A C++ project typically involves writing a structured program that utilizes C++ commands to accomplish a specific task, such as the following example that demonstrates a simple "Hello, World!" application:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++ Programming
Setting Up Your Environment
To embark on your C++ project journey, you need to set up your development environment. First, choose an Integrated Development Environment (IDE) that suits your needs. Popular choices include:
- Visual Studio: A powerful IDE with extensive features, particularly for Windows users.
- Code::Blocks: A user-friendly, open-source option available on multiple platforms.
- Eclipse: An IDE known for its versatility which can be extended with various plugins.
Once you've selected an IDE, install it by following the on-screen instructions. Ensure you have a C++ compiler like GCC or MSVC ready for building your projects.
Basic C++ Concepts
Before diving into actual projects, it's crucial to understand some fundamental C++ concepts:
- Variables and Data Types: Variables store data, and C++ supports various data types such as `int`, `float`, and `char`.
- Control Structures: Loops (`for`, `while`) and conditional statements (`if`, `switch`) help in controlling the flow of the program.
- Functions: Functions allow you to modularize your code and make it reusable. Understanding how to declare and invoke functions is essential for any C++ project.
- Classes and Objects: C++ is an object-oriented language, and knowing how to create classes and objects is pivotal for developing larger applications.
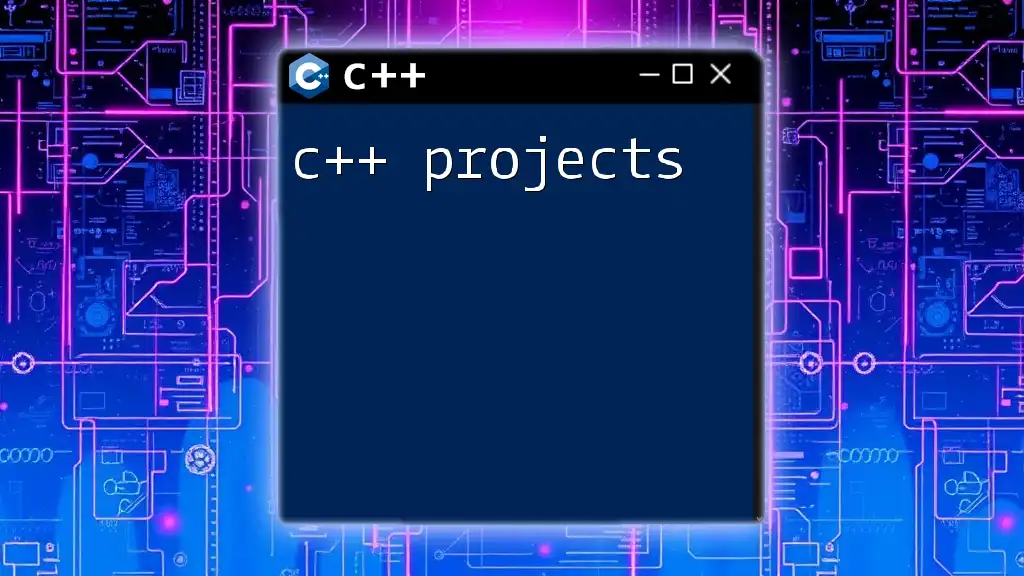
Popular C++ Coding Projects
What is a C++ Coding Project?
A C++ coding project involves creating a standalone application or program that applies various aspects of C++ programming. These projects not only reinforce learning but also enhance problem-solving skills and logical thinking.
Types of Projects for C++
C++ projects can be categorized into several types, allowing you to tailor your learning experience:
- Console Applications: Simple applications that run in a command line or terminal.
- GUI Applications: Graphical User Interface applications, often using libraries like Qt or SFML.
- Game Development: Creating games, which challenges your programming and logical skills.
- Systems Programming: Projects that interact closely with hardware or operating systems.
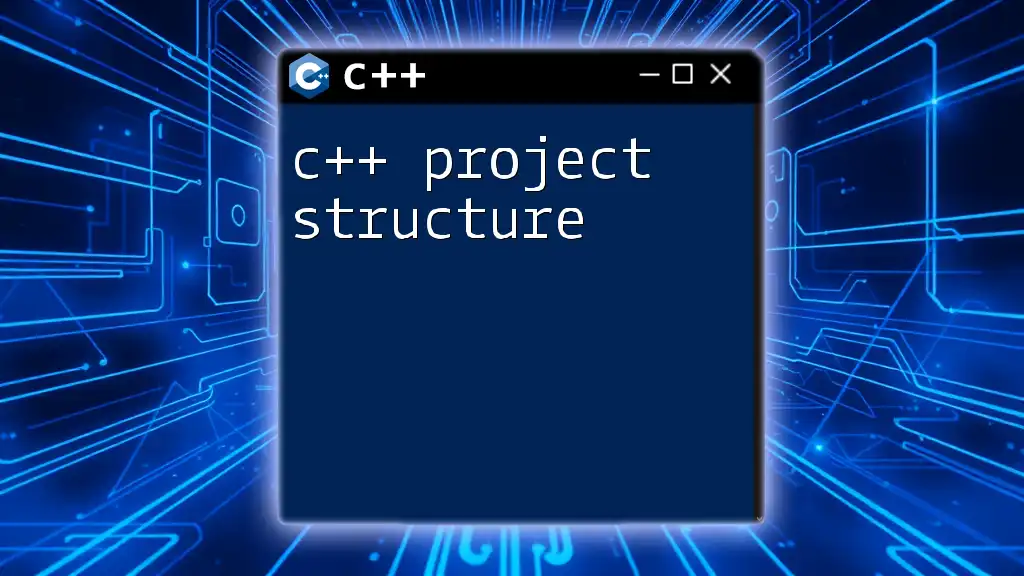
Project Ideas for C++ Beginners
Simple Calculator
Creating a simple calculator is a fantastic starting point for beginners. The objective is to implement basic arithmetic operations which help to solidify control structures and functions.
Example Code Snippet:
#include <iostream>
using namespace std;
int main() {
float num1, num2;
char operation;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter second number: ";
cin >> num2;
cout << "Choose operation (+, -, *, /): ";
cin >> operation;
switch (operation) {
case '+':
cout << "Result: " << num1 + num2;
break;
case '-':
cout << "Result: " << num1 - num2;
break;
case '*':
cout << "Result: " << num1 * num2;
break;
case '/':
cout << "Result: " << num1 / num2;
break;
default:
cout << "Invalid operation!";
}
return 0;
}
This project introduces users to basic input and output operations, along with switch-case statements.
Tic-Tac-Toe Game
Building a Tic-Tac-Toe game reinforces user input handling, loops, and conditional checks while adding an element of fun.
Example Code Snippet:
#include <iostream>
using namespace std;
char board[3][3] = {{'1', '2', '3'},
{'4', '5', '6'},
{'7', '8', '9'}};
// Additional functions for game logic go here
This project provides a great platform to learn about arrays and game logic flow.
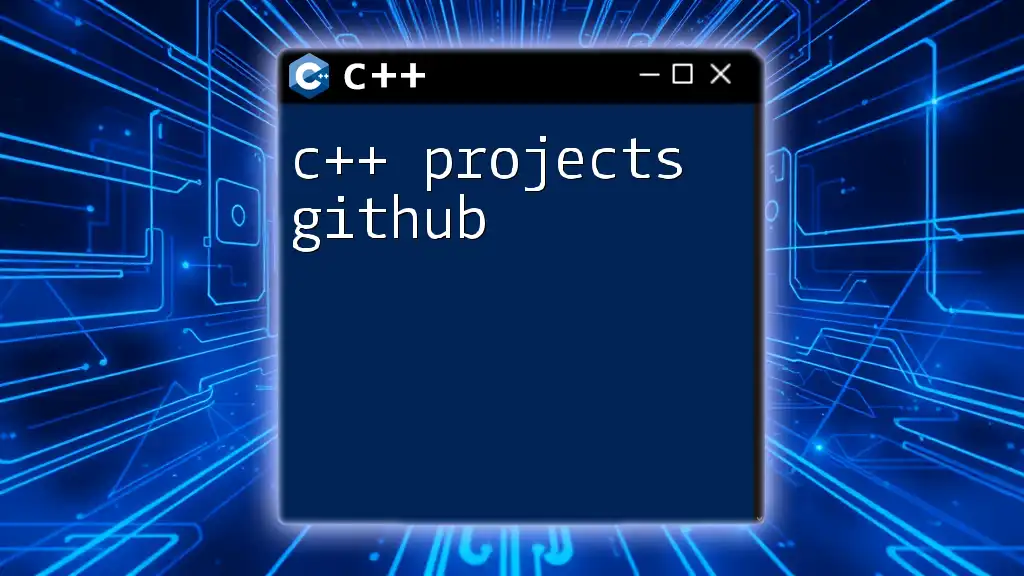
Intermediate C++ Project Ideas
Expense Tracker
An expense tracker can help you learn about data storage and management, as well as the use of classes and file handling.
Description & Objective: This console application enables users to input, categorize, and view expenses, allowing for better financial management. Using classes effectively manages individual expenses and aggregates them.
Example Code:
class Expense {
public:
string category;
float amount;
void display() {
cout << "Category: " << category << ", Amount: " << amount << endl;
}
};
With the Expense class, you encapsulate data and create functions for better organization.
Library Management System
Creating a library management system refines your skills in managing data and working with complex structures.
Description & Objective: This project requires you to build a system that can manage book inventories and track member borrowing.
Sample Code Snippet:
class Book {
public:
string title;
string author;
bool isAvailable;
void display() {
cout << title << " by " << author << (isAvailable ? " is available." : " is not available.") << endl;
}
};
By using classes to represent books and managing their state, students learn to work with larger systems.
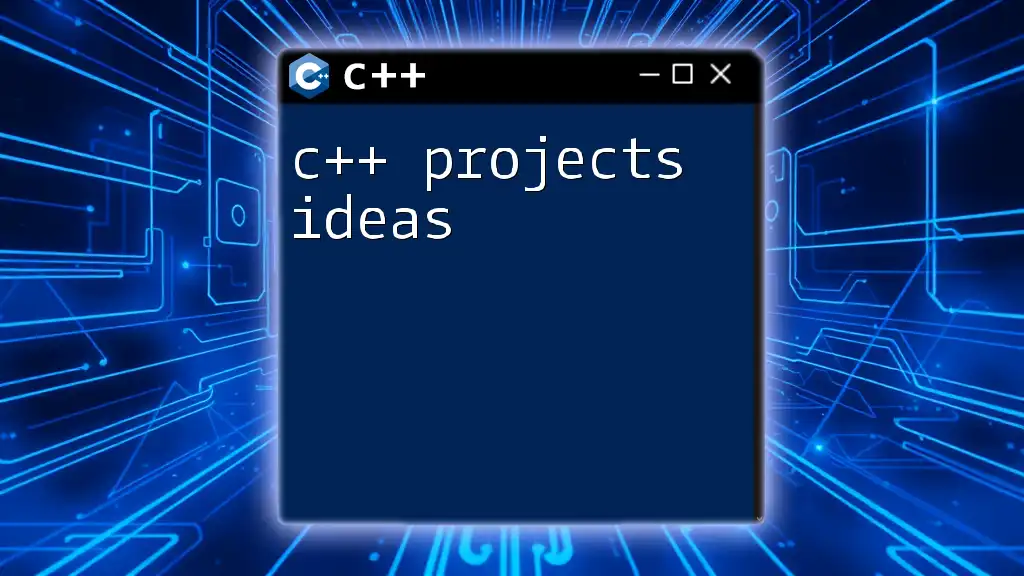
Advanced C++ Project Ideas
Chat Application
Developing a chat application is an ambitious yet rewarding endeavor. It requires understanding of networking, concurrency, and user interface design.
Description & Objective: This project involves creating a console or GUI-based application where users can exchange messages in real-time. Familiarity with sockets and multi-threading is essential.
Game Engine
For those interested in game development, building a simplistic game engine opens doors to graphics programming and design patterns.
Description & Objective: This project encompasses creating a basic engine capable of rendering shapes, managing game loops, and handling user input.
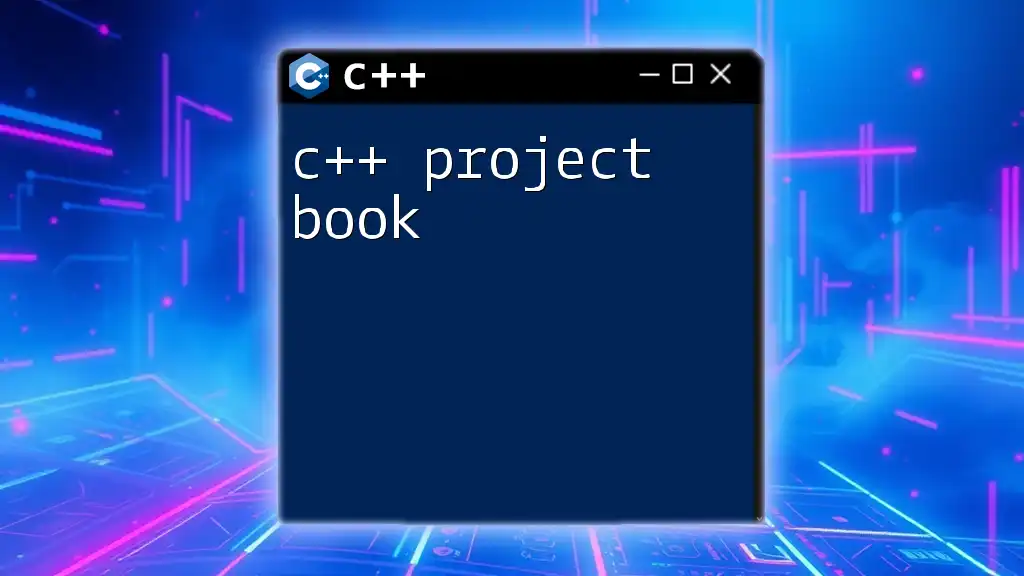
Best Practices for C++ Projects
Code Organization
Organizing your code is critical for maintaining clarity and ease of updates. Group related functions and classes into separate files, and use meaningful names for files, functions, and variables.
Version Control
Implementing a version control system, such as Git, is invaluable for tracking code changes. It enables collaboration with others, fosters backup practices, and allows experimentation without losing work.
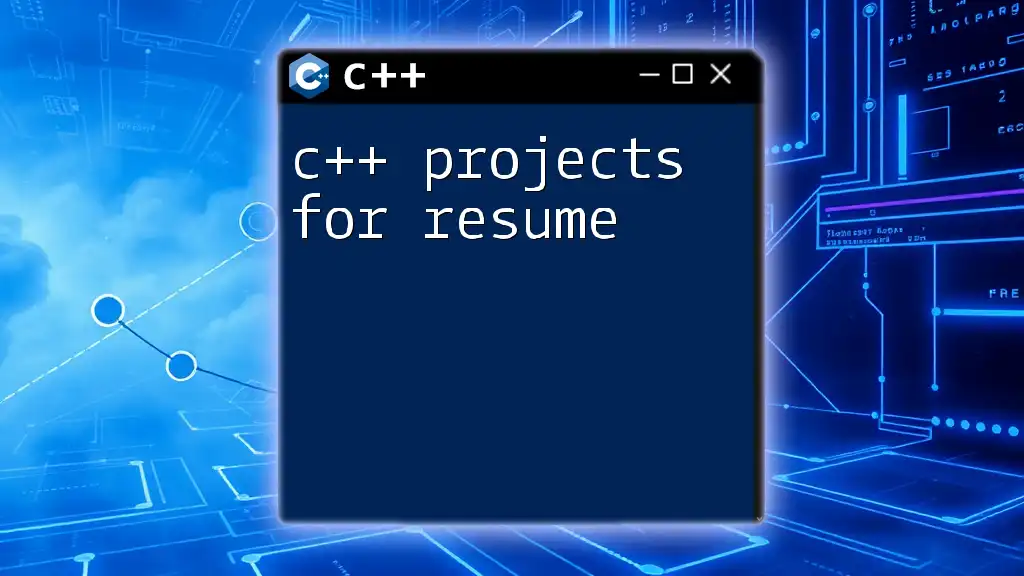
Learning Resources for C++ Projects
Online Tutorials and Courses
To deepen your understanding, explore online platforms like Codecademy, Coursera, and freeCodeCamp. Each offers structured pathways for C++ learning, from beginner to advanced levels.
Books and References
Consider reading classics like "C++ Primer" by Lippman or "Effective C++" by Meyers, which provide in-depth knowledge and best practices.
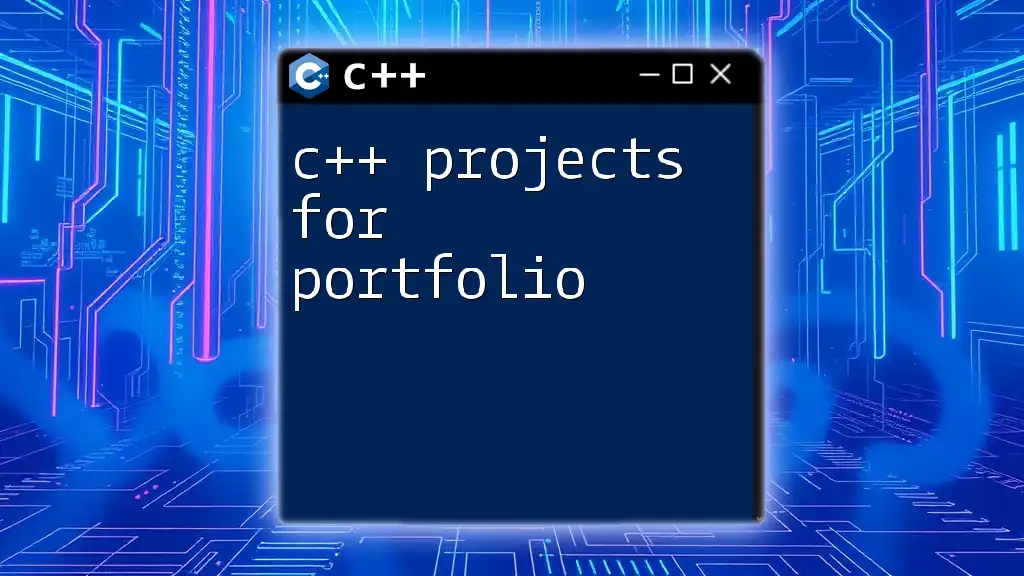
Conclusion
Engaging in a C++ project is an essential pathway to mastery. By applying concepts learned through various projects, you enhance not only your coding skills but also your problem-solving capabilities. No matter your current level, there’s always a project that can challenge you and propel your learning further.
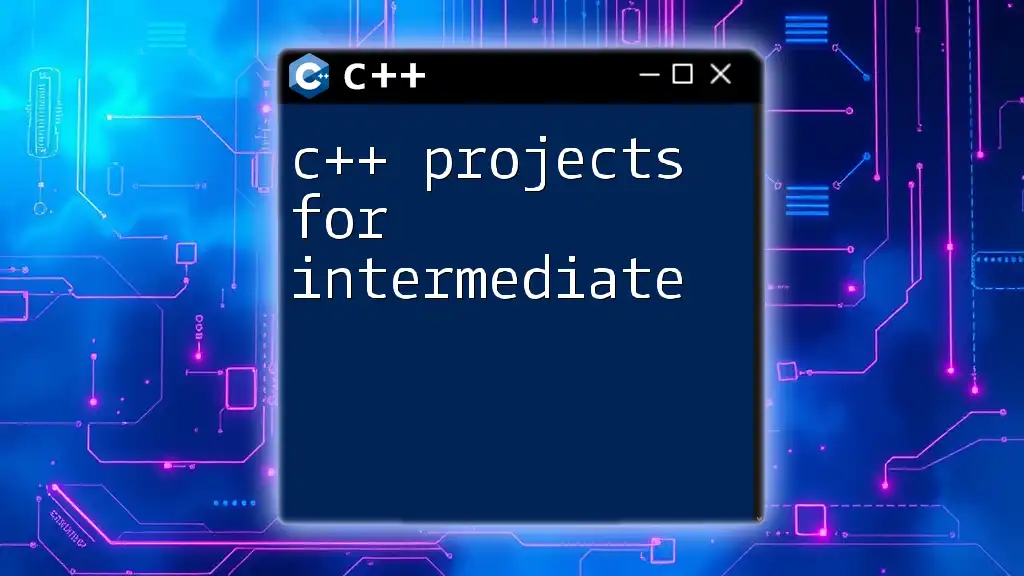
Call to Action
Choose a C++ project that intrigues you and start building today! Feel free to share your project ideas or questions in the comments section below.