A well-organized C++ project structure typically includes directories for source files, headers, and build outputs, promoting readability and maintainability in the development process.
Here's a simplified example of a C++ project structure:
MyProject/
├── src/ // Source files
│ ├── main.cpp
│ └── utils.cpp
├── include/ // Header files
│ ├── utils.h
└── build/ // Build files
And a sample `main.cpp` content might look like this:
#include "utils.h"
int main() {
// Your code here
return 0;
}
Understanding the Basics of C++ Project Structure
What is a C++ Project Structure?
A C++ project structure refers to the organization of files and directories in a C++ software development project. This structure is crucial as it directly affects the maintenance, readability, and scalability of the code. A well-defined project structure aids developers in locating files, understanding the workflow, and collaborating with team members.
For instance, a basic structure might look like this:
MyProject/
├── src/
│ └── main.cpp
├── include/
│ └── myheader.h
├── lib/
├── tests/
├── build/
└── README.md
Common Terminology
Understanding the terminology related to C++ project structures is essential. Familiar terms include:
- Source Files: The `.cpp` files where the main code resides.
- Header Files: Files with a `.h` extension that declare functions, classes, and variables.
- Build Systems: Tools like CMake or Make that automate the compilation process.
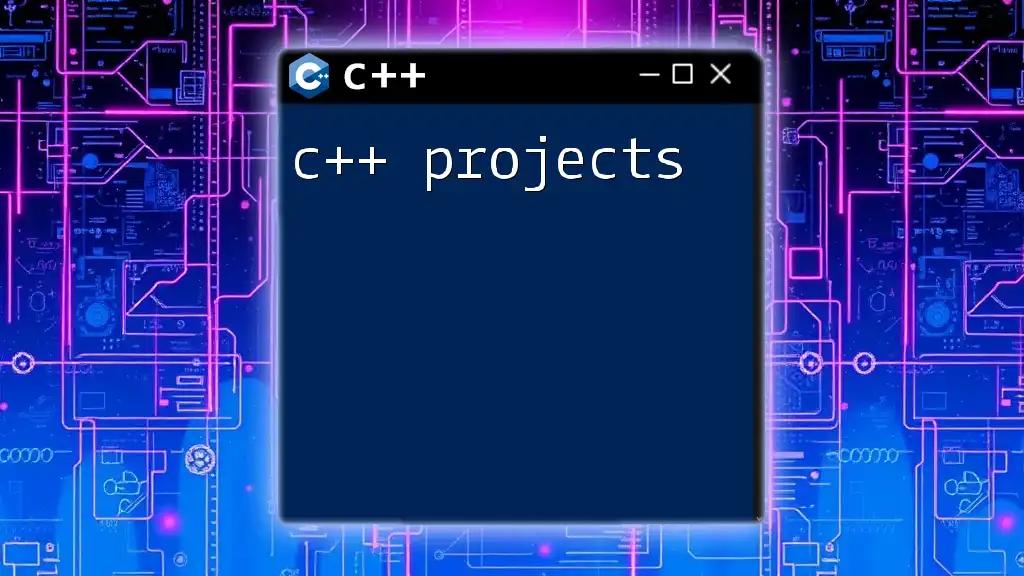
Typical C++ Project Layout
Root Directory
The root directory serves as the main entry point for the project. Key files often found here include:
- `README.md`: A file that typically contains a description of the project, installation instructions, and usage guides.
- `.gitignore`: This file tells Git which files to ignore when pushing to the repository, ensuring that unnecessary files don’t clutter version control.
`src` Directory
The `src` directory is where the source code lives. Organizing code into this directory allows for a clean separation of implementation from other files.
What Goes Here?
Every `.cpp` file associated with the project should be placed here. For instance, if you have a program with two parts, you might structure it as follows:
src/
├── main.cpp // Entry point
├── calculations.cpp // Implementation of calculations
└── utilities.cpp // General utility functions
File Naming Conventions
It’s crucial to adopt consistent file naming conventions. The name of the file should reflect its content. For example:
- Use descriptive names such as `math_functions.cpp` rather than generic names like `file1.cpp`.
- This helps other developers quickly identify its purpose and usage.
`include` Directory
The `include` directory houses header files. These are used to declare functions, structures, and classes that can be shared across multiple source files.
Role of Header Files
Header files allow for code reusability, enabling the same declarations to be included in different source files without duplication.
Organization Best Practices
A well-organized `include` directory might look like this:
include/
├── calculations.h
└── utilities.h
Each header should have a corresponding source file in the `src` directory, and it’s advisable to use guard macros to prevent multiple inclusions.
`lib` Directory
The `lib` directory is for managing third-party libraries. When you integrate libraries, keeping binary files and headers organized is key.
Example of Integrating External Libraries
If you're using a library like Boost, you might include it like this:
lib/
├── boost/
│ ├── libboost_system.a
│ └── boost/
Here, you can store your `Boost` library files separately from your project's code.
`tests` Directory
Testing is a vital part of software development, and a dedicated `tests` directory helps ensure constructed code is functional and reliable.
Why Testing is Essential
By organizing testing files, you allow easier maintenance and a clearer understanding of testing strategies.
Test Folder Structure
A simple test structure might include:
tests/
├── test_calculations.cpp
└── test_utilities.cpp
Example using Google Test Framework
Here’s a basic example of using the Google Test framework:
#include "gtest/gtest.h"
#include "calculations.h"
TEST(CalculationsTest, AddTest) {
EXPECT_EQ(add(2, 3), 5);
}
`build` Directory
The `build` directory is where the build artifacts are located. This is crucial for separating the compiled files from the source code.
What is the Build Directory?
When you compile a project, the binaries and object files will reside here if using a dedicated build directory. This practice keeps the directory clean and organized.
Managed Build Systems
Using a build system like CMake or Makefiles can simplify builds. A simple `CMakeLists.txt` for a project might look like this:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
set(CMAKE_CXX_STANDARD 11)
include_directories(include)
add_executable(MyProject src/main.cpp src/calculations.cpp src/utilities.cpp)
`docs` Directory
The `docs` directory is dedicated to documentation related to the project. Comprehensive documentation is critical for onboarding new developers and clarifies the project's usage.
Importance of Documentation
Good documentation encapsulates design decisions, API usage, and testing guidelines, making it easier for others to understand your code.
Structure of the Documentation Directory
A well-organized `docs` structure might include:
docs/
├── installation_guide.md
└── api_reference.md

Advanced C++ Project Structures
Modular Project Structure
What is Modular Design?
In larger projects, a modular project structure enhances reusability. Each module can encapsulate specific functionality with well-defined interfaces.
Benefits of Modularity
Through modular design, teams can work on separate modules, allowing for parallel development and easier testing.
Example of a Modular Project Structure
A modular structure can be visualized like this:
MyProject/
├── mod1/
│ ├── src/
│ ├── include/
│ └── tests/
├── mod2/
│ ├── src/
│ ├── include/
│ └── tests/
Multi-platform Support
Handling Different Platforms
When developing a cross-platform application, it's vital to structure your project to accommodate various operating systems, such as Linux, Windows, and Mac.
Conditional Compilation
Utilizing conditional compilation for platform-specific code can streamline development. For example:
#ifdef _WIN32
// Windows-specific code
#elif __linux__
// Linux-specific code
#endif
Version Control Integration
Importance of Version Control
Effective version control ensures that changes to the project can be tracked over time. Systems like Git are commonly used in software development.
Project Structure Considerations for Version Control
When utilizing version control, it’s essential to set up your `.gitignore` file correctly to avoid committing unnecessary files, like build outputs or temporary files.
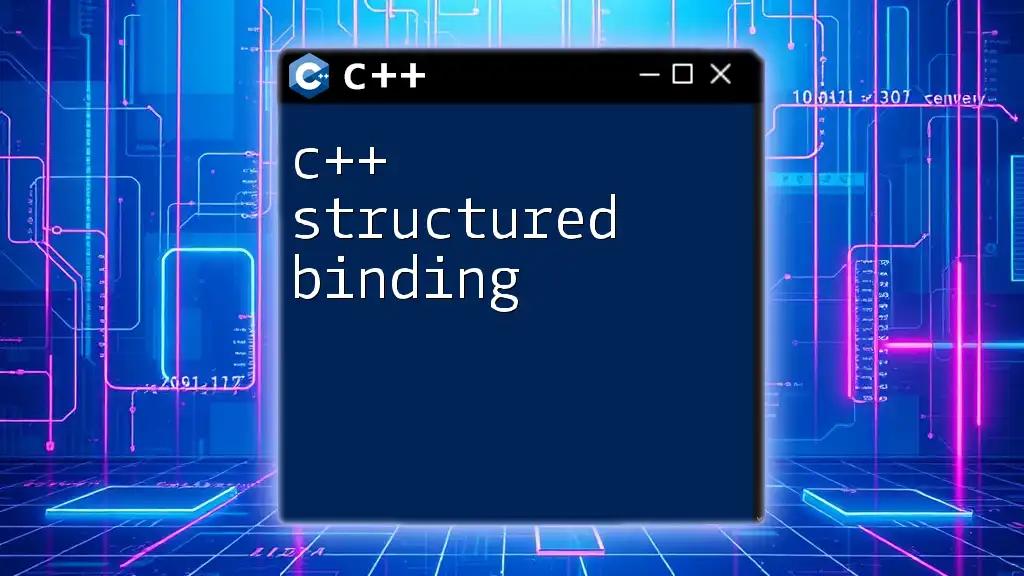
Best Practices for C++ Project Structure
Consistency Is Key
Maintaining consistency in your C++ project structure makes it easier for everyone involved in the project. This consistency helps avoid confusion and miscommunication.
Keep It Simple
Never over-complicate your structure. A straightforward approach enhances the understanding of the codebase for both current and future developers.
Document Your Structure
Incorporate README files and inline comments to outline the purpose of directories and files. This documentation helps onboard new developers and clarifies the project’s workflow.
Regular Refactoring
Refactoring the structure at regular intervals can keep it clean and efficient, allowing the incorporation of best practices and improvements over time.
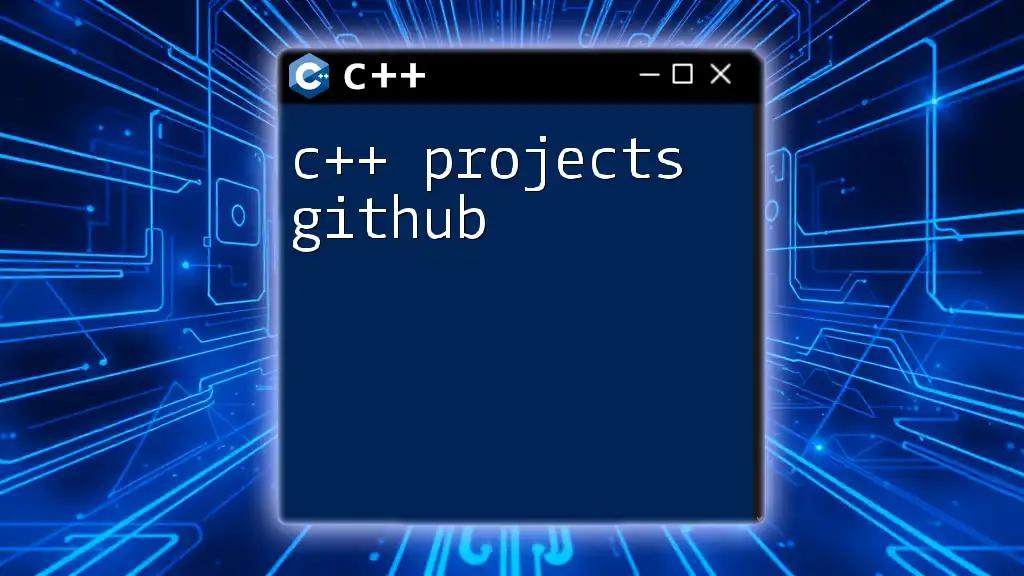
Conclusion
A well-defined C++ project structure serves as the backbone of an efficient development process. By implementing these organizational strategies, you enhance collaboration, maintainability, and scalability within your projects. As you embark on your software development journey, consider these principles to make your C++ projects a success.
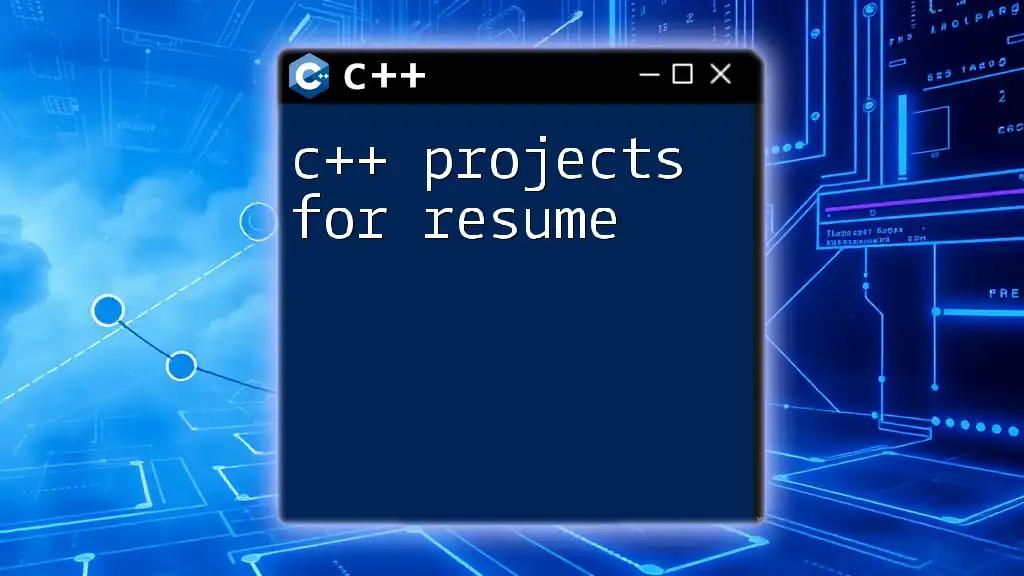
Additional Resources
For more insights, consider exploring books, articles, and online resources that dive deeper into C++ project structuring and best practices. Tools like CMake and version control systems will be invaluable in your quest for a well-structured project.