The `lower_bound` function in C++ is used to find the first position in a sorted range where a given value can be inserted without violating the order, effectively returning an iterator to that position.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 4, 4, 5, 6};
int value = 4;
auto it = std::lower_bound(vec.begin(), vec.end(), value);
std::cout << "The lower bound of " << value << " is at position: " << (it - vec.begin()) << std::endl;
return 0;
}
What is `lower_bound`?
`lower_bound` is a powerful function available in the C++ Standard Library, designed specifically to perform efficient searches in sorted ranges. It helps find the first position where a given value can be inserted while maintaining the order of the range. When working with sorted data, the ability to quickly locate elements or insertion points is crucial for optimizing algorithms.
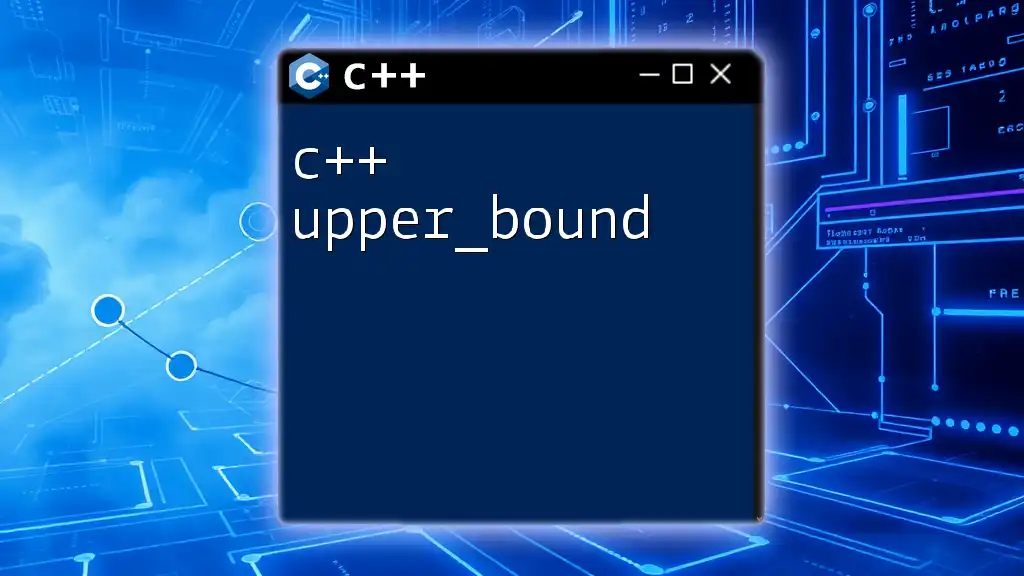
Syntax of `lower_bound`
The syntax for using `lower_bound` is straightforward:
template<class ForwardIt, class T>
ForwardIt lower_bound(ForwardIt first, ForwardIt last, const T& value);
-
Parameters:
- `first`: An iterator pointing to the beginning of the range.
- `last`: An iterator pointing to one past the end of the range.
- `value`: The value you want to find.
-
Return Value: It returns an iterator pointing to the first element that is not less than `value` (i.e., greater than or equal to `value`). If all elements are less than `value`, it returns `last`.
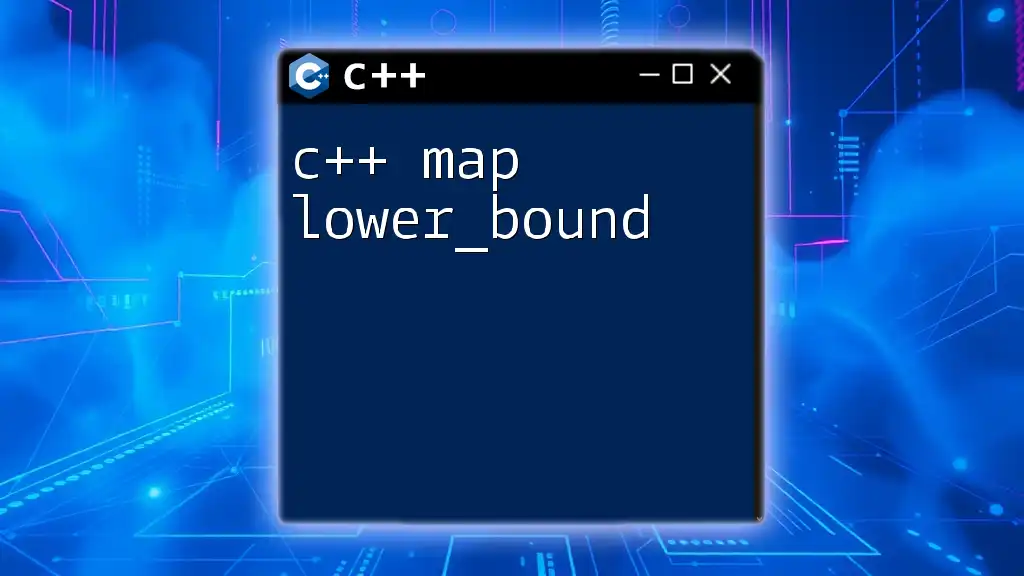
How `lower_bound` Works
To understand how `lower_bound` operates, it's essential to grasp the concept of sorted ranges. The function employs a binary search algorithm, which ensures that the search is efficient. Binary search works by repeatedly dividing the search interval in half, which economizes the number of comparisons required to find the target value.
Comparing Elements
When using `lower_bound`, the elements must be comparable. The comparison operations must maintain a strict ordering, as `lower_bound` relies on being able to determine where the provided `value` fits in the existing order.
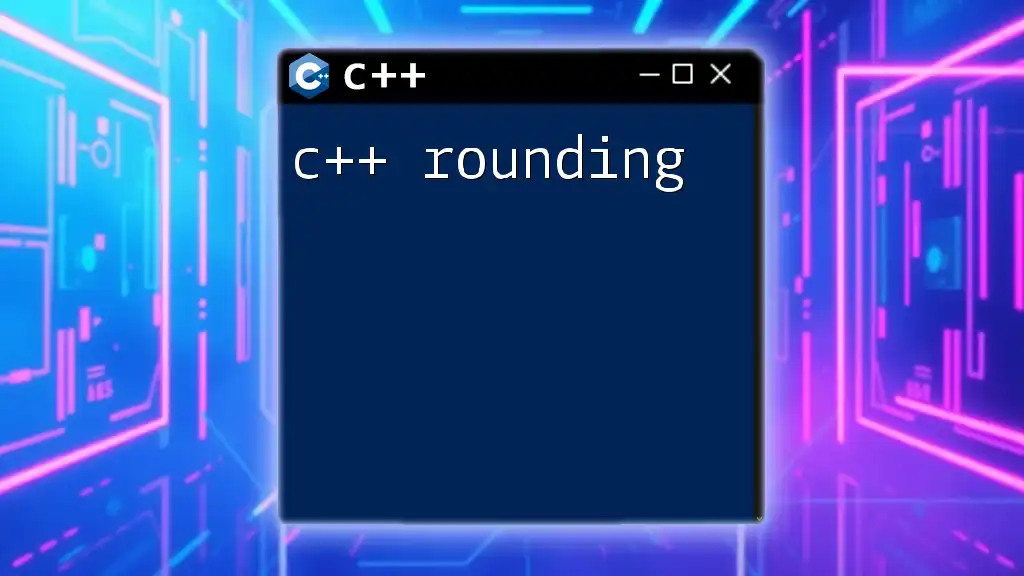
Parameters Breakdown
Range Parameters
-
`first`: This points to the beginning of the range to be searched. If it is an invalid iterator, the behavior of `lower_bound` becomes undefined.
-
`last`: This indicates the end of the range (non-inclusive). This must also be a valid iterator, and `last` should always be greater than or equal to `first`.
Value Parameter
- `value`: This is the target value you are searching for within the range. The function will find the position of the first element that is greater than or equal to this value.
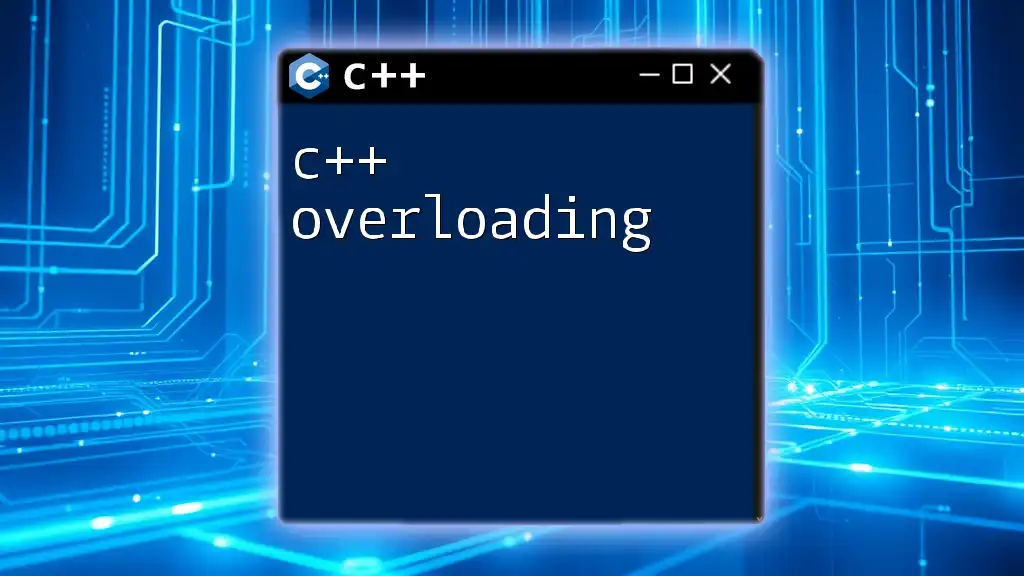
Return Value
The return value of `lower_bound` is crucial for determining the success of your search:
- If `value` is found, the iterator points directly to it.
- If `value` is not present in the collection, it points to the next higher element if any exists; otherwise, it returns `last`.
Example
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 4, 5, 6};
auto it = std::lower_bound(vec.begin(), vec.end(), 4);
if (it != vec.end()) {
std::cout << "Element found: " << *it; // Output: Element found: 4
}
}
In this example, `lower_bound` searches for the value `4` in the sorted vector `vec`. It successfully finds the value and prints it out.
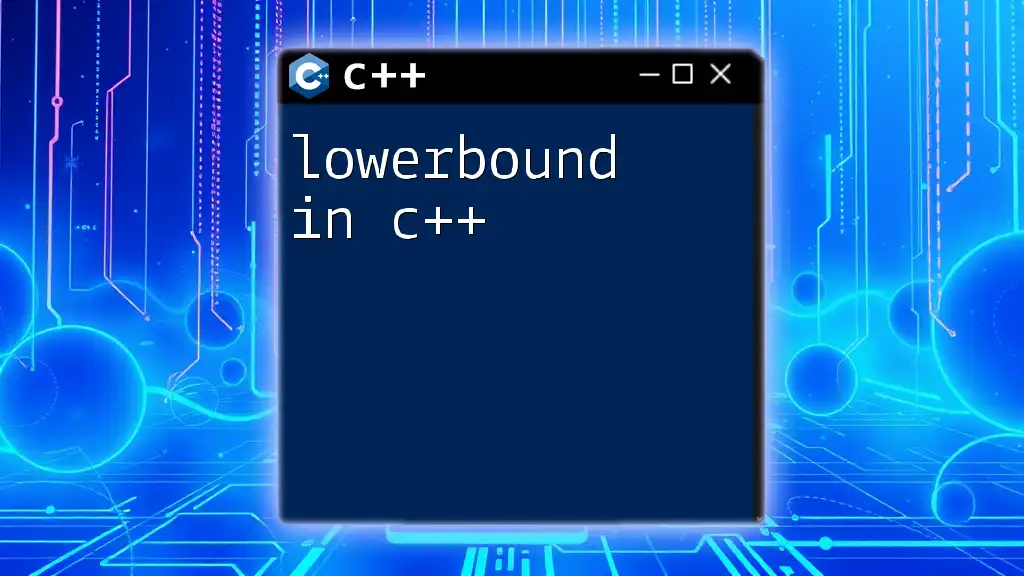
Examples of Using `lower_bound`
Basic Example
To illustrate its usage, here’s a simple snapshot with a sorted vector.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
auto it = std::lower_bound(vec.begin(), vec.end(), 30);
std::cout << "The position of 30 is: " << (it - vec.begin()); // Output based on index
}
Advanced Example
You can also utilize `lower_bound` with custom types. Below, we define a structure and employ a comparator function.
#include <iostream>
#include <vector>
#include <algorithm>
struct Person {
std::string name;
int age;
};
bool compareByAge(const Person& a, const Person& b) {
return a.age < b.age;
}
int main() {
std::vector<Person> people = {{"Alice", 30}, {"Bob", 25}, {"Charlie", 35}};
std::sort(people.begin(), people.end(), compareByAge);
Person searchPerson = {"Unknown", 28};
auto it = std::lower_bound(people.begin(), people.end(), searchPerson, compareByAge);
std::cout << "Found person aged: " << it->age; // Output: Found person aged: 30
}
In this more complex example, we define a `Person` struct and use it with `lower_bound`. The comparator function allows us to search based on age.
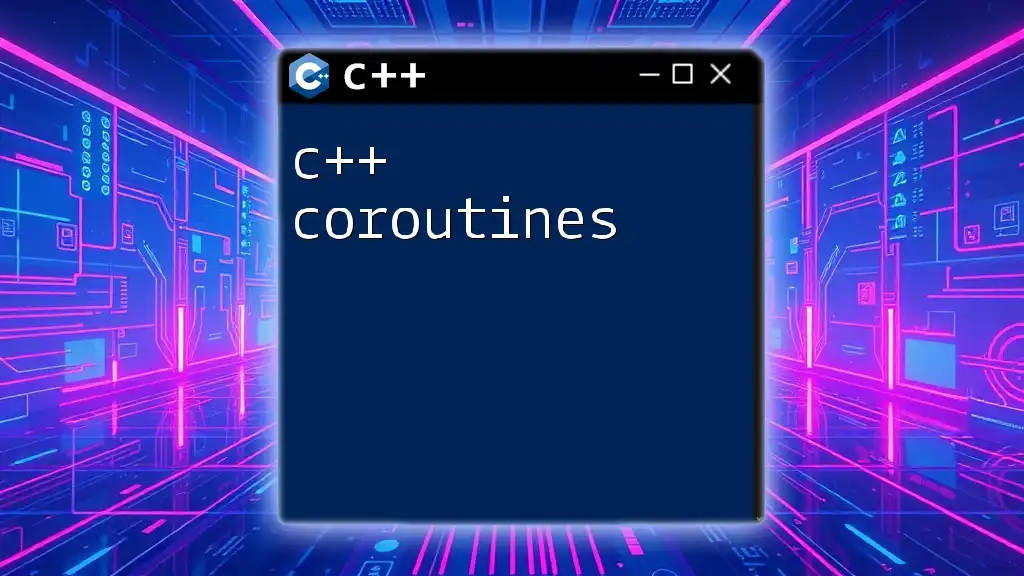
Performance Considerations
When considering performance, the `lower_bound` function has a time complexity of O(log n), as it leverages the binary search algorithm. This efficiency is critical when handling collections with a significant number of elements. The space complexity is minimal, primarily utilizing stack space for recursion in binary search.
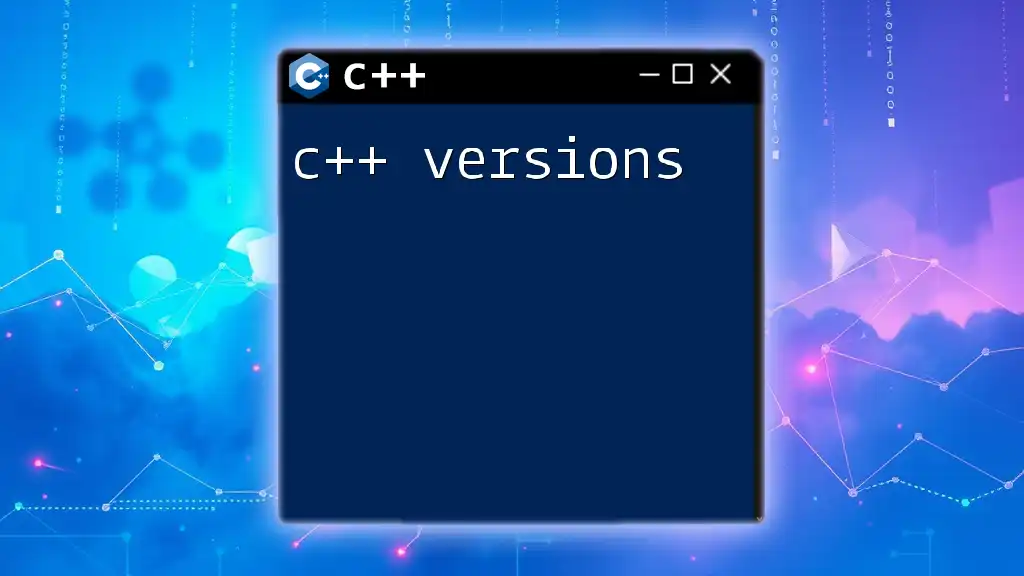
Common Mistakes When Using `lower_bound`
-
Forgetting to Sort: One of the most frequent errors is attempting to use `lower_bound` on an unsorted range. This leads to undefined behavior since the function assumes the data is sorted.
-
Iterator Validity: Another common mistake is using invalid iterators. Always ensure your iterators are valid before passing them to the function.
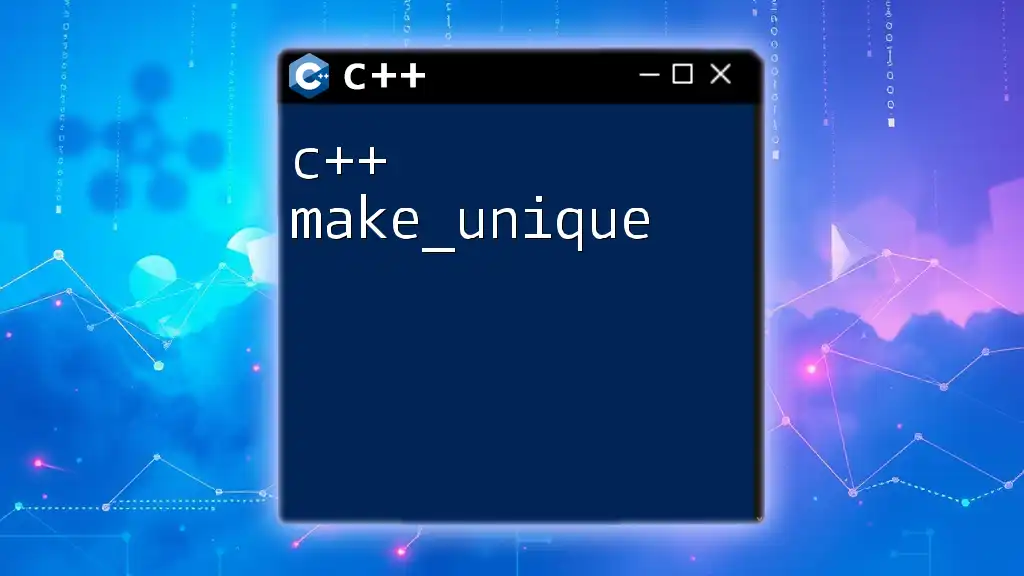
Conclusion
In essence, C++'s `lower_bound` is a vital tool for anyone working with sorted data. Whether you are looking to find a position for insertion or verify the existence of a value, understanding how to effectively use this function can significantly optimize your search operations.
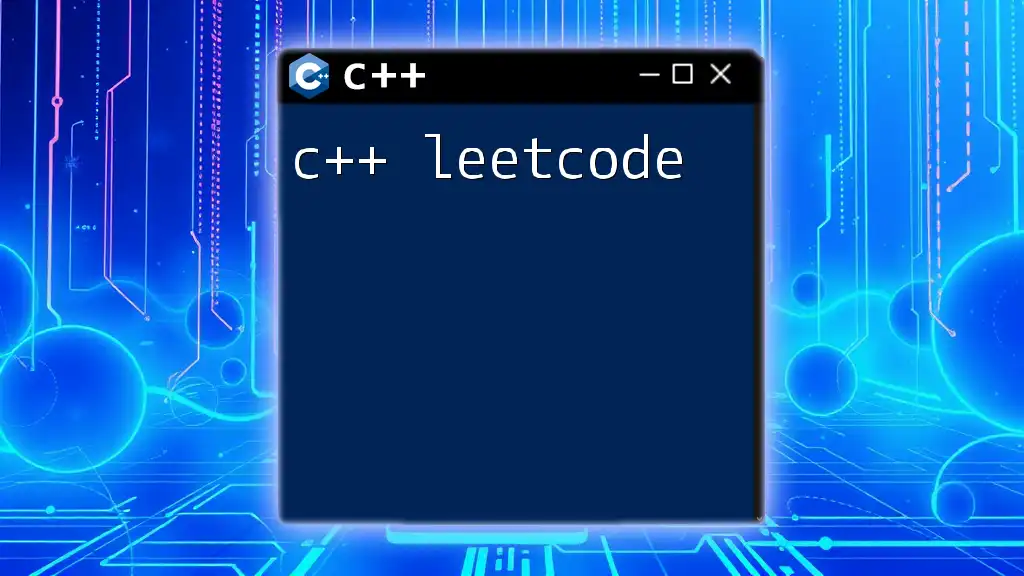
Additional Resources
For more in-depth knowledge, consider checking the official C++ documentation or engaging with online tutorials that teach the practical applications of `lower_bound`. Solve practice problems that involve sorted data to strengthen your understanding.
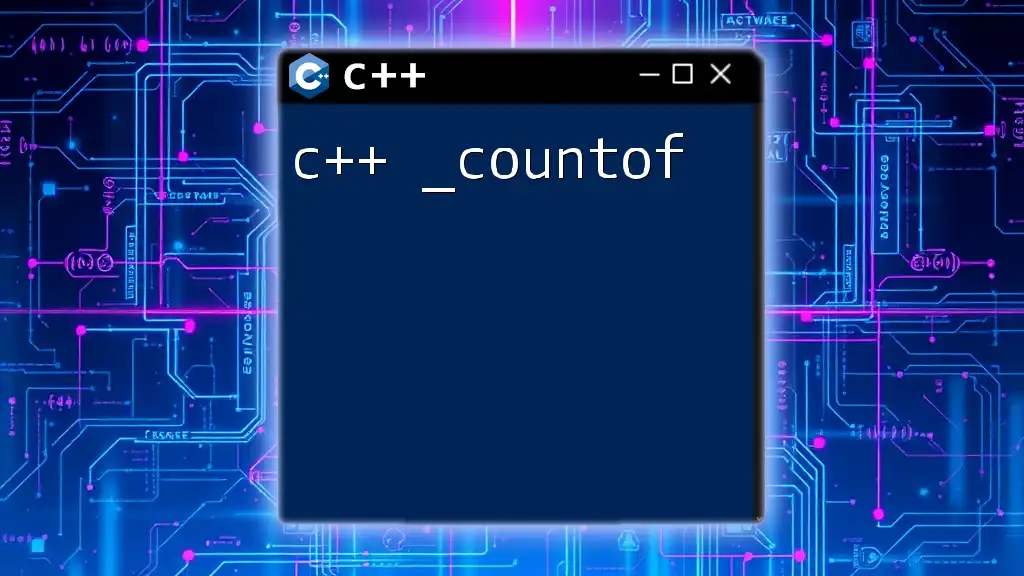
FAQs
What happens if the range is not sorted?
If the range provided to `lower_bound` is not sorted, the results are undefined, potentially leading to incorrect behavior or results.
Can I use `lower_bound` with non-primitive types?
Yes! You can use `lower_bound` with user-defined types or structures, provided that you implement a suitable comparison function.
How can I combine `lower_bound` with other algorithms?
`lower_bound` can complement other algorithms such as `upper_bound`, `binary_search`, or sort functions to create efficient search-and-compare operations in your programs.
This comprehensive guide underscores the importance of `lower_bound` in C++, empowering beginners and experienced developers alike to harness the full potential of this utility in their programming endeavors.