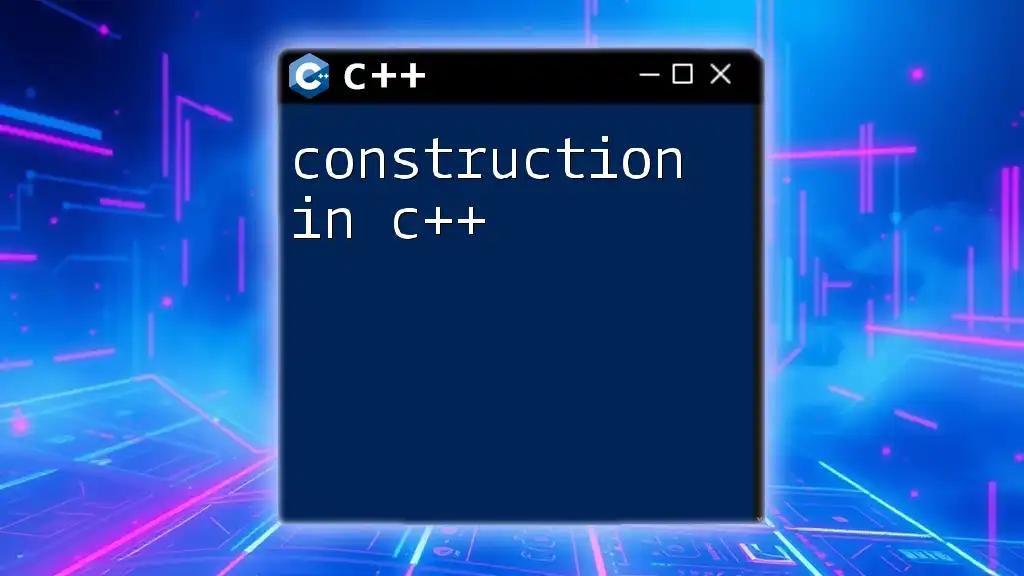
FAQs
What happens if no constructor is defined?
If no constructors are defined in a class, the C++ compiler automatically generates a default constructor for you. This compiler-generated constructor will initialize all member variables to a standard default state.
Can a class have multiple default constructors?
A class cannot have multiple default constructors because they are defined as constructors with no parameters. However, a class can have variations of parameterized constructors by overloading, but only one default constructor can exist.
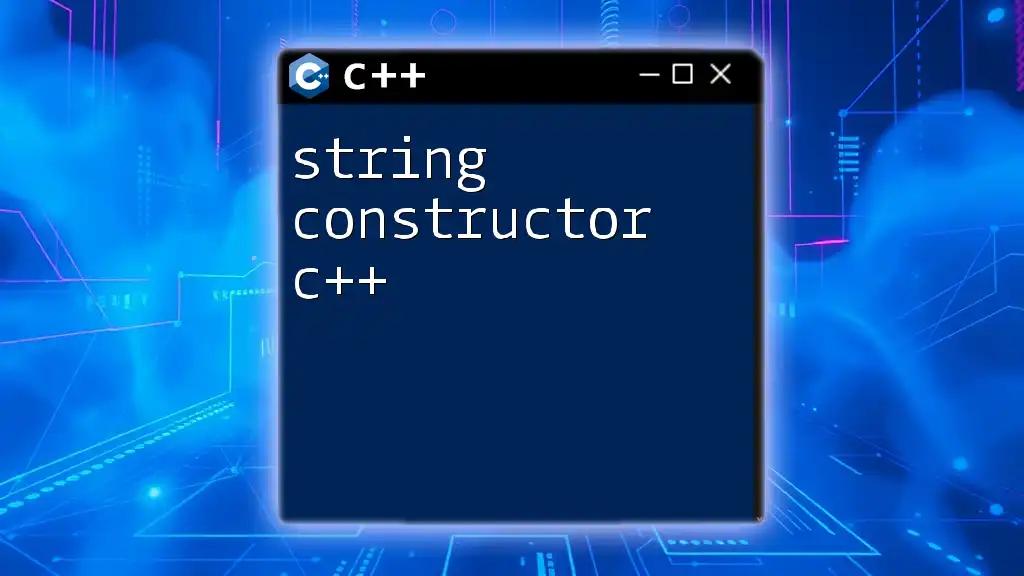
Call to Action
Dive deeper into the world of C++ constructors! Explore our additional resources and tutorials to further enhance your understanding and skills in mastering C++ programming.