In C++, a derived class can explicitly call a parent class constructor to initialize its base part using an initializer list, as shown in the following example:
#include <iostream>
using namespace std;
class Parent {
public:
Parent(int x) { cout << "Parent Constructor: " << x << endl; }
};
class Child : public Parent {
public:
Child(int x) : Parent(x) { cout << "Child Constructor" << endl; }
};
int main() {
Child obj(5);
return 0;
}
In this code, the `Child` class calls the `Parent` constructor with the value `x` to properly initialize the base class before executing its own constructor.
The Basics of C++ Inheritance
Understanding Inheritance
Inheritance is a fundamental concept in object-oriented programming that allows one class to inherit the properties and methods of another. This mechanism facilitates code reuse and establishes a relationship between classes.
- Types of Inheritance: In C++, inheritance can be classified into several types, including single inheritance, multiple inheritance, multilevel inheritance, hierarchy, and hybrid inheritance. Each has its own implications for how constructors are called.
Base Classes and Derived Classes
In the realm of inheritance, the class that is being inherited from is known as the base class (or parent class), whereas the class that inherits is called the derived class (or child class).
- What is a Base Class?: The base class contains attributes and methods that can be shared by its derived classes.
- What is a Derived Class?: The derived class can have additional attributes and methods, along with the properties inherited from the base class.
How Constructors Work in Inheritance
When a derived class is instantiated, C++ automatically calls the constructor of its base class first. This sequential calling ensures that the base part of the object is constructed prior to the derived part.
- Flow of Constructor Calls:
- Call the base class constructor.
- Call the derived class constructor.
Constructors and Initialization Lists
Initialization lists are an effective way to initialize base class members at the time of the constructor call:
- Explanation of Initialization Lists: An initialization list allows values to be passed directly to the base class constructor.
Example:
class Base {
public:
Base(int x) {
// Implementation
}
};
class Derived : public Base {
public:
Derived(int x) : Base(x) {
// Implementation
}
};
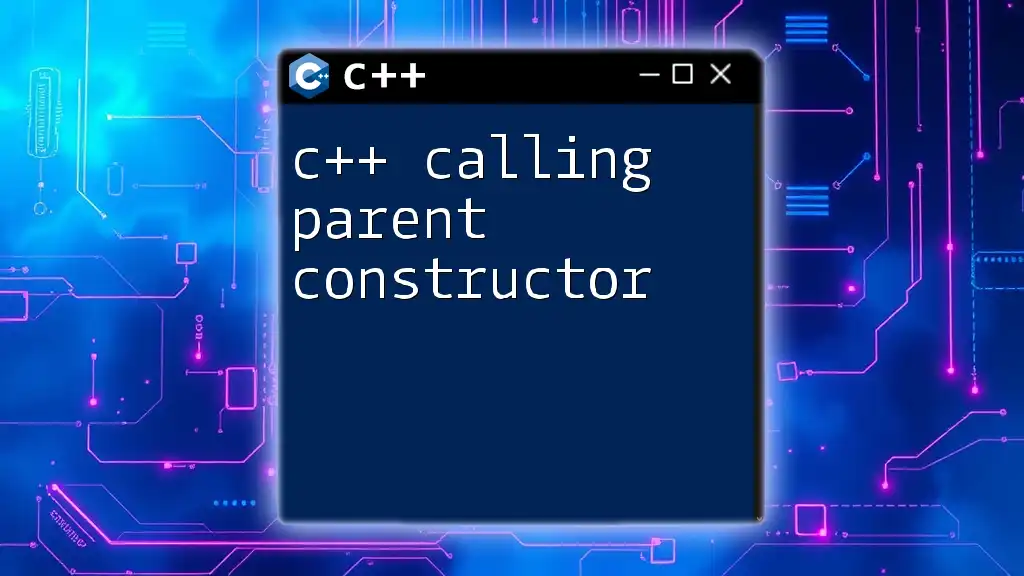
Using the Parent Constructor
Syntax and Implementation
The syntax for calling a parent constructor involves an initialization list following the derived class constructor's parameter list. This approach helps encapsulate the initialization sequence effectively.
Code Snippet Example:
class Base {
public:
Base(int value) {
// Initialize base class member
}
};
class Derived : public Base {
public:
Derived(int value) : Base(value) {
// Initialize derived class member
}
};
In this example, when a `Derived` object is created with an integer value, it first calls the `Base` class constructor and passes that value, ensuring proper initialization.
When to Use Parent Constructors
It's essential to use parent constructors to initialize base class attributes appropriately, especially when the base class contains attributes that are crucial for the derived class's functionality.
- Importance of Proper Initialization: If the base class is not properly initialized, it can lead to undefined behavior in the derived class.
In cases where both the base and derived classes manage resources, such as file handles or network connections, utilizing parent constructors can help in effectively managing these shared resources.
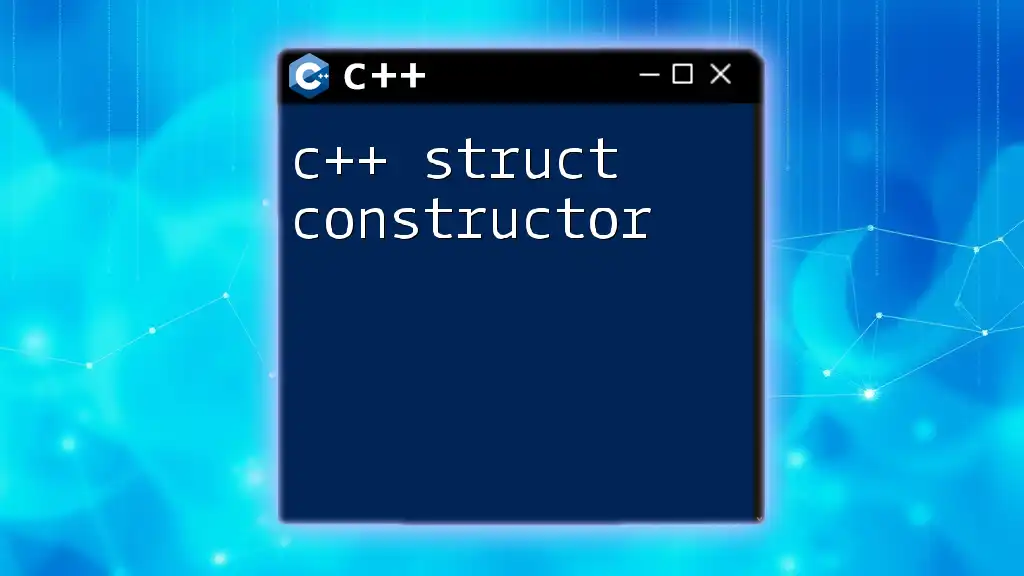
Advanced Concepts
Default Constructors
A default constructor is one that either has no parameters or has default values for all its parameters. In derived classes, the default constructor of a base class can be invoked implicitly or explicitly.
- Using Default Parent Constructors in Derived Classes: When a derived class is created without providing values for the base class's properties, the default constructor of the base class is called.
Code Snippet Example:
class Base {
public:
Base() {
// Default Constructor Implementation
}
};
class Derived : public Base {
public:
Derived() : Base() {
// Derived class implementation
}
};
In this example, when the `Derived` class constructor is called, it automatically invokes the `Base` class's default constructor.
Multiple Inheritance
Multiple inheritance is a powerful feature in C++, allowing a derived class to inherit from more than one base class.
- Understanding Multiple Inheritance: It is essential when a derived class requires functionalities from multiple base classes.
Calling Parent Constructors in Multiple Inheritance
When implementing multiple inheritance, it’s crucial to clearly specify which base class constructor to call, as it maintains clarity and avoids ambiguity.
Code Snippet Example:
class Base1 {
public:
Base1(int a) {
// Base1 Constructor Implementation
}
};
class Base2 {
public:
Base2(int b) {
// Base2 Constructor Implementation
}
};
class Derived : public Base1, public Base2 {
public:
Derived(int x, int y) : Base1(x), Base2(y) {
// Derived class implementation
}
};
In this scenario, when a `Derived` object is created, the respective constructors for `Base1` and `Base2` are called, ensuring both base classes are properly initialized.
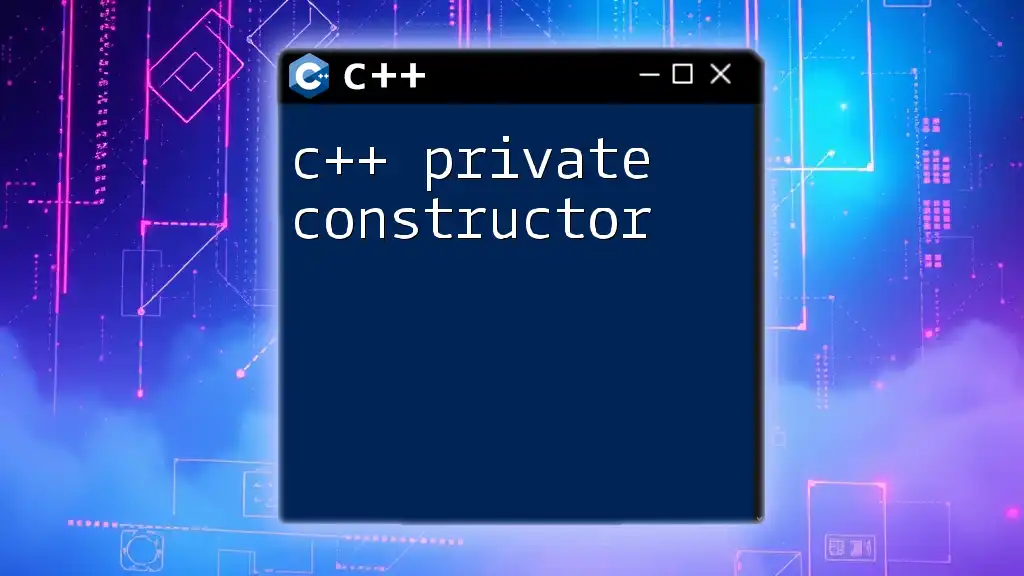
Common Pitfalls and Best Practices
Common Mistakes with Parent Constructors
One of the most common mistakes is forgetting to call parent constructors, which can lead to uninitialized values and unpredictable behavior. Additionally, ambiguity can arise in multiple inheritance scenarios if constructors are not explicitly defined.
Best Practices for Using Parent Constructors
- Be Explicit with Initialization: It is recommended to always explicitly initialize base class attributes. This makes your code cleaner and prevents potential errors.
- Use Parameterized Constructors Wisely: Ensure any critical initialization parameters are passed appropriately through the parent constructor.
- Documentation and Commentary: Always comment on your code, especially when dealing with complex inheritance hierarchies. This practice aids in maintaining clarity for future developers.
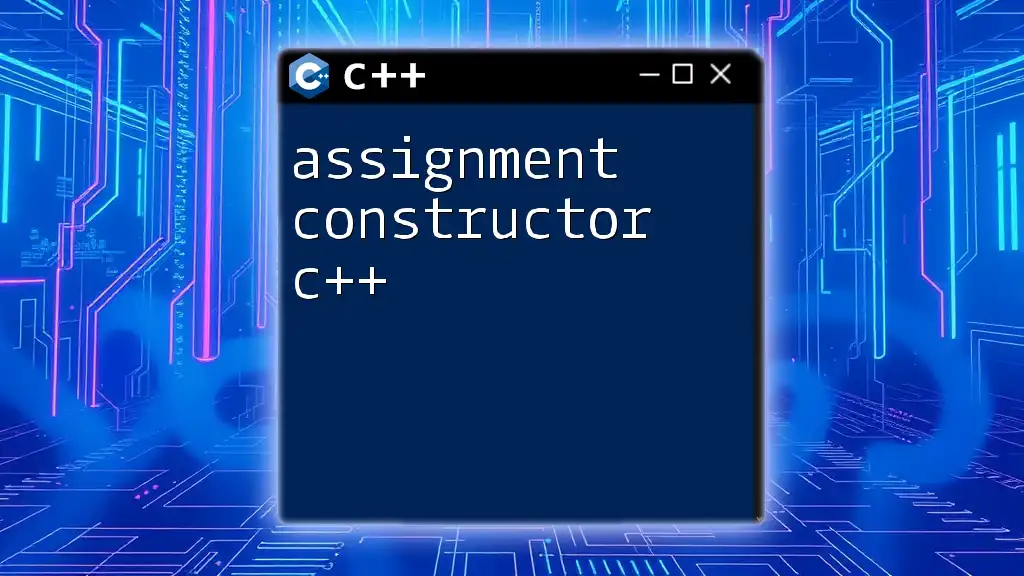
Conclusion
Understanding and effectively using C++ using parent constructor is crucial for any C++ programmer dealing with inheritance. By properly initializing base class constructors, you ensure that your object-oriented design remains robust, efficient, and easy to manage.
As you explore more advanced concepts in C++, remember that practice is key. Try implementing what you learned and experiment with various class hierarchies to deepen your understanding.
Explore additional resources, participate in community discussions, or consider structured courses to further your knowledge of C++ and inheritance mechanisms.