A C++ linked list copy constructor creates a new linked list that is a duplicate of an existing linked list, ensuring that each node is copied and not just the node references.
class Node {
public:
int data;
Node* next;
Node(int val) : data(val), next(nullptr) {}
};
class LinkedList {
public:
Node* head;
LinkedList() : head(nullptr) {}
// Copy constructor
LinkedList(const LinkedList& other) {
if (!other.head) {
head = nullptr;
} else {
head = new Node(other.head->data);
Node* current = head;
Node* otherCurrent = other.head->next;
while (otherCurrent) {
current->next = new Node(otherCurrent->data);
current = current->next;
otherCurrent = otherCurrent->next;
}
}
}
~LinkedList() {
// Destructor to free nodes (not shown for brevity)
}
};
Understanding the Basics of Linked Lists in C++
What is a Linked List?
A linked list is a fundamental data structure used to store a collection of elements. Unlike traditional arrays, where elements are indexed and stored contiguously in memory, linked lists comprise nodes that contain data and a pointer/reference to the next node in the sequence. This characteristic allows for dynamic memory allocation, making linked lists efficient when it comes to frequent insertions and deletions.
Types of Linked Lists
Linked lists can be categorized into three primary types:
- Singly Linked Lists: Each node points to the next node in the sequence, and traversal is one-way, from head to tail.
- Doubly Linked Lists: Nodes contain two pointers, one pointing to the next node and another to the previous node, allowing for bidirectional traversal.
- Circular Linked Lists: The last node points back to the first node, creating a circular connection and allowing for continuous traversal.

Introduction to Copy Constructors
What is a Copy Constructor?
A copy constructor is a special type of constructor in C++ that initializes an object using another object of the same class. When you create a new object as a copy of an existing object, the copy constructor is invoked to ensure that the new object is initialized with the contents and structure of the original object.
Why Use a Copy Constructor?
Utilizing a copy constructor is crucial for proper memory management. Without it, copying objects by default can lead to shallow copies, where both objects point to the same memory addresses. This poses a considerable risk in linked lists, where accidental modifications could corrupt data structures and lead to memory leaks or crashes.

Copy Constructor for Linked List C++
The Concept of Copying Linked Lists
To copy a linked list means to create a new list that duplicates the structure and content of an existing list. This process presents unique challenges, particularly concerning pointer management. If not handled properly, the copied list could end up referencing the same nodes as the original, causing a cascade of issues when either list is modified.
Implementing the Copy Constructor in C++
When creating a linked list class, the copy constructor needs to be explicitly defined to ensure that each node is duplicated correctly. Here's how to structure the linked list class:
struct Node {
int data;
Node* next;
};
class LinkedList {
Node* head;
public:
LinkedList(); // Constructor
LinkedList(const LinkedList& other); // Copy constructor
~LinkedList(); // Destructor
// Additional member functions
};
Code Snippet: Basic Copy Constructor Implementation
The following code demonstrates a fundamental copy constructor implementation for a singly linked list:
LinkedList(const LinkedList& other) {
if (!other.head) {
head = nullptr; // Handle the case of an empty list
return;
}
head = new Node();
head->data = other.head->data; // Copy the head node
Node* current = head;
Node* otherCurrent = other.head->next;
while (otherCurrent) {
current->next = new Node(); // Allocate new node
current = current->next; // Move to the new node
current->data = otherCurrent->data; // Copy data
otherCurrent = otherCurrent->next; // Move to next node in original
}
current->next = nullptr; // End the new linked list
}
Explanation of the Code
In the above implementation, we begin by checking if the original list is empty. If it is, we set the `head` of our new list to `nullptr`—a robust way to handle edge cases. As we allocate new nodes for the copied list, we systematically traverse the original list using a pointer (`otherCurrent`) and assign data to the newly created nodes, thereby ensuring that changes in either list do not affect the other.

Copy Constructor in Action
Example Usage of the Copy Constructor
Here’s how you would utilize the copy constructor in practice:
LinkedList list1;
// Code to populate list1 with data
LinkedList list2 = list1; // Using the copy constructor
This simple assignment creates `list2` as an entirely separate copy of `list1`, with its own nodes and data.
Visual Representation of the Copy Process
Imagine `list1` has nodes representing values: 1 -> 2 -> 3. After invoking the copy constructor to create `list2`, each value is replicated, leading to the structure: 1 -> 2 -> 3. However, the memory addresses of the nodes in `list2` differ from those in `list1`, ensuring that the two lists operate independently.
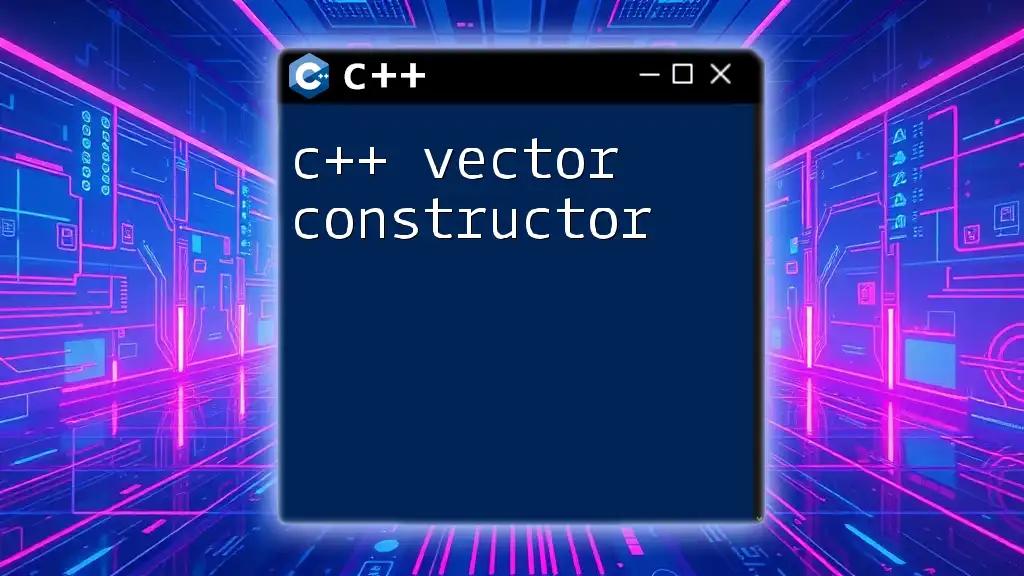
Troubleshooting Common Issues
Shallow Copies vs. Deep Copies
Understanding the difference between shallow and deep copies is essential. A shallow copy merely copies the top-level structure, resulting in two objects referencing the same nested resources. This can lead to catastrophic failures if one object is modified. A deep copy ensures that all nested resources are duplicated, maintaining distinct instances.
Common Mistakes When Implementing Copy Constructors
New C++ programmers often encounter pitfalls while implementing a copy constructor:
- Forgetting to allocate new memory: If you forget to create new nodes, both lists will end up pointing to the same data, leading to unexpected behavior.
- Not handling empty lists correctly: Neglecting to check if the original list is empty can lead to dereferencing `nullptr`, leading to application crashes.

Best Practices for Copy Constructors in C++
Memory Management Considerations
Effective memory management is paramount when working with linked lists. Always ensure that every `new` operation is paired with a corresponding `delete` to avoid memory leaks. Including a destructor is crucial for releasing allocated memory when an object goes out of scope.
~LinkedList() {
Node* current = head;
while (current) {
Node* next = current->next; // Store reference to next node
delete current; // Delete current node
current = next; // Move to next node
}
}
Unit Testing Your Copy Constructor
Testing your copy constructor is essential for validating its accuracy and reliability. Here are some strategies:
- Create test cases with varying data: Test the copy constructor with empty lists, single-node lists, and multi-node lists to check for correctness.
- Check for independent modifications: After copying, modify nodes in the original list and validate that the copied list remains unchanged.
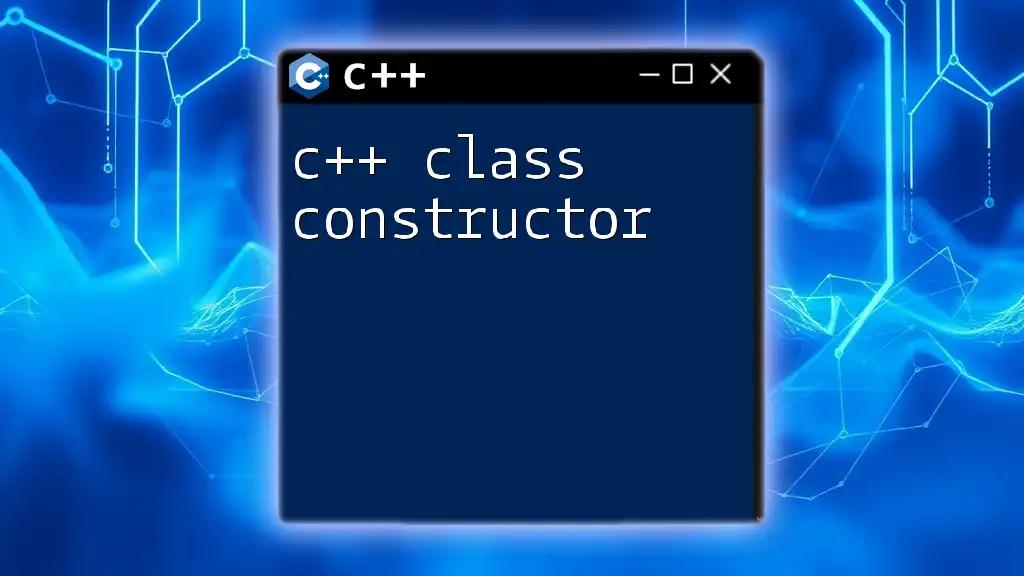
Summary
Recap of Key Points
In summary, the C++ linked list copy constructor is vital for ensuring that your linked lists can be safely duplicated without unintended side effects. By properly implementing deep copies, you preserve the integrity of your data structures while managing memory effectively.
Future Considerations
While linked lists provide excellent dynamic memory management capabilities, consider venturing into other data structures such as vectors or arrays for different use cases. Understanding when to use each structure will enhance your programming toolkit.

Additional Resources
Recommended Books and Online Courses
For further learning and mastery of C++ and data structures, consider resources such as:
- "C++ Primer" by Stanley B. Lippman
- "Data Structures and Algorithms in C++" by Adam Drozdek
- Online courses on platforms such as Coursera or Udacity.
Community and Support
Join forums and discussion groups, like Stack Overflow or Reddit's r/cpp, to seek advice, share insights, and connect with fellow C++ learners and experts.