In C++, you can delete the copy constructor to prevent the copying of an object by declaring it as deleted, ensuring that instances of the class cannot be copied.
class MyClass {
public:
MyClass() = default; // Default constructor
MyClass(const MyClass&) = delete; // Delete copy constructor
};
Understanding Copy Constructors in C++
What is a Copy Constructor?
A copy constructor is a special type of constructor in C++ used to create a new object as a copy of an existing object. It initializes a new instance of a class by copying the properties of another instance, typically under the following syntax:
ClassName(const ClassName&);
The primary role of a copy constructor is to ensure that when an object is copied, all pertinent data gets transferred properly, thus maintaining valid state and behavior.
When is a Copy Constructor Invoked?
The copy constructor comes into play during various scenarios, including:
- Function parameters: When an object is passed by value to a function, the copy constructor is invoked to create a copy of that argument.
- Return values: When a function returns an object by value, the copy constructor creates a copy of the returned object.
- Object initialization: Assigning one object to another triggers the copy constructor.
Understanding these scenarios is crucial in order to properly manage resources and avoid unintended consequences like object slicing.
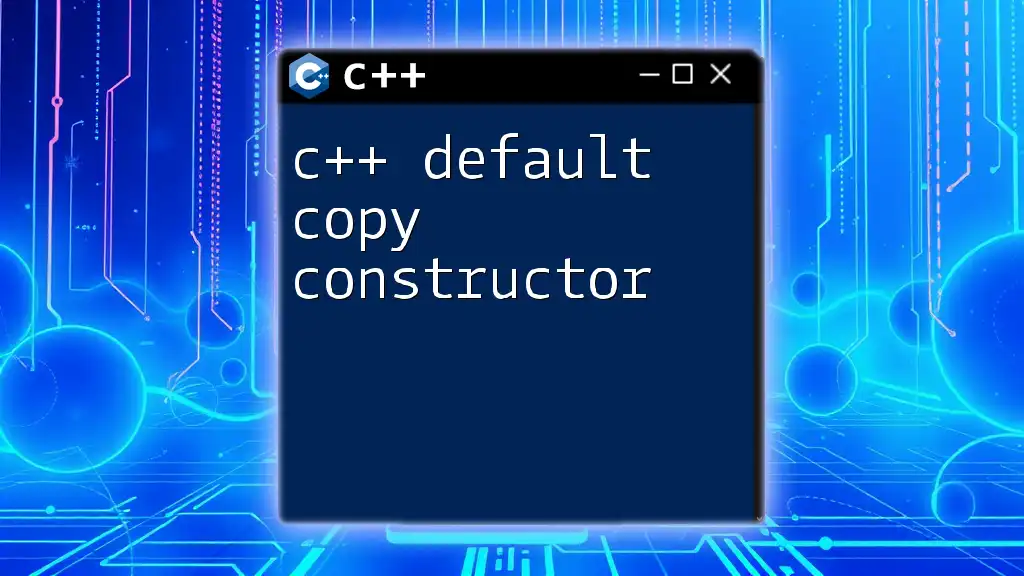
Why You Might Want to Delete a Copy Constructor
Preventing Object Slicing
Object slicing occurs when a derived class object is treated as a base class object. Only the base portion gets copied, causing the derived data to be lost. This is notably problematic in polymorphic designs where full object behavior is expected:
class Base {
public:
virtual void display() { std::cout << "Base" << std::endl; }
};
class Derived : public Base {
public:
void display() override { std::cout << "Derived" << std::endl; }
};
void func(Base b) { b.display(); } // Causes slicing
To avoid such undesirable behavior, one may wish to delete the copy constructor.
Managing Resources and Dynamic Memory
Classes that manage dynamic memory or other resources—like file handles or sockets—must ensure that resources are not inadvertently duplicated, leading to double frees or resource leaks. If a class utilizes dynamic memory through pointers, it’s essential to manage these correctly to prevent memory leaks.
Deleting the copy constructor prevents unintended copies, thus safeguarding resource integrity.
Ensuring Unique Ownership
Unique ownership refers to the property where a resource (like dynamic memory) is owned by a single instance of a class. This is vital in scenarios where resources must not be shared or duplicated. For instance, in classes that wrap around pointers, having a deleted copy constructor ensures no two objects can manage the same resource, thereby preventing potential issues like double deletion.
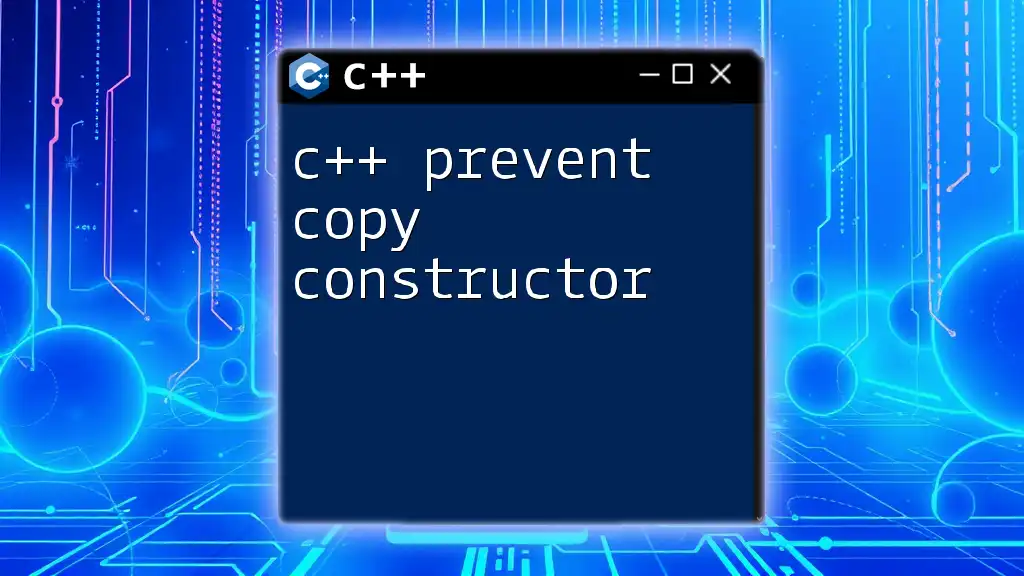
How to Delete a Copy Constructor
Syntax of Deleting a Copy Constructor
To delete a copy constructor, you use the following syntax in your class definition:
class MyClass {
public:
MyClass(const MyClass&) = delete; // Deleting the copy constructor
};
By performing this action, the program will not be able to compile code that attempts to copy an instance of `MyClass`.
The Compilation Error
If a copy is attempted, the program will produce a compilation error, effectively safeguarding against unintended copies. For instance, the following code will not compile:
MyClass obj1;
MyClass obj2 = obj1; // Error: use of deleted function
This safeguards against inadvertent misuse of the class and reinforces the design logic dictated by the programmer.
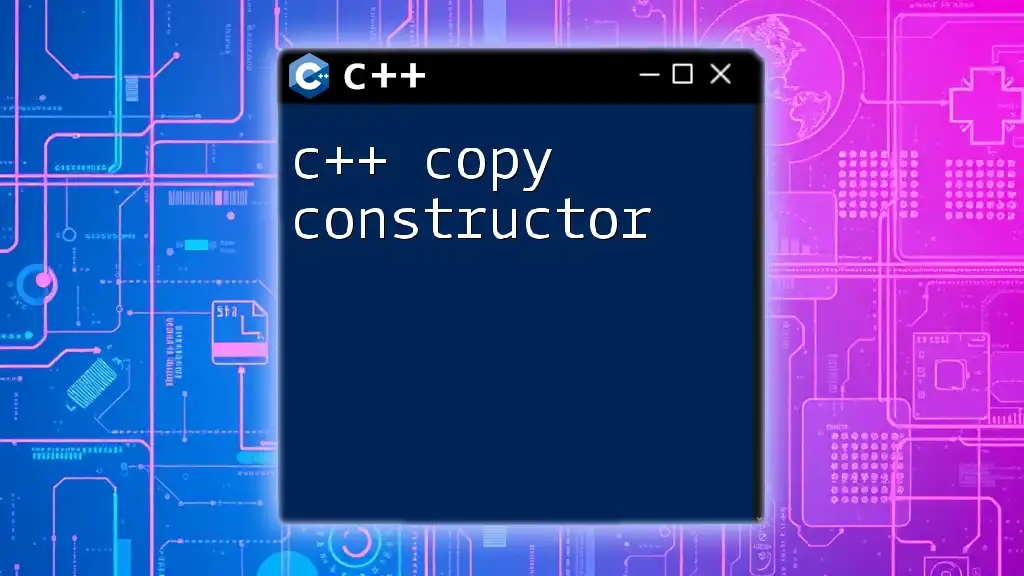
Alternative Strategies
Move Constructors
To complement the deletion of a copy constructor, move constructors become invaluable, especially for classes managing resources. Move constructors transfer ownership from one object to another without the overhead of copying all member variables:
class MyClass {
public:
MyClass(MyClass&&) noexcept; // Move constructor
};
This allows for efficient resource management while still preserving the original object’s resources.
Implementing Copy Control
Understanding the dynamics of both the copy assignment operator and copy constructor is key in resource-sensitive applications. Implementing both provides flexibility while ensuring that unintended copies do not occur.
Using Smart Pointers
Utilizing smart pointers such as `std::unique_ptr` and `std::shared_ptr` can significantly reduce the complexity of memory management in C++. These tools automatically manage the lifetime of dynamically allocated objects and prevent issues like memory leaks or dangling pointers.
By encapsulating raw pointers in smart pointers, you leverage C++'s automatic memory management, avoiding the need to explicitly delete copy constructors in many scenarios.
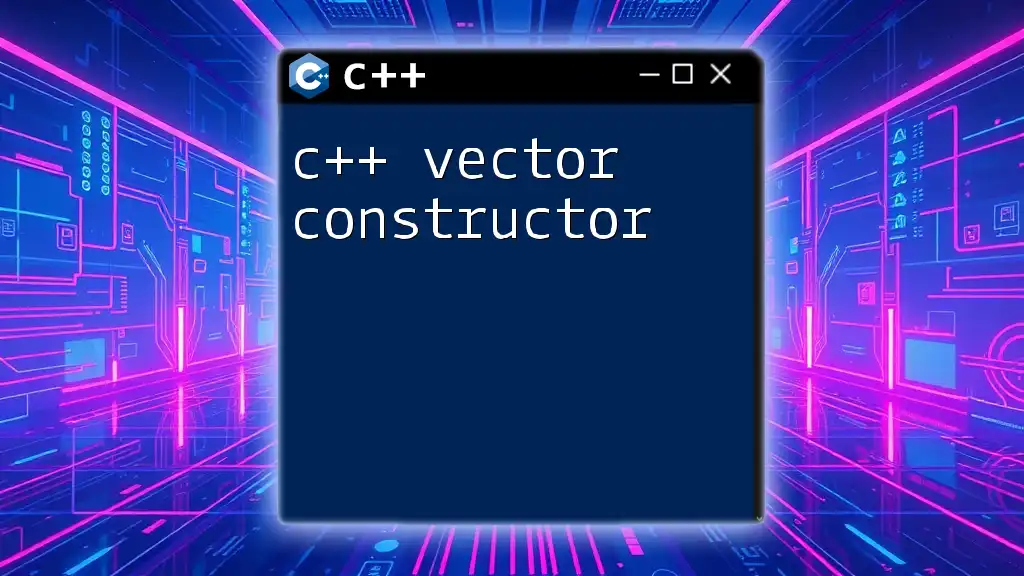
Real-World Use Cases
When to Use a Deleted Copy Constructor
In real-world applications, there are numerous scenarios where deleting a copy constructor is advantageous. For example, if you have a class that manages a large data file, copying the object could result in significant overhead or even corruption of file handles. Deleting the copy constructor protects the integrity of such data structures.
Examples in Popular Libraries
Many modern C++ libraries, including parts of the STL (Standard Template Library), recognize the importance of managing resources and employ deleted copy constructors effectively. For instance, classes like `std::unique_ptr` and `std::vector` are designed to prevent copying, exemplifying best practices in resource management.
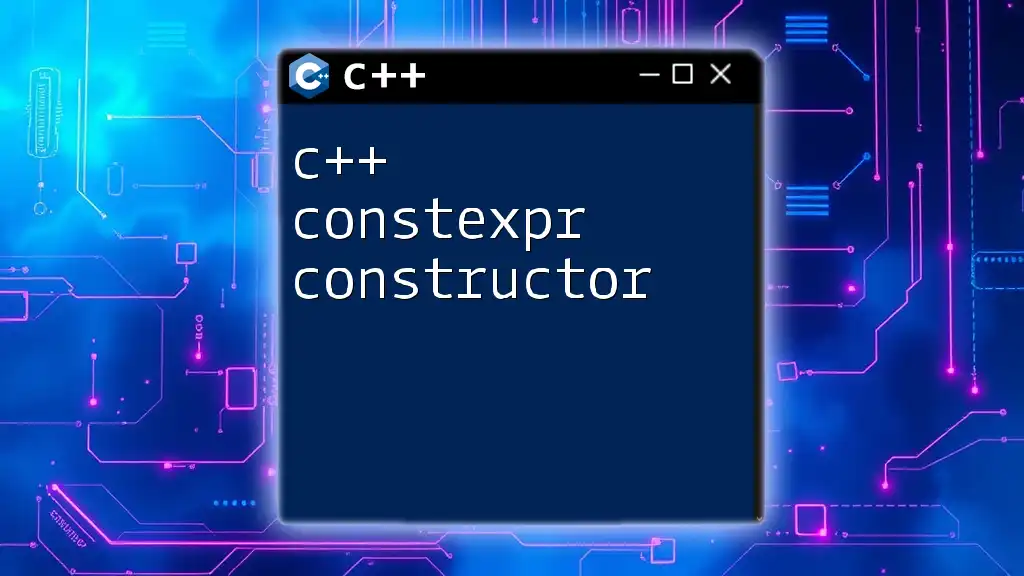
Conclusion
Summary of Key Points
In summary, understanding how to delete a copy constructor is an essential skill in C++. It helps prevent common pitfalls, such as object slicing and resource mismanagement. This guidance not only streamlines memory management but also adheres to C++ best practices, ultimately leading to cleaner and more maintainable code.
Encouragement to Explore Further
By experimenting with copy constructors and unique ownership strategies, you can deepen your understanding of C++ and refine your coding skills. Further exploration into modern C++ techniques, including move semantics and smart pointers, will empower you to write more robust and efficient programs. Always invest time in understanding these concepts to elevate your programming expertise in C++.