In C++, you can convert a lowercase character to uppercase using the `toupper()` function from the `<cctype>` library. Here’s a code snippet demonstrating this:
#include <iostream>
#include <cctype>
int main() {
char lower = 'a';
char upper = toupper(lower);
std::cout << "Uppercase: " << upper << std::endl; // Output: Uppercase: A
return 0;
}
Understanding Character Cases in C++
What are Character Cases?
In C++, character cases refer to the distinction between lowercase letters (e.g., `a`, `b`, `c`) and uppercase letters (e.g., `A`, `B`, `C`). Each character in the English alphabet has a corresponding representation in the ASCII (American Standard Code for Information Interchange) table, which assigns different integer values to lowercase and uppercase letters. For example, the ASCII value for `a` is 97, while for `A`, it is 65.
Importance of Case Sensitivity
C++ is a case-sensitive programming language, meaning that variable names, identifiers, and function names can differ only by their case. For example, `myVariable`, `MyVariable`, and `MYVARIABLE` are considered three distinct identifiers. This case sensitivity affects how programmers declare and use variables, leading to potential errors if case is not consistently applied.

Common Scenarios for Case Conversion
Data Input Normalization
One common scenario for converting strings from lowercase to uppercase in C++ is normalizing user input. For instance, when users enter their names, the input might be inconsistent in terms of case. By converting all names to a consistent uppercase format, you can ensure clarity and uniformity in the data.
Formatting Output
Another significant use of converting lowercase to uppercase is in output formatting. For example, if you're displaying titles or headings, transforming the text to uppercase can enhance readability and impact, making titles stand out.
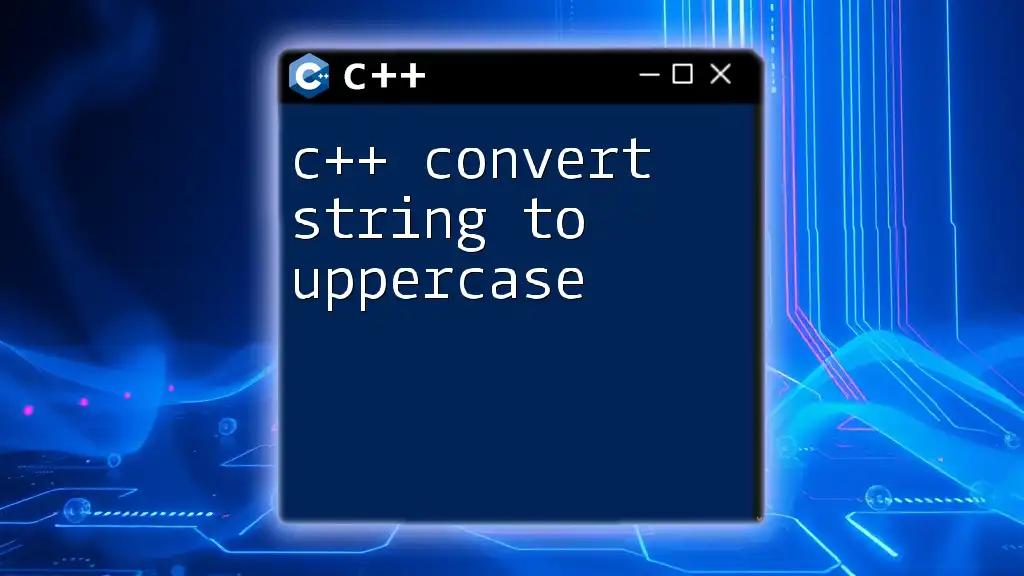
Methods to Convert Lowercase to Uppercase
Using the `toupper()` Function
Explanation
The `toupper()` function is provided by the `<cctype>` library in C++. It is used to convert a single character from lowercase to uppercase. If the character is already uppercase or not an alphabetical character, `toupper()` returns the character unchanged.
Example Code Snippet
#include <iostream>
#include <cctype>
int main() {
char lowerChar = 'a';
char upperChar = toupper(lowerChar);
std::cout << "Lowercase: " << lowerChar << " => Uppercase: " << upperChar << std::endl;
return 0;
}
In this example, the lowercase character `a` is converted to uppercase using `toupper()`, demonstrating its basic functionality.
Converting Entire Strings
Using a Loop
Explanation
To convert an entire string from lowercase to uppercase, we can iterate through each character within the string and apply the `toupper()` function in a loop. This method is straightforward and provides a clear understanding of how character manipulation works in C++.
Example Code Snippet
#include <iostream>
#include <cctype>
#include <string>
int main() {
std::string lowerStr = "hello world";
for (char &c : lowerStr) {
c = toupper(c);
}
std::cout << "Converted String: " << lowerStr << std::endl;
return 0;
}
In this snippet, each character in the string `lowerStr` is converted to uppercase using a range-based `for` loop. The modification is done in place since we pass `c` as a reference.
Using `std::transform`
Explanation
Another efficient way to convert an entire string to uppercase is using the `std::transform` function, part of the `<algorithm>` library. This approach leverages the power of the Standard Template Library (STL) and can be more concise than manually looping through the string.
Example Code Snippet
#include <iostream>
#include <string>
#include <algorithm>
#include <cctype>
int main() {
std::string lowerStr = "hello world";
std::transform(lowerStr.begin(), lowerStr.end(), lowerStr.begin(), ::toupper);
std::cout << "Converted String: " << lowerStr << std::endl;
return 0;
}
In this example, `std::transform` takes four parameters: the beginning and end of the input range, the beginning of the output range (which is the same as the input range in this case), and the conversion function. Here, we use `::toupper` to transform each character of `lowerStr`.

Performance Considerations
Time Complexity
When analyzing the time complexity of these conversion methods, we see that both the loop method and `std::transform` operate in O(n) time, where n is the number of characters in the string. However, using built-in algorithms like `std::transform` is often preferred for performance reasons and readability, as they are optimized for common operations.
Memory Usage
When performing string manipulations, it’s important to remember that strings in C++ are dynamic arrays. As such, memory usage should be considered, especially when dealing with large datasets. Operations that modify the string must factor in potential reallocations, particularly if the original string capacity is insufficient.
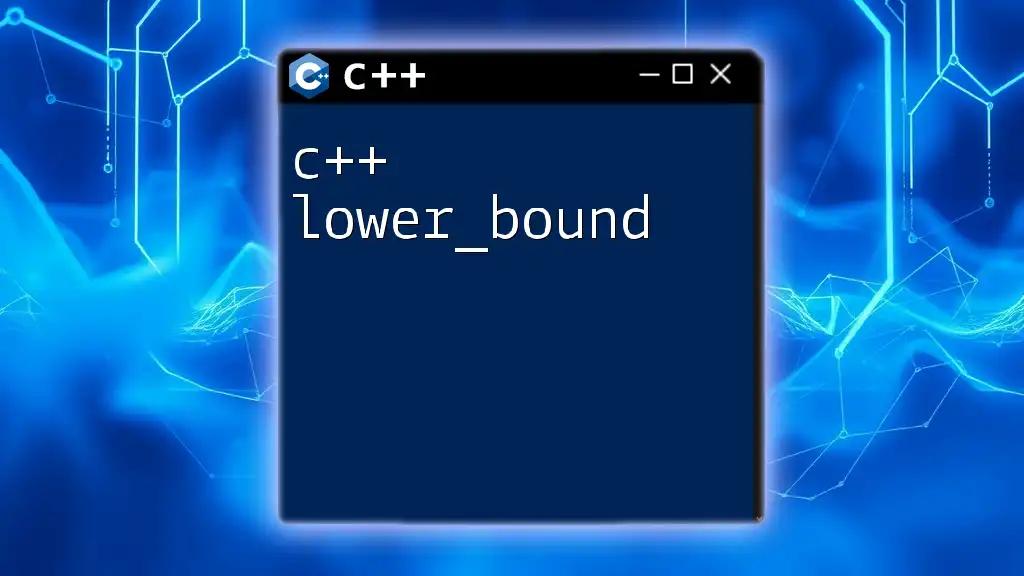
Error Handling
Validating Input Data
Before performing any case conversion, it’s smart to validate the input data. For instance, checking if a string is empty can prevent unnecessary operations and possible errors downstream.
if (!lowerStr.empty()) {
// Perform conversion
} else {
std::cout << "String is empty!" << std::endl;
}
This snippet effectively checks for an empty string and avoids conversion logic if the input lacks content.
Handling Special Characters
In certain applications, you may want to skip special characters or numbers during case conversion. The conversion functions like `toupper()` can seamlessly ignore non-alphabetic characters, but ensuring that your logic correctly manages such situations is important to maintain data integrity.

Best Practices
When to Convert Cases
Consider the context before converting cases in your application. While converting lower to upper is useful for ensuring uniformity, unnecessary conversions could lead to performance overhead. Engaging in case conversion should be a deliberate decision based on user input expectations and output requirements.
Keeping Code Readable and Maintainable
When converting string cases in your C++ programs, strive to write clear and maintainable code. This includes using informative variable names, adding comments explaining the logic, and utilizing functions like `std::transform` whenever possible to enhance code readability.
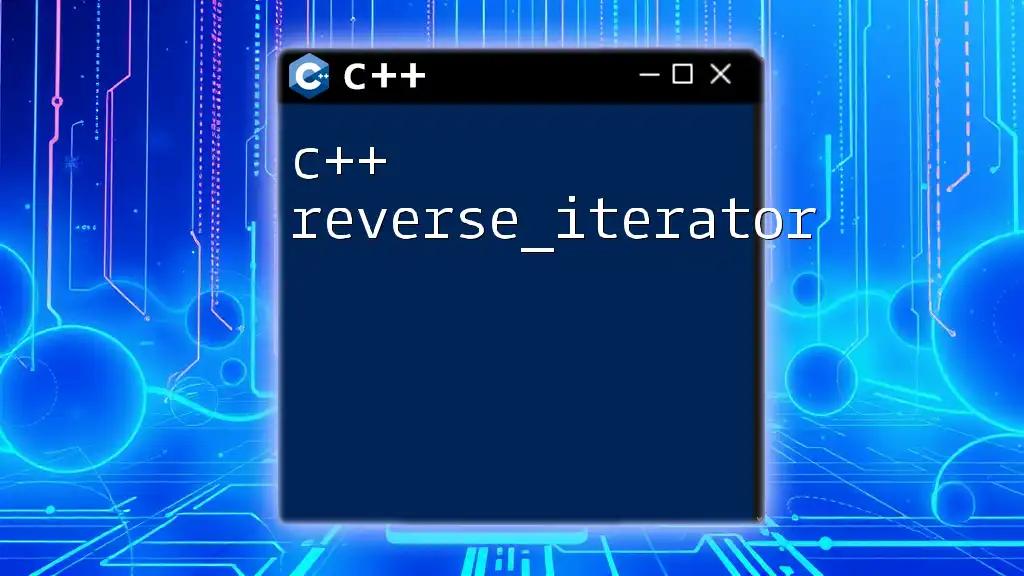
Conclusion
Mastering the conversion from c++ lowercase to uppercase is essential for effective string manipulation and programming in general. Understanding the various methods—such as using `toupper()`, loops, or `std::transform`—empowers you to enhance data integrity and output formatting in your programs. As you practice these techniques, you will gain greater confidence in handling string manipulations in C++.
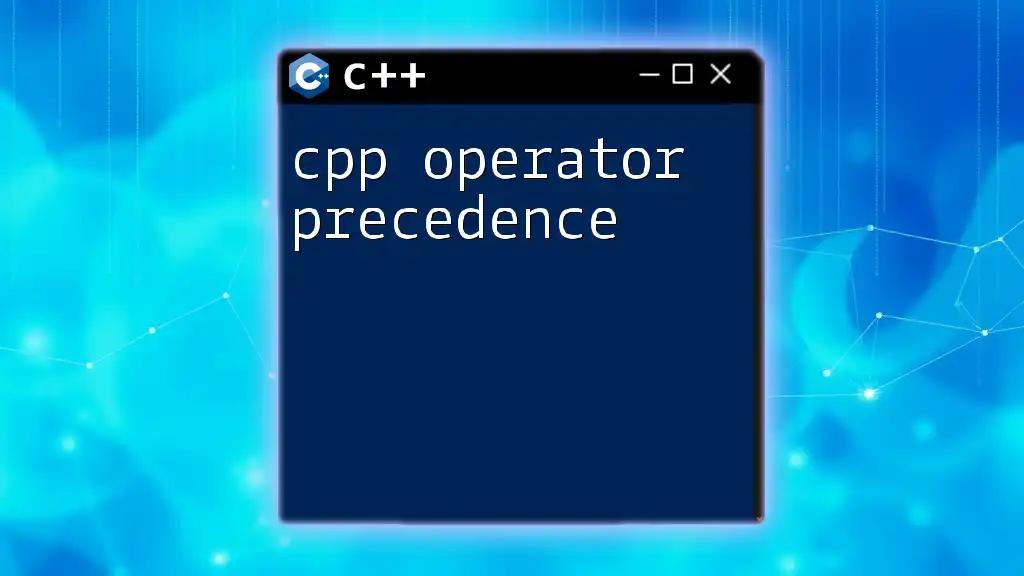
Call to Action
It's time to apply what you've learned! Experiment with the C++ methods discussed here to convert lowercase to uppercase, and don't hesitate to explore further tutorials on string manipulations and related C++ functions for an even deeper understanding.
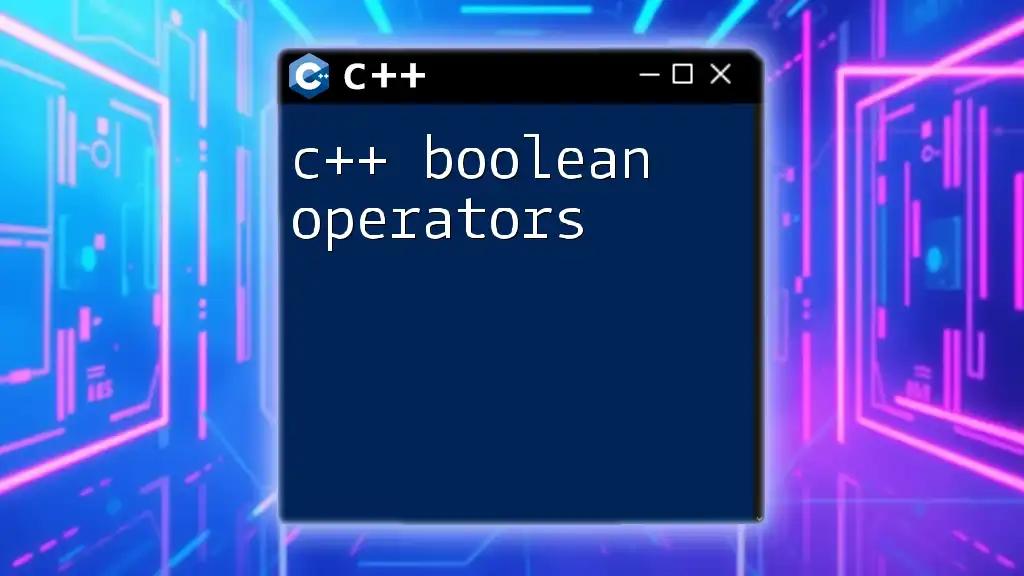
Additional Resources
For further reading, check out the official C++ documentation, focused tutorials on string manipulation, and community resources that delve into character functions and their applications in C++. This knowledge will not only enhance your programming skills but also broaden your understanding of C++ as a robust language for software development.