In C++, a default destructor is automatically provided by the compiler when no destructor is explicitly defined, and it is responsible for releasing resources allocated by the object during its lifetime.
Here’s a code snippet illustrating a default destructor:
#include <iostream>
class MyClass {
public:
int value;
// No explicit destructor defined; the compiler provides a default one
};
int main() {
MyClass obj;
obj.value = 10;
std::cout << "Value: " << obj.value << std::endl; // Outputs: Value: 10
return 0; // Default destructor is called here
}
What is a Destructor?
In C++, a destructor is a special member function that gets invoked when an object of a class starts to go out of scope or is explicitly deleted. The primary purpose of a destructor is to free resources that the object may have acquired during its lifetime. Without destructors, resources could remain allocated, leading to memory leaks.
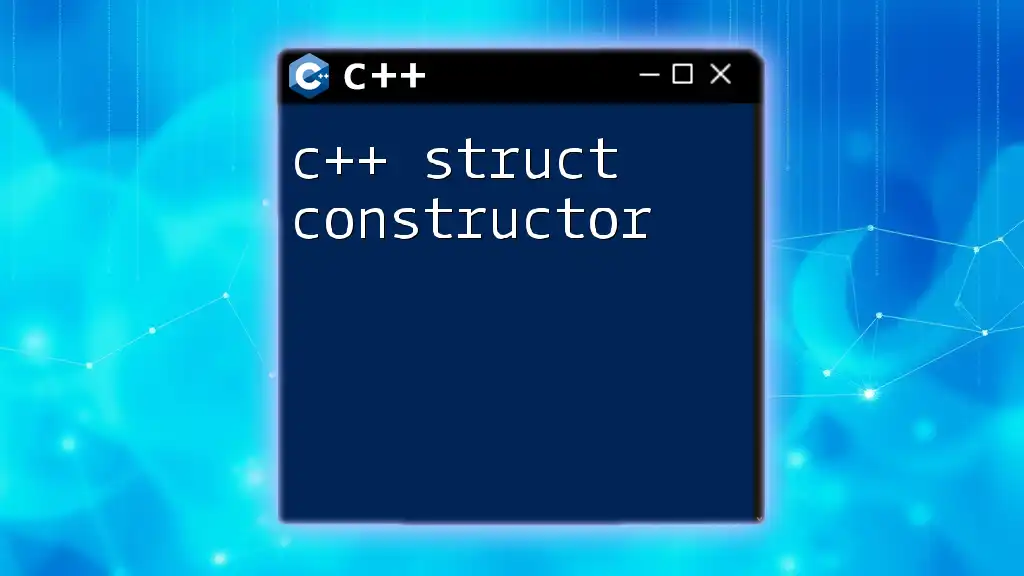
Understanding Default Destructor in C++
A default destructor is a destructor that the C++ compiler automatically generates when no user-defined destructor is provided. The compiler creates a destructor that performs a basic cleanup, which typically involves deallocating memory for primitive data types but does not automatically manage the memory of dynamically allocated resources, such as pointers to other objects.
When a class does not explicitly include a destructor, the compiler will generate one that executes default cleanup for each of the class’s non-static member variables.
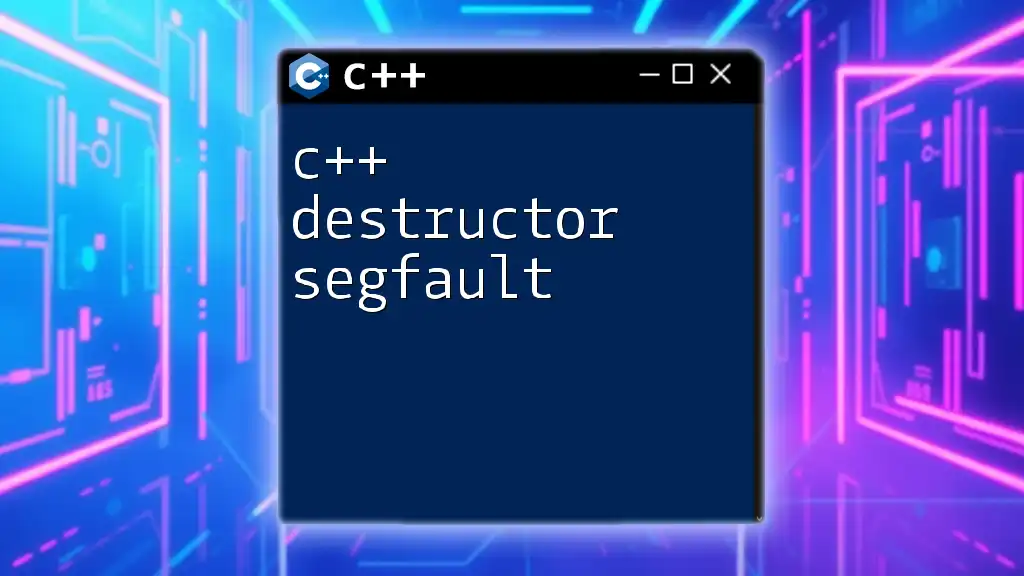
Characteristics of Default Destructors
Automatic Generation by the Compiler
The compiler automatically generates a default destructor, provided that the class does not specify any destructor of its own. This mechanism ensures that even straightforward objects have a way to clean up.
Behavior of Default Destructor
The default destructor operates far more simply than a custom destructor, providing a generic cleanup service. When a default destructor is invoked, it:
- Cleans up non-static members of the class.
- Calls the destructors of base classes (if any).
- Leaves certain resources, such as dynamic memory or file handles, untouched unless managed properly.
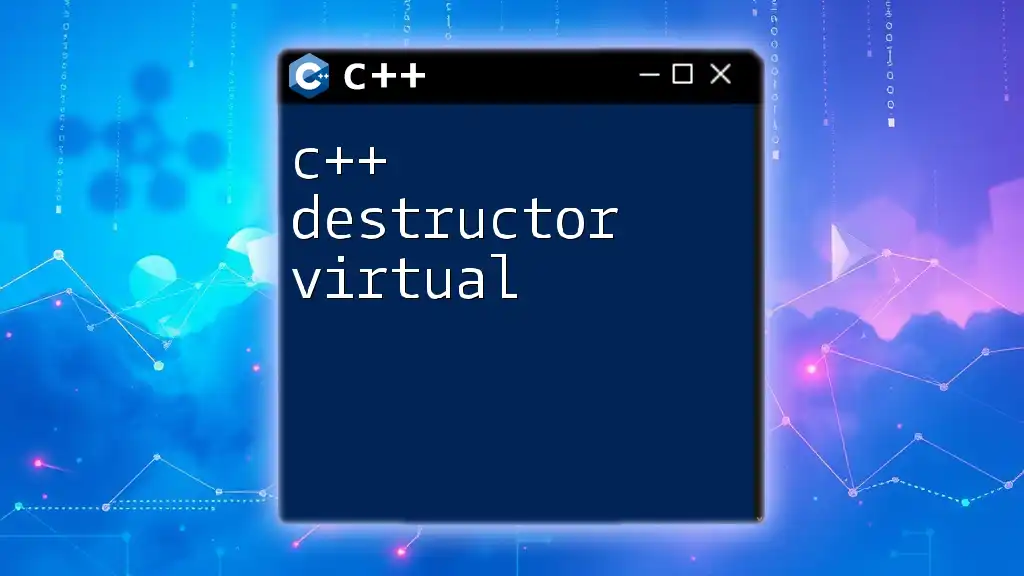
Syntax of Default Destructor
General Syntax
The syntax for defining a default destructor in C++ is straightforward. It has the same name as the class but is prefixed with a tilde (~) symbol.
Example Implementation
Consider the following code snippet illustrating a class with a default destructor:
class Example {
public:
Example() {
// Constructor code
}
~Example() {
// Default destructor—no extra cleanup required
}
};
Here, the `~Example` is the default destructor. When an object of this class is destroyed, it automatically performs cleanup of its members.
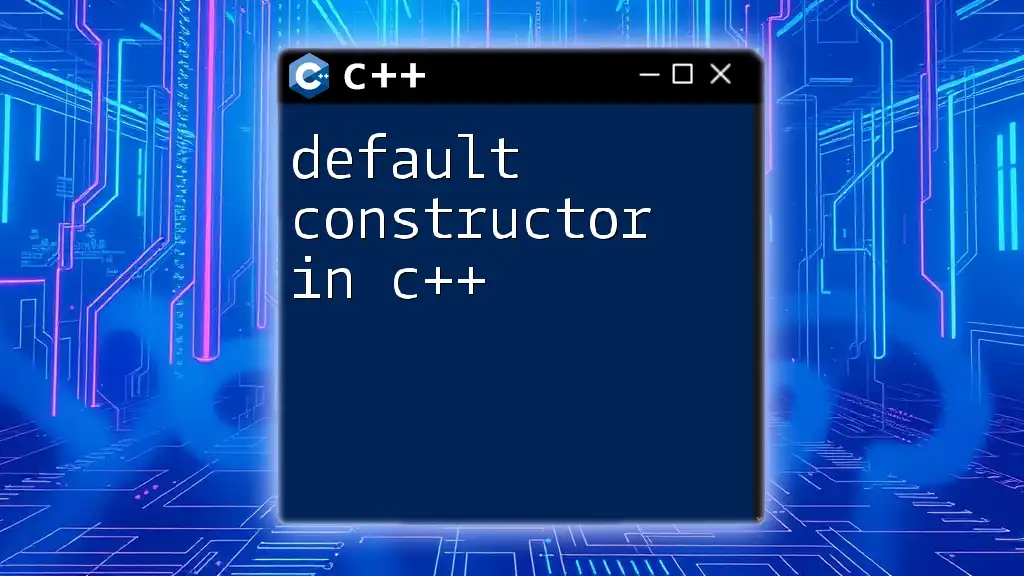
When to Use Default Destructor
Suitable Scenarios for Using Default Destructors
Default destructors are suitable for classes that manage simple or built-in data types where no special resource management is required. These scenarios often include:
- Classes with no pointers to dynamically allocated memory.
- Classes where resources are managed outside of the class.
Pros and Cons of Using Default Destructors
Pros:
- Simplicity: By relying on the default destructor, developers can avoid writing boilerplate code.
- Automatic management: The default destructor is automatically invoked, reducing the risk of forgetting to clean up resources.
Cons:
- Limitations: It does not handle dynamically allocated memory or complex resource management, leading to possible memory leaks.
- Less control: When using default destructors, you cannot control the order in which resources are released.
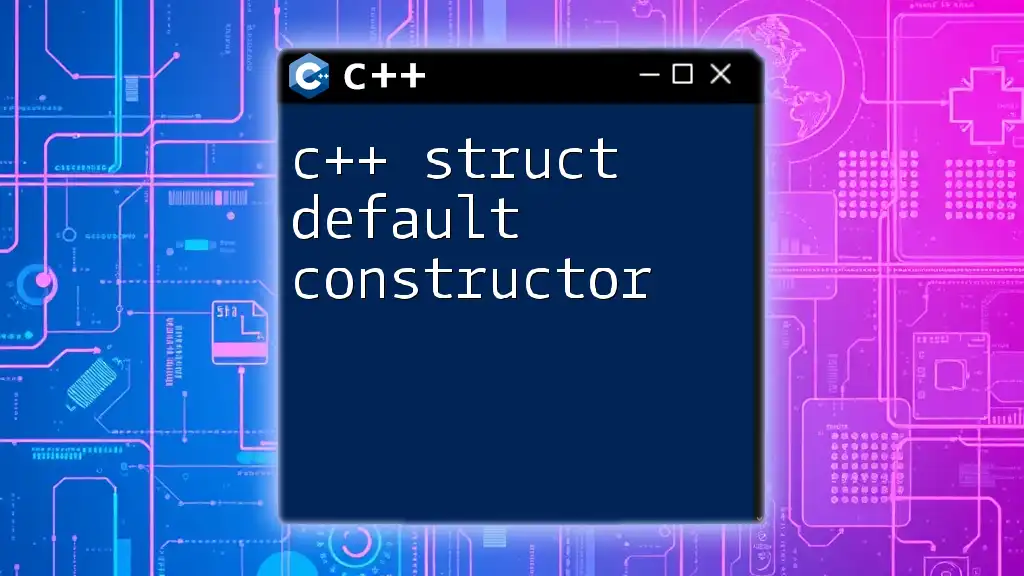
Custom vs Default Destructor
When to Implement a Custom Destructor
While default destructors are useful, there are instances when a custom destructor is necessary. Implement a custom destructor when:
- Your class manages dynamic memory allocations.
- You hold onto resources like file handles or network connections.
Example Comparison
To show the difference, consider the following example of a class using a custom destructor:
class CustomDestructor {
public:
int* data;
CustomDestructor() {
data = new int[10]; // Dynamic memory allocation
}
~CustomDestructor() {
delete[] data; // Custom cleanup to avoid memory leak
}
};
In this example, the custom destructor explicitly frees the dynamically allocated memory, something the default destructor would not handle.
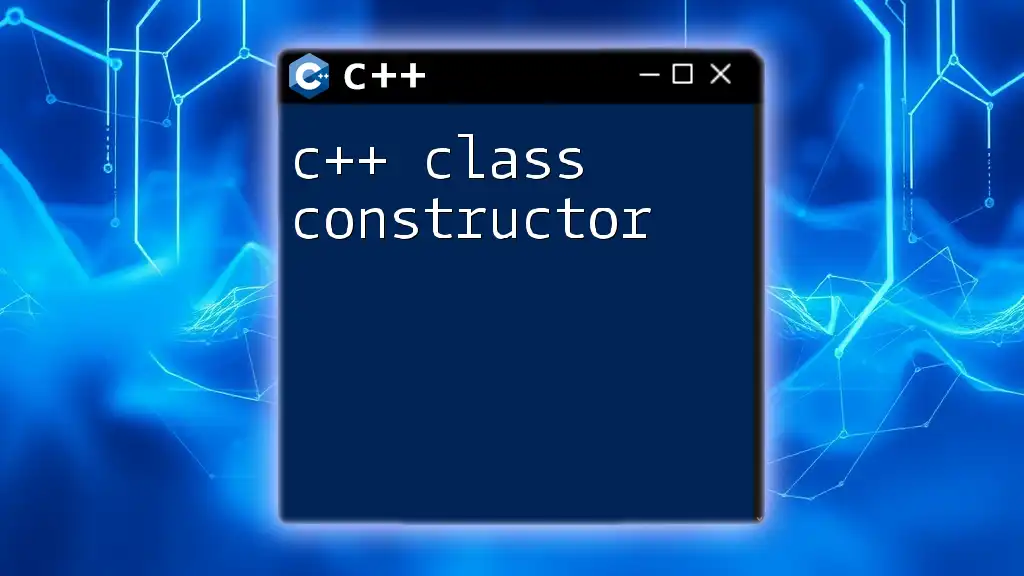
Managing Dynamic Memory with Default Destructor
Role of Default Destructors in Dynamic Memory Management
Default destructors do not manage dynamically allocated memory. If a class has pointers that point to dynamically allocated memory, the default destructor will not call `delete` for those pointers, leading to memory leaks.
Common Issues with Default Destructors
Here are some common issues that can arise when relying on a default destructor in dynamic memory contexts:
- Memory Leaks: When dynamic memory is allocated but not properly released in the destructor.
- Dangling Pointers: If the destructor manages multiple dynamic objects, and one of them is released while still being accessed by another.
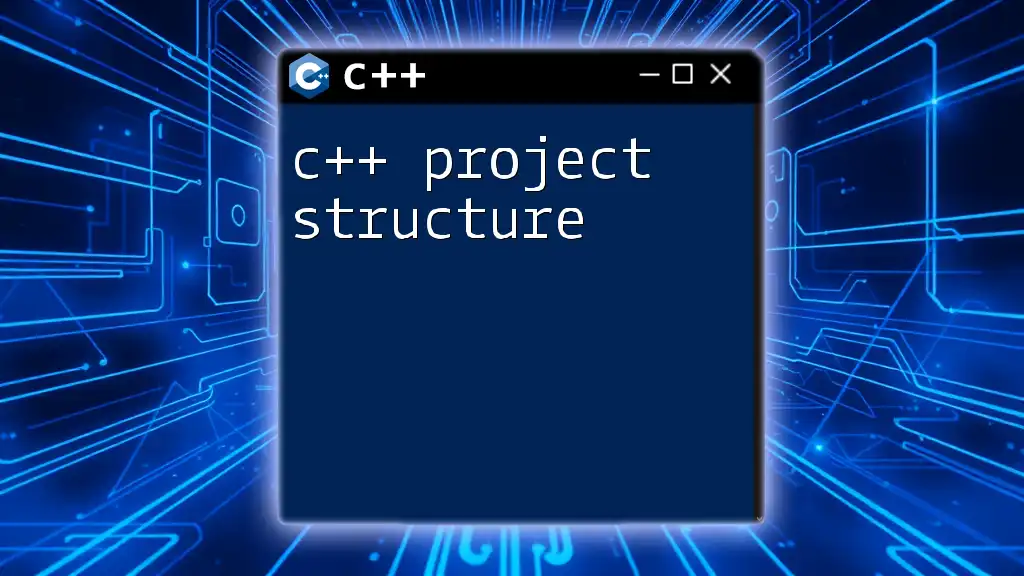
Best Practices When Using Default Destructors
General Guidelines
Consider the following best practices:
- Trust the default behavior for classes containing only basic data types.
- Implement custom destructors for classes managing dynamic memory, ensuring proper resource cleanup.
Testing and Debugging Destructors
To ensure destructors are functioning as expected:
- Use debugging tools such as Valgrind to detect memory leaks.
- Perform thorough testing to confirm that destructors are called at the correct times, especially in complex class hierarchies.
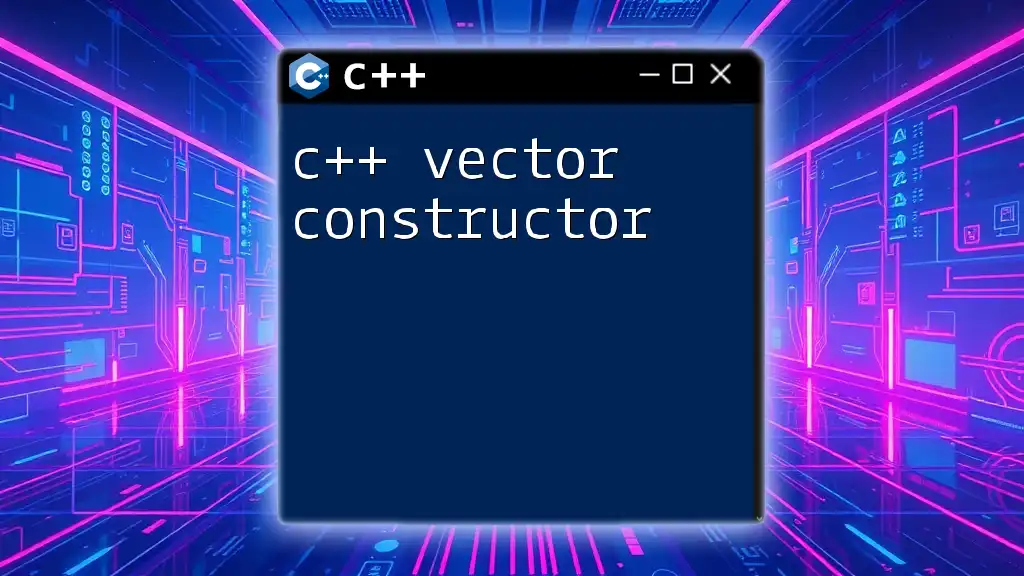
Conclusion
In wrapping up, understanding the C++ default destructor is crucial for effective resource management in your applications. By leveraging default destructors where simple cleanup is needed and implementing custom destructors for more complex resource handling, you can manage memory safely and effectively. Always remember to evaluate your resource management needs and choose the appropriate destructor strategy accordingly.
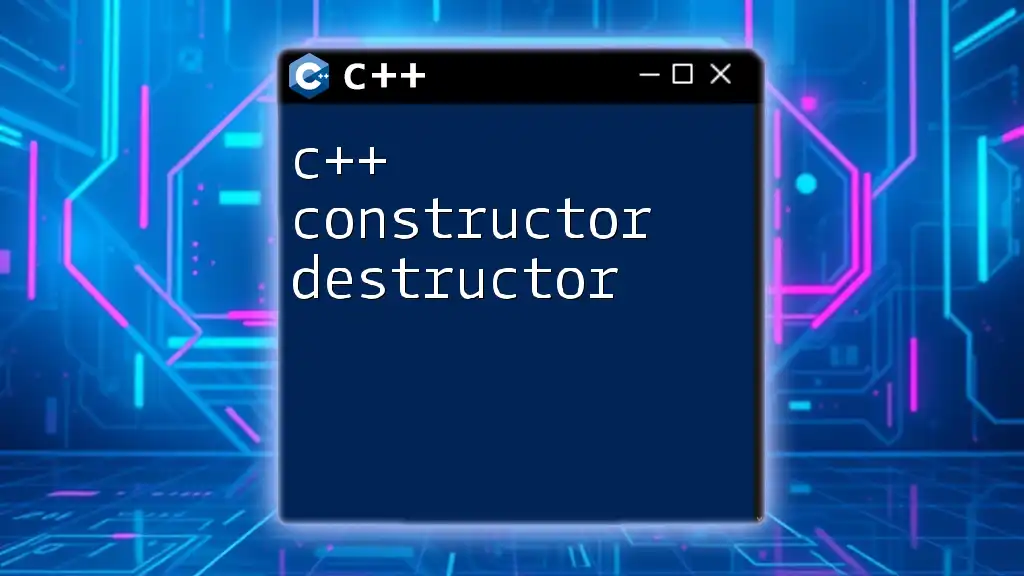
Additional Resources
For further reading and resources, you can consult the following:
- C++ Documentation on Destructors
- Community forums focused on C++ programming for interactive discussions and support
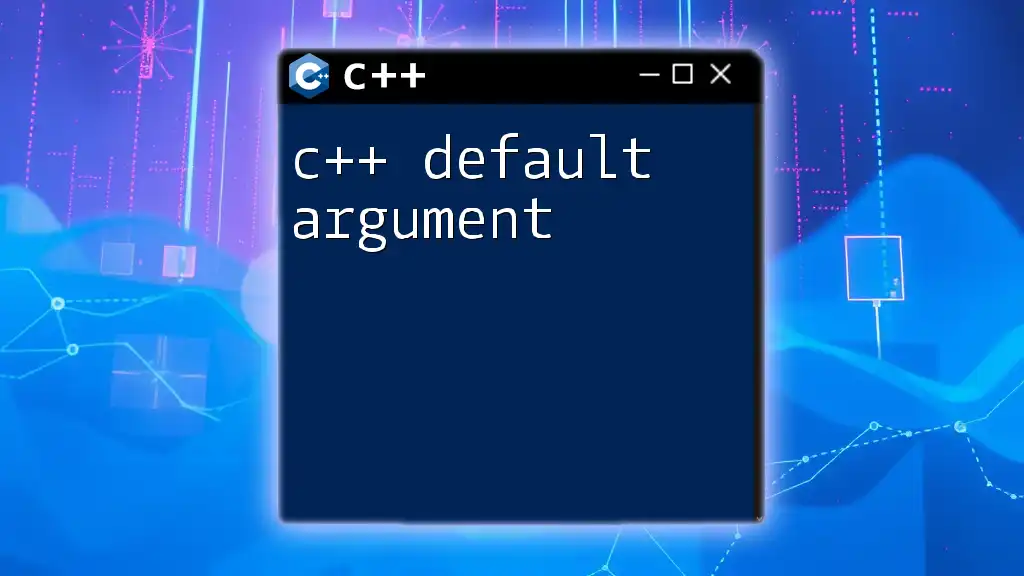
FAQ Section
Common Questions About Default Destructors
-
What happens if no destructor is defined? If no destructor is defined, the compiler provides a default destructor, which will clean up non-static members.
-
Can a default destructor be explicitly called? No, default destructors are called automatically by the compiler when an object goes out of scope or is deleted.
-
How do default destructors work with inheritance? Default destructors will automatically call the destructors of base classes, ensuring proper cleanup in class hierarchies.