A private constructor in C++ restricts the instantiation of a class to within the class itself, often used to implement the Singleton design pattern. Here's an example:
class Singleton {
private:
static Singleton* instance;
Singleton() {} // Private constructor
public:
static Singleton* getInstance() {
if (!instance) {
instance = new Singleton();
}
return instance;
}
};
Singleton* Singleton::instance = nullptr;
What is a Private Constructor?
A private constructor in C++ is a constructor that is declared as `private`, preventing direct instantiation of a class from outside the class itself. This design enforces specific patterns and controls how objects are created, thereby maintaining certain conditions or states.
Importance of Private Constructors: By restricting access to class instantiation, developers can implement design patterns such as the Singleton pattern, ensuring a single instance of a class while controlling the instances' creation.

Use Cases for Private Constructors
There are several scenarios in which a private constructor is advantageous:
- Singleton Pattern: Ensures that a class has only one instance, allowing access to that instance through a static method.
- Utility Classes: These classes may contain static functions and do not need to be instantiated, thus utilizing a private constructor helps prevent object creation.
- Factory Pattern: This approach allows the creation of objects via factory methods, rather than exposing the public constructor.
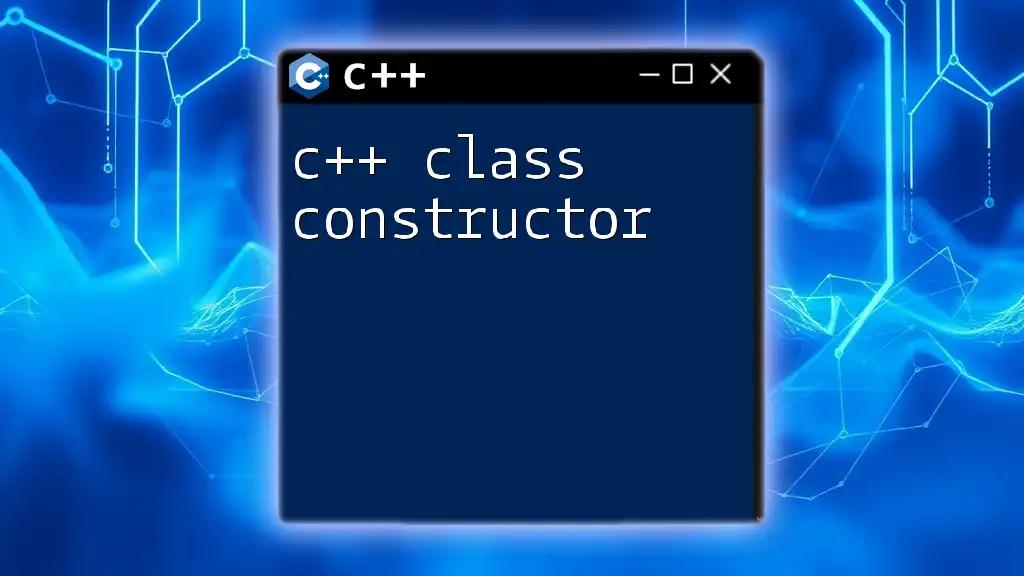
Understanding Access Modifiers in C++
Overview of Access Modifiers
C++ provides access modifiers to control the visibility of class members and methods. The three primary access modifiers include:
- Public: Members can be accessed from anywhere in the program.
- Private: Members are accessible only within the class.
- Protected: Members are accessible within the class and by inherited classes.
Importance of Private Access Modifier
The private access modifier is crucial for encapsulation in object-oriented programming. By declaring variables and methods as private, developers can safeguard the internal state of an object from unintended interference or misuse. This is especially vital in complex systems where data integrity is paramount.

How to Implement a Private Constructor
Creating a Class with a Private Constructor
To create a class with a private constructor, simply define the constructor under the private section of the class. Here’s an example:
class MyClass {
private:
MyClass() {
// Initialization code
}
public:
static MyClass createInstance() {
return MyClass();
}
};
In this example, `MyClass` cannot be instantiated from outside the class, but it can be created via the static member function `createInstance`.
Instantiation via Static Member Function
The implementation of a static member function provides a way to create instances of a class with a private constructor, which is essential for encapsulating object creation. The static function acts as a factory method. It’s a clear example of the Factory Pattern.

The Singleton Pattern: An Illustration of Private Constructors
Explaining the Singleton Design Pattern
The Singleton Pattern is a design pattern that ensures a class has only one instance and provides a global point of access to it. This is particularly useful when exactly one object is needed to coordinate actions across the system.
Implementing a Singleton with a Private Constructor
Here’s a classic implementation of a Singleton using a private constructor:
class Singleton {
private:
static Singleton* instance;
Singleton() {} // Private Constructor
public:
static Singleton* getInstance() {
if (instance == nullptr) {
instance = new Singleton();
}
return instance;
}
};
Singleton* Singleton::instance = nullptr;
In this code snippet:
- The constructor is private, preventing any external instantiation.
- The static method `getInstance` checks if an instance already exists; if not, it creates one. This ensures a single instance throughout the application.
Thread Safety in Singletons
When multiple threads might access the singleton instance, it is crucial to ensure thread safety. Using a mutex can help synchronize instance creation, preventing multiple threads from creating separate instances:
#include <mutex>
class ThreadSafeSingleton {
private:
static ThreadSafeSingleton* instance;
static std::mutex mutex_;
ThreadSafeSingleton() {}
public:
static ThreadSafeSingleton* getInstance() {
std::lock_guard<std::mutex> lock(mutex_);
if (instance == nullptr) {
instance = new ThreadSafeSingleton();
}
return instance;
}
};
ThreadSafeSingleton* ThreadSafeSingleton::instance = nullptr;
std::mutex ThreadSafeSingleton::mutex_;
With this implementation, the mutex ensures that even in a multi-threaded environment, only one instance of `ThreadSafeSingleton` is created.
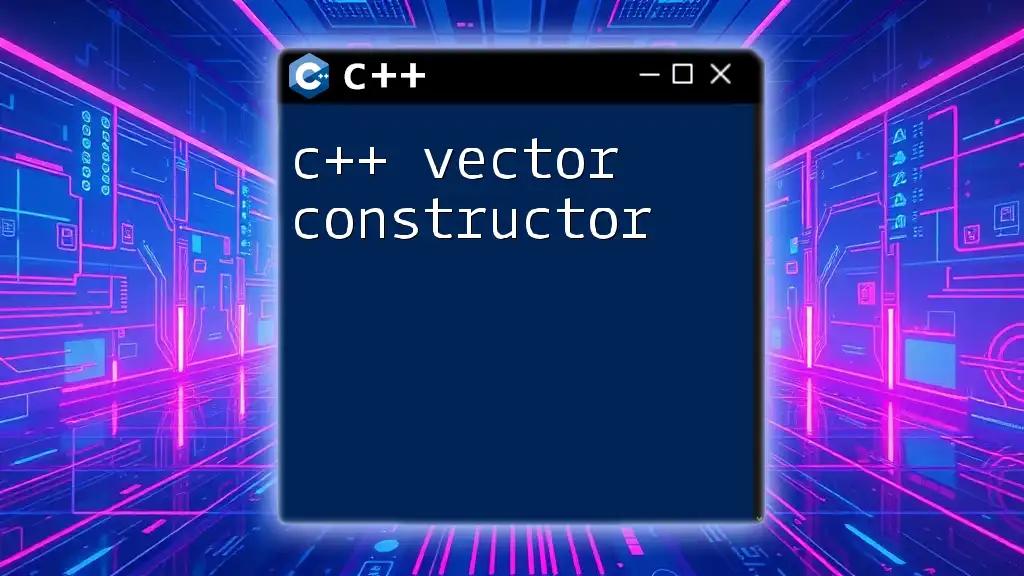
Advantages and Disadvantages of Using Private Constructors
Advantages
- Enhanced Encapsulation: By restricting visibility, private constructors enhance data encapsulation, protecting the internal state and behavior of a class.
- Control over Instance Creation: Limiting instance creation through static methods allows more control and flexibility in how and when instances are generated.
Disadvantages
- Complexity: The introduction of private constructors can complicate class structures and may lead to confusion for developers unfamiliar with the pattern.
- Testing Challenges: Classes with private constructors may present complications in unit testing, as traditional instantiation methods are not available.

Common Pitfalls When Using Private Constructors
Overlooking Static Methods
One common mistake is overlooking the need to provide static methods for instance creation. This can lead to developer frustration and confusion regarding how to instantiate the class.
Memory Leaks with Singleton
Developers should also be cautious about memory management in Singleton designs. If a Singleton instance is never deleted, it can lead to memory leaks in applications, particularly in long-running processes.
Testing Challenges
Testing becomes less straightforward with classes that have private constructors, as it’s challenging to create instances directly in unit tests. Developers may need to employ mocking techniques or adapt their tests to accommodate the encapsulated structure.
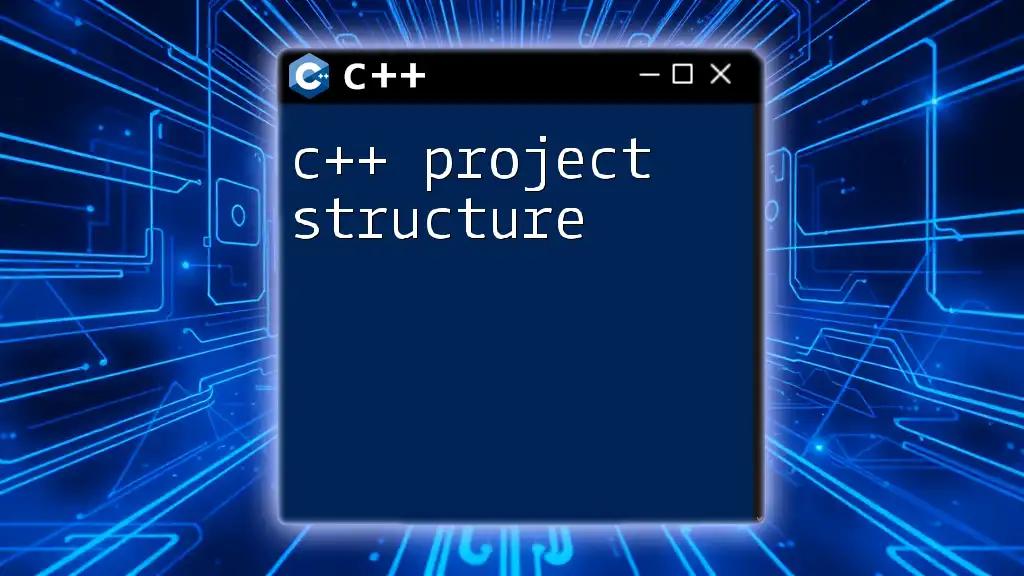
Conclusion
In summary, C++ private constructors are an important tool for enforcing encapsulation and controlling instantiation. By leveraging private constructors wisely, developers can implement efficient design patterns that lead to cleaner, more maintainable code. Whether applying these principles in real-world applications or experimenting in personal projects, understanding private constructors can enhance the quality of your software.
Embrace the practice of using private constructors and explore how they can help achieve better design strategies in your C++ programming endeavors.