In C++, you can call a parent class's constructor from a derived class using an initializer list in the constructor of the derived class.
Here's an example:
#include <iostream>
using namespace std;
class Parent {
public:
Parent() {
cout << "Parent constructor called." << endl;
}
};
class Child : public Parent {
public:
Child() : Parent() { // Calling the parent constructor
cout << "Child constructor called." << endl;
}
};
int main() {
Child obj; // This will call both Parent and Child constructors
return 0;
}
Understanding Constructors in C++
What is a Constructor?
A constructor is a special type of member function that is automatically called when an object of a class is created. Its primary role is to initialize the object and allocate resources, setting it up for use. Constructors can be categorized into three types:
- Default Constructor: A constructor that takes no arguments. It initializes the object with default values.
- Parameterized Constructor: A constructor that takes arguments, allowing the initialization of an object with specific values.
- Copy Constructor: A constructor that creates a new object as a copy of an existing object.
The Role of Parent Constructors
In C++, constructors of base classes (parents) are critical when creating objects of derived classes (children). This is because derived classes inherit attributes and behaviors from base classes, making it essential to initialize those inherited parts during the derived class's construction. Failure to properly call and initialize parent constructors can lead to uninitialized variables, undefined behaviors, or even runtime errors.
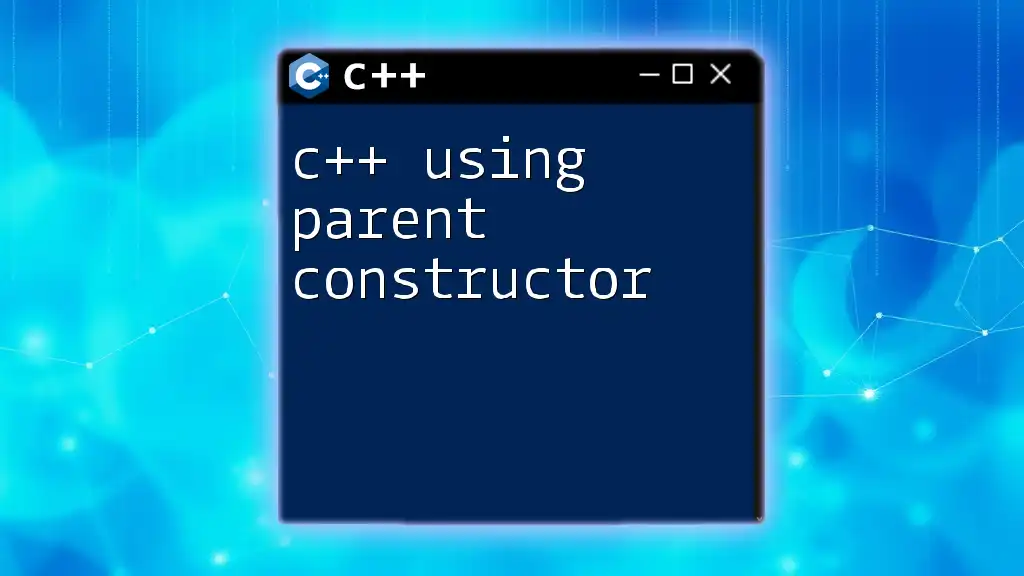
C++ Inheritance Overview
Types of Inheritance
Inheritance in C++ allows one class to acquire properties and methods from another. There are several types of inheritance:
- Single Inheritance: A derived class inherits from a single base class.
- Multiple Inheritance: A derived class inherits from more than one base class.
- Multilevel Inheritance: A derived class inherits from a base class, which in turn inherits from another class.
- Hierarchical Inheritance: Multiple derived classes inherit from a single base class.
Understanding these concepts is imperative as they determine how constructors are invoked and how inheritance hierarchies are structured.
The Concept of Base and Derived Classes
Base classes serve as the foundational structures from which derived classes inherit.
For example:
class Base {
public:
void display() { /*...*/ }
};
class Derived : public Base {
public:
void show() { /*...*/ }
};
In this code snippet, `Derived` is inheriting functionality from `Base`, allowing it to reuse and extend its behavior.
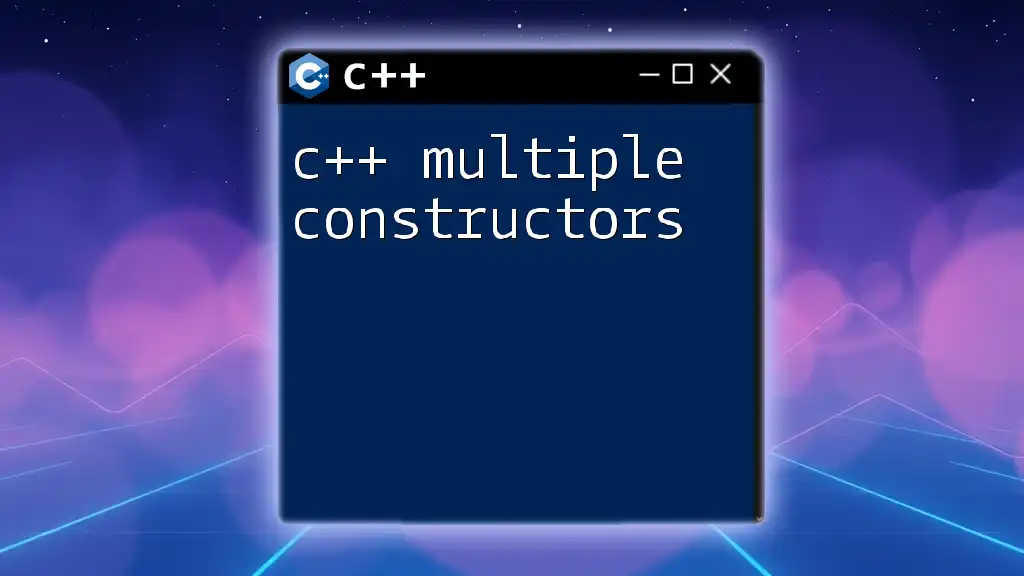
C++ Calling Parent Constructor
Syntax for Calling Parent Constructors
The primary method of calling a parent constructor in C++ is through the use of constructor initialization lists. This approach provides a clear way to initialize base class attributes before the derived class's constructor body executes. The syntax is concise:
Derived(parameters) : Base(parameters) { /* Derived class initialization */ }
Using Constructor Initialization List
When you want to call a parent constructor, the initialization list is crucial. This method directly passes the parameters to the base class constructor.
For example:
class Base {
public:
Base(int value) { /* initialization code */ }
};
class Derived : public Base {
public:
Derived(int value) : Base(value) { /* additional initialization */ }
};
In this example, when you create a `Derived` object, it successfully calls the `Base` constructor using the provided value.
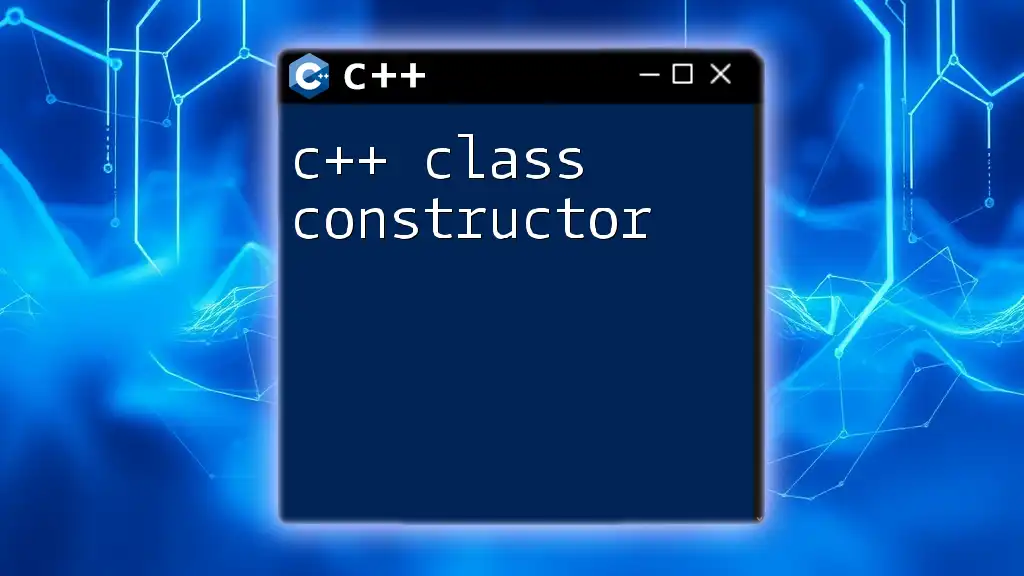
Different Ways to Call Parent Constructors
Using Default Constructor
If you do not specify a parent constructor in the derived class's constructor, C++ automatically invokes the default constructor of the parent class.
Example:
class Base {
public:
Base() { /* default initialization */ }
};
class Derived : public Base {
public:
Derived() { /* derived initialization */ } // Base() is called by default
};
Here, when a `Derived` object is instantiated, the `Base` class constructor executes without needing a specific call.
Calling Parameterized Constructors
To initialize base classes with specific values, you must explicitly call the parameterized constructor.
For instance:
class Base {
public:
Base(int x) { /* initialization code with x */ }
};
class Derived : public Base {
public:
Derived(int x) : Base(x) { /* derived class initialization */ }
};
In this case, the `Derived` constructor forwards the `x` parameter to the `Base` constructor, ensuring proper initialization.
Handling Multiple Inheritance
When working with multiple inheritance, each base class requires an explicit constructor call in the derived class's initialization list.
Example:
class Base1 {
public:
Base1(int x) { /*...*/ }
};
class Base2 {
public:
Base2(int y) { /*...*/ }
};
class Derived : public Base1, public Base2 {
public:
Derived(int x, int y) : Base1(x), Base2(y) { /*...*/ }
};
In this multilevel hierarchy, both `Base1` and `Base2` constructors are called using the initialization list.
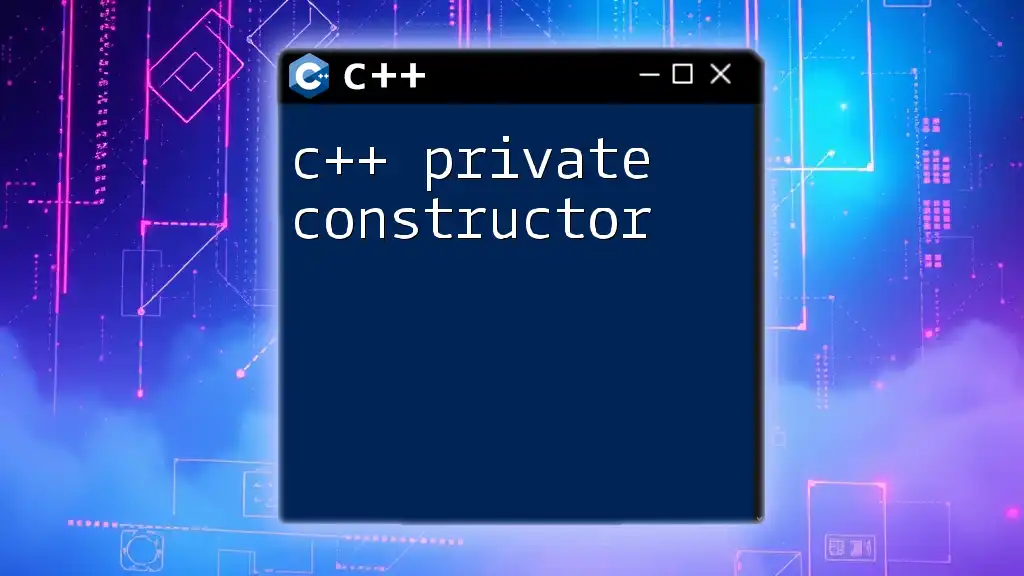
Common Pitfalls and Best Practices
Common Errors When Calling Parent Constructors
When calling parent constructors, programmers may face various pitfalls:
- Forgetting to call a constructor: Always remember that failing to call parent constructors when required can lead to uninitialized data.
- Mismatching parameter types: Ensure that the types of the arguments in the derived class match those expected in the base class constructors.
- No default constructor in base class: If the base class lacks a default constructor, you must call a parameterized constructor explicitly.
Best Practices When Using Constructor Initialization Lists
To maximize the benefits of constructor initialization lists, consider the following best practices:
- Use initialization lists for better performance. They directly initialize member variables rather than assigning values inside the constructor's body.
- Keep initialization concise and focused on the necessary parameters. This approach maintains readability and clarity in your code.
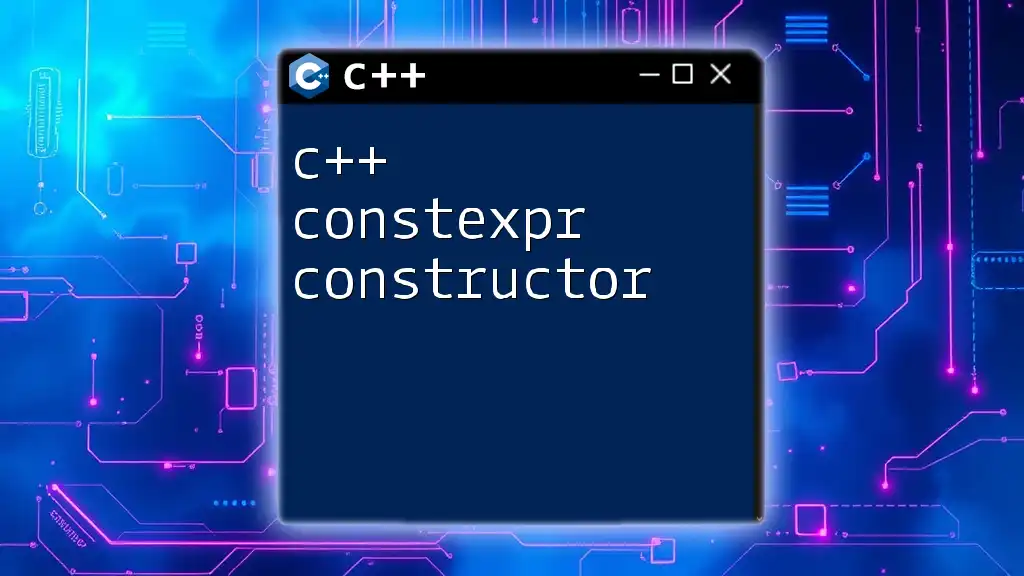
Conclusion
Mastering the process of C++ calling parent constructor is essential for any C++ programmer working with class inheritance. It ensures that both base and derived class parts are properly initialized, promoting robust and error-free application development. By practicing the concepts discussed, you will enhance your understanding of constructors and improve your C++ coding skills. Dive into creating your classes and explore the power of inheritance and initialization in C++!
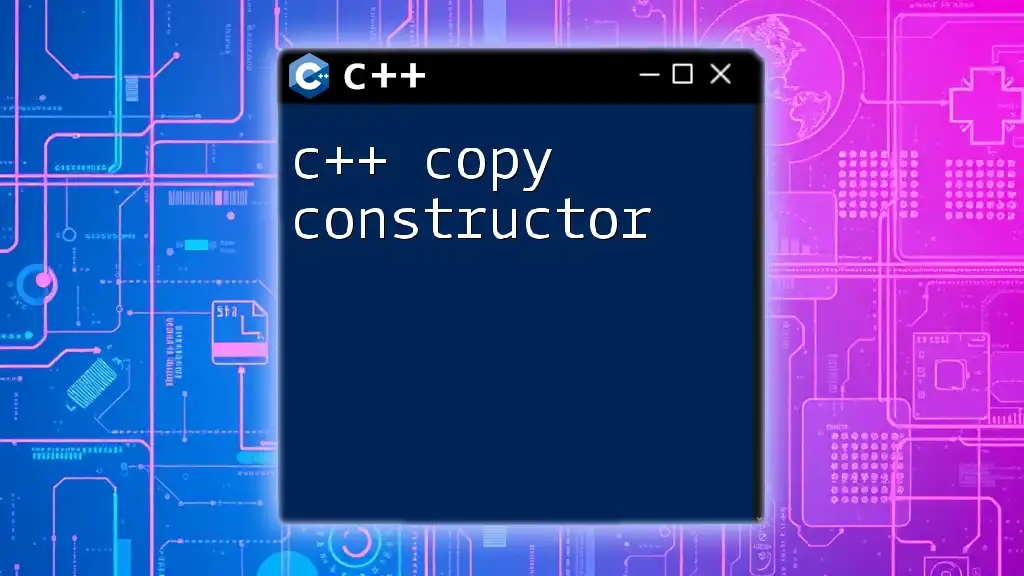
Additional Resources
For further reading, consider exploring C++ documentation and resources like online challenges devoted to C++ programming practices to cement your knowledge and skills in this essential area.