The C++ default copy constructor creates a new object as a copy of an existing object, performing a member-wise copy of the object's attributes.
class MyClass {
public:
int value;
// Default copy constructor
MyClass(const MyClass &other) = default; // or simply do not define it
};
Understanding Copy Constructors
What is a Copy Constructor?
A copy constructor in C++ is a special type of constructor that initializes a new object as a copy of an existing object. Its primary role is to create a new object that is a duplicate of an existing instance, ensuring that the new object retains the same data values as the original.
Syntax of Copy Constructors
The general syntax for defining a copy constructor is as follows:
ClassName(const ClassName& other);
In this definition, `ClassName` represents the class name, and `other` is a reference to an instance of that class.
This syntax differs from other constructors, which may take different constructors or parameters specific to initializing the object.
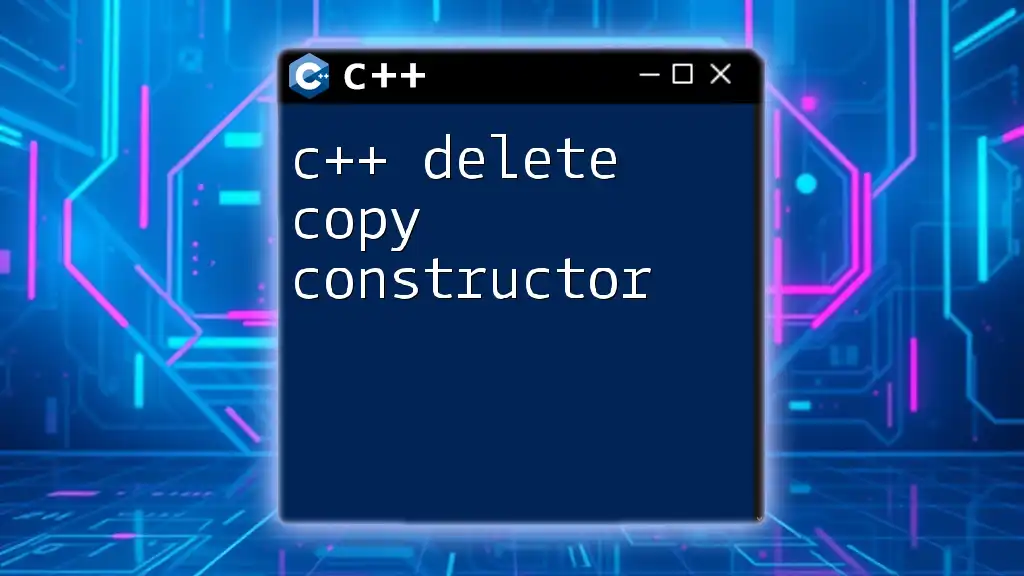
The Default Copy Constructor
Definition of Default Copy Constructor
The default copy constructor is automatically provided by the C++ compiler if the programmer does not define one. This constructor performs a member-wise copy of the class members from the source object (the object being copied) to the new object.
Characteristics of the Default Copy Constructor
The default copy constructor is known for performing a shallow copy, which means it copies the values of all member variables directly from the source object to the destination object. However, it's essential to recognize the scenarios where this behavior might be problematic:
-
Shallow copy versus deep copy: Shallow copying does not create copies of dynamically allocated memory, which can lead to issues like double deletion or memory leaks if both objects try to free the same memory.
-
What members are copied: All primitive data types (like integers and floats) are copied directly, while pointers are copied by reference, which means both objects will point to the same memory location.
-
Why the default copy constructor may lead to issues: If a class manages resources such as dynamic memory, file handles, or network connections, the default copy constructor can introduce bugs related to resource management, including segmentation faults and data corruption.
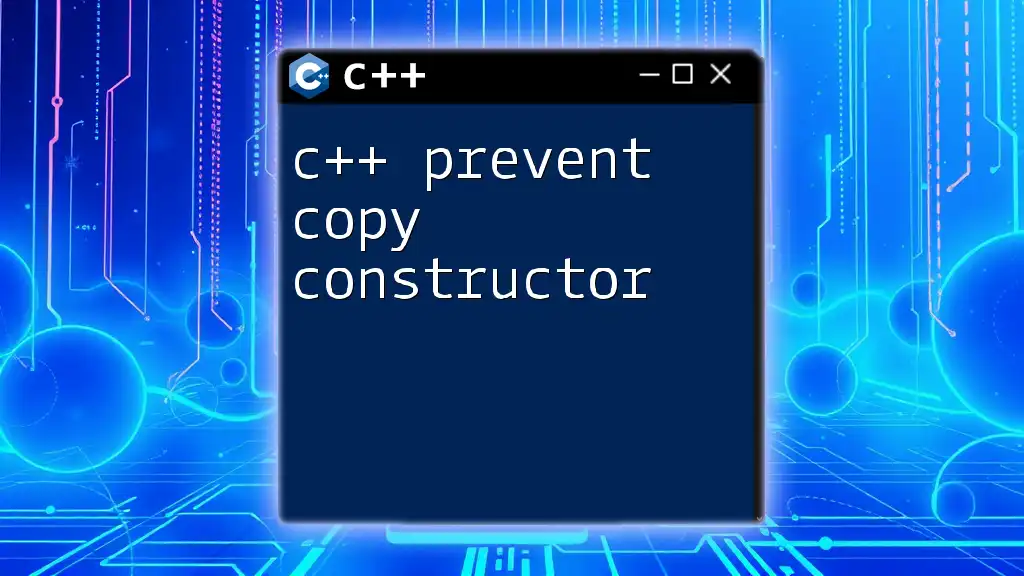
When is the Default Copy Constructor Used?
Implicit Copy Construction
The default copy constructor is invoked implicitly in various situations. One such case is when an object is passed by value:
void displayObject(MyClass obj) {
// Function logic
}
In this instance, when the function `displayObject` is called, a copy of the passed object is created using the default copy constructor.
Explicit Copy Construction
The default copy constructor can also be explicitly invoked by creating a new object based on an existing one:
MyClass obj1;
MyClass obj2 = obj1; // Invokes the default copy constructor
Here, `obj2` is initialized as a copy of `obj1`, immediately calling the default copy constructor.
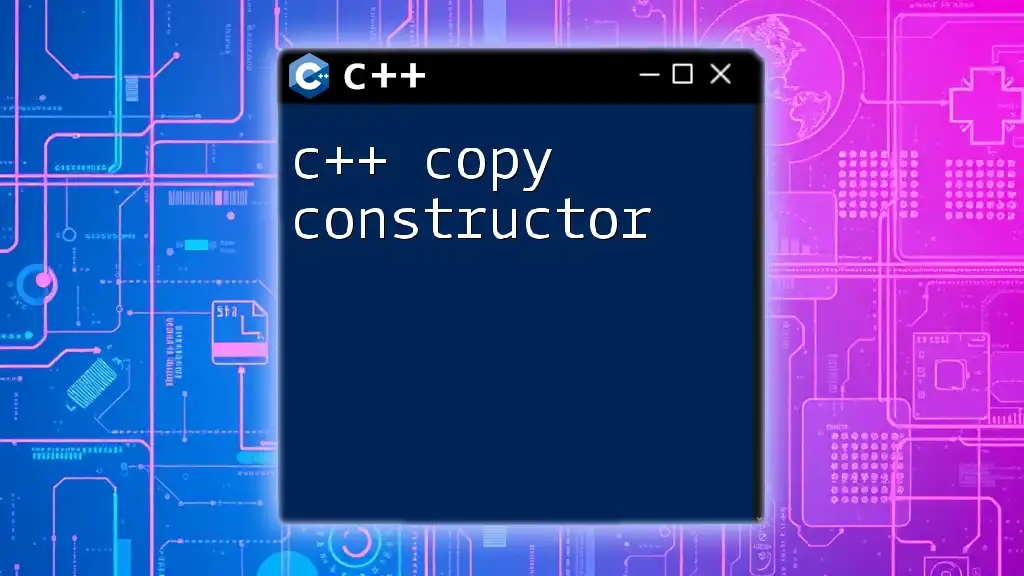
Limitations of the Default Copy Constructor
Shallow Copy Challenges
The use of the default copy constructor can lead to several pitfalls, particularly when dealing with dynamic memory allocation. For instance, consider the following class:
class MyClass {
public:
int* data;
MyClass(int value) {
data = new int(value);
}
~MyClass() {
delete data;
}
};
In this example, if you create an instance of `MyClass` and then use the default copy constructor, both objects will point to the same memory location. When the destructor is called for each object, it will try to free the same memory twice, leading to undefined behavior.
Addressing Limitations
When a class incorporates dynamic memory, implementing a custom copy constructor becomes necessary. This ensures that each object has its copy of the allocated memory.
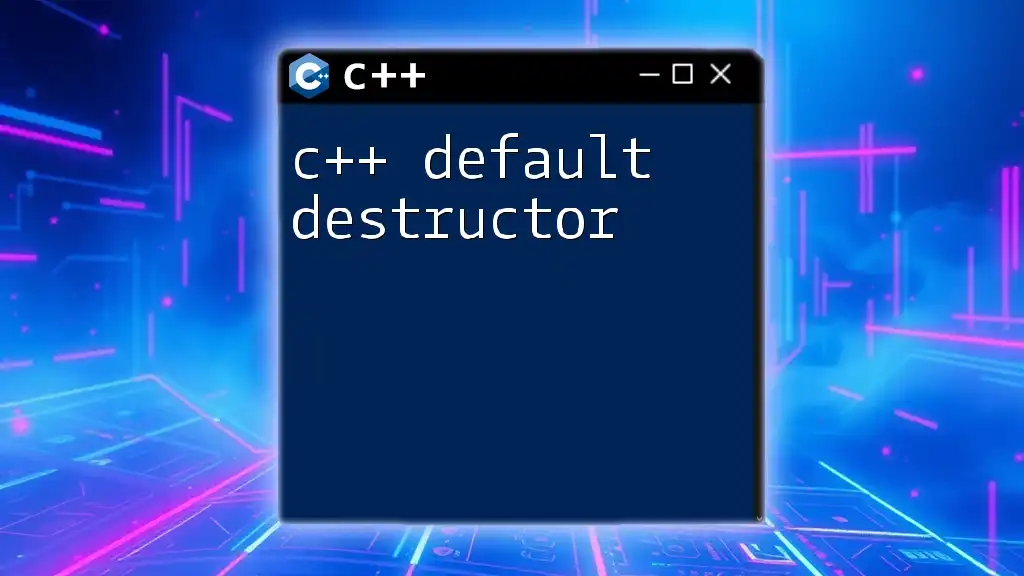
Overriding the Default Copy Constructor
When to Implement a Custom Copy Constructor
Programmers should implement a custom copy constructor when their class allocates dynamic resources or if they require a specific copy behavior that is not satisfied by the compiler-generated copy constructor.
Creating a Custom Copy Constructor
To create a custom copy constructor, you need to define how the class will copy its resources. Here’s how it can be done:
class MyClass {
public:
int* data;
MyClass(int value) {
data = new int(value);
}
// Custom copy constructor
MyClass(const MyClass& other) {
data = new int(*other.data); // Perform deep copy
}
~MyClass() {
delete data;
}
};
In the example above, the custom copy constructor allocates new memory for `data` and assigns the value from `other.data`, ensuring that each object maintains its memory, thus preventing issues related to shallow copying.
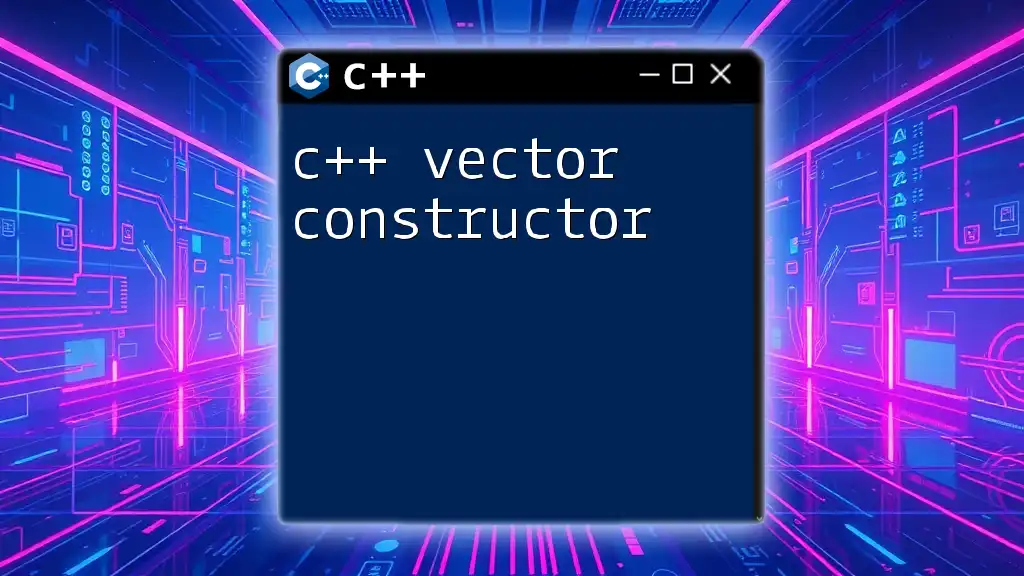
Best Practices for Using Copy Constructors
Guidelines for Effective Copy Constructor Design
When designing copy constructors, it’s essential to adhere to a few best practices:
- Always implement a corresponding destructor to manage resource deallocation properly.
- Consider implementing the Rule of Three, which states that if you define a copy constructor, you should also define a destructor and a copy assignment operator to handle resource management effectively.
Using `std::copy` and `std::copy_if`
Utilizing STL algorithms can enhance copy operations. Functions like `std::copy` provide a robust means to manipulate data within containers:
#include <algorithm>
#include <vector>
std::vector<int> source = {1, 2, 3, 4, 5};
std::vector<int> destination(source.size());
std::copy(source.begin(), source.end(), destination.begin());
By combining aspects of the STL with your copy constructors, you can achieve clearer and more efficient code in managing collections and dynamic resources.
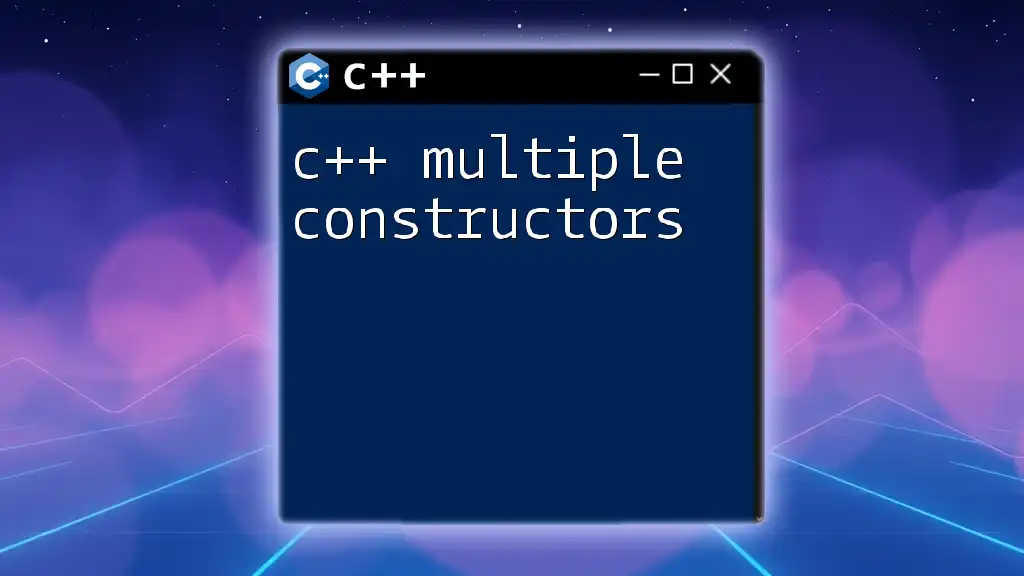
Summary
In conclusion, a solid understanding of the C++ default copy constructor is crucial for effective programming. Default copy constructors perform shallow copies, which can lead to unexpected behavior when dealing with dynamic data. By recognizing when to implement a custom copy constructor and following best practices, developers can ensure reliability and maintainability in their C++ applications.
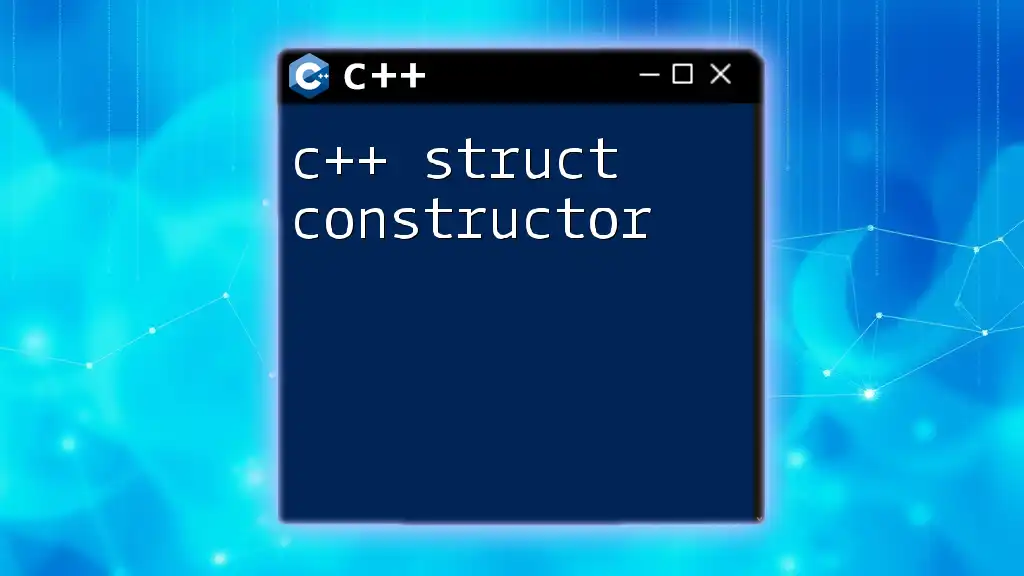
Additional Resources
For those aiming to deepen their understanding, numerous books, tutorials, and official documentation are available online. Exploring these resources can provide greater insight into the intricacies of C++ constructors and memory management.
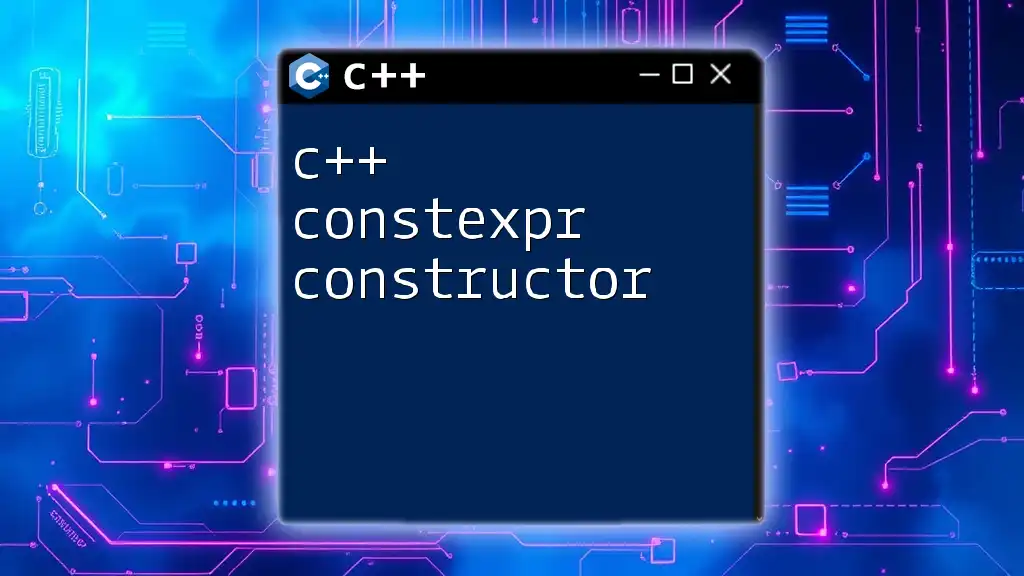
Conclusion
Practice is key to mastering copy constructors in C++. Implement your own copy constructors in various scenarios, and feel free to share your experiences or ask questions in the comments section. This interactivity could foster a vibrant community of learners and professionals dedicated to enhancing their C++ skills.