In C++, `std::atomic<bool>` is a data type that provides a thread-safe boolean variable, allowing for safe concurrent reads and writes without the need for explicit locks.
#include <atomic>
#include <iostream>
#include <thread>
std::atomic<bool> flag{false};
void setFlag() {
flag.store(true);
}
int main() {
std::thread t(setFlag);
t.join();
std::cout << "Flag is " << flag.load() << std::endl; // Output: Flag is 1
return 0;
}
Understanding Atomic Types
What are Atomic Types?
Atomic types in C++ are special types that support operations which are guaranteed to be performed in a single step, meaning they complete without interruption. The importance of atomicity in multithreading cannot be overstated, as it prevents data races where multiple threads attempt to read and write shared data simultaneously, leading to inconsistencies and unpredictable behavior.
Benefits of Using Atomic Types
Utilizing atomic types offers several benefits in concurrent programming:
-
Thread Safety: By ensuring that operations on atomic types are indivisible and provide memory visibility guarantees, you reduce the risk of data races and improve the integrity of shared data.
-
Performance Considerations: Depending on the programming context, atomic operations can be more efficient than using mutexes or other locking mechanisms, particularly for simple operations.
-
Use Cases: Scenarios where atomic types shine include flags for synchronization, counters that are incremented by multiple threads, and status indicators in multithreaded applications.
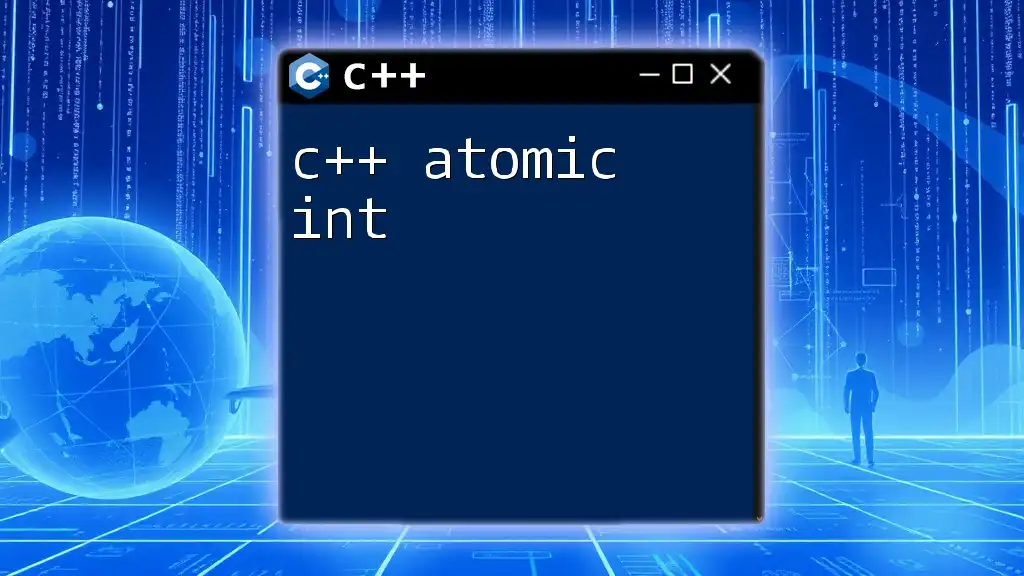
Introduction to std::atomic<bool>
What is std::atomic<bool>?
`std::atomic<bool>` is a specialized atomic type defined in the C++ standard library. It allows for safe manipulation of a boolean value across multiple threads. The significant difference between a regular `bool` and `std::atomic<bool>` is that the latter guarantees atomic operations, making it safe for concurrent usage.
Basic Operations on std::atomic<bool>
Initializing std::atomic<bool>
To declare and initialize an atomic boolean variable, use the following syntax:
#include <atomic>
std::atomic<bool> myAtomicBool(false); // Initialized to false
This initializes `myAtomicBool` to `false`, allowing it to be safely modified by multiple threads.
Loading and Storing Values
You can interact with `std::atomic<bool>` using the `load()` and `store()` methods. For instance:
myAtomicBool.store(true); // Store true
bool currentValue = myAtomicBool.load(); // Load the current value
These methods enable loading a value or storing a new one while ensuring that the operations are atomic.
Atomic Operations on std::atomic<bool>
Compare and Exchange
One of the powerful features of `std::atomic<bool>` is the ability to implement a compare-and-exchange operation. This is essential for establishing control over state changes in concurrent environments. The functions `compare_exchange_weak` and `compare_exchange_strong` allow you to conditionally set a new value:
bool expected = false;
if (myAtomicBool.compare_exchange_strong(expected, true)) {
// Success: myAtomicBool is now true
}
In this code, `compare_exchange_strong` checks if `myAtomicBool` is `expected` (in this case, `false`). If it is, it sets `myAtomicBool` to `true` and returns `true`. If not, it sets `expected` to the current value of `myAtomicBool`, allowing you to handle the failure condition appropriately.
Boolean Operations
Logical operations on atomic booleans can offer additional control within multi-threaded applications. While `std::atomic<bool>` does not directly support logical operators, you can implement logical behavior with conditional statements as shown in the previous sections or by utilizing bit manipulation techniques, depending on your specific requirements.
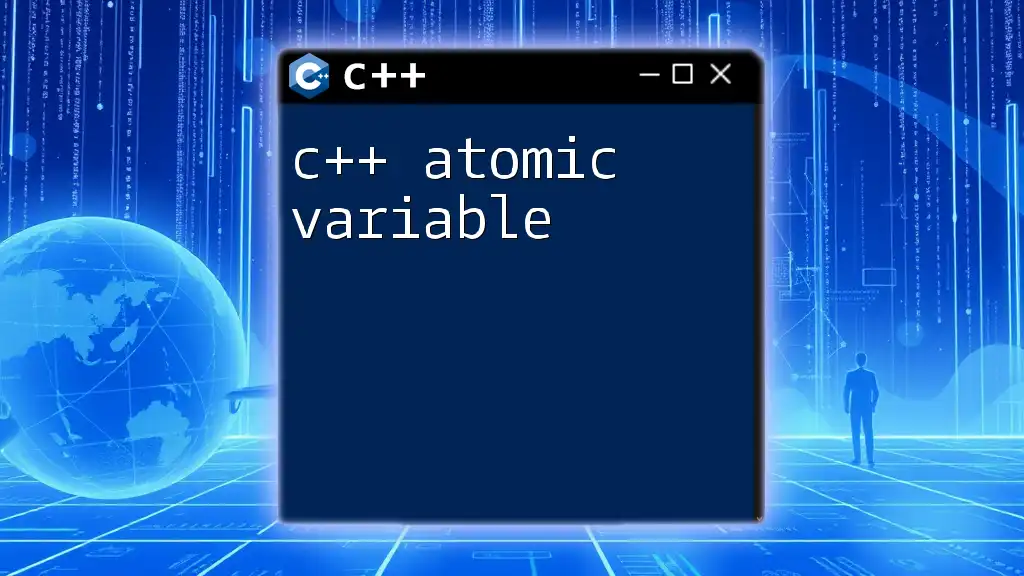
Practical Examples of std::atomic<bool>
Use Case: Flag Control in Multithreading
A common use case for `std::atomic<bool>` is controlling flags between producer and consumer threads. Here’s an example that illustrates its utility:
#include <iostream>
#include <atomic>
#include <thread>
std::atomic<bool> ready(false);
void producer() {
// Produce data (simulated with a sleep)
std::this_thread::sleep_for(std::chrono::seconds(1));
ready.store(true); // Indicate that the data is ready
}
void consumer() {
while (!ready.load()); // Wait for data to be ready
std::cout << "Data is ready!" << std::endl;
}
int main() {
std::thread p(producer);
std::thread c(consumer);
p.join();
c.join();
return 0;
}
In this example, the `producer` thread simulates data production, while the `consumer` thread waits for the `ready` flag to turn `true`, thus ensuring a controlled mechanism for synchronization between threads.
Use Case: Synchronization in Threads
Synchronization mechanisms between threads can also leverage `std::atomic<bool>`. Consider a scenario where you want to toggle a feature based on user inputs processed across threads:
#include <iostream>
#include <atomic>
#include <thread>
#include <chrono>
std::atomic<bool> toggle(false);
void toggleThread() {
while (true) {
toggle.store(!toggle.load());
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
}
void checkThread() {
while (true) {
if (toggle.load()) {
std::cout << "Toggle is ON" << std::endl;
} else {
std::cout << "Toggle is OFF" << std::endl;
}
std::this_thread::sleep_for(std::chrono::milliseconds(200));
}
}
int main() {
std::thread t(toggleThread);
std::thread c(checkThread);
t.join();
c.join();
return 0;
}
This code snippet creates two threads, `toggleThread` which continuously toggles the `toggle` variable, and `checkThread` which prints the current state of the toggle. This demonstrates how `std::atomic<bool>` can facilitate communication and synchronization between threads effectively.
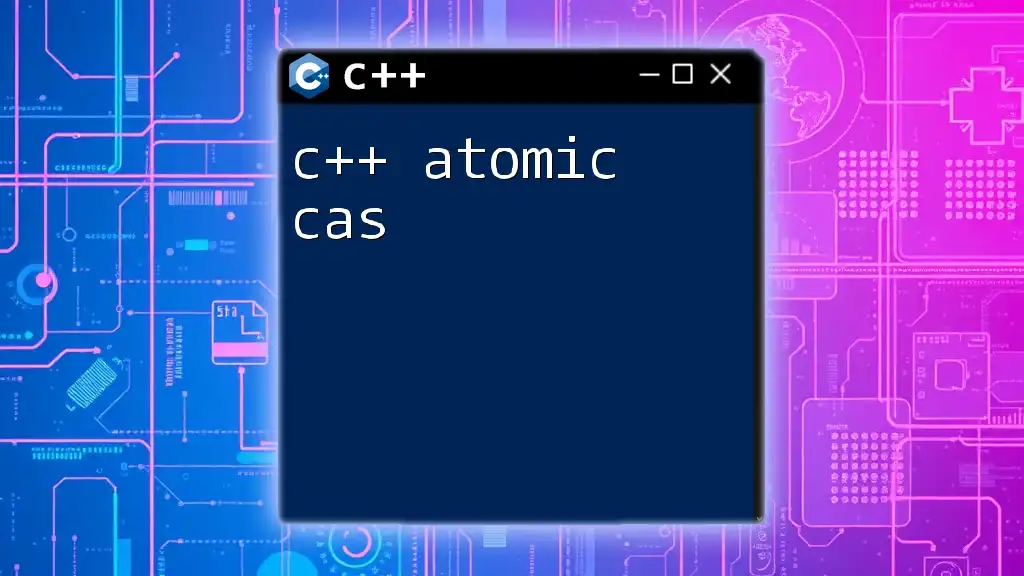
Best Practices for Using std::atomic<bool>
Guidelines for Thread Safety
To ensure thread safety when working with `std::atomic<bool>`, consider the following guidelines:
- Always use atomic types when sharing data among multiple threads to avoid data races.
- Prefer atomic operations over locks when the logic is simple, as they can minimize overhead while maintaining concurrency.
- Understand the memory model: be cautious about memory visibility and the behavior of your compiler and CPU architecture.
Performance Considerations
While atomic types are often more efficient than mutexes for simple operations, misuse can lead to performance degradation if overused or used in complex scenarios. Evaluate your application to determine whether atomic types or traditional locking mechanisms are more appropriate, especially in cases of high contention.
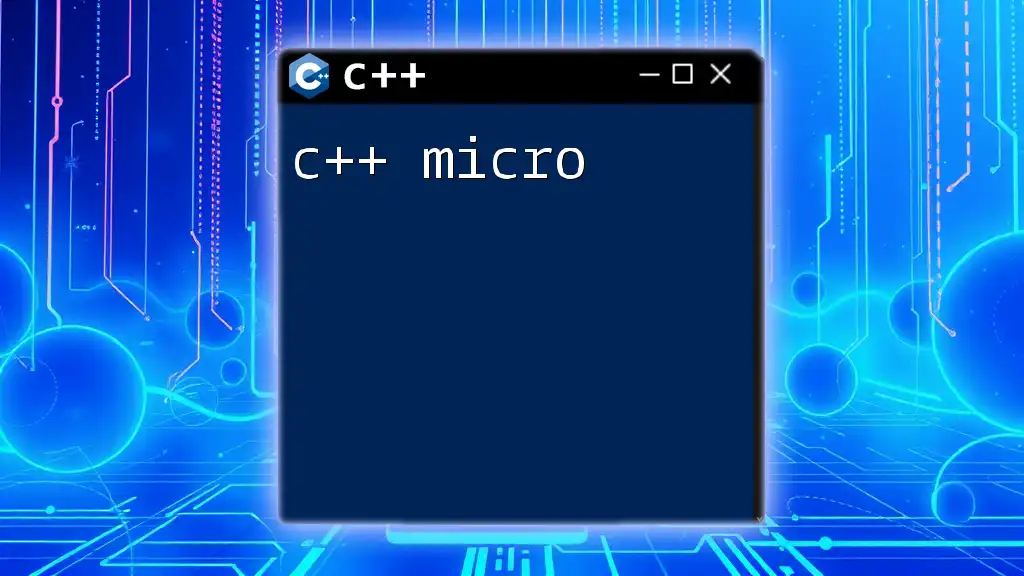
Conclusion
The `std::atomic<bool>` is an invaluable tool in the arsenal of C++ programmers, especially when designing concurrent applications. Its ability to facilitate safe interaction with boolean states among multiple threads is crucial in preventing data races and ensuring integrity. By understanding how to effectively use `std::atomic<bool>`, including its operations and best practices, you can enhance the reliability and performance of your multithreaded applications. Explore further and consider implementing `std::atomic<bool>` in your own projects for a more robust and safe concurrent programming experience.
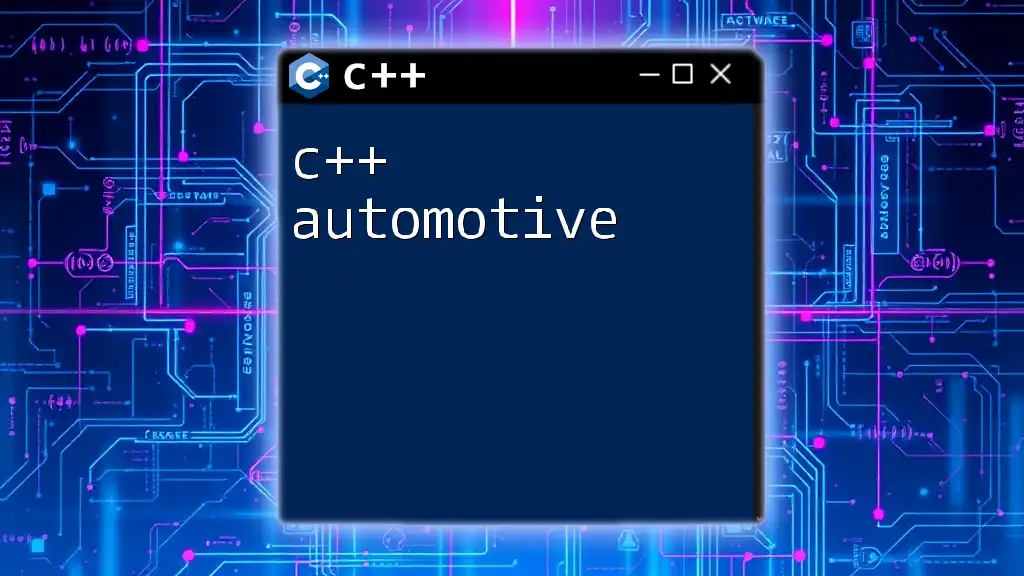
Additional Resources
For those interested in diving deeper into the topic of `C++ atomic bool` and associated best practices, consider the following resources:
- [C++ Reference - std::atomic](https://en.cppreference.com/w/cpp/atomic/atomic)
- ["C++ Concurrency in Action" by Anthony Williams](https://www.manning.com/books/c-concurrency-in-action)
- Online courses and tutorials focused on multithreading and concurrency in C++.
By taking advantage of the built-in features provided by C++, you can harness the power of atomic operations to streamline your multithreaded programming efforts.