A "C++ project book" serves as a comprehensive guide, offering practical examples and projects to help learners efficiently master C++ commands through hands-on coding.
Here's a simple code snippet demonstrating a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Introduction to C++ Project Books
Purpose of C++ Project Books
A C++ project book serves as an invaluable resource designed to accelerate your learning process in C++. It combines theoretical concepts with practical applications, allowing both budding and experienced programmers to explore a variety of projects. Central to these books are hands-on projects that help you solidify your understanding of C++ concepts as you implement them in real-world scenarios.
Who Can Benefit from a C++ Project Book
C++ project books can benefit several types of learners:
- Beginners looking to grasp the fundamentals will find structured projects that guide them through the basics of programming in C++.
- Intermediate programmers seeking to refine and expand their skill set can tackle projects that challenge their understanding of more complex concepts and paradigms.
- Advanced developers wanting to explore new project ideas and frameworks can find advanced projects that push their boundaries and deepen their expertise.
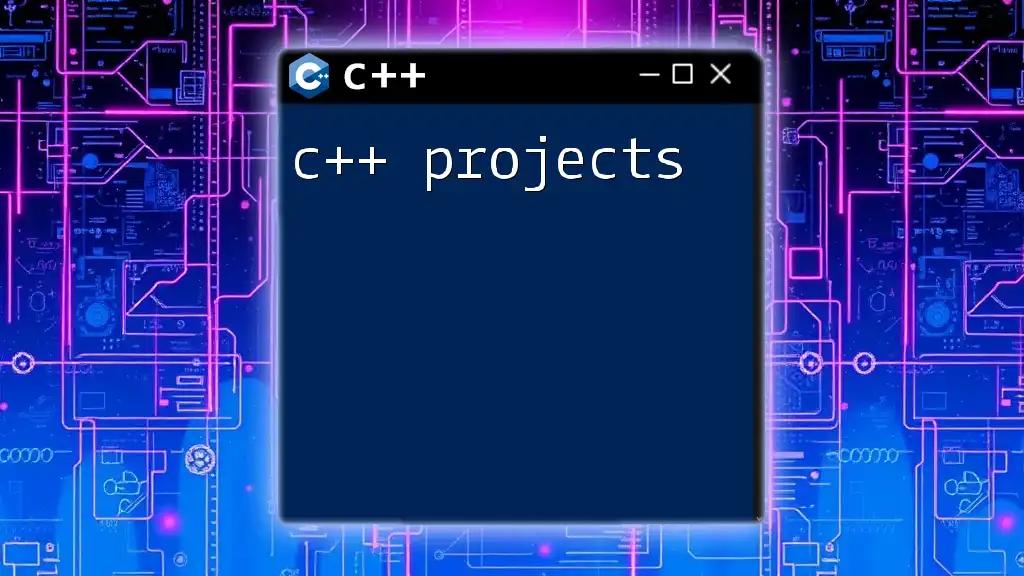
Understanding C++ and Its Projects
What is C++?
Brief History and Evolution
C++ was first developed by Bjarne Stroustrup in the late 1970s as an enhancement to the C programming language. It introduced Object-Oriented Programming (OOP) principles, allowing developers to create more reusable and modular code. Over the years, C++ has evolved with several new standards (C++11, C++14, C++17, and C++20), each bringing new features and performance improvements.
Why Choose C++ for Projects?
Choosing C++ for your projects can be particularly advantageous due to its balance between high performance, control over system resources, and rich set of libraries. This versatility makes it ideal for various applications, including graphics programming, game development, and system-level work.
Types of C++ Projects
Beginner Projects
For newcomers, beginner projects are crucial for laying a solid foundation. Projects often include:
- Simple console applications, such as creating a basic calculator that can perform different arithmetic operations, or building a simple to-do list manager that allows users to add, delete, and view tasks.
Here’s a simple example of a calculator in C++:
#include <iostream>
using namespace std;
int main() {
double num1, num2;
char oper;
cout << "Enter first number: ";
cin >> num1;
cout << "Enter an operator (+, -, *, /): ";
cin >> oper;
cout << "Enter second number: ";
cin >> num2;
switch (oper) {
case '+':
cout << num1 + num2 << endl;
break;
case '-':
cout << num1 - num2 << endl;
break;
case '*':
cout << num1 * num2 << endl;
break;
case '/':
cout << num1 / num2 << endl;
break;
default:
cout << "Invalid operator!" << endl;
break;
}
return 0;
}
Intermediate Projects
Once you have grasped the fundamentals, you can move towards intermediate projects like:
- File handling applications, where you can create a text editor or a file organizer.
- Mini-games like Tic-Tac-Toe or Hangman, which help you explore concepts such as loops and arrays.
Advanced Projects
For seasoned programmers, advanced projects broaden horizons significantly. You might consider:
- Game development using frameworks like SDL or SFML, where you can create a fully functional game from scratch.
- System programming projects, like creating an operating system or scripting up a device driver, challenging your knowledge of underlying systems.
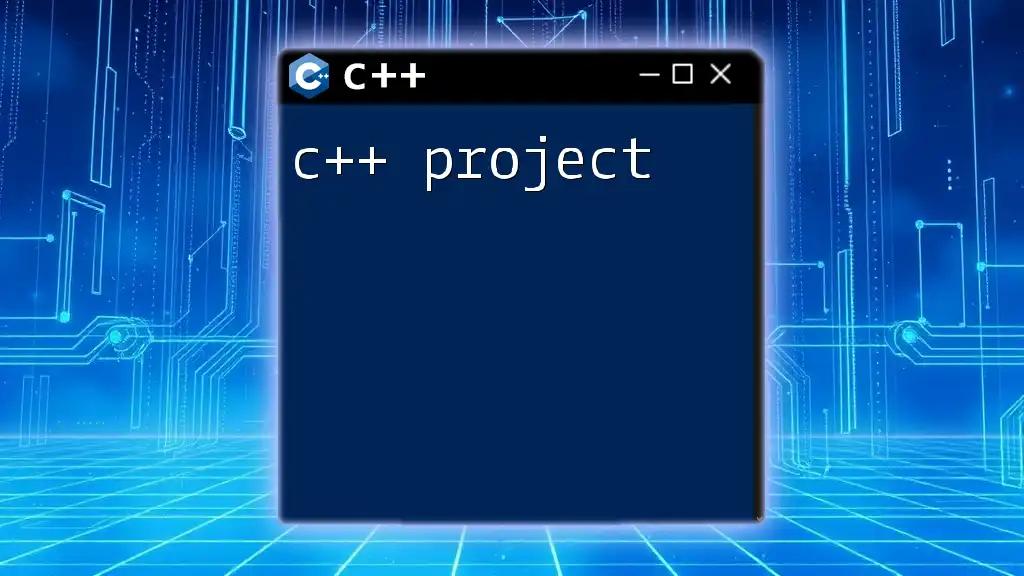
What to Look for in a C++ Project Book
Key Features of an Effective C++ Project Book
Clear Project Instructions
Clarity in project instructions is paramount. A good book will provide detailed steps for setting up the environment, explaining concepts, and running the projects. This clarity helps prevent confusion and accelerates learning.
Code Snippets and Examples
Learning from code snippets that are well-commented and structured can greatly enhance comprehension. Ensure the book you choose includes ample examples that illustrate complex topics in an accessible manner.
Concepts Behind Projects
Understanding the underlying concepts, such as OOP principles or data structures, is crucial. An effective project book will not only guide you through projects but also explain why certain methods or algorithms are used.
Choosing the Right Book
Reading Reviews and Recommendations
It is wise to read reviews and seek recommendations from experienced programmers when selecting a project book. Many resource websites list books considered essential for C++ development.
Online vs. Printed Books
Consider whether you prefer online or printed formats. Digital books offer the convenience of quick searches and links, while printed books provide a tactile experience that some learners prefer.
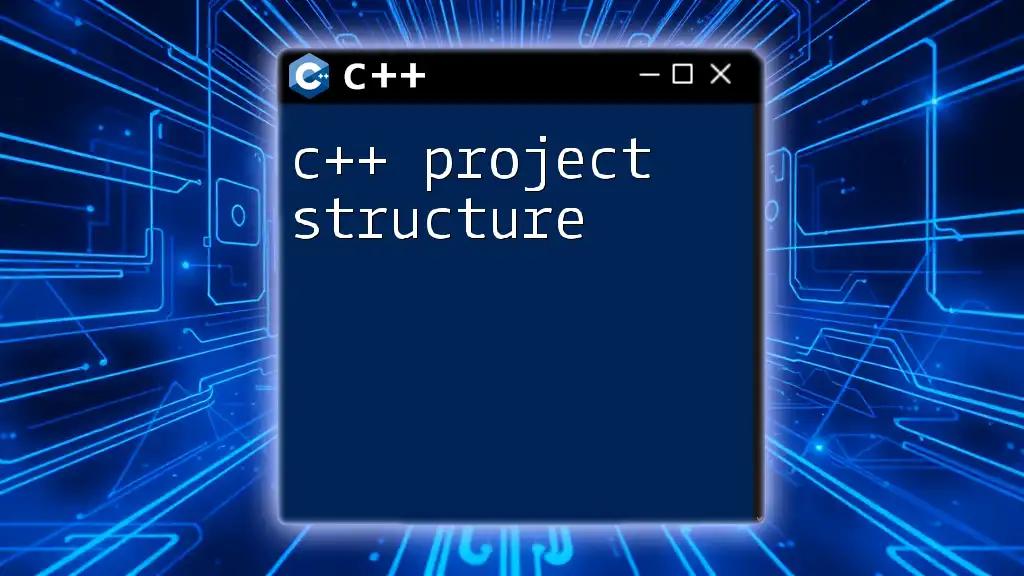
Recommended C++ Project Books
Top Titles for Beginners
"C++ Primer" by Stanley B. Lippman
This classic book offers a broad introduction to the language and includes numerous relevant projects. It emphasizes understanding as you progress, making it an excellent choice for beginners.
"Beginning C++ Through Game Programming" by Mickey Mouse
This book engages readers with gamified projects, providing an exciting approach to learning C++ while building simple games. It’s a fun way to hone your skills in a dynamic way.
Titles for Intermediate to Advanced Programmers
"Effective C++" by Scott Meyers
For those who have a handle on the basics, this book covers essential programming principles and best practices in a series of thoughtfully crafted projects, enhancing your coding efficiency.
"The C++ Programming Language" by Bjarne Stroustrup
Penned by the creator of C++, this comprehensive resource covers advanced features through insightful project examples, perfect for developers looking to expand their toolkit.
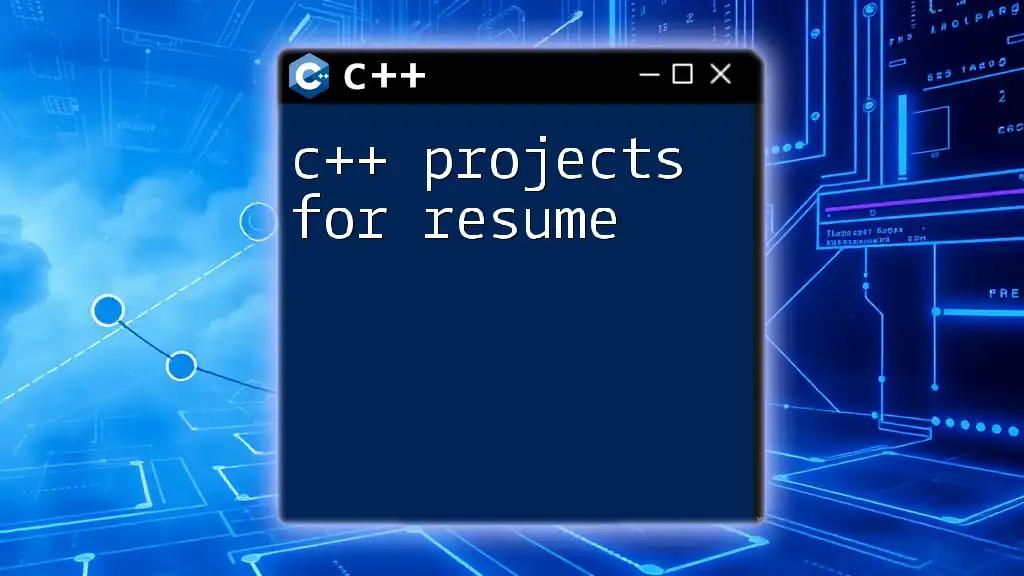
How to Approach Projects in a C++ Project Book
Step-by-Step Project Execution
Understanding the Project Objective
Before starting any project, it’s essential to understand its objective fully. Assess what you aim to learn through this project—be it new syntax or concepts like OOP—and formulate a plan for execution.
Writing Clean and Efficient Code
Importance of Code Readability
Writing clean code greatly enhances your ability to maintain and debug it later. Use meaningful variable names, consistent indentation, and comments to explain crucial parts of your code.
Efficient Handling of Resources
Memory management is a critical aspect of C++ programming. Utilize smart pointers and clear data structure management practices to reduce memory leaks and improve efficiency.
Debugging and Testing Your Projects
Techniques for Debugging C++ Code
Be prepared to encounter bugs and errors; this is part of the learning process. Employ debugging tools like GDB to trace your code, and don't hesitate to use print statements to monitor variable states during execution.
Unit Testing in C++
Incorporating a testing framework such as Google Test allows you to conduct unit tests on your code, ensuring each component functions as expected. This practice will help you catch bugs early and enhance code quality.
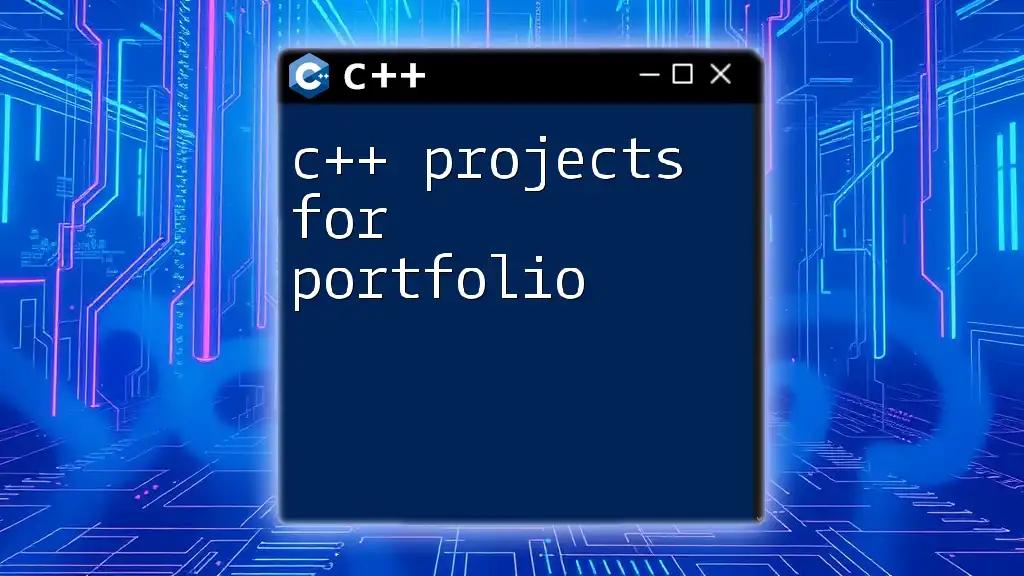
Conclusion
A C++ project book is a powerful tool for anyone looking to deepen their knowledge and skills in programming with C++. By providing structured projects and clear guidelines, these resources make it easier to learn complex concepts and apply them practically. As you embark on your journey with a C++ project book, choose one that aligns with your current level and goals, and don’t hesitate to dive into hands-on coding. The world of C++ awaits you, full of opportunities and challenges that will help you grow as a developer.
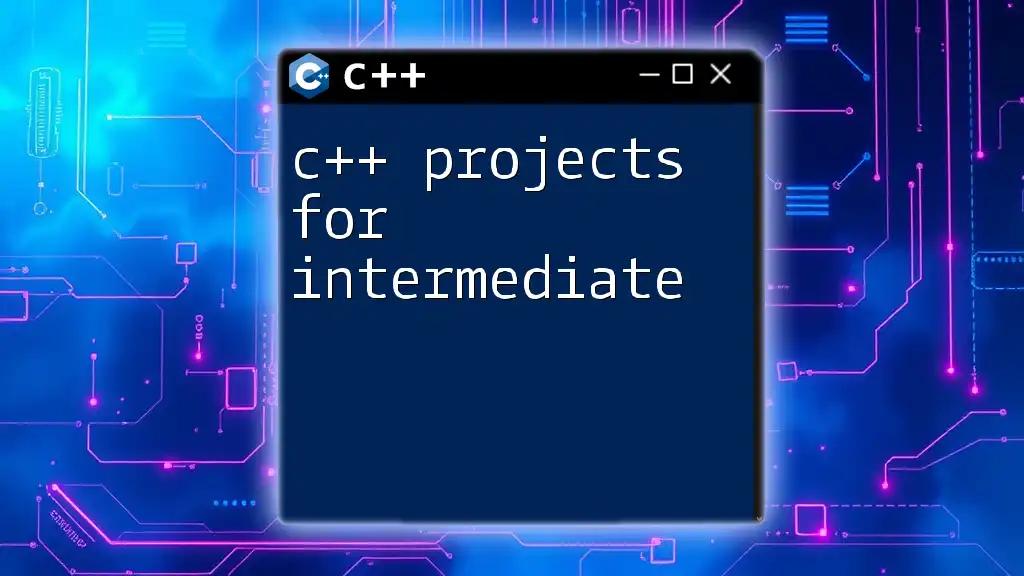
Additional Resources
While C++ project books are incredibly valuable, you might also want to explore online communities and forums such as Stack Overflow and GitHub, where you can engage with other learners and experienced programmers. In addition, consider enrolling in tutorials and courses available on platforms like Coursera or Udemy to complement your learning. They often provide additional examples and projects that can enrich your understanding of C++.